Build a Customer Review App with Twilio, HTMX and Go
Time to read:
Build a customer review App with Twilio, HTMX and Golang
Getting customer feedback is critical for any company looking to improve its products and services. That is why having a customer review application provides an interactive way to collect valuable insights, while improving user engagement.
In this tutorial, I’ll walk you through the process of building a dynamic customer review app using Golang, HTMX, Twilio, and Gemini.
Prerequisites
Before we proceed, ensure that you have the following:
- Basic knowledge of Go and its concepts
- Go version 1.22 or higher
- A Twilio account (either free or paid). If you are new to Twilio, click here to create a free account.
- A Twilio phone number
- A Google account with permission to create an API key, such as a personal Google account
How the application works
Here’s a brief description of how the app will work. The user completes and submits a form with their name, description, and rating. Once submitted, Gemini runs a Sentiment Analysis of the feedback and provides a response based on it. Twilio then delivers an SMS notification to the product owner with the rating submission.
Get an API key from Gemini
To use Gemini for sentiment analysis, you need an API key, and there are two ways for that. The first is through Google’s AI Studio. The second is through the Google Cloud console.
Through Google AI Studio
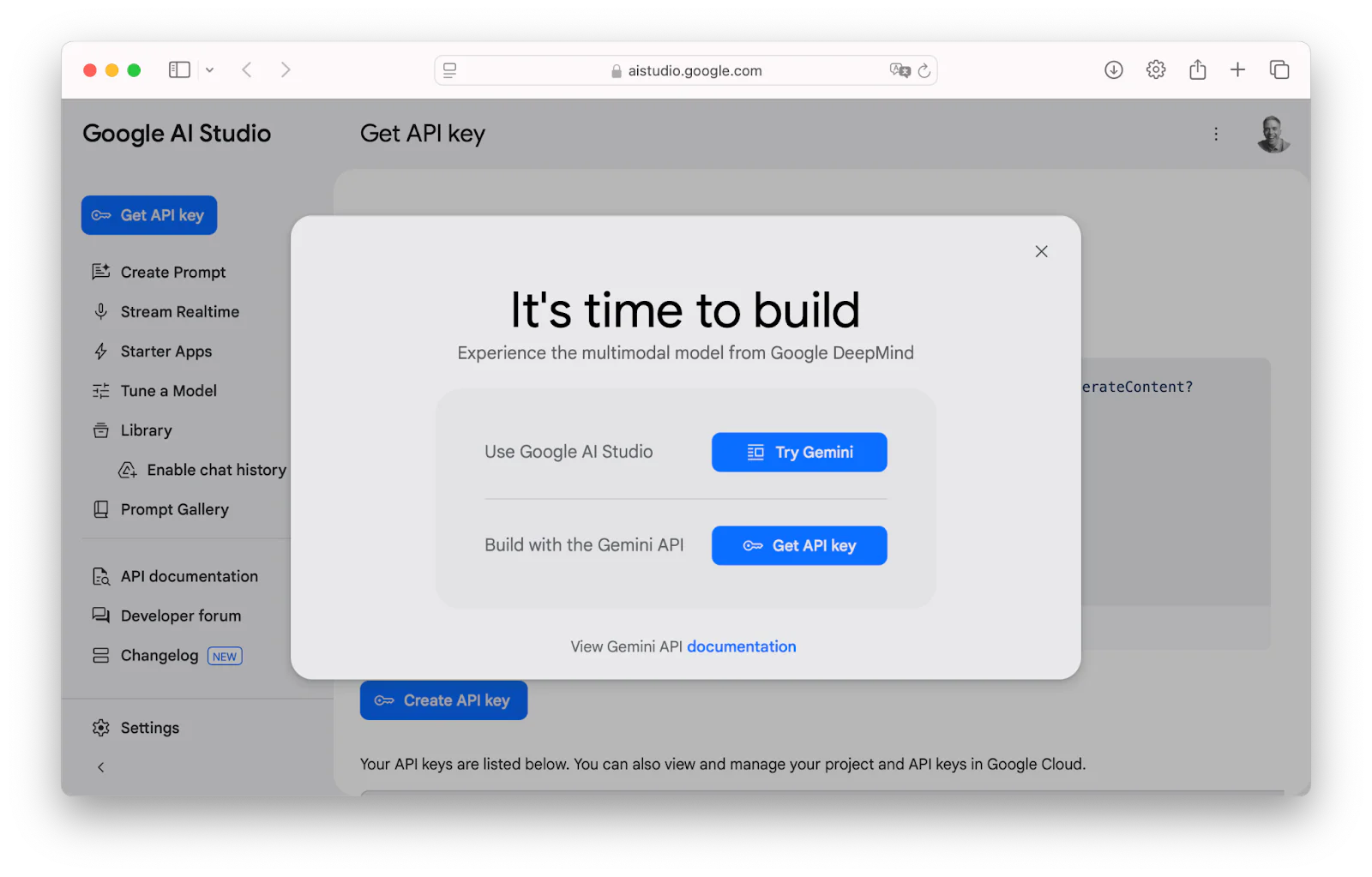
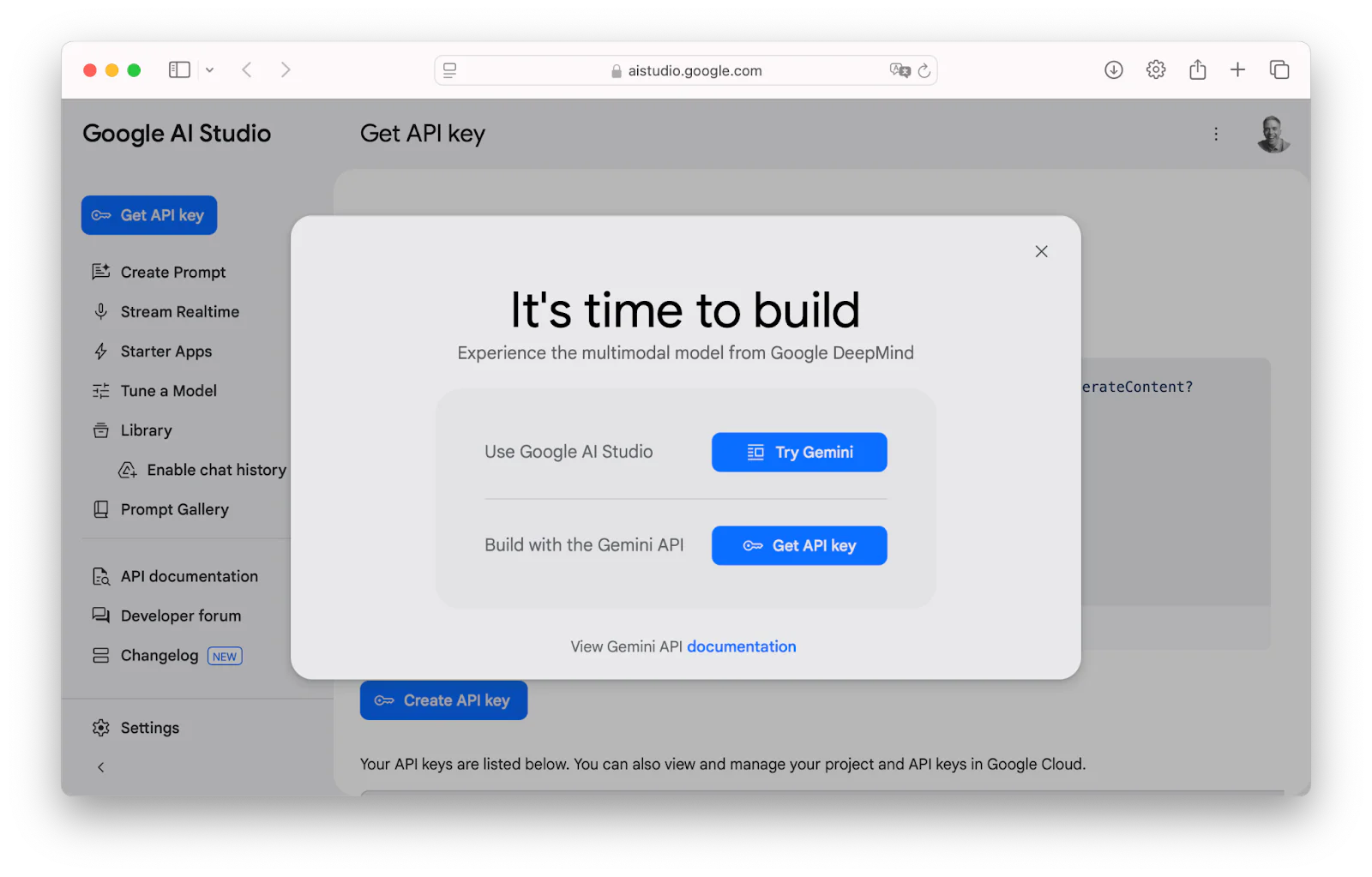
To create an API through Google Cloud Console, head over to the Get API key section of Google AI Studio.
