Understanding Vue.js
Time to read: 6 minutes
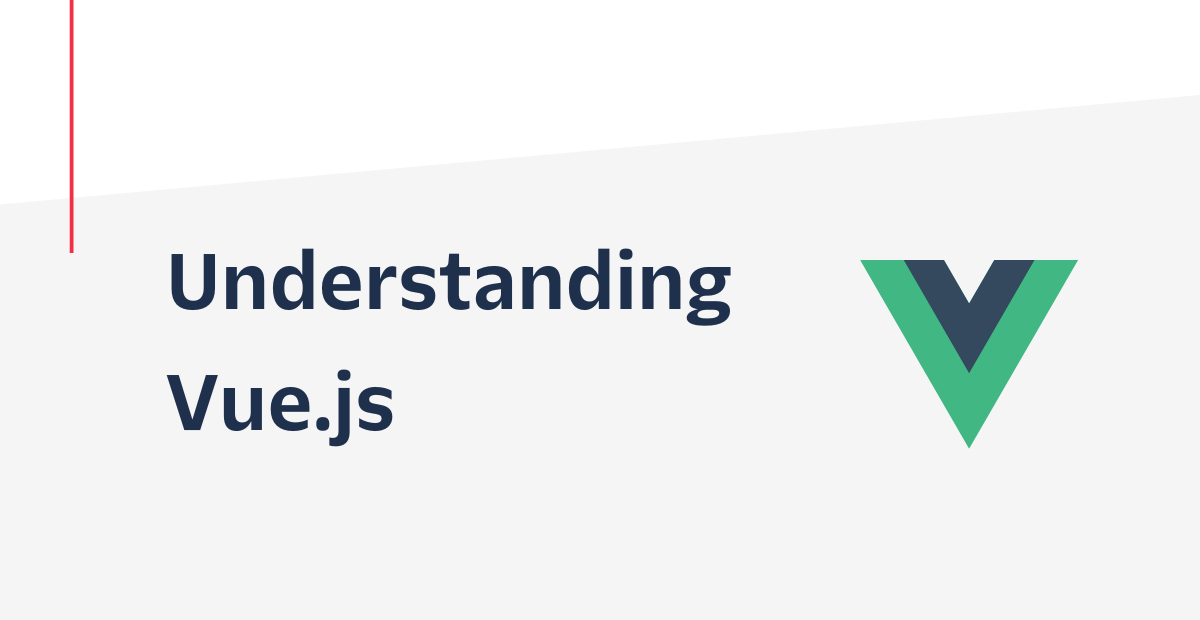
If you’ve been working with JavaScript frameworks in the past few years, you’ve probably heard of Vue.js before. In this post, you’ll learn what Vue is, its benefits and drawbacks, and resources to help you learn and build with it.
Prerequisites
This blog post briefly introduces Vue. However, some code and technical concepts are discussed, so you should understand basic JavaScript, HTML, and CSS.
Table of contents
What is Vue.js?
Vue is a JavaScript framework for building user interfaces. Since its initial release in 2014, it has become one of the most popular JavaScript frameworks: It was the sixth-most common web technology used by respondents of the 2022 Stack Overflow Developer Survey and is the most-starred JavaScript framework on GitHub at the time of this post’s publishing.
Its two core features are declarative rendering and reactivity. Vue lets you declaratively describe the rendered DOM based on the component’s state using an HTML-based template syntax. Its reactivity system automatically tracks property access and mutations of reactive objects and efficiently updates the DOM during state changes.
Additionally, it’s important to know that Vue has two APIs: the Options API and Composition API. The Options API lets you define a component’s logic by declaring an object of options; the Composition API lets you define component logic using API functions and compose state by combining multiple functions.
A basic example
Below is an example of a basic counter component written with the Options API.
Here’s what the code looks like in action:
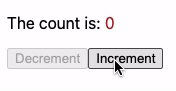
This example demonstrates a few more features of Vue: the Single-File Component format, reactivity, template syntax, and event handling. The following sections briefly explore these features and the code.
Single-File Component
A Single-File Component (SFC) is a file format that lets you use one file to define the JavaScript logic, HTML template, and CSS styles of a Vue component. SFCs have a .vue
file extension and get compiled to JavaScript and CSS by the framework.
The <script>
block is where the component’s logic is defined. This example uses the Options API, so the <script>
defines the component’s reactive state with the data
option. In this case, a count
property is defined with 0
as the initial value.
The <template>
block is used to declare the component’s view using an HTML-like syntax. This template syntax will be explored further below.
Lastly, the <style>
block contains a component’s styles. This example declares a .count
class used by the <span>
element in the <template>
block. Also note that the scoped
attribute is present on the <style>
tag, which allows you to further encapsulate the styles by only applying them to the current component.
Template syntax, reactivity, and event handling
The syntax used to define templates in Vue is HTML-based and syntactically valid HTML. In the example above, the template uses regular HTML elements and attributes, JavaScript, and CSS.
However, you might have noticed the attributes that start with v-bind:
and v-on:
. These are special Vue attributes called directives, and the colon is semantically-valid for attribute names.
The v-on
directive lets you execute some code when a DOM event is triggered. In this case, count
is incremented or decremented whenever one of the buttons fires an onclick
event. v-bind
binds an element’s attribute to a JavaScript expression; the decrement <button>
has the disabled
attribute if count === 0
.
You can interpolate text in templates using double curly braces. In this example, the count
state value gets interpolated within the <span>
. The double curly brace syntax also binds the data: whenever count
is incremented or decremented, the rendered HTML changes.
Benefits of Vue.js
Vue has gained popularity because of its many benefits. In particular, it’s easy to learn, performant, lightweight, and flexible.
