Make Phone Calls with Twilio, Blazor & C#
Time to read: 6 minutes
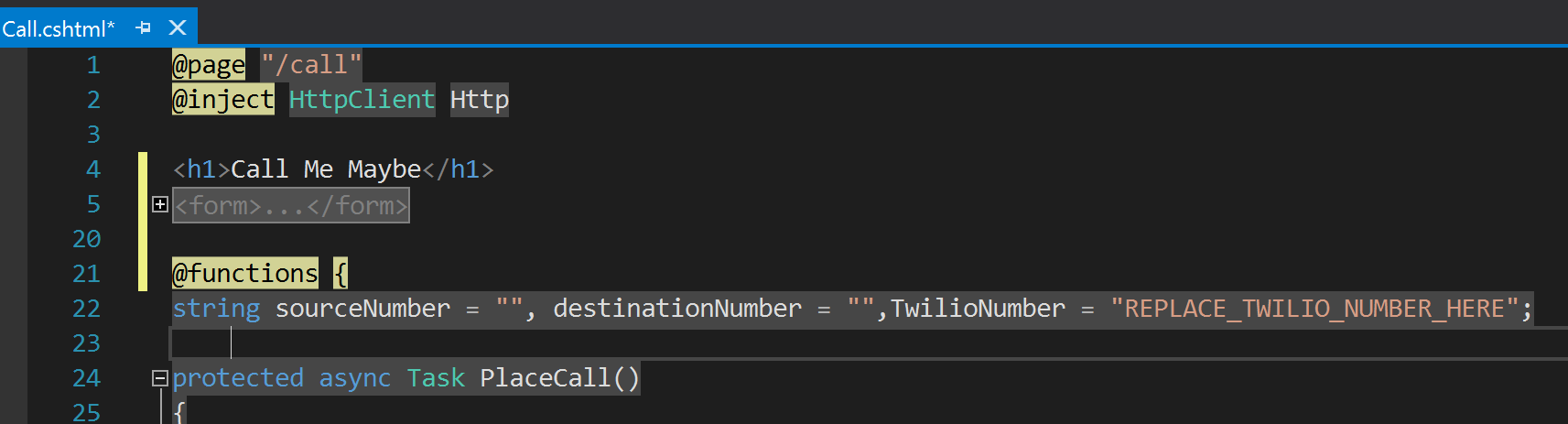
In this blog post, we are going to use Blazor to build a simple web page that makes telephone calls straight from your browser with C# and Twilio. What is Blazor you ask? It is a new experimental framework that allows developers to build web applications using HTML, C#, and WebAssembly. With WebAssembly, your browser downloads the web application’s binaries from the server and runs the code locally in a sandboxed environment from your browser. This means you’ll write your client side code with a compiled language like C# and have it run faster and more safely from your browser. You can check out the code on Github. If anything else sounds foreign to you, keep calm and carry on! We’ll take care of you.
LAB TOOLKIT
Now to kick off our post, we should make sure we have some tools to follow along:
- .NET Core 2.1 SDK (version 2.1.300 or greater)
- Visual Studio 2017 (15.7 or greater)
- Blazor
- Twilio Account & Phone Number
Now because there are some really strict version dependencies, check your installed .NET Core version by opening up a command prompt and typing dotnet --version
. Your output should match this: 2.1.300-preview2-008533
if you’ve got 2.1.300 installed. To verify your Visual Studio version, go to Help
> About Microsoft Visual Studio
after you start up Visual Studio. A dialog box should open up that contains your version number, as shown in the picture below.
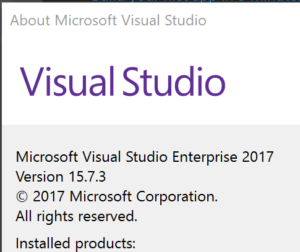
CREATE A BLAZOR PROJECT
To get started you’ll first need to create a Blazor application, which is just an ASP.NET Core web application. In Visual Studio 2017 go to File > New > Project. Then select the ‘ASP.NET Core Web Application’ and name your project ‘BlazorCall’. When the popup opens make sure ASP.NET Core 2.0 is selected at the top and then in the main box select Blazor.
