Get SMS Alerts with Twilio When GitHub Actions Fail
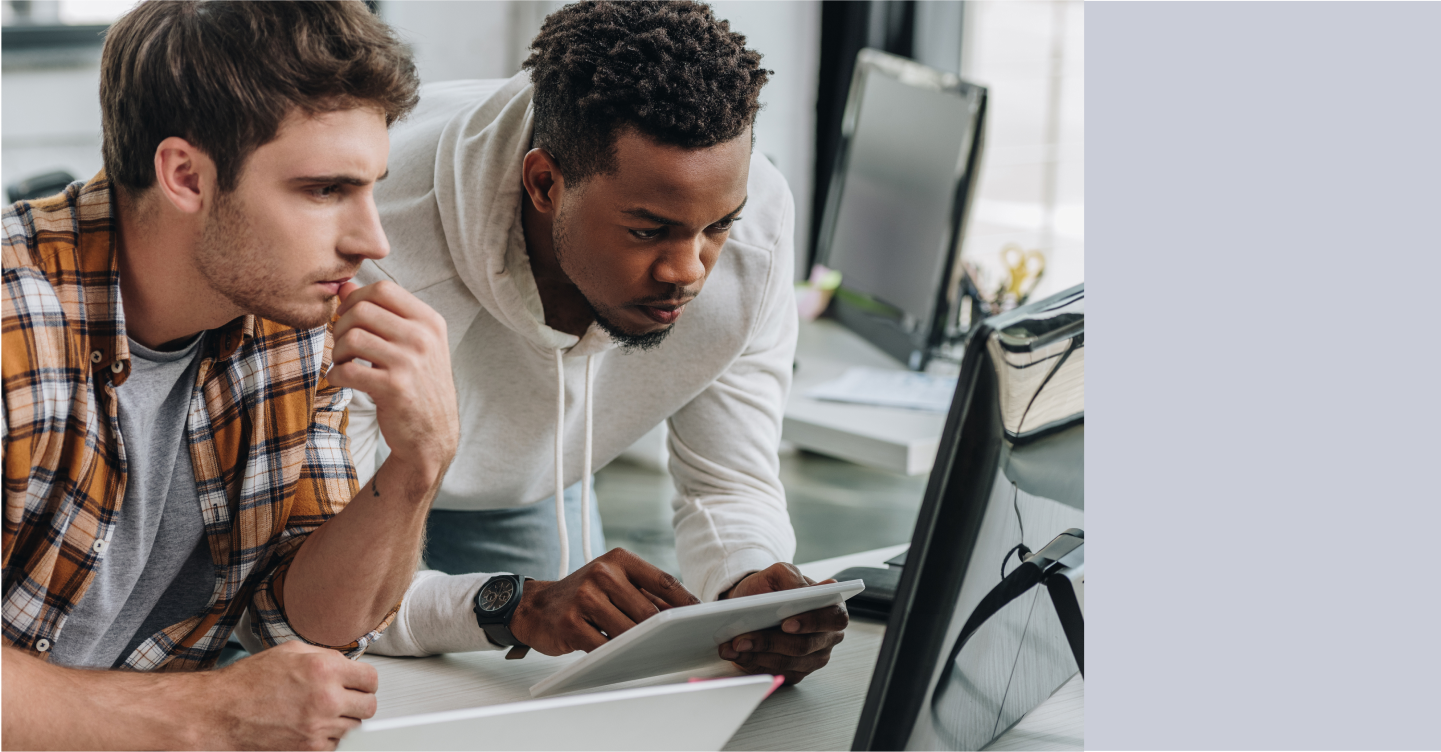
Time to read: 3 minutes
GitHub has a native feature that sends an email when a GitHub Action fails. It works when you are working on an individual project, however, I wanted to receive SMS alerts on my phone if any of the CI/CD actions for my personal website failed.
In this blog post, you’ll see how you can build a workflow that allows you to achieve this.
Prerequisites
What you’ll need for this tutorial:
- A Twilio Account (Sign up for free)
- A Twilio phone number
- A Twilio API Key and Secret
- GitHub repository for GitHub Actions & YAML
Creating a sample workflow
Let’s write a simple GitHub Actions workflow that prints the infamous “Hello World”. Create a .github/workflows
directory in your GitHub repository if this directory does not already exist. In the .github/workflows
directory, create a file named build.yml
. For more information, see the GitHub Actions quickstart guide.
Copy the following YAML contents into the ‘build.yml’ file.
The on.push.branches
means that this GitHub Actions workflow will be triggered each time you push your main branch.
