Sending Email with Attachments using SendGrid and Node.js
Time to read: 2 minutes
You're sending emails from your Node.js app with the Twilio SendGrid API. Now you want to attach files to your emails. The Twilio SendGrid API makes it very straightforward to include attachments to the emails that you send. In this post, we’ll attach a pdf document to an email sent via SendGrid. If you have not sent your first email with SendGrid, my colleague Sam has written a post about it, or if you prefer video my colleague Brent has published a video about it. We’ll be picking up where that post ended.
Prerequisites
Before we begin, make sure you have the following setup:
- Node.js and npm installed (do this first if you haven't already)
- A Free Twilio SendGrid Account
- The Twilio SendGrid Node Library installed in your project
Setup
If you haven’t done so already, sign up for a free Sendgrid account.You can use the free tier for this tutorial. Once you have your account, create an API Key. You can call it whatever you want, but make sure to hold on to it for later.
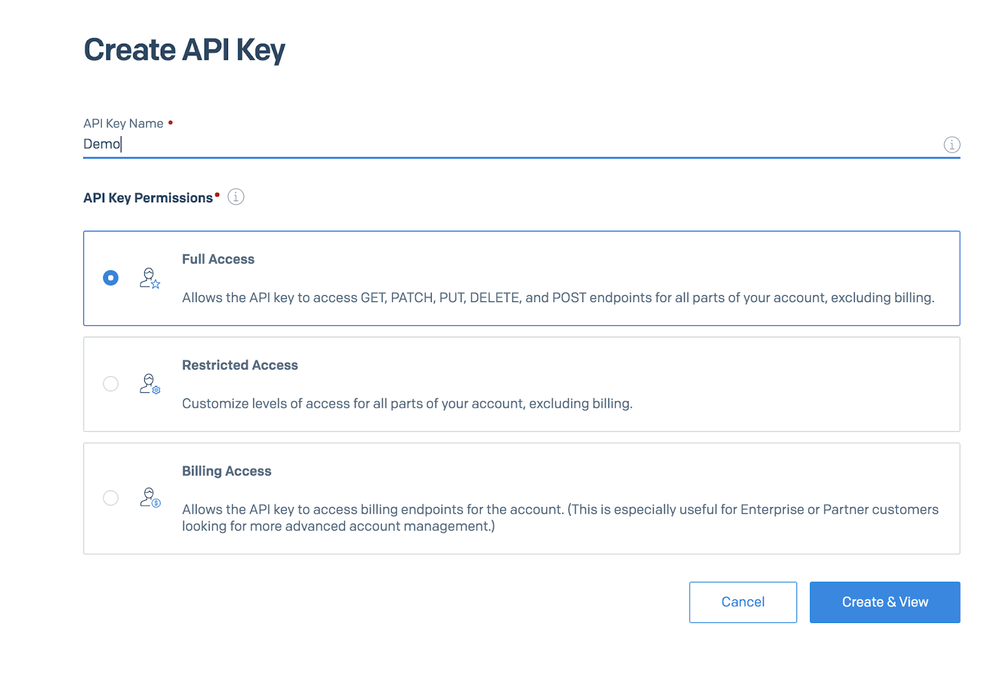
A good way to save this API key is to set it as an environment variable that you can access from your JavaScript code in order to avoid writing it directly in your code. Set the value of the SENDGRID_API_KEY
environment variable to be the API key from your SendGrid account. Here's a useful tutorial if you need help setting environment variables. We will use this later on.
Sending an Email
Let’s pick up where my colleague’s blog post left off, sending an email. You should have an index.js
file that looks like this:
Make sure you have the Twilio Sendgrid Node Library installed. If you haven’t run this command in your project folder.
We’ll be using the Node File System API to read our file and encode it as a base64 string. I have used the fictional attachment.pdf
file, so make sure to replace the name of the file with a document that you have stored in the same folder as your index.js
file.
Let’s add an attachment property to our msg
object. We can pass an array of attachment objects to quickly send multiple attachments, but for now let’s just send a single attachment.pdf
.
Before running this code, make sure you have the SENDGRID_API_KEY environment variable set, and remember to replace the to
value with your own email address to verify that your code worked correctly.
Finally, save your code and then in your terminal run the following command to send yourself an email:
Check your inbox and you should see something like this!
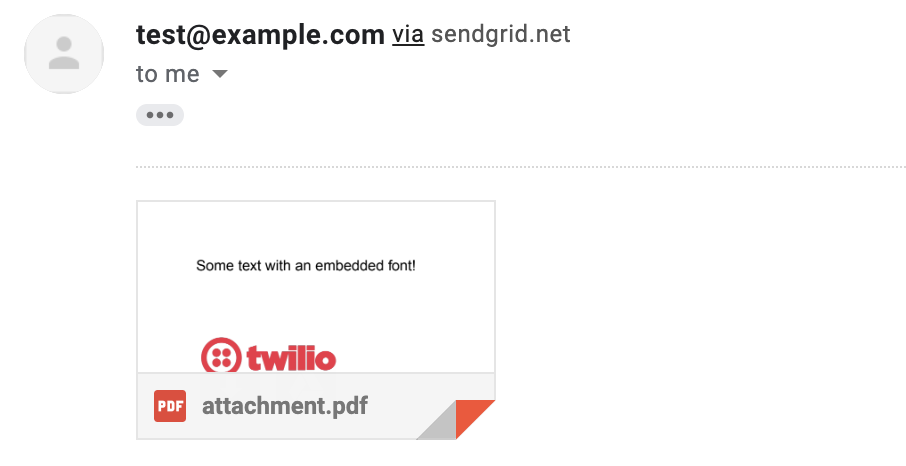
Wrapping Up
Now you can add attachments to the emails you send with Twilio SendGrid, but you can do so much more! You can respond to incoming emails using SendGrid's Inbound Email Parse Webhook, integrate it into a smart Facebook Messenger Bot or even forward incoming emails as a text.. You can also check out the SendGrid docs for a ton of other cool features and uses.
Feel free to reach out to me with any questions or to talk about the amazing things you are building. I can’t wait to see what you build!
- Twitter: @ChatterboxCoder
- Instagram: @ChatterboxCoder
- Email: nokenwa@twilio.com
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.