SMS Forwarding and Responding Using Twilio and JavaScript
Time to read: 3 minutes
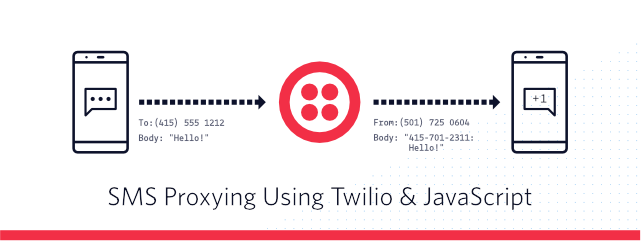
There are a variety of reasons why you sometimes don't want to give out your phone number to people but still want to receive SMS and be able to reply. Maybe you are trying to sell something on the internet, maybe you want to set up a way for people to report code of conduct violations at an event, or maybe you just don't trust the newly met person that asked for your number. In the past I showed how you can quickly set up a message forwarding with practically no coding using TwiML Bins. However, it doesn't let you respond using that number.
Let's take a look at how we can change that using Twilio Functions. If you prefer to follow along a video, check out this tutorial on our YouTube channel.
Creating a Twilio Function
Before we get started, make sure you have a Twilio account. Sign up here for free: www.twilio.com/try-twilio
Once you are logged in, head to the Functions section of the Runtime part of the Twilio Console. Create a new Twilio Function and choose the "Hello SMS" template.