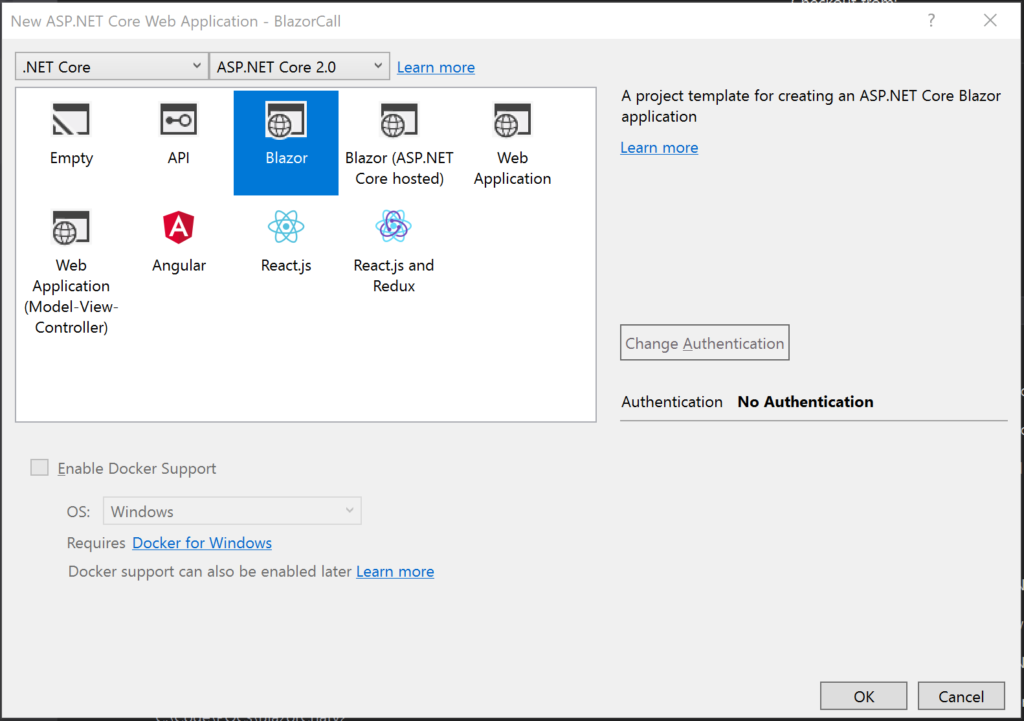
Now if you’re a Visual Studio pro, you are probably used to hitting F5 and seeing a sample application run but debugging support is coming in a future release. So make sure to press ‘Ctrl+F5’ on your keyboard to run your first Blazor application or hit the big ‘BlazorCall’ button in the toolbar.

There’s a nice sample application here that shows some of the things you can do with Blazor. Once the project is loaded, hit ‘F5’ on your keyboard to run your first Blazor application or hit the big ‘BlazorCall’ button in the toolbar. It should look like the photo below.
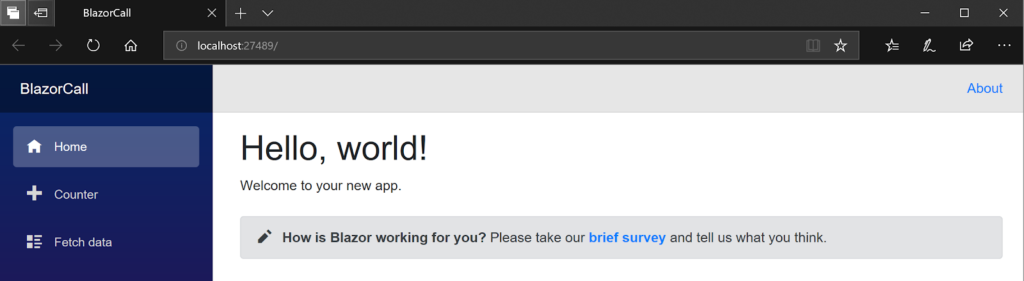
Now it’s time to build a Blazor component to make our phone call.
CREATING A BLAZOR COMPONENT
Blazor applications are ASP.NET web applications that route HTTP requests to Blazor components. Creating a new component is as simple as creating a new ‘Razor View’ in the Pages folder. Let’s create that file by right clicking on Pages > Add > New Item. Then we will expand Visual C# > ASP.NET Core > Web, select Razor View in the middle of the window and name it ‘call.cshtml’.
