Getting Started with Twilio and Google App Engine for PHP
Time to read: 3 minutes
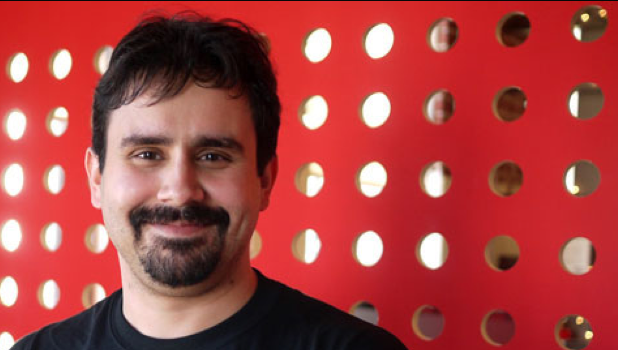
This is a syndicated blog post by Twilio Developer Evangelist, Keith Casey, originally published on Google App Engine’s blog. Keith shows you how to use Twilio with Google App Engine on the PHP runtime.
I’ve wanted to explore Google App Engine for years. Between its SLA, automatic scaling, and queuing system, it has always been compelling. Unfortunately, since my Python skills are somewhere between “Hello World” and “OMG What did I just do!?” I decided to save myself the embarrassment. When Google announced PHP support for App Engine, I was both ecstatic and intrigued about what might be possible. To get something running in just a few minutes, I decided to use our Twilio PHP helper.
When experimenting with a new Platform as a Service, there are nuances of which you should be aware like dealing with a virtualized file system and needing a separate service for email. However, the remaining nuances are usually pretty minimal and required only the “tweaking of my module” rather than a heavy “rebuilding of my app”.
Knowing that up front, let’s dig in.
Set Up The PHP on App Engine Environment
First, check out and follow Google’s Getting started with PHP on Google App Engine to set up your local environment. Their instructions will cover setting up the SDK, connecting to your account, and some details on debugging. It should set up your environment under http://localhost:8080/ which serves as the root of your application.
Upgrade Your Twilio Helper Library
Next, with respect to Twilio’s PHP Helper library, we’ve taken care of the nuances for you, the most important one of which involved falling back to PHP’s Streams when cUrl isn’t available. In your case, simply upgrade the library to v3.11+ or install it for the first time. You can use the library to send text messages and make phone calls exactly as you would in any other PHP environment:
If you’re interested in the the specific changes for our library, you can explore the relevant pull requests here and here. I also have an article called “Preparing your PHP for App Engine” in next month’s php|architect magazine.
URL Routing in PHP
Within App Engine, the entire routing system is powered by the app.yaml file. If you’re familiar with development using frameworks like Zend or Symfony, defining your routes will come naturally and may be marginally more difficult than copy/paste. If you’re only familiar with doing non-framework development in PHP, you’ll have to define a route for each and every PHP file the user accesses. In Google’s example “hello world” app, your app.yaml file should should begin with something like this:
The request handlers are the important part. If you’re using an MVC framework or OpenVBX, you’ll most likely have one primary element mapping your index.php file and a set of elements for static assets like JavaScript, CSS, and images.
Alternatively, if you’re not using a using a framework, app.yaml will map different URL patterns to individual PHP scripts, like this:
Within those individual PHP scripts, no changes are necessary, so our make-call.php is straightforward and identical to our quickstart:
Also, the send-sms.php can be the same as our normal quickstart:
Now you can access your scripts via http://localhost:8080/make-call.php and http://localhost:8080/send-sms.php respectively. If the file is one that doesn’t need to be accessible publicly, such as your credentials.php file, it shouldn’t have a listing.
Voila. You now have your first PHP application on Google App Engine!
I hope this is helpful as you test and deploy your PHP applications on Google App Engine. If you have any tips, feedback, suggestions, or just a good joke please let me know via email or Twitter: @CaseySoftware.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.