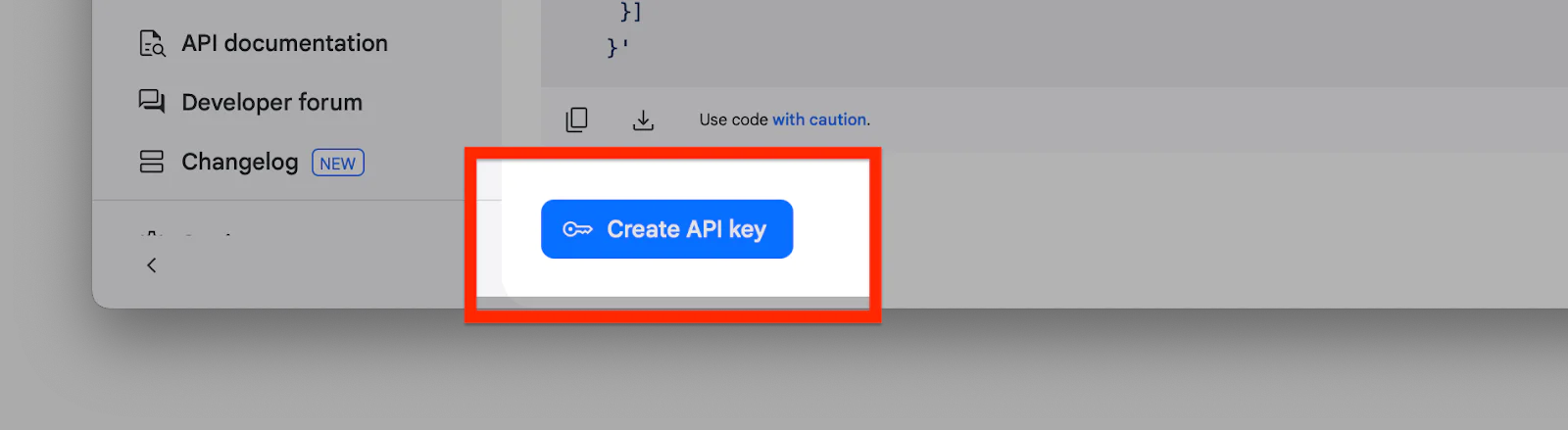
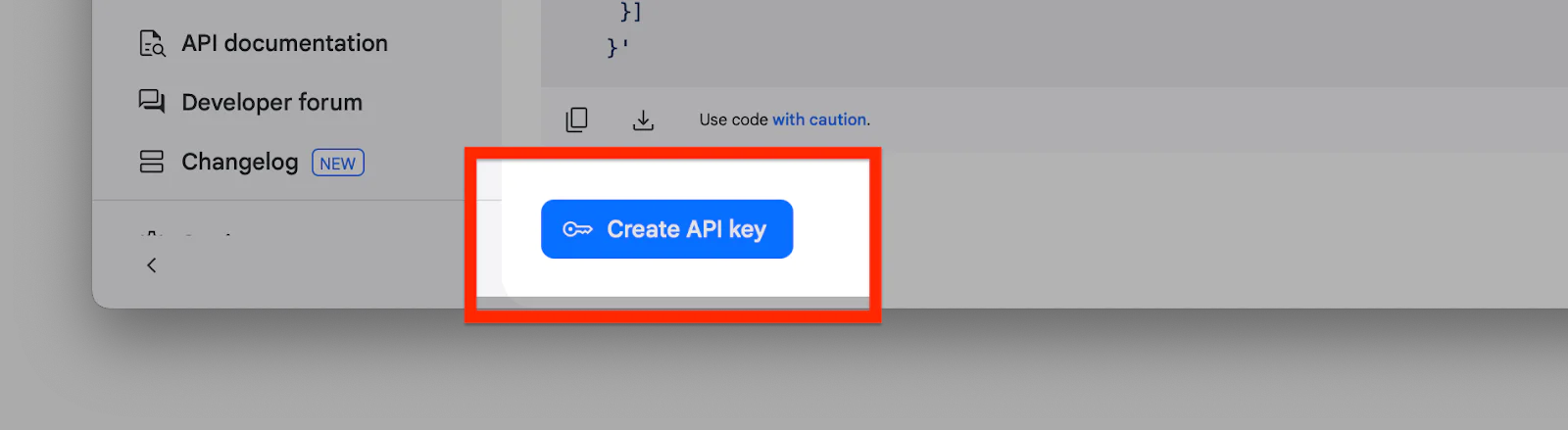
There, click on GetCreate API key. Then, click Create API key to create your API key.
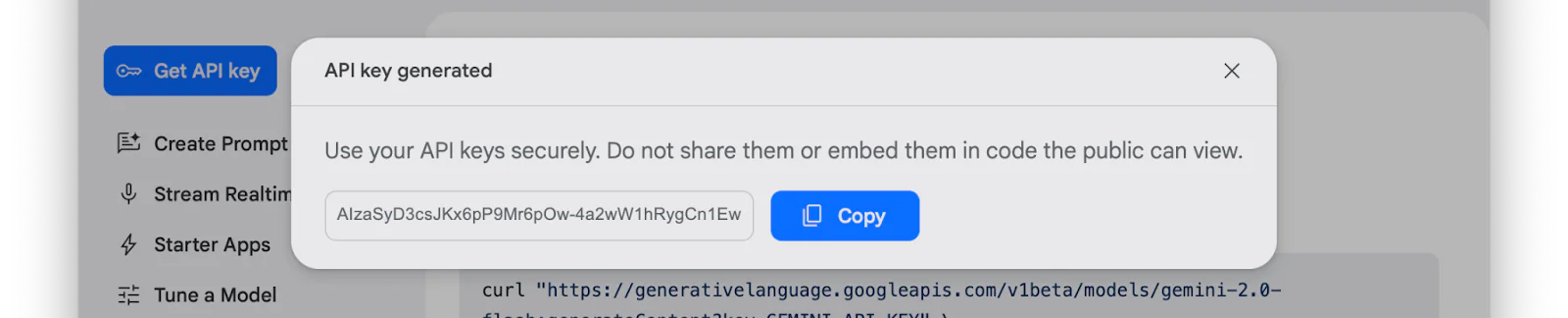
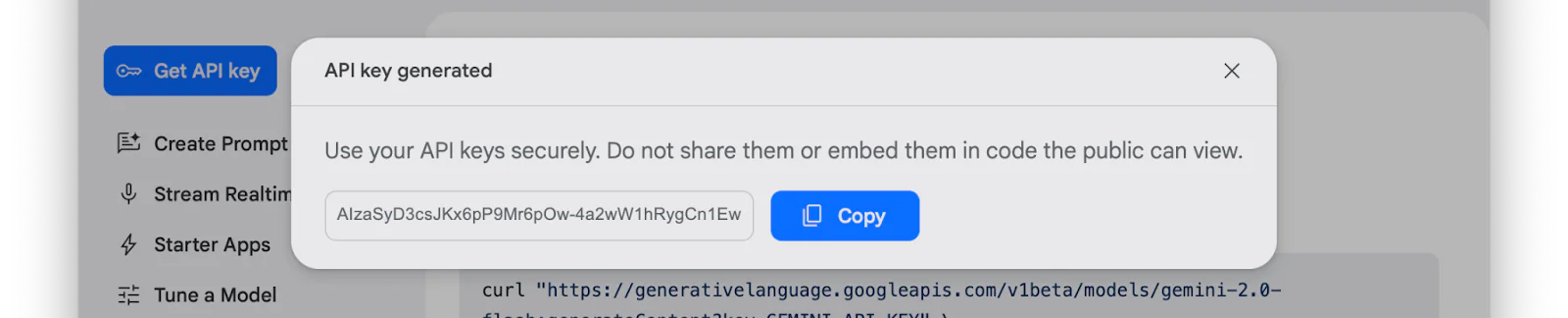
After that, store the API key somewhere safe, as you’ll need it later.
Through Google Cloud Console
