Call Tracking with C# and ASP.NET MVC
Time to read: 4 minutes
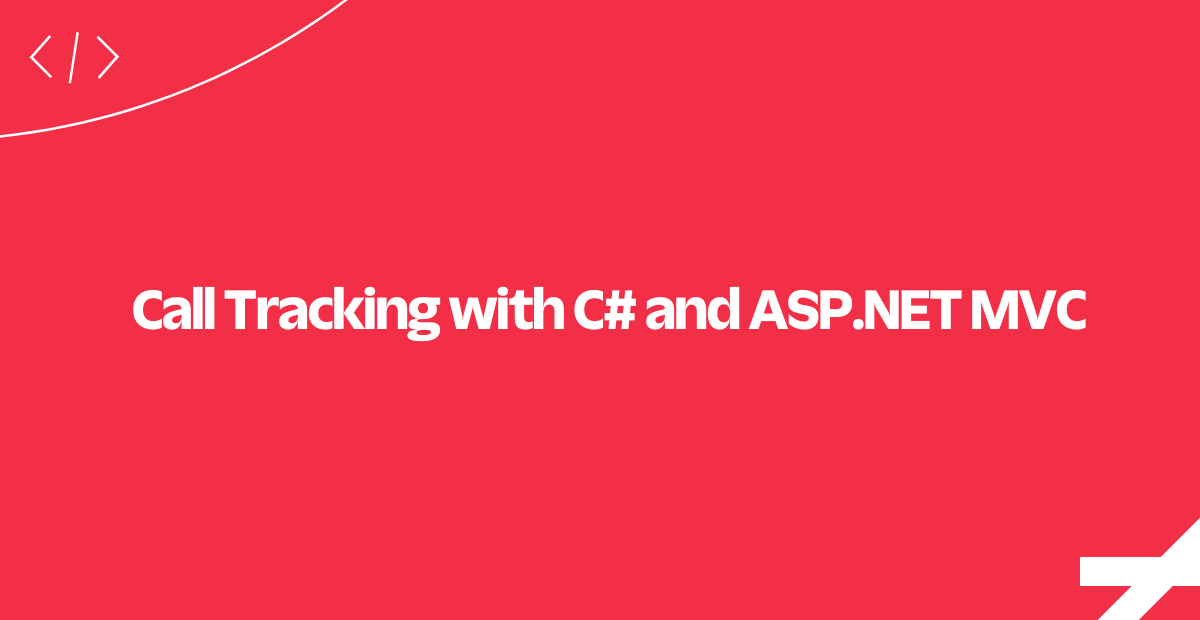
This ASP.NET MVC web application shows how you can use Twilio to track the effectiveness of different marketing channels.
This application has three main features:
- It purchases phone numbers from Twilio to use in different marketing campaigns (like a billboard or a bus advertisement)
- It forwards incoming calls for those phone numbers to a salesperson
- It displays charts showing data about the phone numbers and the calls they receive
In this tutorial, we'll point out the key bits of code that make this application work. Check out the project README on GitHub to see how to run the code yourself.
Search for available phone numbers
Call tracking requires us to search for and buy phone numbers on demand, associating a specific phone number with a lead source. This class uses the Twilio C# Helper Library to search for phone numbers by area code and return a list of numbers that are available for purchase.
Now let's see how we will display these numbers for the user to purchase them and enable their campaigns.
Display available phone numbers
We display a form to the user on the app's home page which allows them to search for a new phone number by area code. At the controller level we use the Twilio.RestClient
we created earlier to actually search for numbers. This will render the view that contains a list of numbers they can choose to buy.
We've seen how we can display available phone numbers for purchase with the help of the Twilio C# helper library. Now let's look at how we can buy an available phone number.
Buy a phone number
Our PurchasePhoneNumber
method takes two parameters, the first one is a phone number and the second one is the application SID. Now our Twilio API client can purchase the available phone number our user chooses.
If you don't know where you can get this application SID, don't panic, the next step will show you how.
Set webhook URLs in a TwiML Application
When we purchase a phone number, we specify a voice application SID. This is an identifier for a TwiML application, which you can create through the REST API or your Twilio Console.
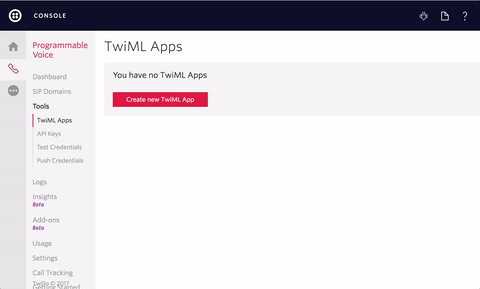
Associate a phone number with a lead source
Once we search for and buy a Twilio number, we need to associate it with a lead source in our database. This is the core of a call tracking application. Any phone calls to our new Twilio number will be attributed to this source.
So far our method for creating a Lead Source and associating a Twilio phone number with it is pretty straightforward. Now let's have a closer look at our Lead Source model which will store this information.
Define the LeadSource model
The LeadSource
model associates a Twilio number to a named lead source (like "Wall Street Journal Ad" or "Dancing guy with sign"). It also tracks a phone number to which we'd like all the calls redirected, like your sales or support helpline.
As the application will be collecting leads and associating them to each LeadSource or campaign, it is necessary to have a Lead model as well to keep track of each Lead
as it comes in and associate it to the LeadSource
.
Define the Lead model
A Lead
represents a phone call generated by a LeadSource
. Each time somebody calls a phone number associated with a LeadSource
, we'll use the Lead
model to record some of the data Twilio gives us about their call.
The backend part of the code which creates a LeadSource
as well as a Twilio Number is complete. The next part of the application will be the webhooks that will handle incoming calls and forward them to the appropriate sales team member. Let's us see the way these webhooks are built.
Forward calls and create leads
Whenever a customer calls one of our Twilio numbers, Twilio will send a POST request to the URL associated with this action (should be /CallTracking/ForwardCall
).
We use the incoming call data to create a new Lead
for a LeadSource
, then return TwiML that connects our caller with the ForwardingNumber
of our LeadSource
.
Once we have forwarded calls and created leads, we will have a lot of incoming calls that will create leads, and that will be data for us but we need to transform that data into information in order to get benefits from it. So, let's see how we get statistics from these sources on the next step.
Getting statistics about our lead sources
One useful statistic we can get from our data is how many calls each LeadSource
has received. We use LINQ to make a list containing each LeadSource
and a count of its Lead
models.
Up until this point, we have been focusing on the backend code to our application. Which is ready to start handling incoming calls or leads. Next, let's turn our attention to the client side. Which, in this case, is a simple Javascript application, along with Chart.js which will render these stats in an appropriate way.
Visualizing the statistics with Chart.js
On the client side, we fetch call tracking statistics in JSON from the server using jQuery. We display the stats in colorful pie charts we create with Chart.js.
That's it! Our ASP.NET MVC application is now ready to purchase new phone numbers, forward incoming calls, and record some statistics for our business.
Where to next?
If you're a C# developer working with Twilio, you might also enjoy these tutorials:
Instantly collect structured data from your users with a survey conducted over a voice call or SMS text messages.
Learn to implement appointment reminders in your web app with Twilio. Appointment reminders allow you to automate the process of reaching out to your customers in advance of an upcoming appointment.
Did this help?
Thanks for checking this tutorial out! If you have any feedback to share with us please contact us on Twitter, we'd love to hear it.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.