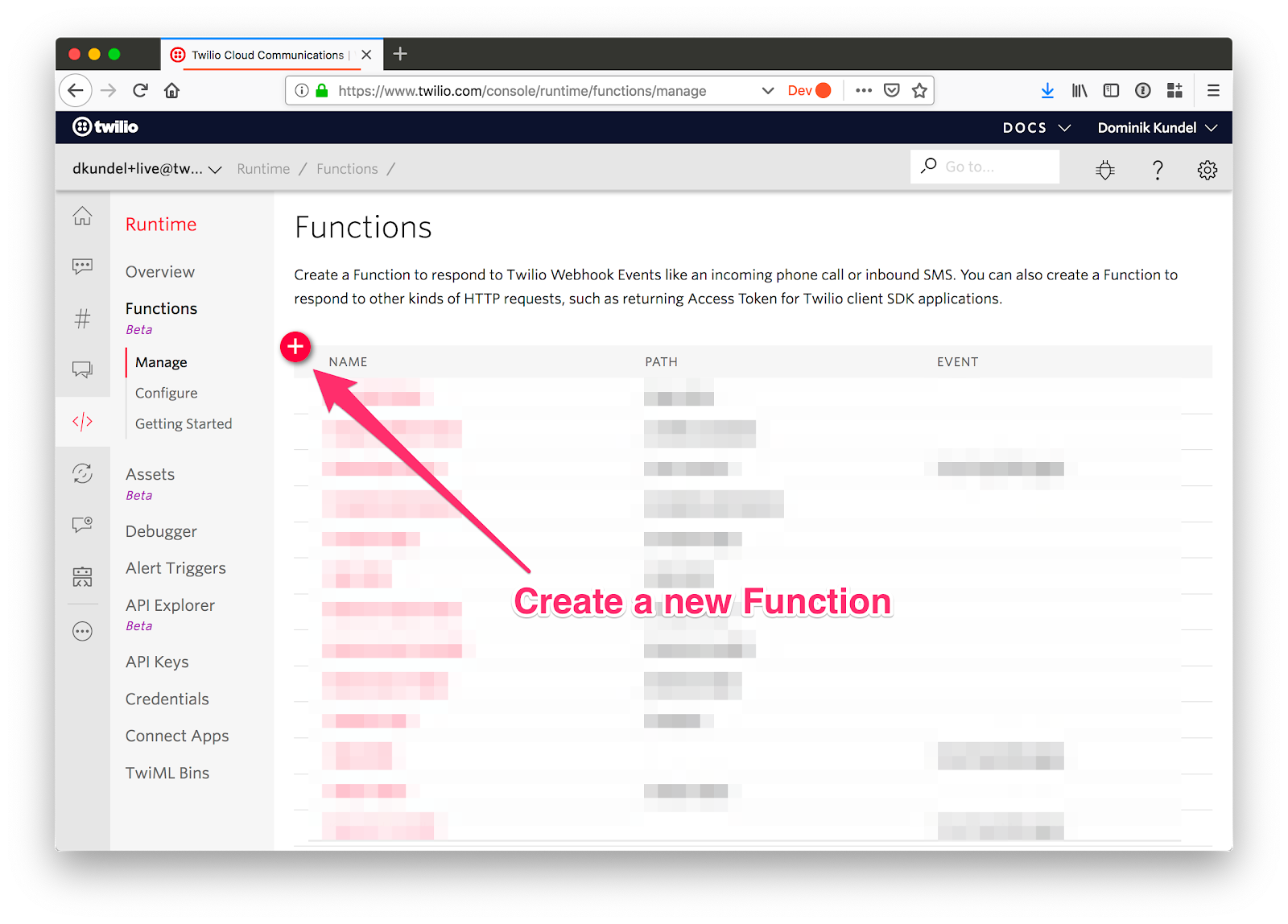
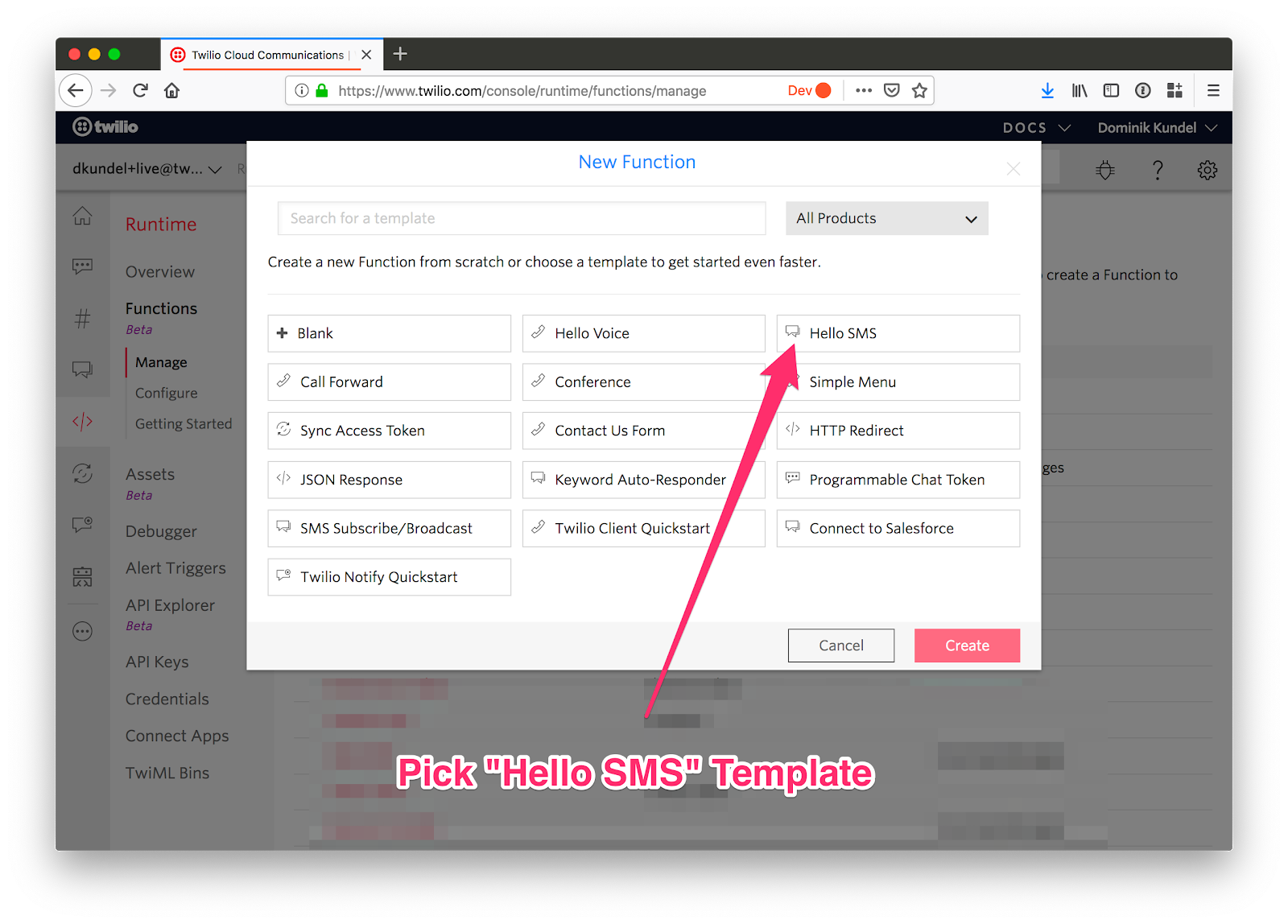
Change the name of the Twilio Function to something that gives you a hint on what it does. I'll name mine "My SMS Forwarder" and give it the path /forward-sms
.
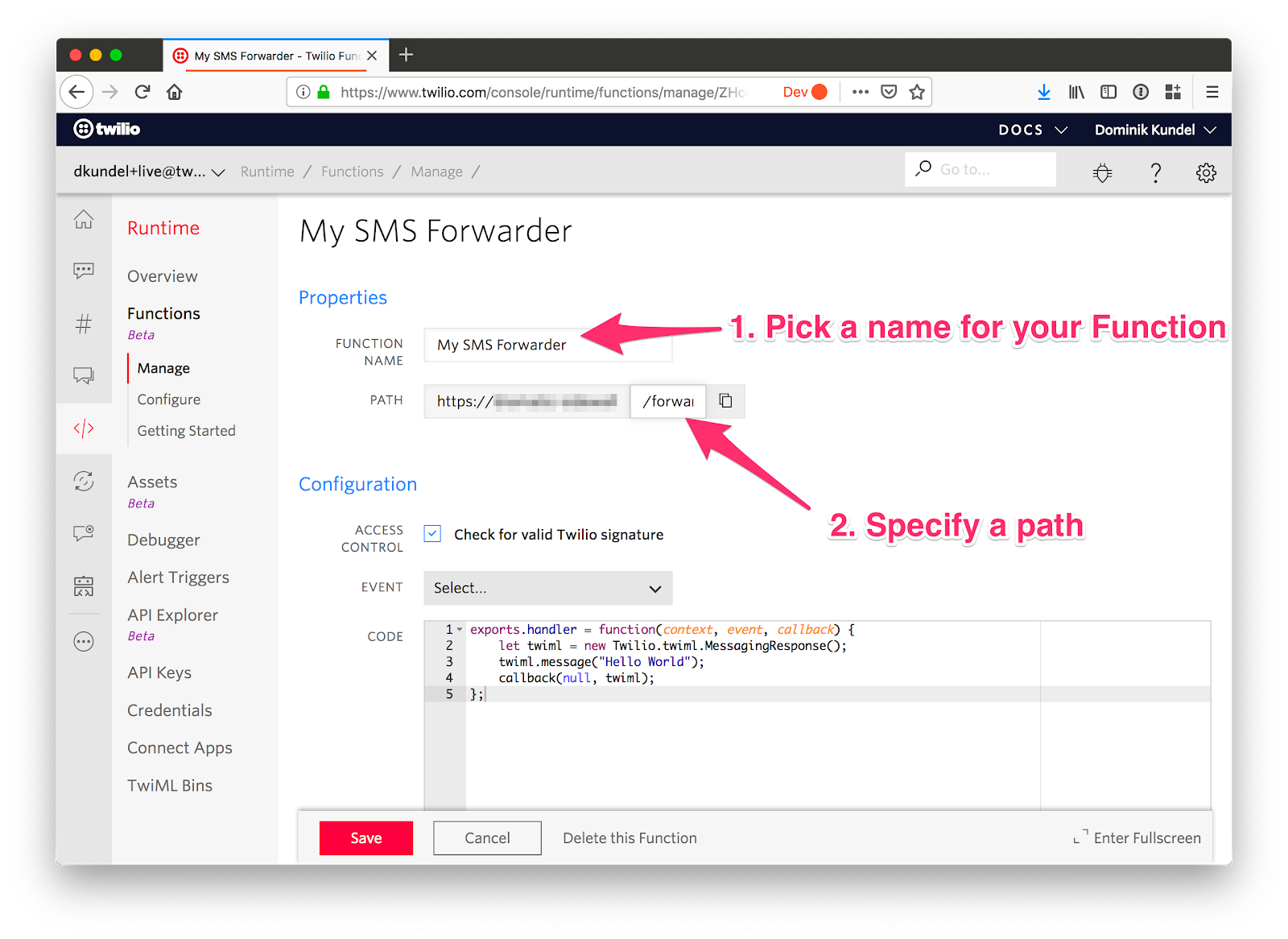
Right now our Function is just an auto-responder returning "Hello World". In order to actually forward SMS similar to our previous blog post, update the code the following way:
Make sure to replace YOUR_NUMBER_HERE
with the phone number you want the incoming SMS forwarded to.