Commit and push your code to GitHub and run the above GitHub Action. This simple action runs and outputs “Hello, world!”
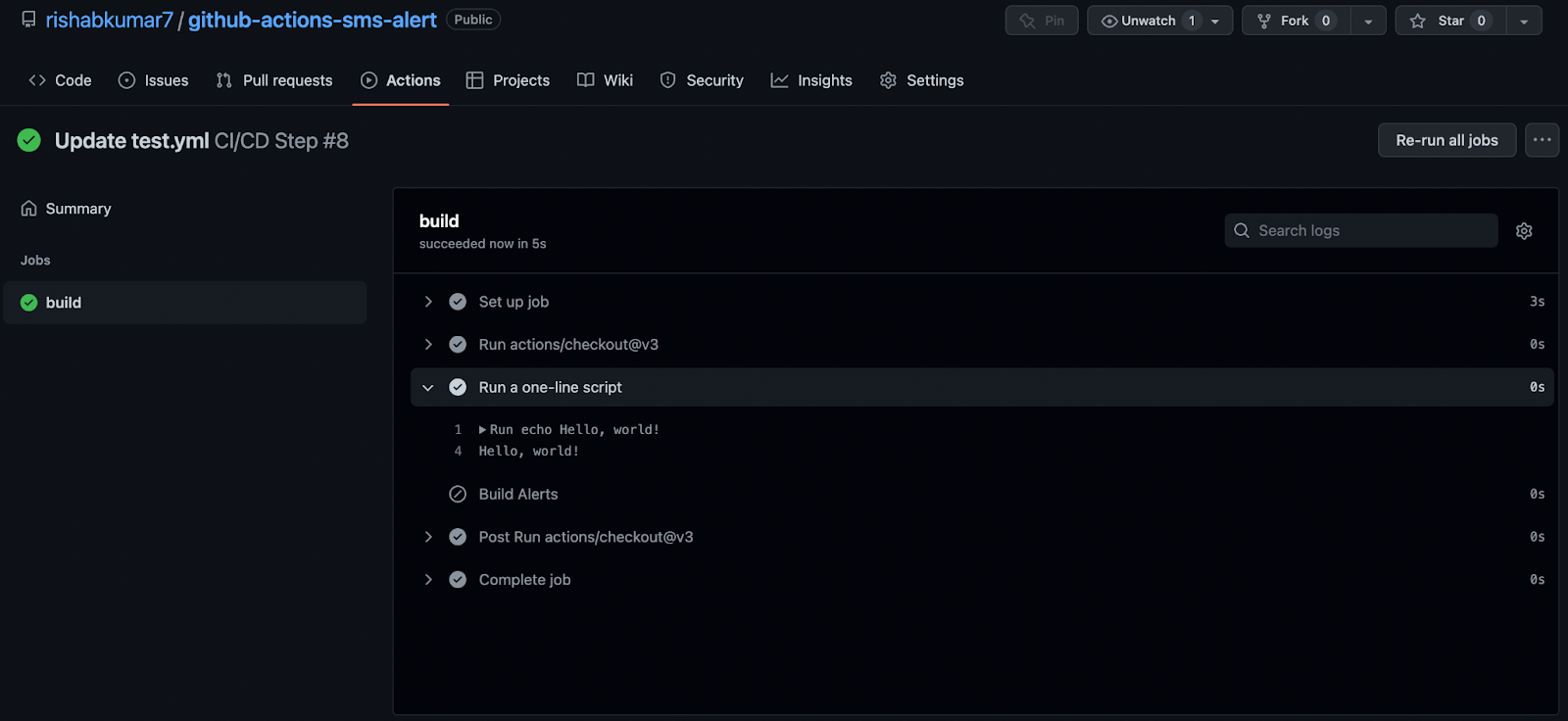
Integrate Twilio SMS GitHub Action
The GitHub Action for Twilio SMS can be found on the GitHub Actions marketplace and it will allow us to send a SMS.
After signing up for a Twilio account, buy a number and after creating an API Key and Secret, we need to store these values as secrets in your repository settings using TWILIO_ACCOUNT_SID
, TWILIO_API_KEY
, and TWILIO_API_SECRET
.
You will also need to add two additional variables:
FROM_NUMBER
TO_NUMBER
FROM_NUMBER
is the Twilio Number that we created in our Twilio account and TO_NUMBER
is our personal number where we want to receive the SMS alerts. In your GitHub repository, under Settings, go to Actions and create the following repository secrets with the New repository secret button.