To create an API key using Google Cloud Console, navigate to the API credentials page, login to the Cloud Console dashboard. There, pick an active project. Then, in the navigation menu, click APIs and services > Credentials.
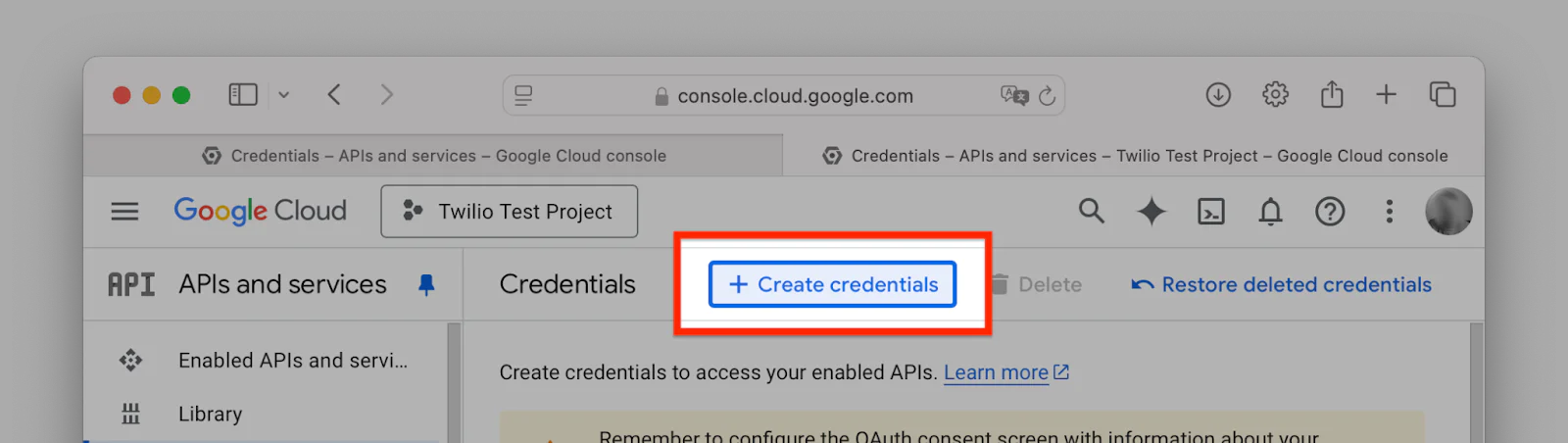
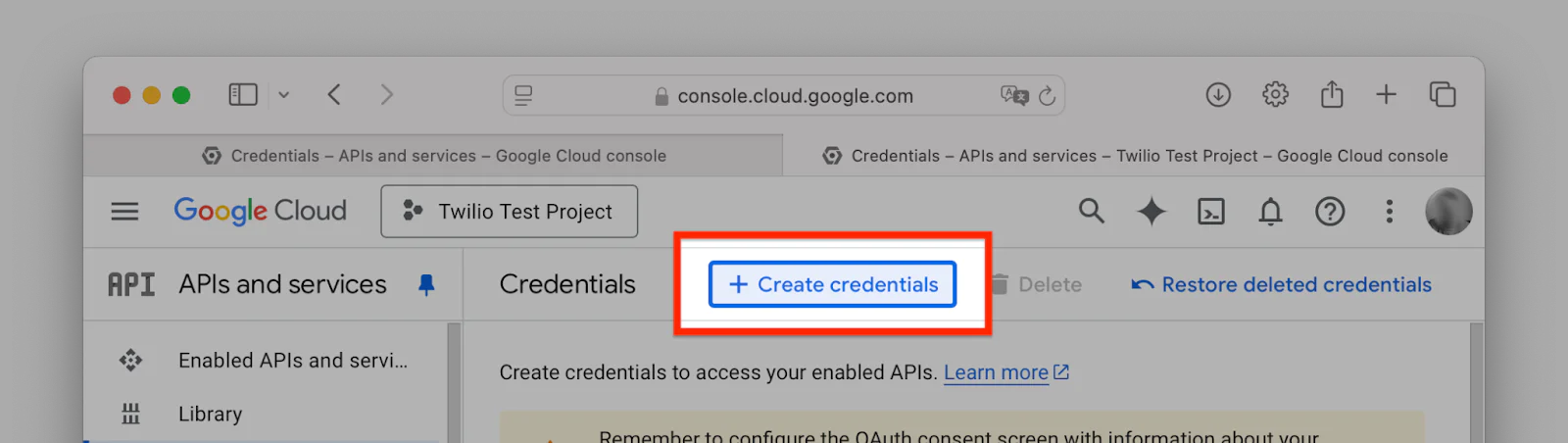
Following that, click the Create Credentials button at the top, and in the drop-down menu, click API key:
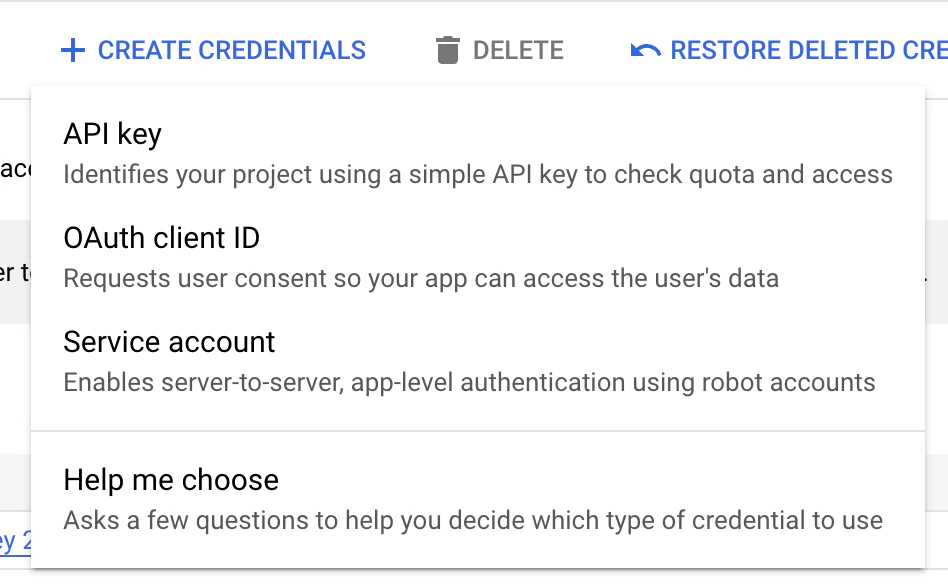
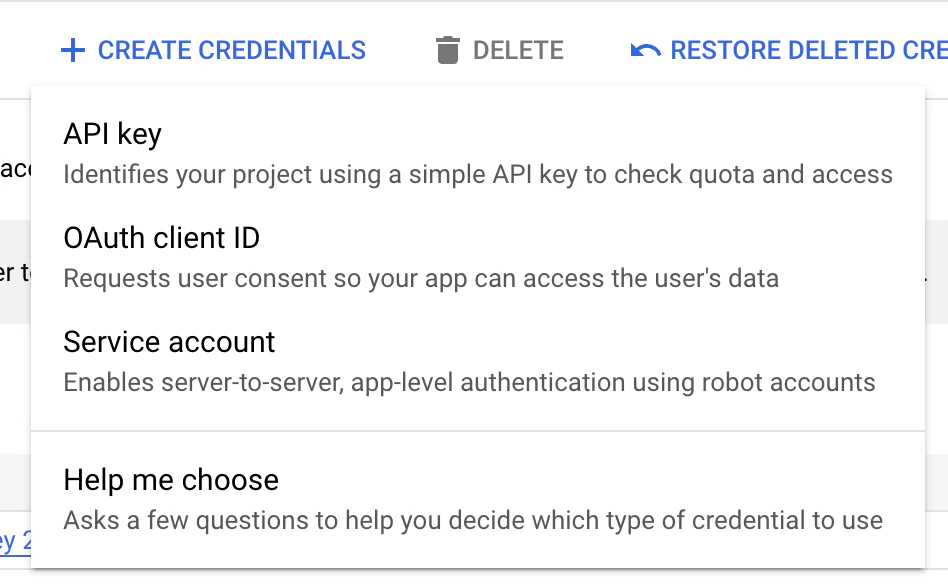
After that, store the API key somewhere safe, as you’ll need it when you start writing codes.
Create a new Go project
Now, let's get started building the application by creating a new project directory (wherever you create your Go projects) and initialising a Go module inside it, by running the commands below.