In Twilio Functions we can access webhook data such as the From
number or the message Body
using event.
. The new twiml.message
line will now return the TwiML needed to forward an SMS to the specified number, containing the number of the person who sent it and the original message body.
Save the code by clicking the "Save" button. Head to the Phone Numbers section in the Twilio Console and pick the number that you want to use for SMS forwarding or buy a new one.
Once you are in the configuration screen, scroll to the bottom of the page, and under 'A message comes in' select the value "Function", and then the name of your Function. In my case that's "My SMS Forwarder".
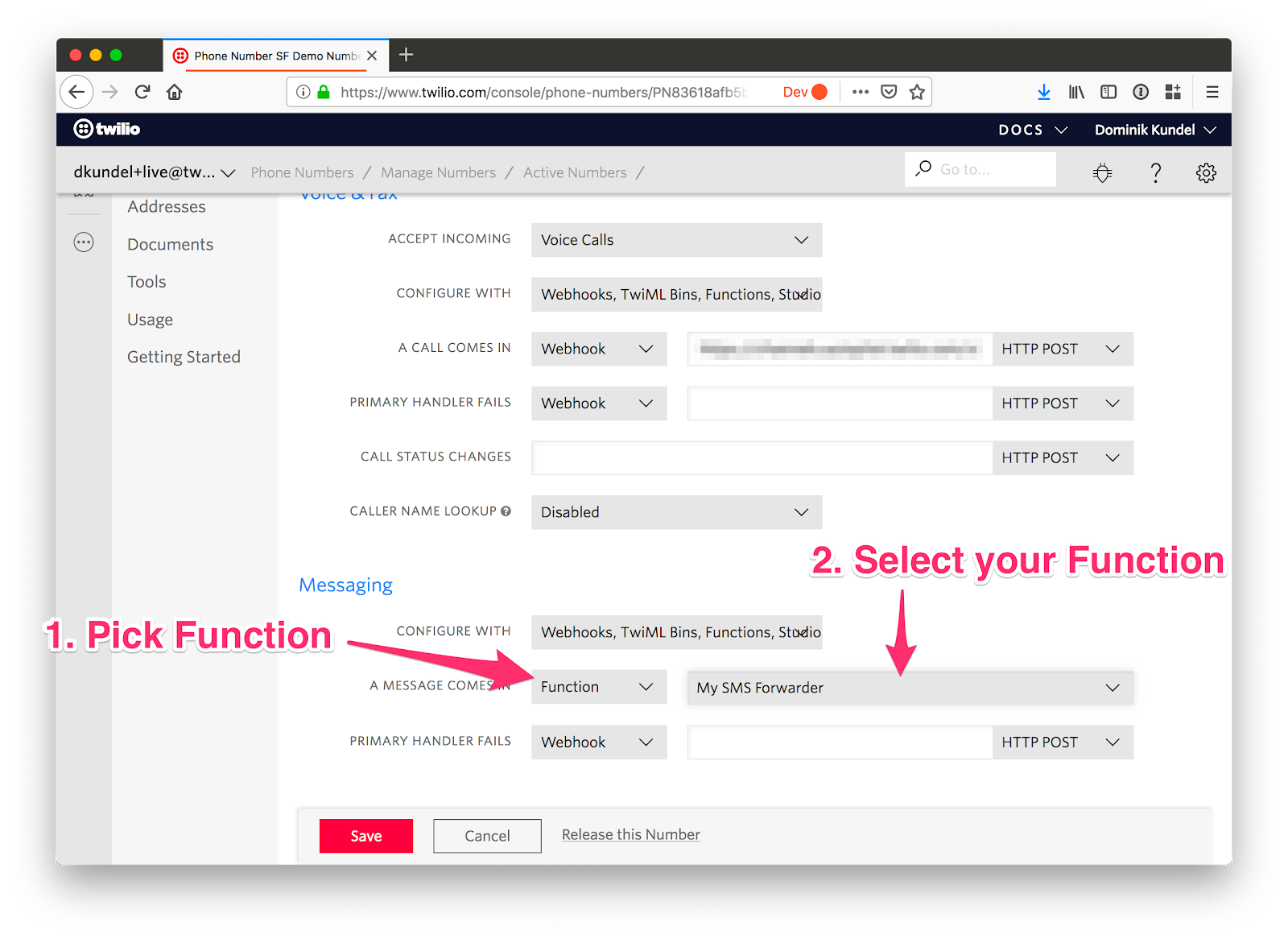
Afterwards click "Save" and grab your phone to text any message to it. You should see a reply with your phone number and the message you sent:
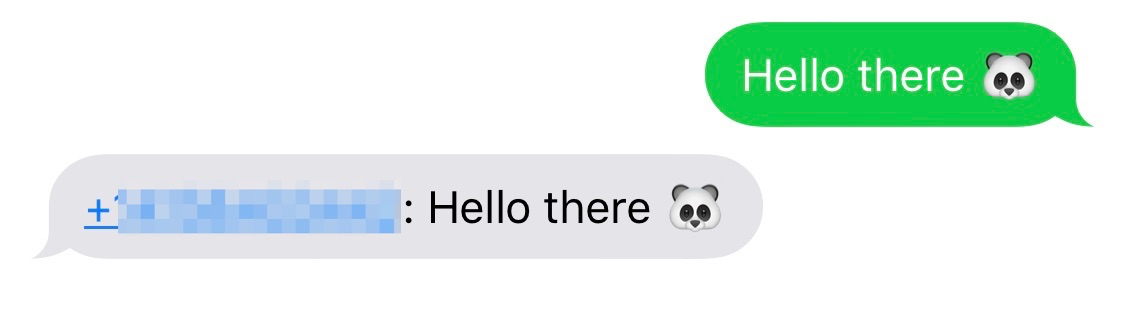
If you want to see if it properly works, ask a friend or colleague to text your Twilio number and you should see their message. Alternatively get a second Twilio number and use the API Explorer's "Message Create" functionality to send an SMS to your forwarding number.