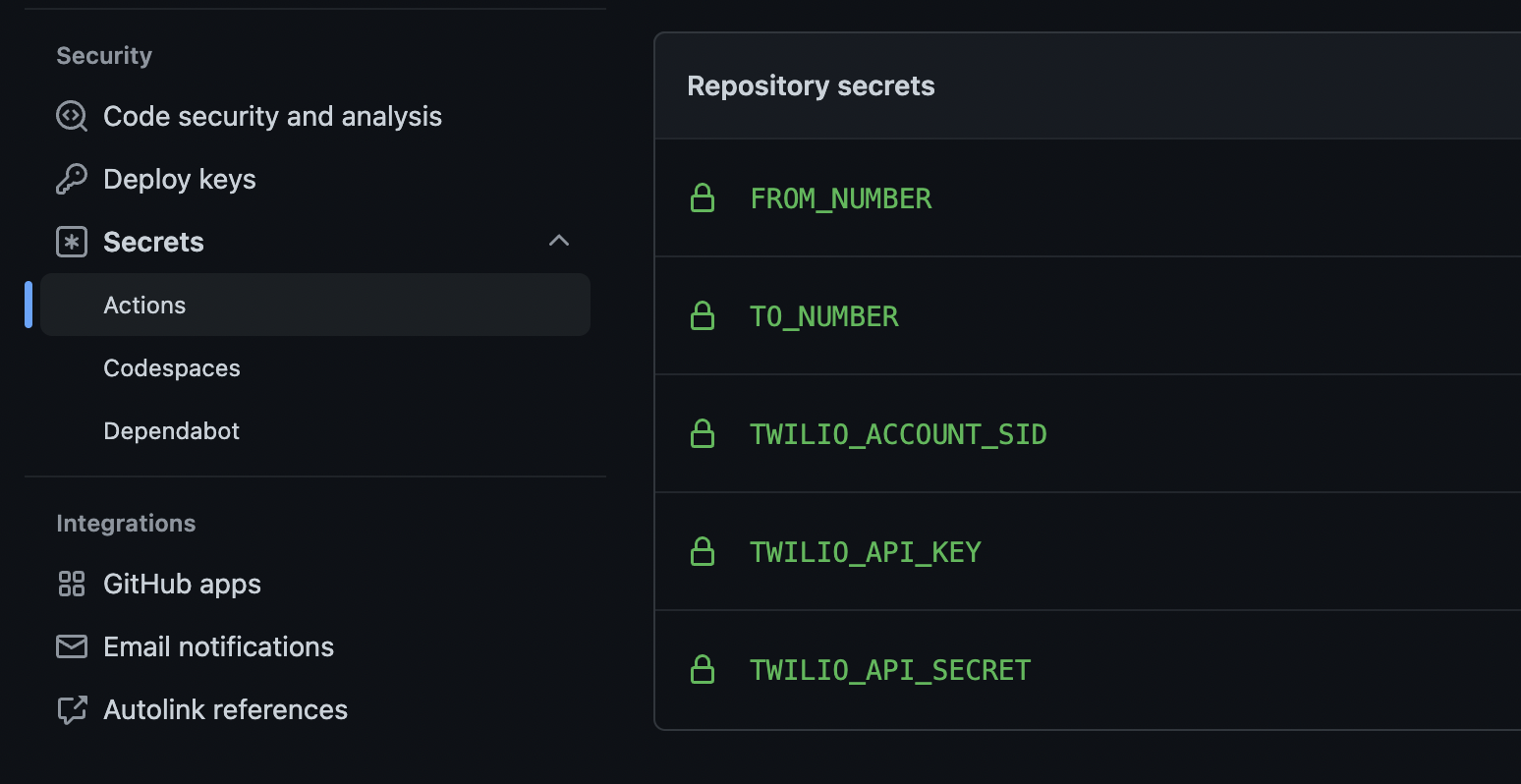
Now, let’s add the following to the end of our workflow file, ‘build.yml’ (GitHub action):
So the above YAML will send us a text message “Hello from Twilio” every time the action runs, which is on every commit/push we make to the main branch. We don’t want to send the text message on successful runs of the CI/CD or Build action, instead we only need the action to alert us if the CI/CD or build action fails. Let’s modify it by pasting the following after the previous snippet.
As you can see, we have an if: ${{ failure() }}
statement which means the Twilio Build Alerts will only run if the build action fails.
Now let’s also change the message body to something more informational.
We have included the GitHub job id and the repository, along with the status of the job for informational purposes..
