Cheesecake Labs Builds SMS User Verification With Python and Django
Time to read: 1 minute
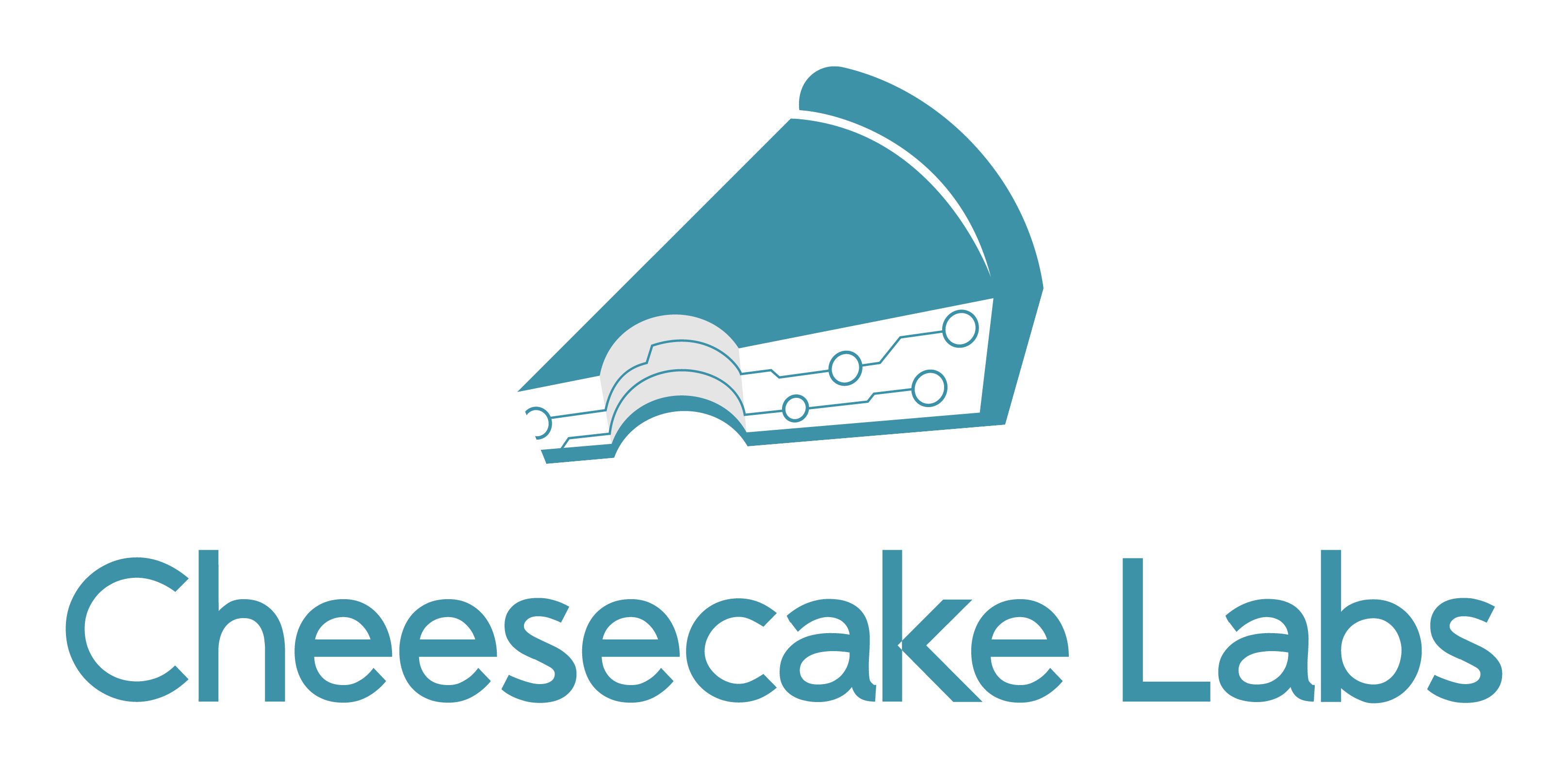
Cheesecake Labs, a development shop, built their SMS user verification app in less than a day using Python and Django. Here’s how it works from the user’s end.
1. The user enters his/her phone number in the app
2. The app pings the server, and the user quickly receives an SMS with a confirmation 6-digit pin-code.
3. As soon as the user types the correct pin in the app, the phone number is validated.
This was iOS developer, Marcelo Salloum‘s first Twilio hack. Marcello wanted to create a verification feature that didn’t require the users to create a log-in and password. Instead, he used the username everyone has in their pocket at all times — your phone number.
Here’s the code he used to build the feature, which Marcello based off of these docs.
Cheesecake Lab’s SMS Verification Solution — Built With Python + Django
When a new user signs up, a http request is triggered through @receiver(user_signed_up), then the SMSVerification model creates a pin code for that user and sends it to the user’s phone.
In the case where the user asks for another SMS, Cheesecake Labs sends ReSend logic:
That’s all the code it takes to build User Verification. Check out a tutorial on how to build it here.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.