Add the environment variables
Next, you'll need the Gemini (Google) API key you created earlier, your Twilio credentials and Twilio phone number, and the phone number to which you want the message to be sent.
Create a file named .env in your project folder, then copy the configuration below into the file.
Then, replace the <<your_account_sid>>
<<your_auth_token>>
, and <<your_twilio_phone_number>
with your Twilio Account SID, Auth Token, and phone number, respectively. You can find them in the Account Info panel of the Twilio Console dashboard.
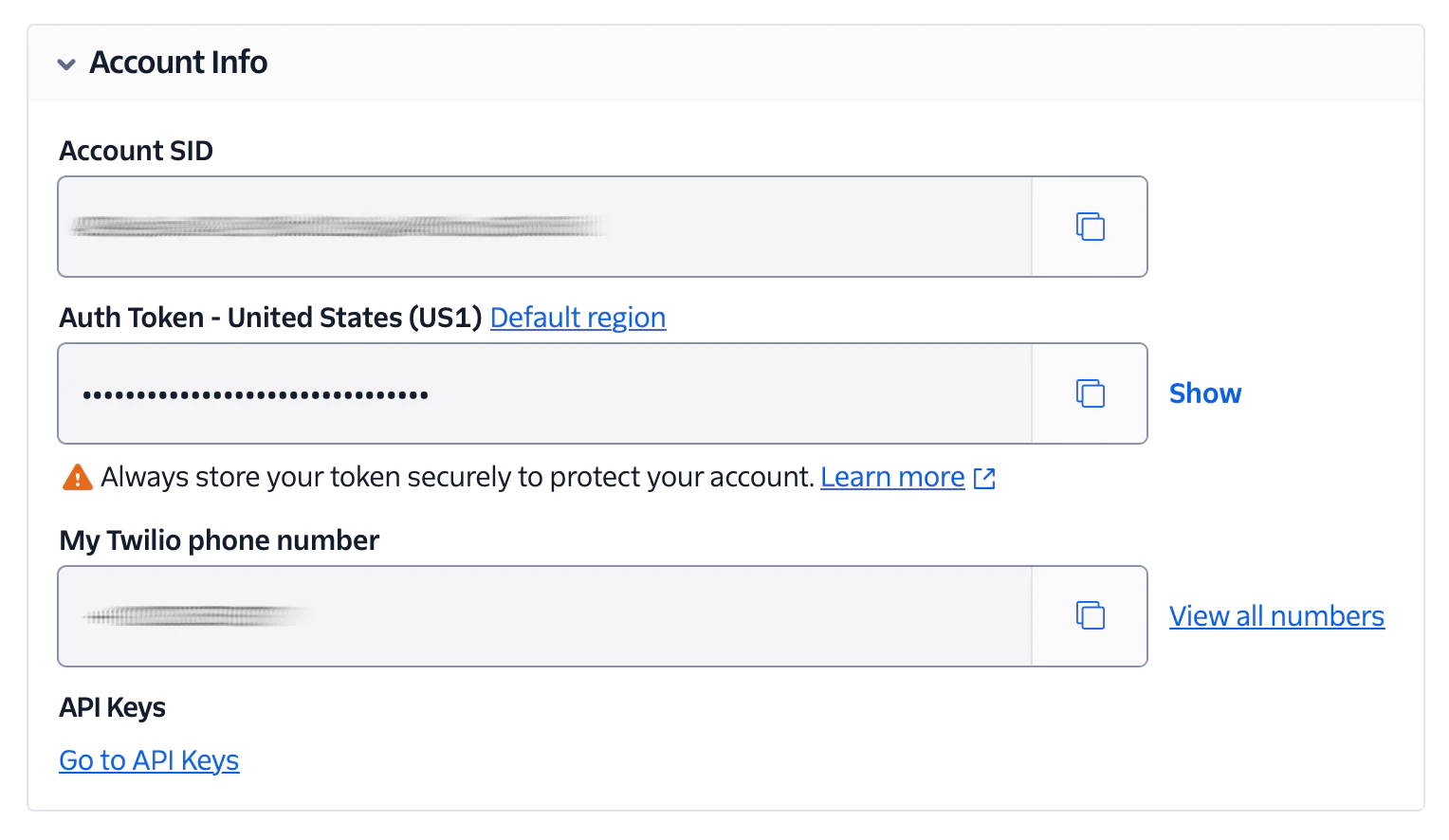
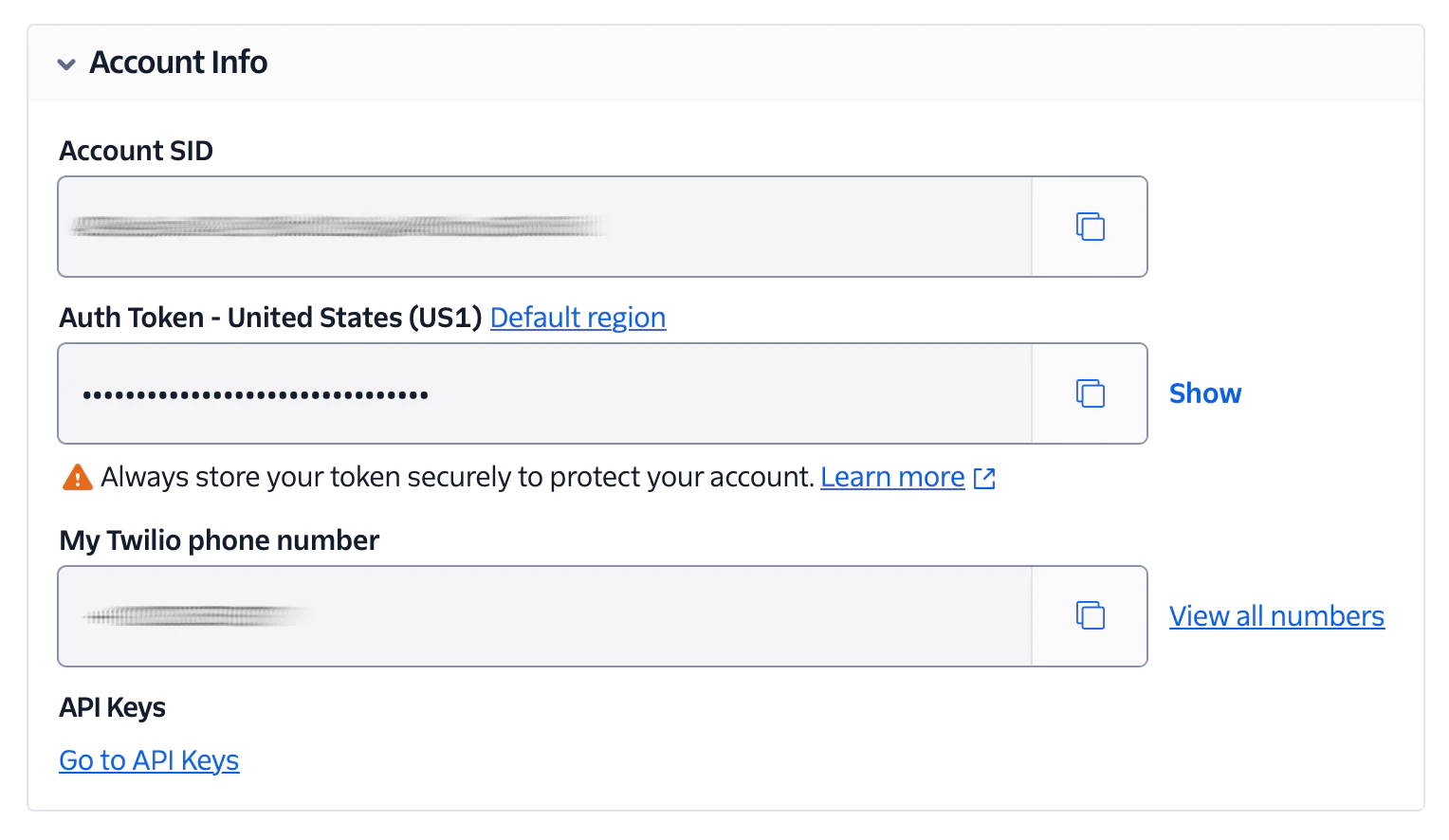
Next, replace the <<recipient_phone_number>>
placeholder with the phone number that you want the message sent to. Finally, replace <<your_Gemini_API_key>>
with the API that you created and copied, earlier in the tutorial.
Install the required dependencies
You need to install the dependencies needed for this project, namely:
- github.com/google/generative-ai-go/genai: This is a Go client library for interacting with Google's Generative AI API. It allows you to integrate Google's AI-powered generative models into your applications, enabling you to use it for any AI functionality you choose. In this case, it'll be used for sentiment analysis.
- github.com/mattn/go-sqlite3: It registers the SQLite driver, which allows you to utilize SQLite as the database backend to store customer reviews.
- github.com/twilio/twilio-go: This is the official Twilio Helper Library for Go, which simplifies interacting with Twilio services like SMS, voice, and other communication tools. For this project, it will be used to send SMS notifications to the product owner, whenever a new review is submitted.
- google.golang.org/api/option: This package provides client options for Google APIs. It is often used to configure settings such as authentication (e.g., providing credentials via API keys or service accounts), timeouts, and other options when interacting with Google services. This project will use it to configure the Gemini API key.
- github.com/joho/godotenv: The GoDotEnv package will help us manage our environment variables. This is optional because you might decide not to use this method, but set the environment variables in your terminal before running the Go application.
To install the dependencies, add the following:
Let’s proceed to build the project.
Create the static and template files
The static files are the HTML and CSS files while the templates files are Go’s HTML templates. For the static templates, create a subdirectory in your root directory called static. This will contain both the HTML and CSS files. Inside this directory, create an index.html file and paste the following code:
Also, create a styles.css file inside the static directory and add the following code:
For the template, create a subdirectory inside the root directory of your project called templates and inside it, create a file called success.html. Add the following code:
That's all for the static files. Let’s move on to the Go part.
Build the backend
Create a file called main.go and paste in the following code:
In the code above, the initDB()
function initializes an SQLite database. It also ensures the database file, named reviews.db, exists and then creates a table for storing user reviews if it doesn't already exist. Each review contains an ID, name, rating, and description.
