Bulk Email Address Validation in Node.js
Time to read: 4 minutes
Bulk Email Address Validation in Node.js
Email address validation helps identify email addresses in your marketing lists and customer base that are likely to produce a hard bounce and impact your sender score. The validation algorithm runs numerous checks to determine if an address is legitimate, such as if a domain is set up to receive email, if it’s disposable, if the name was likely button mashed, and more.
Email address validation is one meaningful component of building strong email sending practices and reaching your customers. This blog post will provide an in depth tutorial of how to integrate with Twilio SendGrid’s Bulk Email Address Validation using Node.js.
Real Time Validation vs Bulk Email Address Validation
When it comes to validating email addresses, there are two main approaches: real time validation and Bulk Email Address Validation. Real time validation has been around for a while, and involves validating email addresses one by one. This method is best suited for scenarios where only a few email addresses need to be validated or when validation needs to be seamlessly integrated into a user-facing application, like during the sign-up process on a website
Bulk email address validation is a crucial process for businesses and organizations engaged in email marketing that need more confidence within large subscriber lists or customer databases. This method is particularly useful for managing extensive amounts of data, and SendGrid’s implementation enables the validation of up to a million email addresses in one go. By ensuring the accuracy and deliverability of email lists before launching campaigns, Bulk Email Address Validation helps maintain good email deliverability and ensures that messages reach real, active recipients.
How do I get access?
Bulk Email Address Validation is exclusively accessible to Email API Pro and Premier level accounts. Until your account is upgraded to Pro or Premier, the option to generate an email address validation API key will not be visible. The email address validation API key is distinct from your other keys, and is essential for both asynchronous bulk email address validations and real-time email address validations. Employing this Bulk Email Address Validation feature aids in purifying your current database; however, SendGrid advises verifying email addresses upon their entry into your customer funnel.
You can use this API to:
- Validate a CSV of email addresses asynchronously in a single job
- The CSV can contain up to 1M emails or 50MB of data
Results are sent to the email address linked with the SendGrid account.
Prerequisites
- Twilio SendGrid Pro or Premier Account. If you don’t yet have one, you can sign up for an account here.
- Node.js installed on your computer.
- email address validation API Key. If you do not have one, please create one using the email address validation API Key Creation Guide. Here is a view of my API Keys page in my SendGrid console:
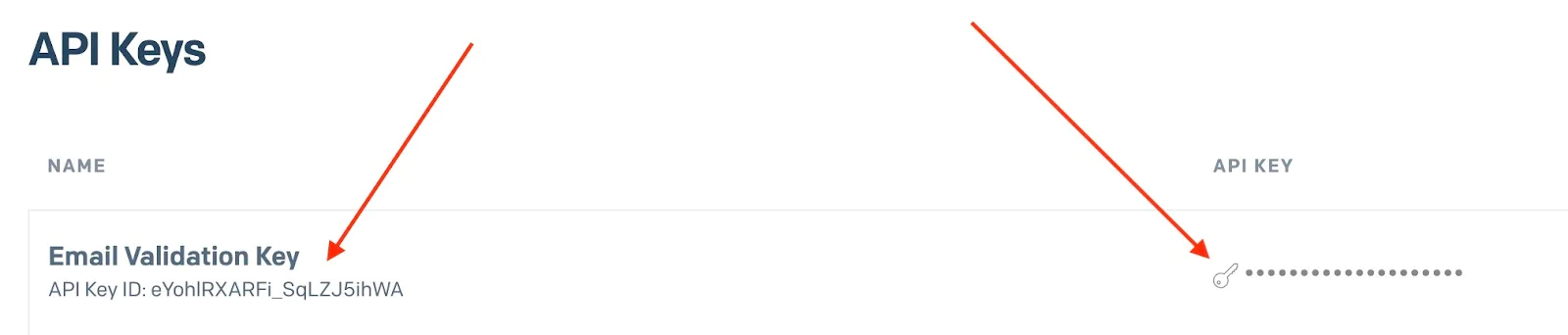
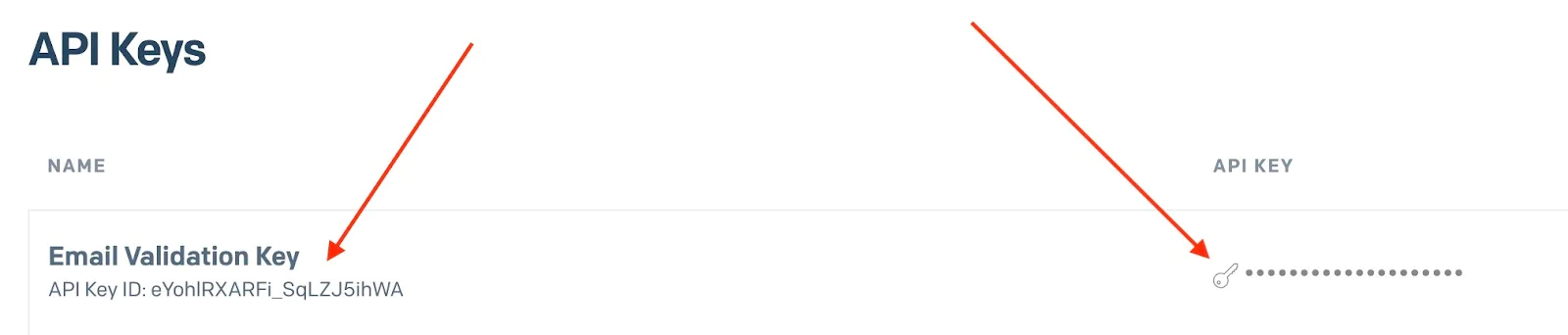
Let’s get started
Here are the basic steps of our build today:
- Upload a CSV
- Get job status list
- Get job status
Upload a CSV
Set Environment Variables
Create an .env
file to store your environment variables SENDGRID_API_KEY
in the following format. Further details about your node environment variables can be found here. It is advisable not to include this file in version control.
