How To Validate and Look Up Phone Numbers in Google Spreadsheets
Time to read:
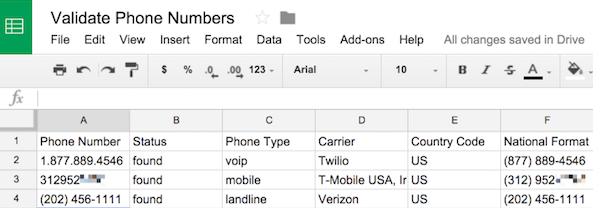
In ten minutes, you can teach your spreadsheet some new tricks, even if you’ve never written a line of code. We’ll use Google Spreadsheet and Twilio Phone Number Lookup API to validate, clean up and format phone numbers easily.
The Twilio Lookup API can be used to:
- Tell if phone number a cellphone or landline.
- Tell if a phone number is real or fake
- Clean up incorrectly formatted phone numbers
- Properly format a list of phone numbers
The Twilio Bits
If you don’t have a Twilio account, sign up here. A free trial account gives you $20 in Twilio credit to play with. At $0.005 per lookup, you can look up 4,000 phone numbers before upgrading your account.
Once you’re signed-in, go to your dashboard and click Show API Credentials. Keep your credentials handy — we’ll be pasting them into our code in a minute.
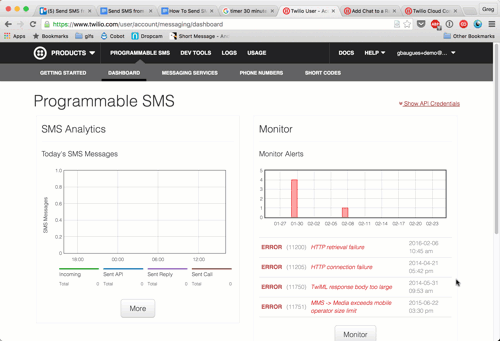
The Google Bits
Go to Google Drive and create a new spreadsheet. Create column headings for:
- Phone Number – this is the column you fill out.
- Status – is the phone number is real (found), or fake (not found)?
- Phone Type – is this a cell phone number? Can it receive SMS? (if this is interesting to you, check out this tutorial on sending SMS with Google Spreadsheets).
- Carrier – Which mobile or landline carrier operates this phone?
- Country Code – Is this an international phone number? If so, what country it from?
- National Format – What is the proper formatting of this phone number?
Select all of the cells in your spreadsheet and format them as “Plain Text”.
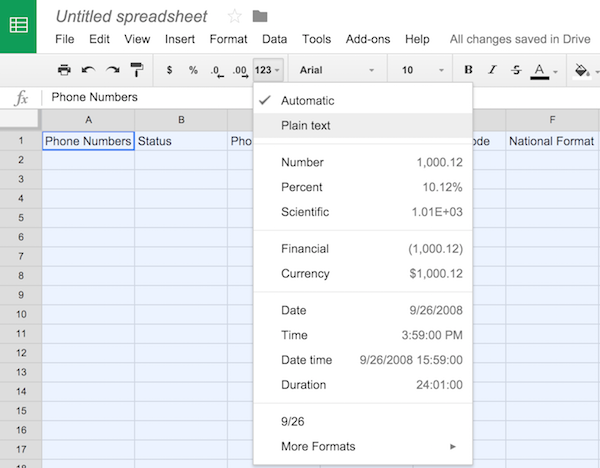
Add a few phone numbers you want to look up. You can enter these numbers in pretty much any format you’d like. One of the powerful uses of the Lookup API is cleaning up a list of improperly formatted numbers.
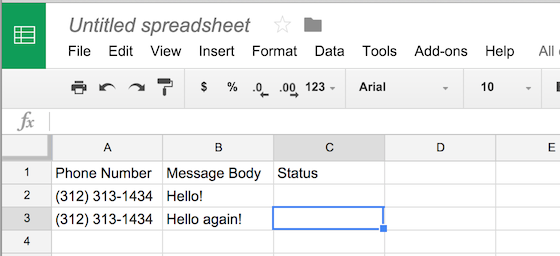
In the top menu bar, click Tools -> Script Editor to open the code editor.
The rest of this post walks you through the code to look up and validate the phone numbers. If you’re the kind of person that likes to skip to the end of the mystery novel, you can copy in this code for the final product (take note of the placeholders for ACCOUNTSID and AUTHTOKEN from your dashboard):
If you want to see how we arrived at that result, carry on.
Look Up a Phone Number With Apps Script
We’ll write this code in two steps:
- Write a lookup function to look up a single phone number.
- Write a lookupAll function to run lookup on every phone number in the spreadsheet.
Replace the two lines of code in your script with:
Replace that 3123131434 with your cellphone number.
The job of lookup is to make an HTTP POST request to the Twilio Lookup API. You don’t need to know what that means to complete this tutorial, but you can think of it as submitting a “Lookup Phone Number” form with a single field of “Phone Number”.
All the code in this step goes inside the lookup function. Define the URL for the phone number lookup API.
Create an options hash to tell Apps Script that this is a GET request:
Add headers to options to authorize the request with your Account SID and Auth Token from the dashboard:
Finally, execute your HTTP request and log the result:
Save your file and run your script. You’ll be prompted to authorize your app the first time you run it. Click View->Logs to see the data from the request.
Look Up Phone Numbers from the Spreadsheet
It’s not terribly practical to hard code phone numbers into our script. Let’s add a function to read the numbers from the spreadsheet, pass that data to lookup, then update our spreadsheet with what we discovered.
Replace myFunction with:
Immediately above myFunction (and below lookup ), create the lookupAll function. The rest of the code goes here.
Fetch the data from your spreadsheet:
Then we:
- iterate through the data
- if it’s not not blank, try to lookup the phone number
- if the phone number does not exist, update the “Lookup Status” column with a “not found”
- If the phone number does exist, update the remaining columns with the data returned from the lookup API
- if an error occurs along the way, log the error and update the status column (you can view your logs under the Tools menu in the Script Editor)
Run your app again! You’ll need to reauthorize it since it’s now trying to access your spreadsheet as well.
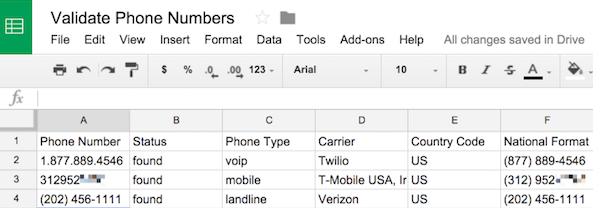
Onward
Google Sheets and Apps Script provide a surprising amount of functionality for a programming language that requires no installation or configuration. If you found this useful, you may want to check out our tutorial on how to send a text message from a Google Spreadsheet. With that, you could text the number that you just discovered is a cellphone.
If you’d like to learn more about the Lookup API and how to send text messages, check out these docs:
If you have any questions or come up with any cool use cases for looking up phone numbers, I’d love to hear about it. You can find me at gb@twilio.com or @greggyb.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.