Deliver XKCD To Your Phone with Node and Twilio
Time to read: 7 minutes
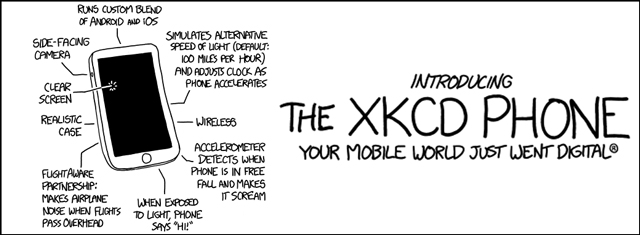
Who doesn’t love XKCD? I know I do. The comic appeals to us engineers since it draws humor from some of the nerdiest math and science subjects. As much as I love to read XKCD, it frequently gets lost in the many RSS feeds in my reader which means I miss new comics as they are published. When Twilio released support for MMS on US and Canadian phone numbers I thought what better use for MMS than to let me push XKCD comics right to my phone, as soon as they are published.
In this post I’ll show you how I built a simple Node application that lets me deliver XKCD comics to my phone via Twilio MMS. The app allows me to request and receive specific XKCD comics, and have newly published comics automatically sent to my phone.
Want to give it a try? Send a text message with the ID of your favorite XKCD to +1 201-654-3624. Need some ID suggestions? Try sending 325, 285 or 149.
Expressions in our Formula
To build the app I’m going to use Node.js and the Express web framework to combine together several different services including:
- Twilio – To send the XKCD comic to my phone I’ll use an MMS-enabled phone number from Twilio. Don’t have a Twilio account? Go sign up for a free!
- SuperFeedr – To monitor the XKCD Atom feed for new comics, I’ll use Superfeedr. Superfeedr will notify me when a new entry is added to the feed by requesting a URL that I’ll expose from my app.
- Firebase – Finally, to keep a list of phone numbers that want to receive the new XKCD comics as they are published, I’ll use Firebase.
Can’t wait for me to explain the code? Grab the completed app from GitHub and get started sending XKCD today. Otherwise read on and I’ll walk you through how I built it.
Comics On Demand
I started building my comic delivery application by creating an endpoint which accepts a string representing the ID of a specific XKCD comic from an incoming text message, looks up that comic from xkcd.com and returns the comic image, title and alt text as a MMS message.

Lets start building the application by running the npm init command:
npm init
This will create a new package.json
file with the basic configuration for our application.
Now lets add the set of dependencies that the application will use. You can do this using npm by running the following command:
npm install express body-parser request twilio --save
This command installs the express, body-parser, request and twilio pages from npm and populates the dependency section of the package.json file for us.
Once the dependencies are installed its time to write the application code.
Start by creating a new file named app.js
and declaring which libraries are required in this file:
With the required libraries added, create a new instance of Express. Since requests coming into the application will contain both json and form-encoded data, we can configure the Express app to use the body-parser library to parse that data for us:
Finally start an instance of the Express application:
At this point feel free to run your application and make sure the server starts. You’ll know its running correctly if you see the text “Server started!” output to the console.
With the Express application configured and started we can create a new route named process. As the name implies, this route will process incoming text messages from Twilio.
The code in this route checks to see if the text message contains a number and if it does, uses the Request HTTP client to get a JSON representation of that specific XKCD comic:
If a JSON representation for that ID is found the application uses the Twilio Node helper library to generate and return TwiML that tells Twilio to respond to the inbound text message with an MMS message containing the comics image, title and text.
If the incoming text message did not contain a number or no XKCD comic was found for the number received, then a response message telling the user to try again is generated and sent back to the user.
Thats it! All of the code needed to request delivery of a specific XKCD comic is now in place. All that is left is to point a Twilio phone number at this newly created URL. To do this we will buy a new MMS-enable US or Canadian phone number and configure its Message Request URL with the URL of the application.
But wait! How is Twilio going to be able to make a request to a node website running on your local computer? Through the magic of ngrok which we can use to expose our newly created website out to the internet via a public URL.
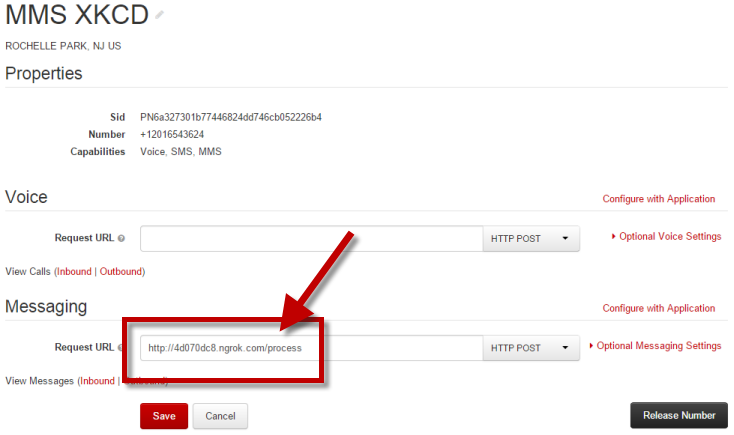
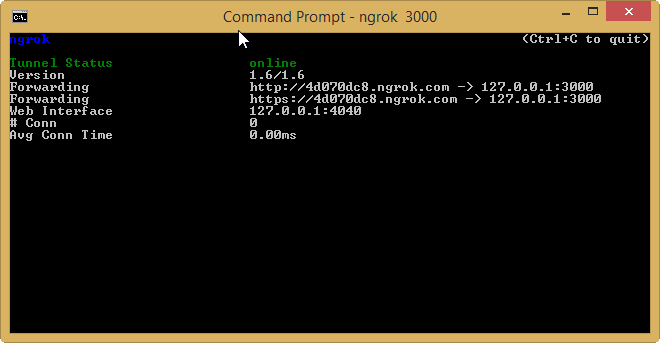
Awesome! If you are following along you should now be able to receive XKCD comics on your phone. Send a text message containing your favorite XKCD comic number to your Twilio phone number and if its a valid XKCD comic ID, you should receive a response containing the comic image, title and alt text.