Request the presigned URL and headers
The bulk email address validation process takes place asynchronously. This endpoint returns a URL (upload_uri
) and HTTP headers (upload_headers
) which can subsequently be used to PUT a file of email addresses to be validated.
Uploaded CSV files can be in the following formats: .csv
or .zip
.
You must include the field file_type
with the value csv
or zip
in your request body.
You can find the code repository here: https://github.com/yuktiahuja-twilio/EmailBulkValidationApi.git
Example Node.js code
Example code output
The output of this script will return job_id
, upload_uri
, and upload_headers
.
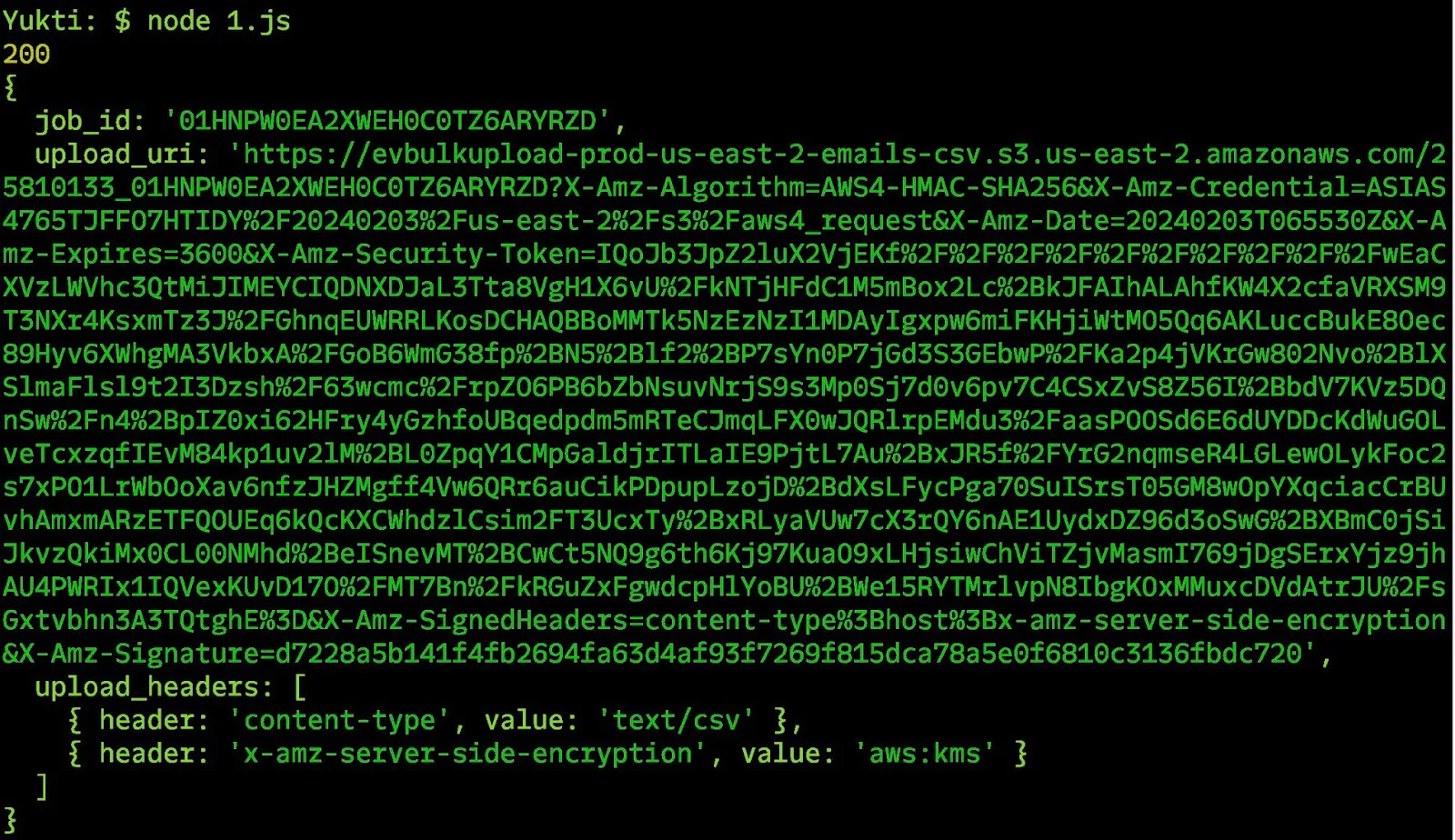
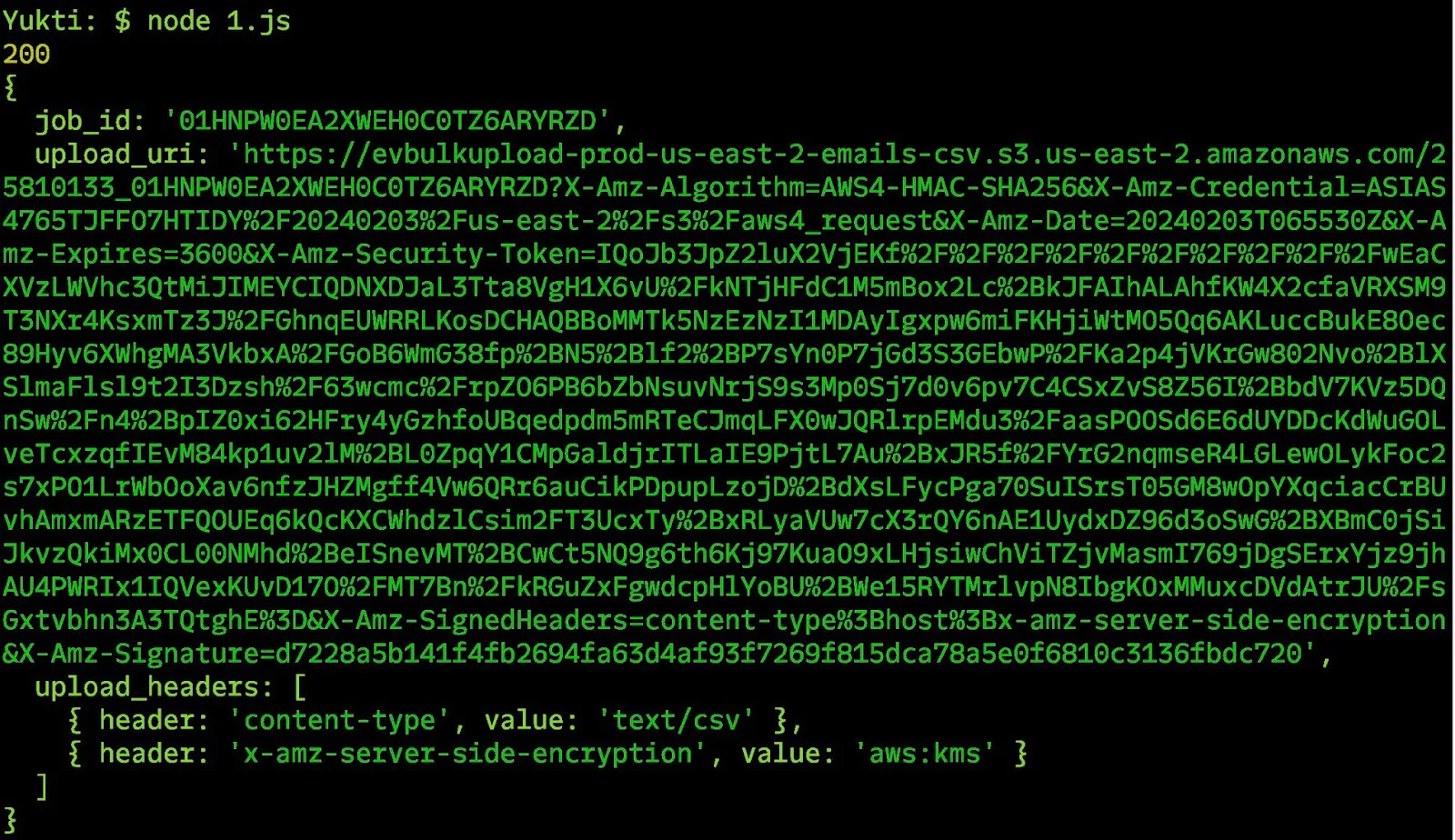
Make a second API call
Once you receive the response body you can then initiate a second API call where you use the supplied URL and HTTP header to upload your file.
File Format
The uploaded file must be saved either as a CSV or ZIP, ensuring alignment with both the file type specified in the initial request and the expected headers in the response containing the URL. The file should have the header "emails" and exclusively contain the email addresses intended for validation.
For csv file sizes please refer here.
Example CSV Contents
Example Node.js code
Example code output
The upload is successful!


Get Job Status List
This endpoint retrieves information on all bulk email address validation operations associated with a user.