The complete YAML should look like this:
Testing the SMS alerts
Since the action just says ‘Hello World’ everytime we run it, if it’s a successful run we won’t receive a text message. Let’s set the exit status as 1, which means the action has a failed status. You can read more about GitHub action exit codes here.
As we commit the change we made to the workflow file, the action should be triggered. Let’s check the status of the action:
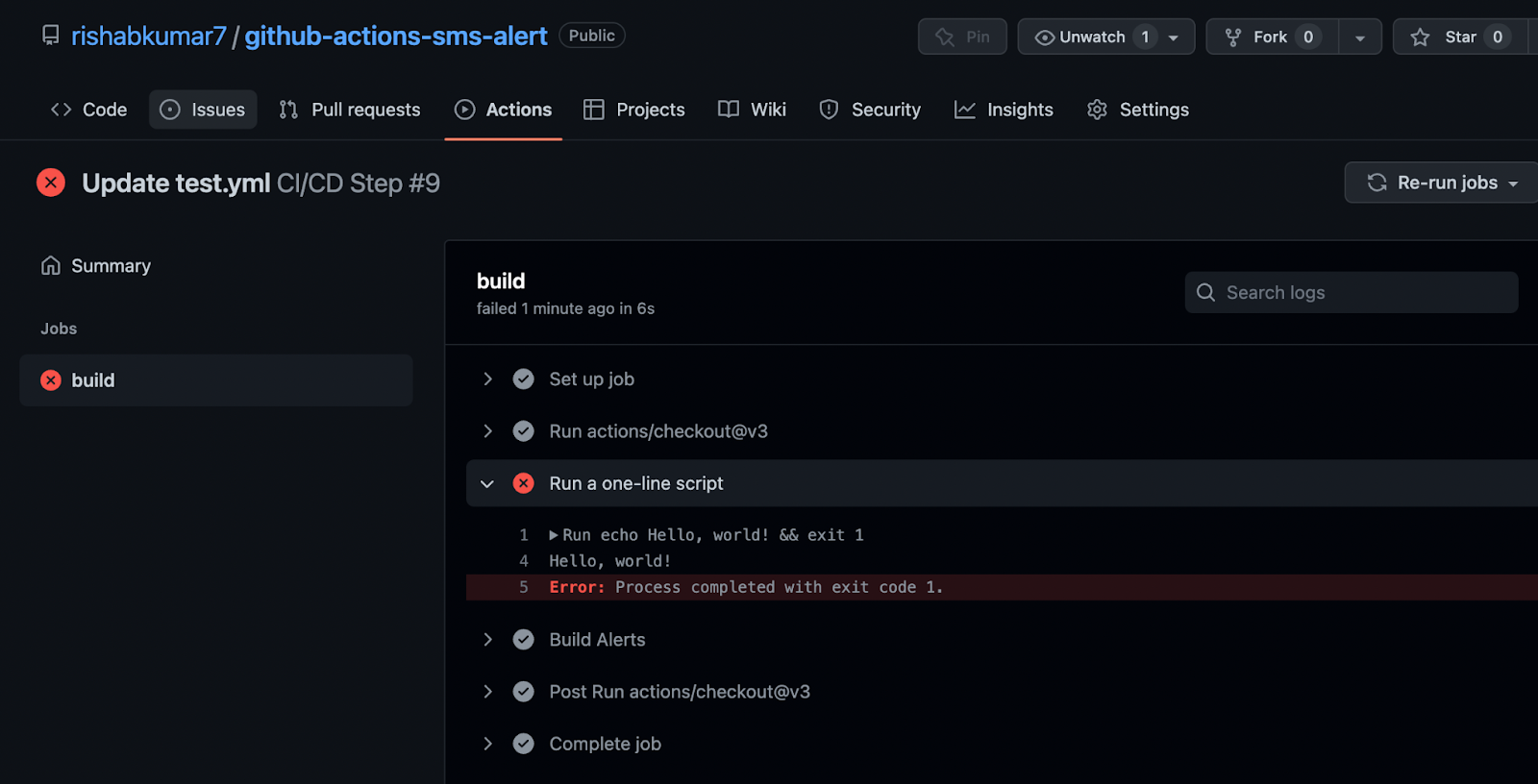
So the job failed, and we received a text alert on the number that we stored in the TO_NUMBER
variable.