Comic Delivery
Sending and receiving specific XKCD comics is pretty cool, but it still does not solve my original problem of missing newly published comics. To fix that, lets modify our application to be a bit more proactive and have it push newly published comics directly to me, or anyone else who wants subscribe.
To do this we’ll need to add two new pieces to the application. First we’ll need a way to store a list of users who want to receive new comic deliveries. For that we’ll use Firebase. Second, we’ll need a way to watch and be notified when a new XKCD comic is published. For that we’ll use a cool service named SuperFeedr.
Lets start by modifying our application to allow users to subscribe to new comic deliveries by storing their phone number in Firebase. Begin by adding the Firebase package to the application:
npm install firebase --save
With the Firebase package installed in our application we can require it in the app.js file:
Next, we need to get the list of phone numbers that we already have stored in Firebase. To do this create a new instance of the Firebase client, passing in the URL that points to the location in Firebase where you are storing your list of phone numbers.
Notice that I’ve also attached an event handler that will be called whenever a new phone number is added to Firebase.
With the list of phone numbers received, we can modify the existing process route to accept the new command ‘subscribe’, which tells the application to save the users phone number into Firebase if its its not already there.
Fantastic. We’ve now got a list of phone numbers that we’ll send new comics to as they are published. Now we just need to set up SuperFeedr to let us know that happens.
Configuring SuperFeedr is easy. Once logged in to the site open the PubSubHubbub Console tool and make sure Mode is set to Subscribe
. Drop in the URL for the XKCD Atom feed and then add a URL that points to a new route in our application named relay. We’ll create that new route in just a bit. Finally check the Convert to JSON box.
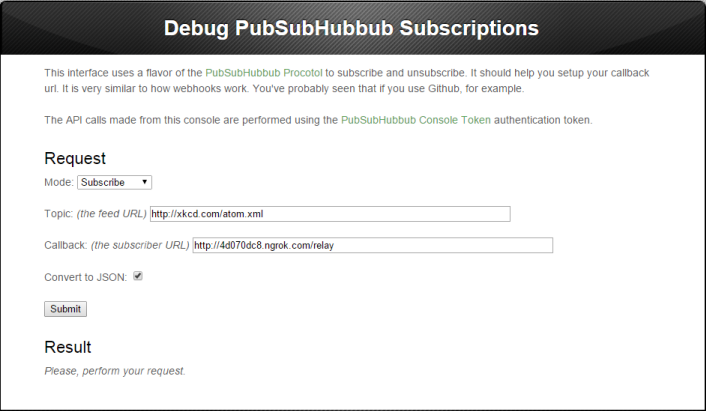
Its the job of the relay route to take the new comic notification data from SuperFeedr, parse it and then send out MMS messages to all of the subscribers in our list of phone numbers.
Even though we configured SuperFeedr to send us JSON, some of the Atom content that we need remains formatted as XML. To parse that remaining XML, we need to add one final package you our application named xml2js:
npm install xml2js --save
In app.js require the library:
With xml2js installed we can add the new relay route to the app.
The code in this route is pretty straight-forward. We loop through all of the new entries sent to us from SuperFeedr and parse the XML snippet included in each, extracting the comics image source and title.
Next we loop through all of the phone numbers stored in Firebase and use the Twilio Node helper library to send each one an MMS containing the comic image, title, text and permalink.
And that’s it. Now each time the XKCD Atom feed adds a new entry, SuperFeedr will tell our application about it and we will send out text messages with the comic to all of our subscribers.
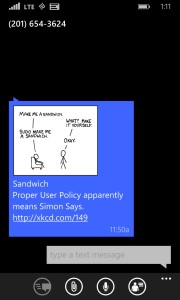
Wrapping It Up
Congratulations! By combining a little bit of Node and some awesome services like Firebase, SuperFeedr and Twilio, we’ve built an XKCD delivery service. Now I’ll never miss another newly published XKCD again, and neither will you!
Grab the code from GitHub and host your own delivery service, or just send a text message to +1 201-654-3624 to subscribe to mine. And when you do, send me an email or hit me up on Twitter and let me know what your favorite XKCD is.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.