Example Node.js code
Example code output
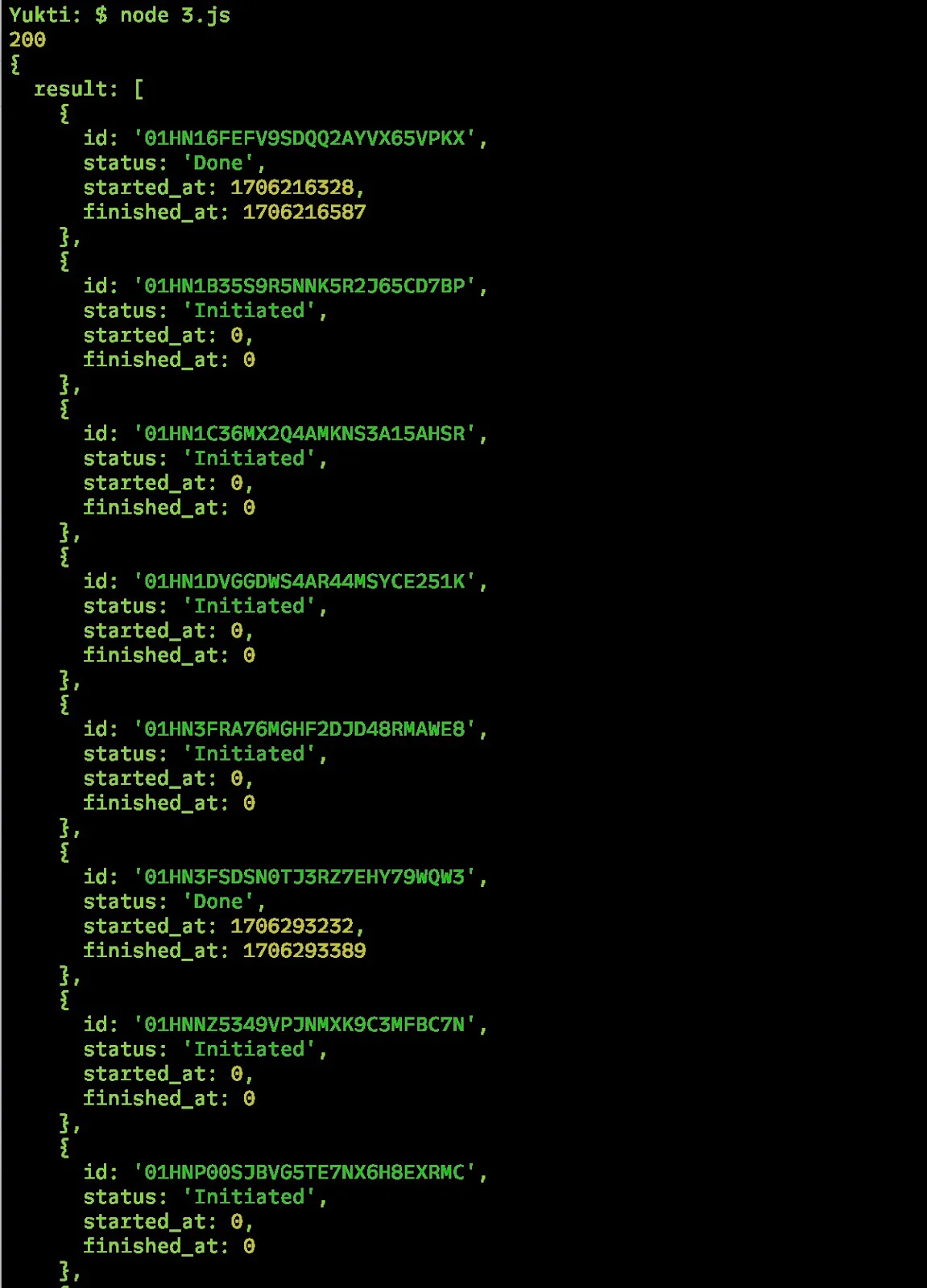
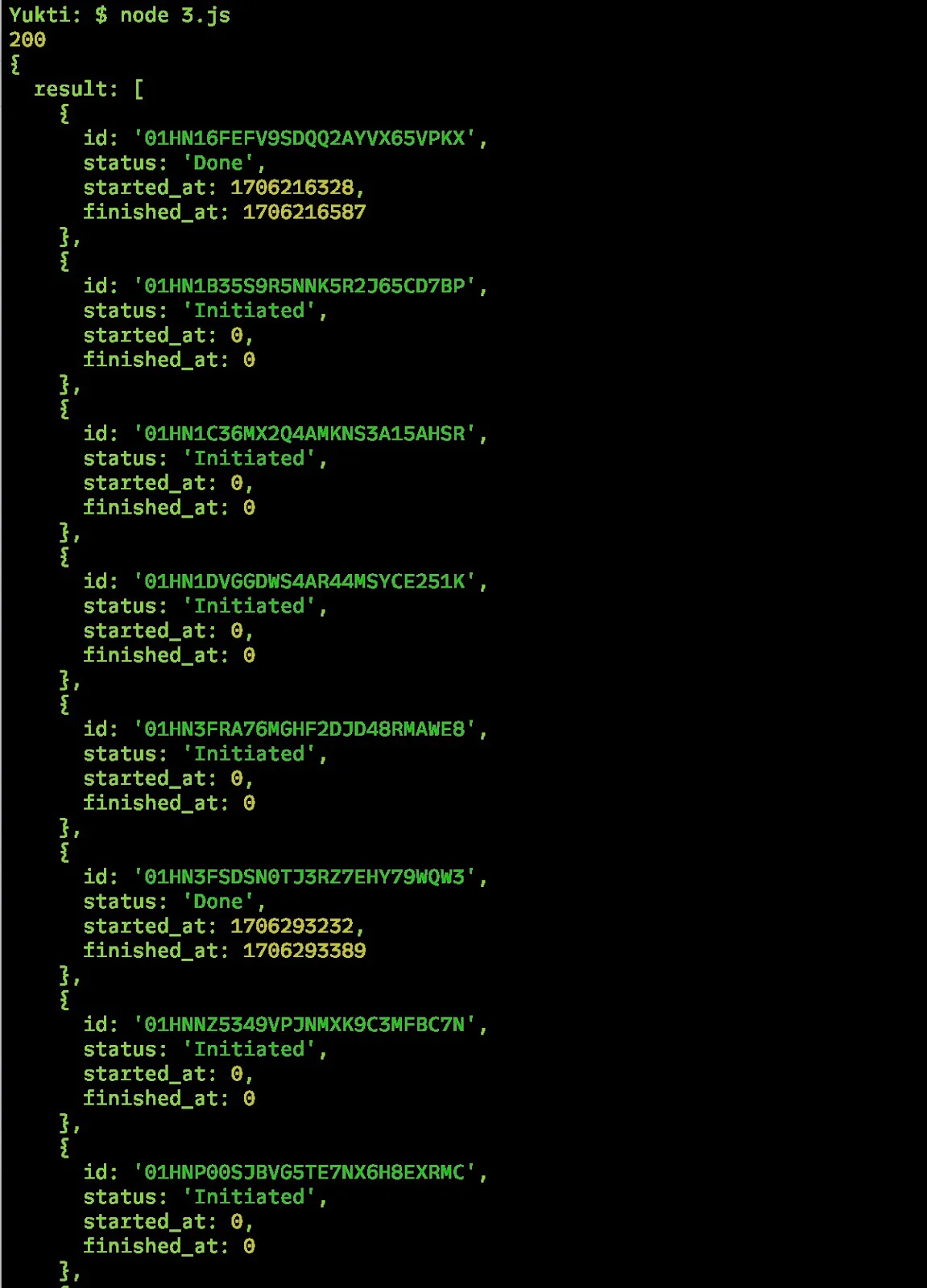
Get Job Status
This endpoint gives you all the details about a specific bulk email address validation job. Take note of the id
parameter mentioned earlier and substitute it in the following code snippet.
