Instant Lead Alerts with PHP and Laravel
Time to read: 2 minutes
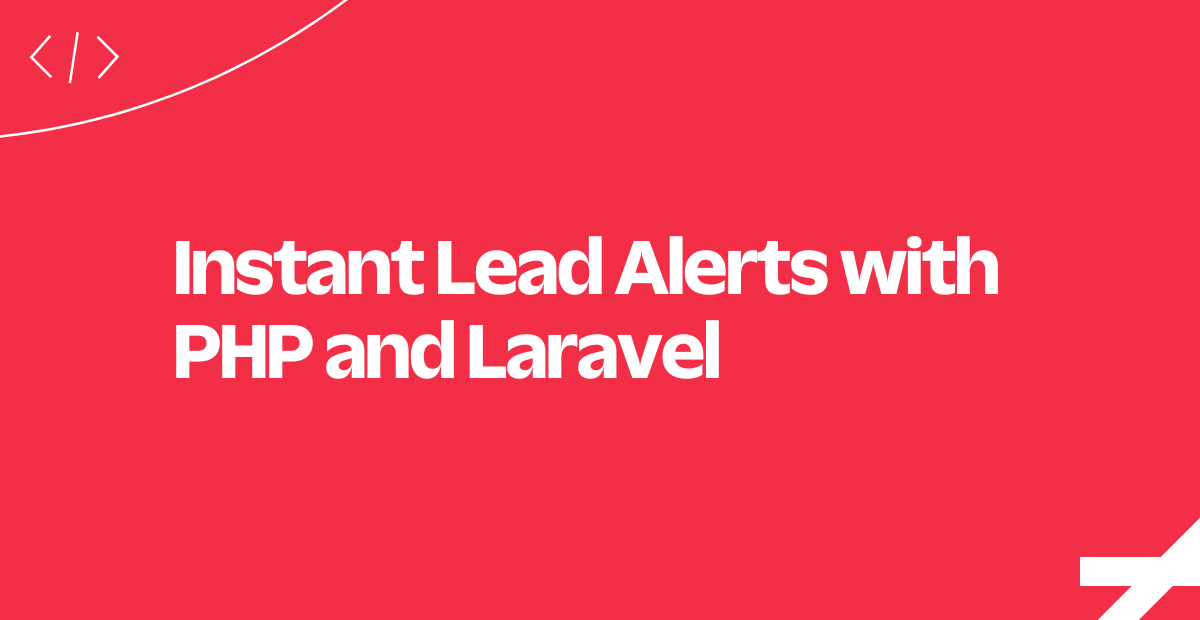
You probably already have landing pages or product detail views in your web application which you're using to generate some excellent leads for your business. Would you like to let the sales team know when you've got a new qualified lead?
In this tutorial, we'll use Twilio Programmable SMS in a PHP and Laravel application to send a message when a new lead is found.
In this example, we'll be implementing instant lead alerts for real estate.
We'll create a landing page for a new house on the market with a form to request more information. When a user submits the form we'll automatically notify a real estate agent in the field.
Let's begin!
Populate the Landing Page Data
To display a landing page for our house, we'll first need to store some data.
For demonstration purposes, we've hard-coded an associative array containing the information we'd like to display.
Next, let's see how to render the landing page.
Render the Landing Page
In our blade template we'll insert data about the house. We'll also include a form in the sidebar to collect user contact details when they request more information.
Now that we have a landing page, let's look at how we use the Twilio REST Client to send messages.
Creating a Twilio REST API Client
Here we create a helper class with an authenticated Twilio REST API client that we can use anytime we need to send a text message.
We initialize it with our Twilio Account Credentials stored as environment variables. You can find the Auth Token and Account SID in the console:
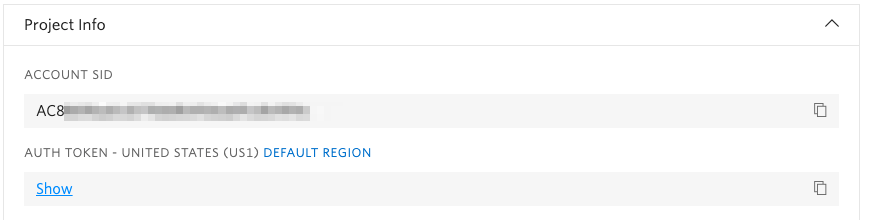
Our Twilio REST Client is ready! Now, let's see what we do when a new lead comes in from the form.
Handle the POST Request
This code handles the HTTP POST
request issued by our landing page.
It uses our sendMessage
method to send an SMS message to the real estate agent's phone number, which is stored in an environment variable. We include the lead's name, phone number, and inquiry directly into the body of the text message we send to the agent.
And that's it! The agent has all the required information to immediately follow up on the lead.
We've just implemented an application to instantly route leads to sales people in the field using text messages. Next, we'll take a look at some other easy to integrate features for your PHP application.
Where to Next?
PHP and Twilio go together so well - here's a couple of tutorials to prove it:
Twilio Client allows your website users to make and receive phone calls directly from their web browsers.
Call Tracking helps you measure the effectiveness of your marketing campaigns.
Did this help?
Thanks for checking out this tutorial!
Tweet @twilio to let us know what you're building.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.