Giphy and Twilio Text A GIF Using RapidAPI
Time to read:
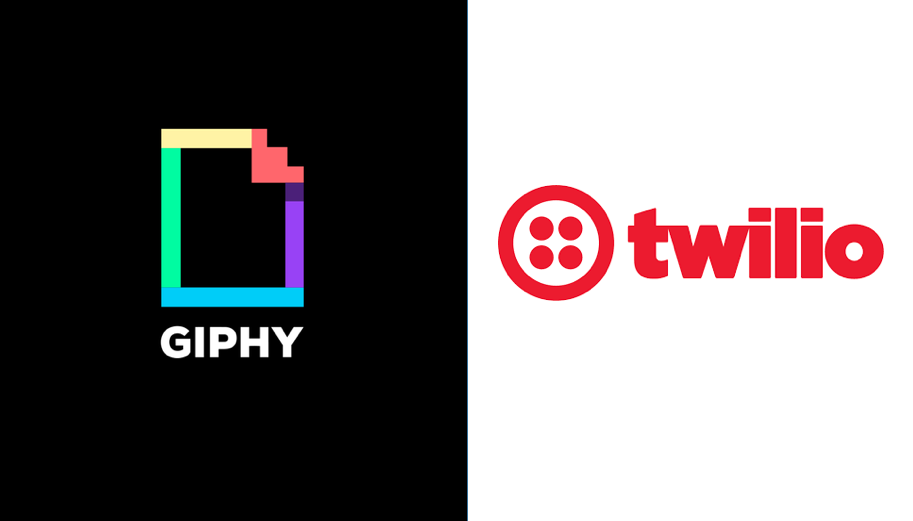
This post originally appeared on the RapidAPI blog by Lindsey Kirchoff. RapidAPI is a marketplace where developers can test and connect to multiple APIs from one endpoint. Lindsey created an API Smash using Twilio SMS and Giphy to text GIFs.
One of the reasons that we built RapidAPI is to make it easier to call multiple APIs through one console. No more juggling multiple API libraries!
We’re starting a series where we smash two APIs together to make a project. Today? We’re putting together Giphy and Twilio to make “Text A GIF” script that texts someone a GIF based on a user-input phrase.
Demo: “Text a GIF”
We created a “Text a GIF” app that runs in the Terminal. The user types in a phrase and a phone number and the “Text A GIF” app sends a related, random GIF. See below for a demo!
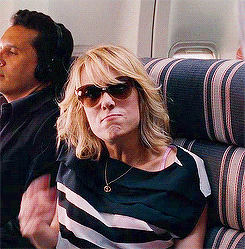
Aaaaaand here’s the source code. You can also find it on GitHub.
We built this project by exporting JavaScript code snippets from RapidAPI’s Giphy and Twilio API packages. You can recreate this app in your preferred language by filling in the parameters on RapidAPI and exporting the snippet in the language of your choice (ex. Python, PHP, Java, Objective-C and cURL).
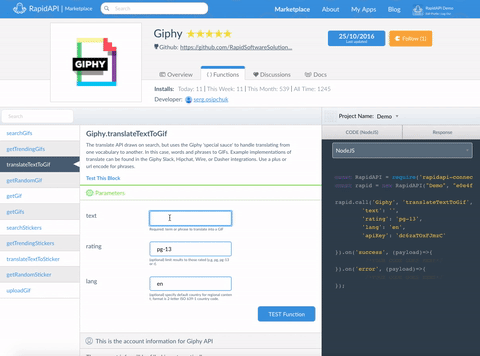
Now, here’s how we built it!
Step 1: Storing user inputs in variables
The first lines of code call RapidAPI. (If you haven’t already installed it, you’ll be walked through that process when you sign up for a free RapidAPI account).
Next, the “Text a GIF” app requires the user to input text and a phone number from the command line. The following lines of code store these user inputs into two variables.
Note: In order for Twilio to read the phone number, it must be written in E.164 formatting. All that means is “ ” then country code, then number, with no dashes or spacing. For example, 18008675309.
Step 2: Connecting to the Giphy API
How to Call the Giphy API
Our next step was connecting to the Giphy via the RapidAPI package and exporting the code snippet in JavaScript.
The endpoint we used for this app was translateTextToGif
. This endpoint generates a GIF based on the word that you type in. You can even filter the results by rating (G, PG, PG-13, R) and language (using the ISO 639-1 country code—English is “en”).
You can test this endpoint right within your browser. Type in the parameters above and use dc6zaTOxFJmzC as an access_token
. Giphy provides a public beta key for anyone to use. (If you want to submit an app for production, you can request a production key here.)
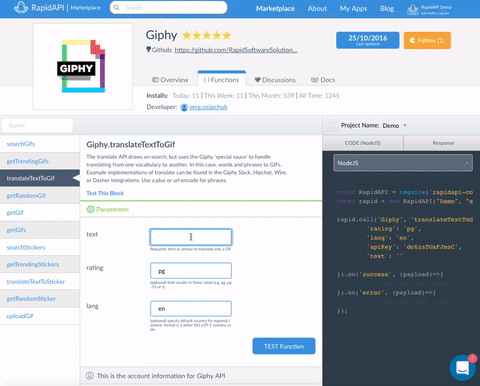
Incorporating the Giphy API into the code
Using the translateTextToGiF
endpoint, the Giphy API generates a GIF based on the text the user input in the Terminal (in this case, gifText
).
If the call is successful, the script will call Twilio’s API to text a MMS with the generated GIF. If the call isn’t successful, the script will read an error message that lets the user know the problem was with the GIF generation.
Step 3: Call the Twilio API
How to call the Twilio API
Our next step was connecting to the Twilio API to send a text message. In order to connect to the Twilio API, you’ll need an accountToken
, accountSid
, messagingSid
and to generate a Twilio phone number. These can all be generated for free in the Twilio console . Here’s a quick post on how to get the credentials that you need.
Note: If you generate a free “from” phone number with your Twilio developer account, then the phone number you associate with the free “from” number is the ONLY one you can send messages to. In this case, the “Text A GIF” app will only send GIFs to your phone number. In order to send a message to any number, you must purchase an upgrade (see more in the Twilio developer console).
Incorporating the Twilio API into the code
For the “Text a GIF” project, we called the sendMms
endpoint. Using this endpoint, Twilio can send a multi-media message to the phone number the user entered (in this case, the variable phoneNumber
).
The Giphy API translateTextToGiF
endpoint returns a variety of data. To store the image URL, we created a variable called gif
. (Scroll down through code to display).
If the Giphy API call is successful in generating a GIF, then the Twilio API will retrieve the image URL (gif
) and text it to the phone number the user inputted initially (phoneNumber
). The script will then prompt the user with “Check your phone!” If the Twilio API call is unsuccessful, the script will return the prompt “Error sending MMS.”
Ta-da!
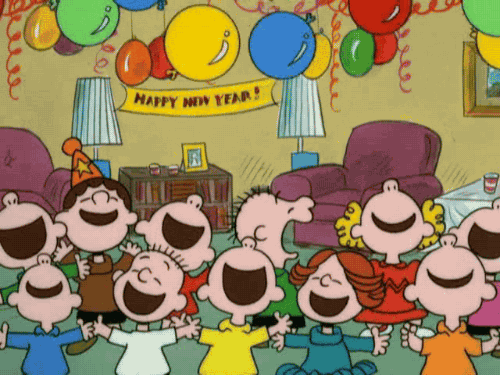
This article wouldn’t be complete without at least one celebratory GIF. Let us know what you think of this project or the Giphy API in the comments below. Or give us some suggestions on which APIs we should mash up next!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.