Easy to learn
Since Vue is built on top of standard HTML, CSS, and JavaScript, it’s easier to pick up than other JavaScript frameworks that require you to learn additional markup syntaxes and languages, such as JSX and Typescript. Additionally, the comprehensive official documentation and other learning resources make it easy to start learning.
Performant
Vue is designed to be efficient and fast. It uses a virtual DOM to render nodes efficiently, and makes optimizations to improve performance at runtime.
Compared to Angular and React, two other popular JavaScript frameworks, Vue is generally more performant. The following table from Stefan Krause on GitHub compares some common benchmarks between each framework:
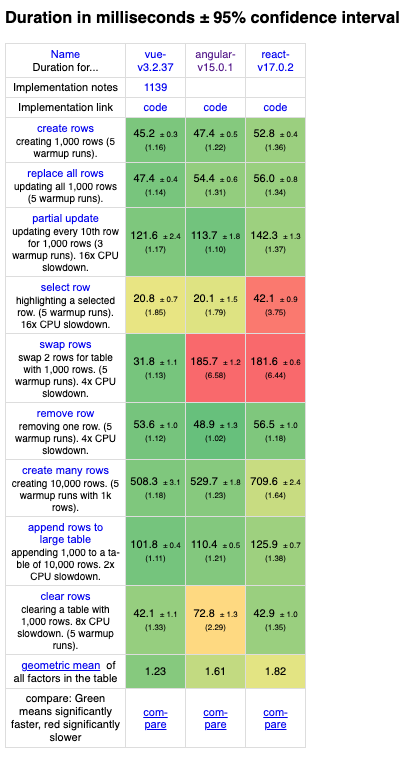
Lightweight
Vue was built to be lightweight from the start: In an interview with Between the Wires, Vue creator Evan You said, “I figured, what if I could just extract the part that I really liked about Angular and build something really lightweight without all the extra concepts involved?” Vue is lightweight, with a file size of roughly 58 kB for the minified global build of v3.2.
Flexible
Vue is a very flexible framework suitable for a wide-variety of projects. You can use it to build Single-Page Applications, Web Components, SSGs, and even desktop and mobile applications. It’s “incrementally adoptable,” meaning that you can easily use and extend the framework for your application’s needs.
Drawbacks of Vue.js
While Vue has many advantages, it has a few drawbacks to consider before you begin using it.
Community support
Vue has less community support than other popular frameworks, such as Angular and React.
For example, at the time of this post’s publishing, a Stack Overflow search for questions tagged with Vue yields around 100,000 questions; a search for Angular results in 290,000 questions, and searching for React shows 437,000 questions. Further, the Vue subreddit has 90,000 members, while the React subreddit has 331,000 members.
Libraries
Vue has fewer third-party libraries than some other popular frameworks, meaning there might not be an existing solution for your particular use case within Vue. An NPM search yields roughly 35,000 packages tagged with the keyword “Vue,” while 97,000 packages have the tag “React.”
Job opportunities
If you’re interested in getting a job that uses Vue, you may have a more challenging time than if you were to get employed with another framework.
According to Enlyft, Vue has a smaller market share: Around 14,500 companies build with Vue, while 235,000 companies use Angular and React each. Furthermore, in the June 2022 Hacker News Hiring Trends report, 25.5% of job posts were for React, while only 3.5% were for Vue.
Best resources to learn Vue.js
There are many great free and paid resources to help you start learning Vue.
The best place to begin learning Vue is the official Vue documentation. The Vue documentation is a comprehensive guide to the framework’s essentials and dives deep into more complicated subjects and best practices.
You can go through the official Vue tutorial or MDN’s short Vue tutorial if you like to learn with tutorials. For high-quality, free and paid video courses, consider Vue Mastery, Vue School, and Vue.js Developers.
If you prefer written content, DEV Community, CSS-Tricks, LogRocket’s blog, and Smashing Magazine have many Vue blog posts and tutorials.
Lastly, Vue has an active Discord community and many in-person and remote events
Tutorials to help you use Twilio products with Vue.js
Ready to start building? The Twilio blog has many great tutorials to help you build your next Vue app with Twilio products. Check out the list below to get started.
Twilio Conversations
- Creating a web chat app with Twilio Conversations and Vue.js (Part 1) by Stephenie Minami Nakajima
- Creating a web chat app with Twilio Conversations and Vue.js (Part 2) by Stephenie Minami Nakajima
Twilio Functions
- Send an SMS with Vue and Twilio Functions by Treasure Porth
- Deploy your Vue.js Application with the Twilio Serverless Toolkit by Miguel Grinberg
Twilio Sync
- Build Your Own API Analytics in Vue.js with PHP, Laravel, and Twilio Sync by Michael Okoko
- Building TwilioQuest with Twilio Sync, Django, and Vue.js by David Prothero
SendGrid
- How to Create a Landing Page with Laravel, Vue.js, and SendGrid by Matthew Setter
- Create a Mailing List in PHP using Laravel, Vue.js, and Twilio’s SendGrid Email API by Anumadu Udodiri Moses
Conclusion
Vue.js is one of the most-used JavaScript frameworks and focuses on declarative rendering and reactivity. Vue has many benefits, such as being easy to learn, performant, lightweight, and incrementally adoptable. It also has some disadvantages, such as fewer third-party libraries and job opportunities than other frameworks.
Generally, Vue is an excellent framework for building many types of applications, and many great resources exist to start learning and creating with it.
Zach is a software engineer based in Washington State. He works with JavaScript and React and enjoys writing about code on his blog. You can get in touch with him on LinkedIn, Twitter, or at me[at]zachsnoek.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.