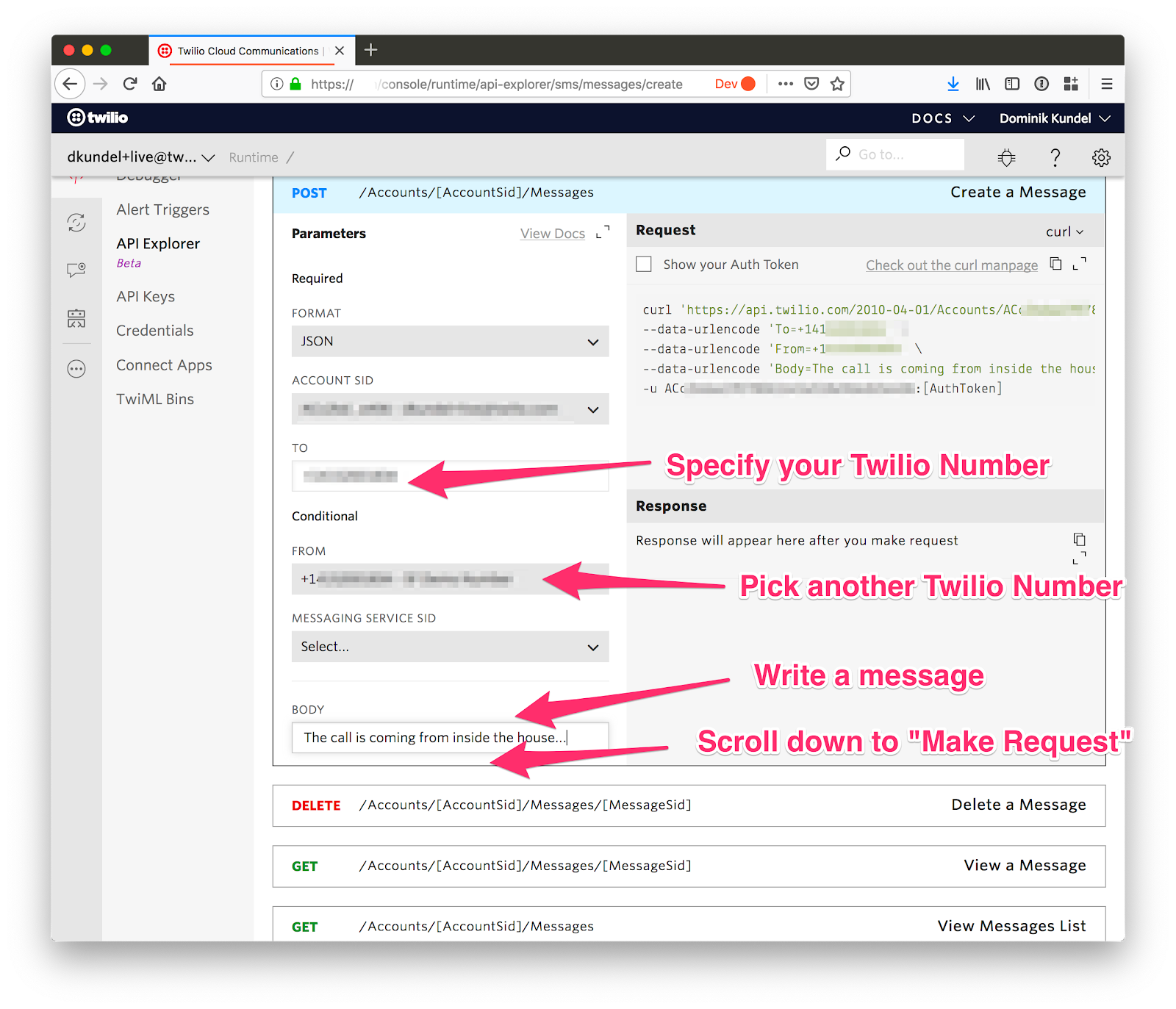
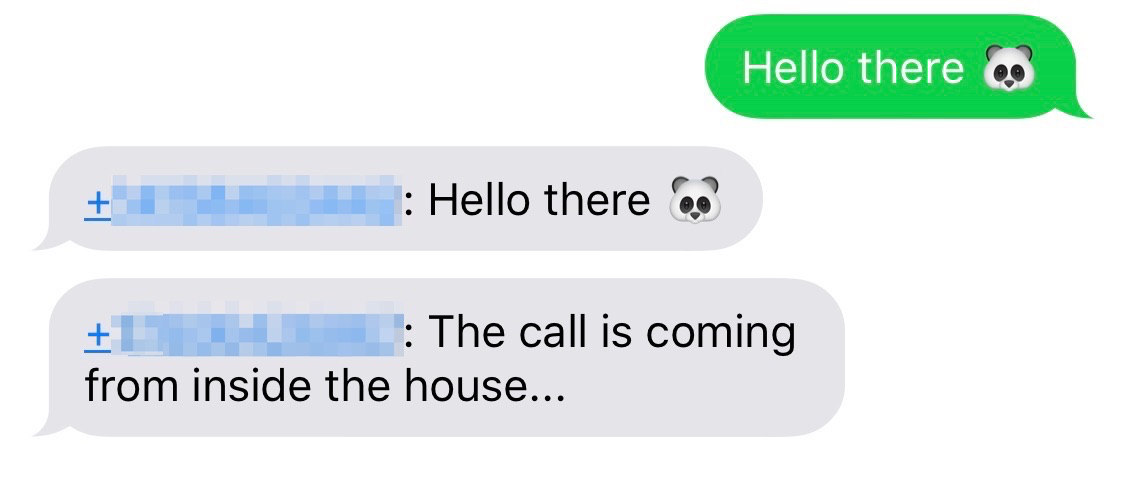
Responding to Messages
Now that we have the message forwarding solved, how do we reply to those messages? If you want to respond with your actual number, that's easy, copy the number from the SMS and write them from your phone. If you want to keep on using your masked number it's not quite that easy.
Right now if you reply to any SMS it will trigger the same SMS webhook and basically just echo to you. We'll modify this behavior by adding the following logic to this:
Check if the message came from our own number:
- Came from someone else -> Forward the SMS like we previously did
- Message is from us -> Parse message to receive intended recipient and forward the message