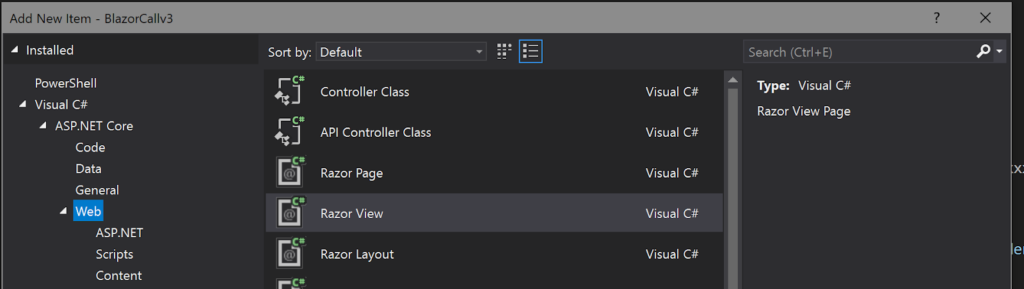
When our new file opens up, we are going to replace the code in the file with ‘@page “/call”‘ at the very top of the file. Doing this creates a route to navigate to our component.
The component is going to be a basic HTML form that has three fields: a Twilio phone number, your phone number, and a destination number to dial from the website along with a button to place a phone call. Copy and paste the below HTML markup into the ‘call.cshtml’ file right underneath the previous routing code we added above:
If you were to run this app, it won’t build because we need to add some code to make a phone call when someone clicks on the button. Check out the next section to see how we will use the Twilio REST API to make the phone call.
USING TWILIO TO PLACE A PHONE CALL
To add some code for the button handler, drop it into a functions block at the bottom of the cshtml file. In the same call.cshtml file, paste the below code to the bottom of the file:
If you’re anything like me, this is the part where you start thinking what does this code do? First, it makes an HTTP request to Twilio’s REST API and calls your cell phone. After you pick up the phone, Twilio makes an HTTP request to the URL specified in the callTwimlUrl parameter. The HTTP response from this request contains some Voice TwiML that then makes a call to your destinationNumber using your TwilioNumber as the caller ID. Here is what that TwiML looks like:
Now before you go hitting that BlazorCall button, there are two really important steps before things will work:
- For the application to make that HTTP request, we need to inject an HttpClient object into our component. So on the second line of call.cshtml page, copy @inject HttpClient Http
- Make sure to replace the prompts in the code with your specific Twilio account details
Once you take care of these things, hit Ctrl+F5 to run your application, and browse to http://localhost:xxxx/call. Once your form loads, type in two valid phone numbers and watch the magic happen.
WRAP UP
Now just like the members of our cool scientist hall of fame, we have been armed with a cool, new technology that allows us to build websites using HTML and C#.
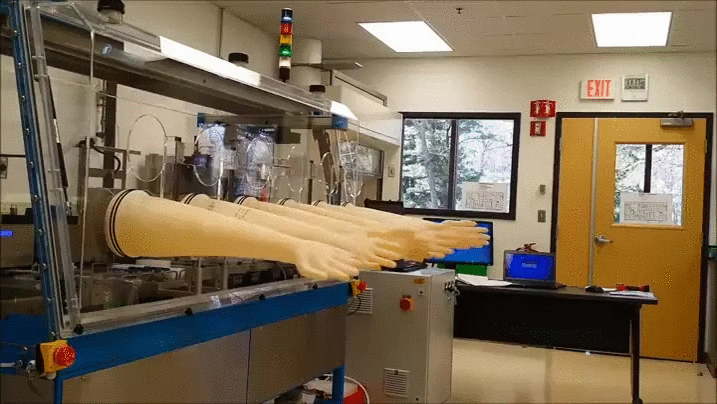
This is only scratching the surface of what you can do. WebAssembly is changing the way we write code for the web. It runs native code from your browser and is estimated to run 20x time faster than Javascript. Check out more Blazor resources here, here, and here. Keep in mind – Blazor is an experimental framework that is still under development. So it’s probably best to keep this out of a production environment for now.
Hit me up on Twitter, email, or in the comments below. I can’t wait to see what you build.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.