Example Node.js Code
Example code output
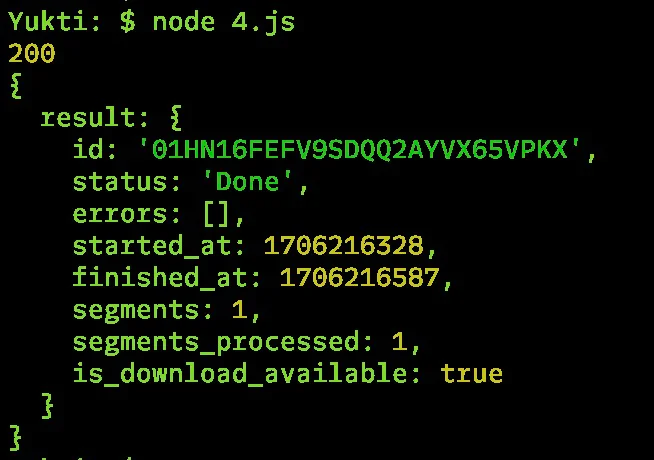
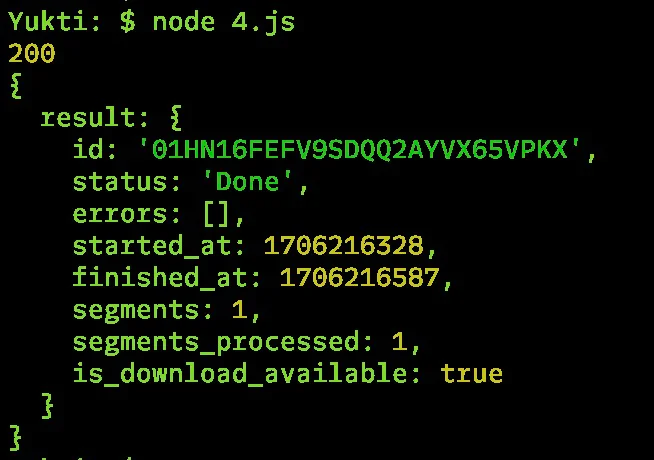
Final Results
Once the job is finished, you'll receive an email in your inbox with a link to download the output CSV. If there's an error during the job processing, you'll also get an email notification in your inbox.