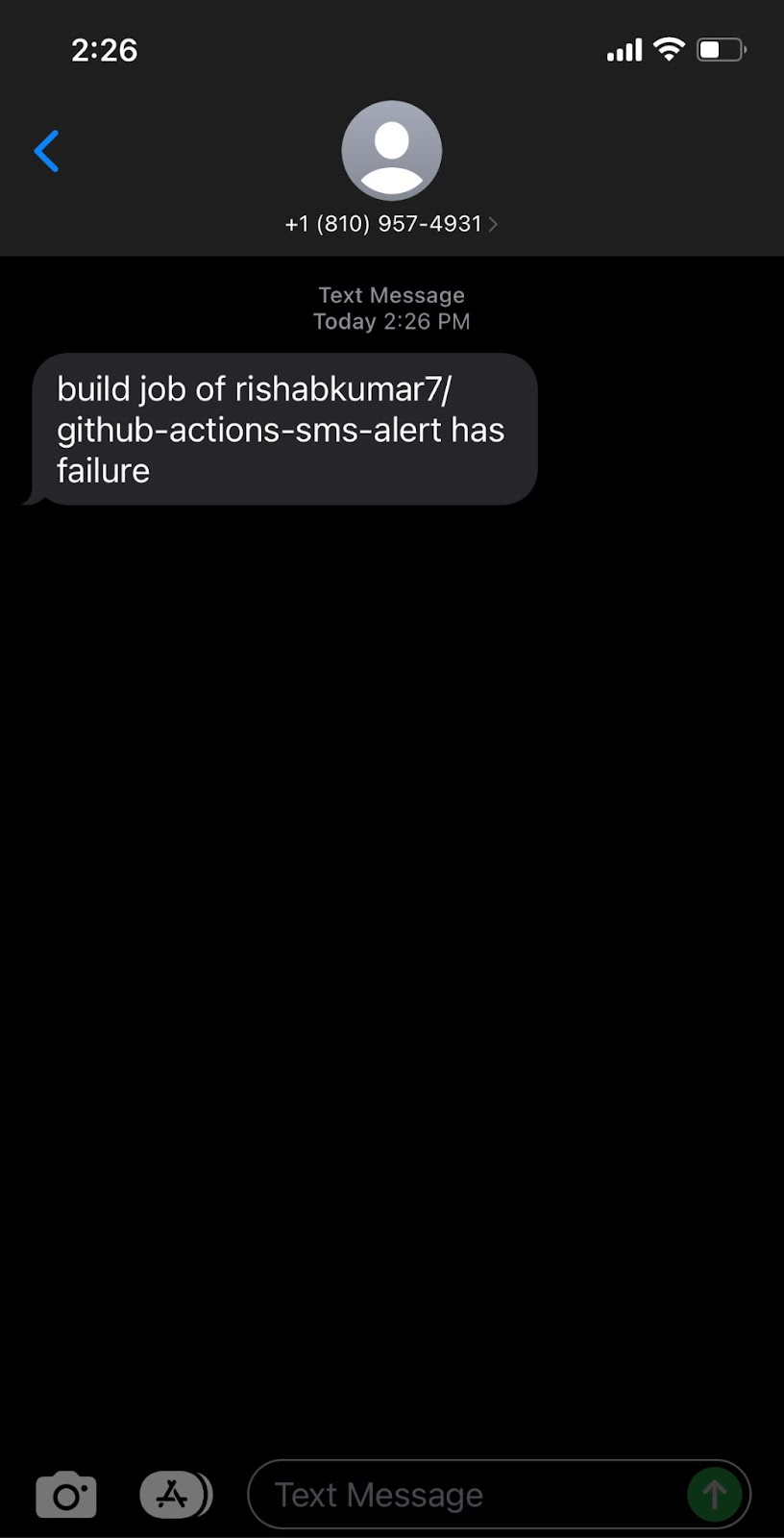
Voila, we have an SMS alert system for our failed GitHub actions.
Show me what you build
Now that you can be alerted on builds that fail in your GitHub projects via GitHub Actions, you can also configure the alerts to be sent when someone opens an issue for the repository/project or creates a pull request!
Thanks so much for reading! I also have a video version of this tutorial available on my YouTube channel. If you found this tutorial helpful, have any questions, or want to show me what you’ve built, let me know. And if you want to learn more about me, check out my intro blog post.
Rishab is a Developer Evangelist at Twilio, is passionate about helping people get into cloud and sharing his learnings from his time in cloud, DevOps and now DevRel, reach out to him at rikumar[at]twilio.com.
Twitter @rishabk7
LinkedIn @rishabkumar7
GitHub @rishabkumar7
Email: rikumar@twilio.com
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.