In order to find out the intended recipient, we'll establish a certain pattern that all of our responses have to follow. It will be the same way that we are currently forwarding messages:
RECIPIENT_NUMBER: MESSAGE_BODY
.
Update your Twilio Function code to apply this logic:
Click save and wait until the updated Function is deployed (there will be a green box saying the deployment was successful).
Grab your phone and try to send an SMS to a friend or your other Twilio number. The SMS has to be in the format +RECEIPIENT_NUMBER: message
.
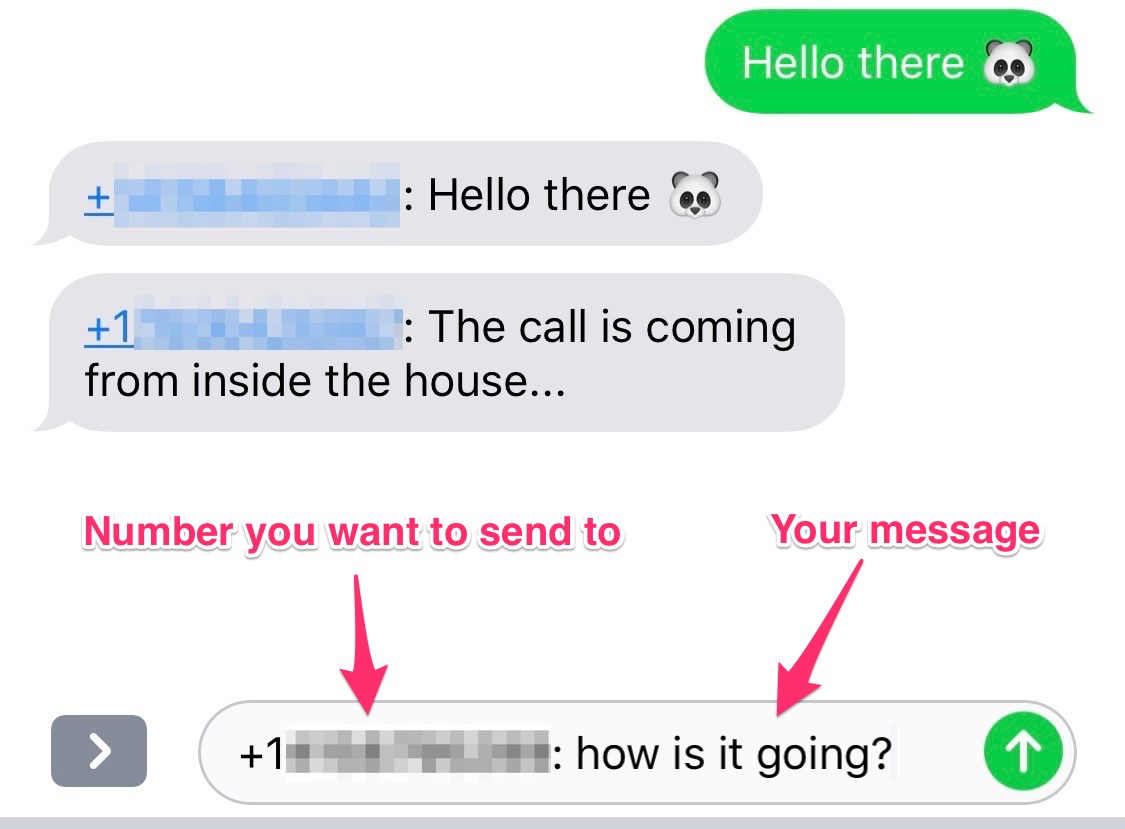
The other person should receive only the text you sent. If you sent it to another Twilio number, check your messaging logs instead.
You can also try sending a text that doesn't fit the format and you should receive a reply that the message is not appropriately formatted.