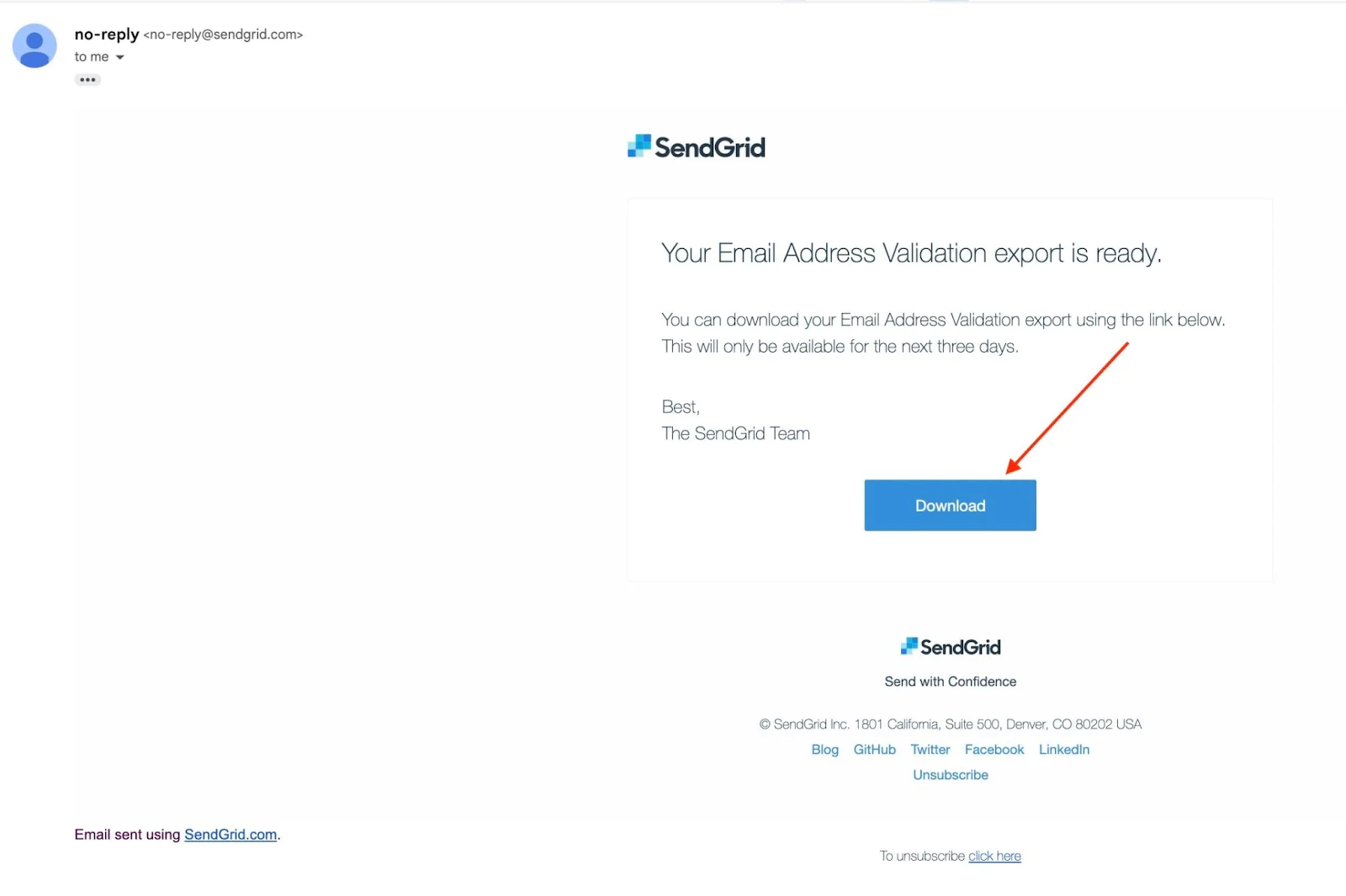
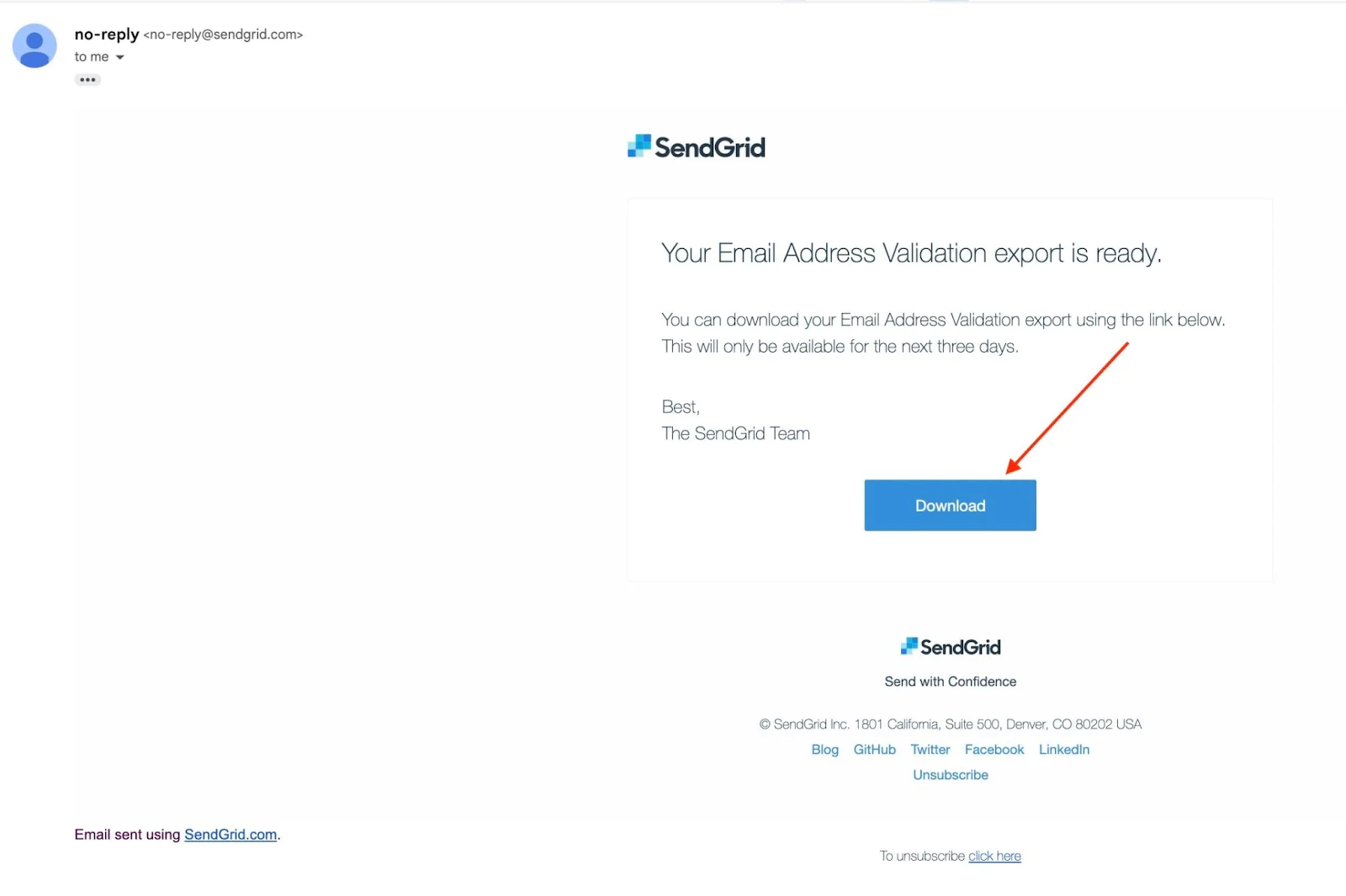
When you click the Download option, the file opens in your SendGrid portal and should initiate an automatic download.
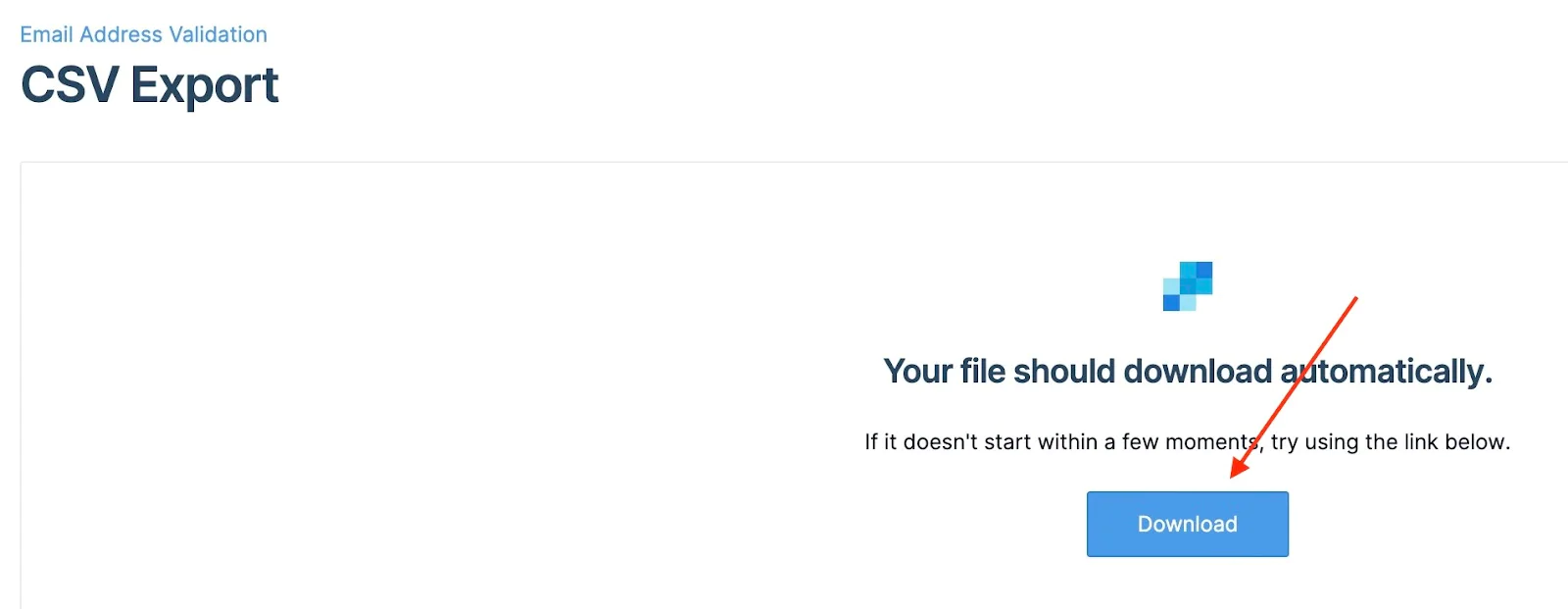
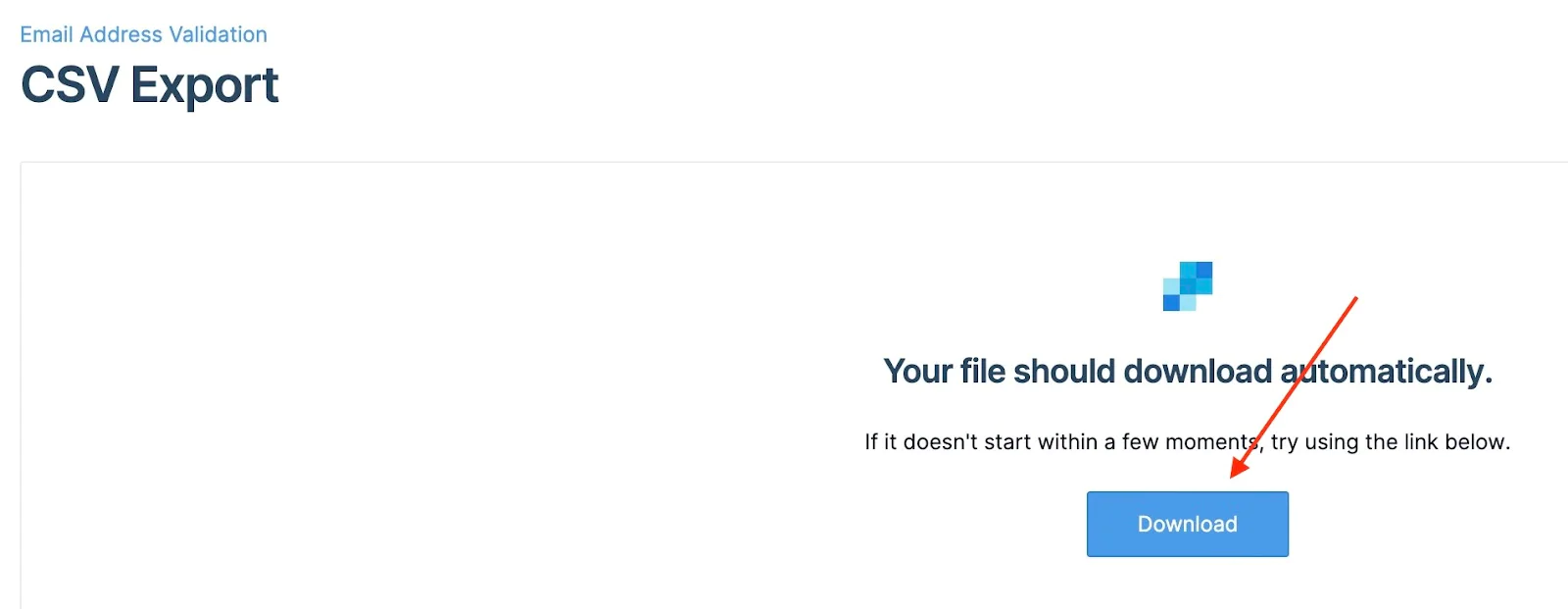
Here is a sample output for my csv:


You can use a mix of these fields to refine your search for what you consider a valid address. Verdict section provides one of three classifications: "Valid," "Risky," or "Invalid." These categories are based on the detailed results. Score is the value ranging from 0 to 1, indicating the probability that the email address is valid, expressed as a percentage. For example, a score of 0.94 could be interpreted as a 94% likelihood that the email is valid.
The API returns a verdict of VALID, RISKY, or INVALID. The score is also returned to provide more fine grain details. A score of 0 maps to INVALID. A score > 0 but < 0.66 maps to RISKY. A score >= 0.66 maps to VALID.
Additional details on interpreting the results can be found here.
Conclusion
Conducting an email address validation check is a proactive measure that positively impacts your long-term email marketing efforts. This not only reduces the risk of emails bouncing but also plays a crucial role in building a positive sender reputation, ultimately boosting the success of your email campaigns. For more information on Single vs Bulk Email Address Validation API please visit the technical documentation here .
Now that you have the capability to programmatically utilize the Bulk email address validation API, I'm excited to see what you build! Don't hesitate to reach out to sendgrid support to share your experiences, or ask any questions .
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.