Initialize Gemini
Now, you need to declare a global variable that will be used to interact with the generative model. To do this, add the code below at the top of main.go — after your imported dependencies:
Then, add a function that initializes the Gemini AI client using the API key fetched from environment variables. Right after the database function, add the following code below:
Create the sentiment analysis function
You next need to create a function that sends a user review description to the Gemini model for sentiment analysis. To do this, add the following code right after the initGemini()
function:
This function responds with one word: "Positive", "Negative", or "Neutral", based on the sentiment of the review.
Send an SMS with Twilio
You now need to create a function that sends an SMS via Twilio when a new review is submitted. To do this, paste the following code below the sentiment analysis function:
The message will contain the reviewer's name and the star rating, which will be sent to the product owner's phone number.
Handle form submission
Almost done. You need to create a function that handles and processes the form submission. Add the following code to your code below the sendTwilioMessage()
function:
The code above parses the form data, saves the review to the database, calls the sentiment analysis function, and sends an SMS notification. It also sends a response back to the user, including a sentiment message which is based on the customer’s opinion about the product.
Create the main function
This is the last piece of the project. You need to create the main()
function. This function will:
- Initialize the connection to the database and the Gemini API
- Set up the HTTP server
- Listen for incoming requests
Right after the submitReview()
function code, add this:
And we are done!
You can access the full code on GitHub.
Test the application
To test the application, run the following command:
After that, navigate to http://localhost:8080/ on your browser. When the user inputs the feedback, he gets a response analyzed by Gemini based on his feedback. The product owner also gets an sms message notification about a customer rating the product.
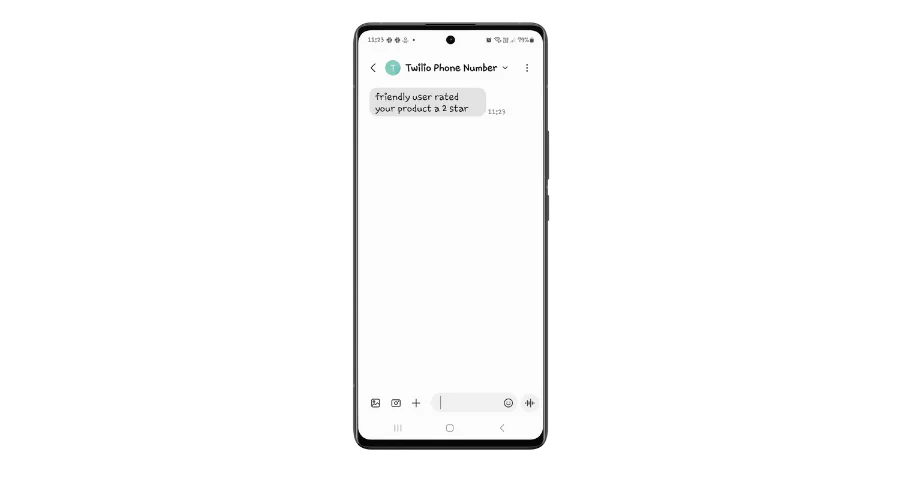
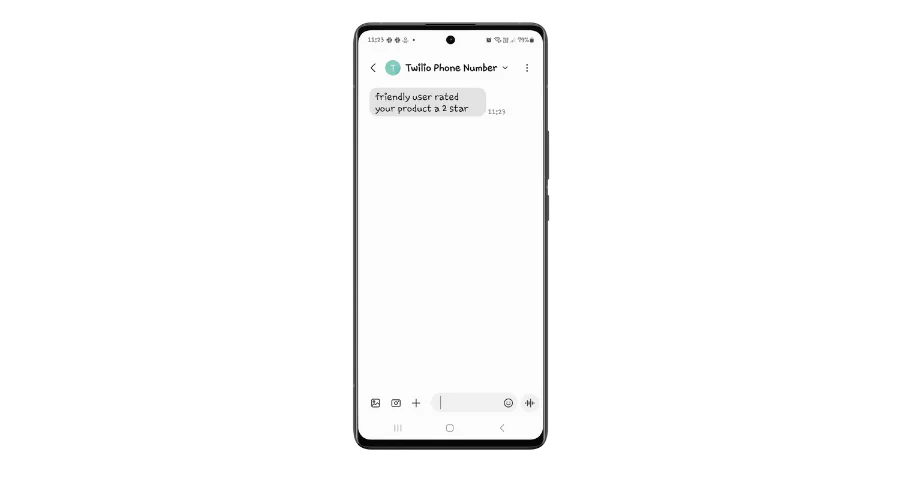
That’s how to build a product review system in Go
In the tutorial, we examined how to build a product review system using HTMX, Golang, Gemini, and Twilio. We created the form using HTMX, used Golang as the backend, used Gemini for sentiment analysis, and sent SMS using Twilio.
Communication is key to every business, and Twilio understands this. That's why it's at the forefront of providing the best platform for communication and also engaging with your customers. When you think of communication, think of Twilio.
Temitope Taiwo Oyedele is a software engineer and technical writer. He likes to write about things he’s learned and experienced.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.