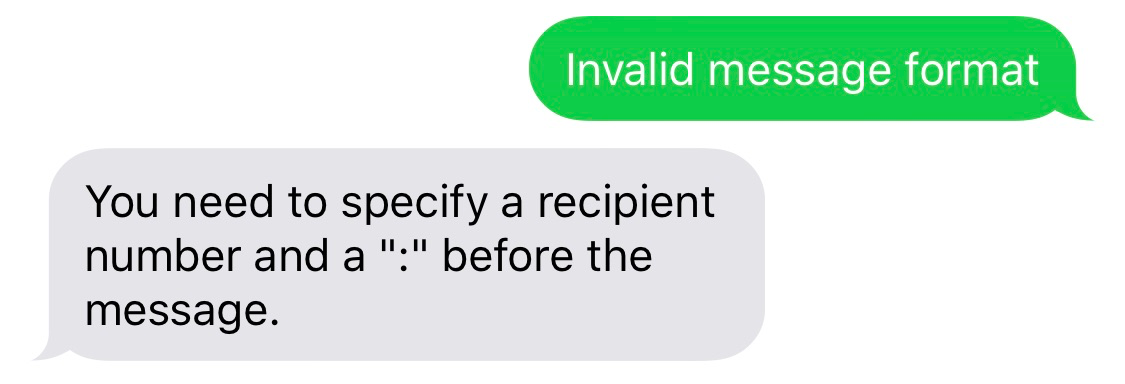
Congratulations!
That's it. You are all set up with an SMS forwarding number that you can use whenever you don't want to give out your normal phone number. But this is really just the start. With Twilio Functions you have access to the npm ecosystem. So why not hook up a Google Spreadsheet or the API of your preferred contacts host to look up names instead of having to write down the phone number to respond. Or create a list of blocked phone numbers. Or maybe you have a completely different idea, I would love to hear what you come up with.
Feel free to reach out to me:
- Email: dkundel@twilio.com
- Twitter: @dkundel
- GitHub: dkundel
- Web: dkundel.com
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.