How To Implement OTP Authentication in Symfony With WhatsApp
Time to read:
How to Implement OTP Authentication in Symfony With WhatsApp
As the demand for seamless user experiences continues to grow, implementing secure and efficient user verification mechanisms, such as sending One-Time Passwords (OTP) via SMS, voice call, email, or WhatsApp, becomes increasingly important.
In this tutorial, you will learn how to implement and send an OTP to users via WhatsApp using Twilio Programmable Messaging API for WhatsApp. The application will include registration, login, and verification pages. During the authentication process, users are directed to the verification page where they can verify their account using an OTP sent to their registered WhatsApp number.
Prerequisites
To complete this tutorial, you will need the following:
- PHP 8.3 or higher (ideally PHP 8.4)
- Composer installed globally
- The Symfony CLI
- A Twilio account. You can create a free Twilio account if you don't already have one
- Access to a MySQL database
Getting started
To create a new Symfony application and change into the new project directory, navigate to the folder where you want to scaffold the project and run the commands below:
Set up the database
Let's connect the application to the database. To do this, open the .env file in the project’s root directory. Then, comment out the default DATABASE_URL setting for PostgreSQL and uncomment the DATABASE_URL for MySQL. Update the DATABASE_URL with the actual MySQL database name, username, and password as follows, replacing the placeholders with the appropriate values for your database.
Now, run the command below to connect and create the database.
Create a Users entity
Let’s create the Users entity, which represents the users table schema and its properties, such as username, phone, email, password, etc. To do this, run the command below:
The above command will prompt you to enter the entity names and their properties. Set them as shown in the screenshot below.
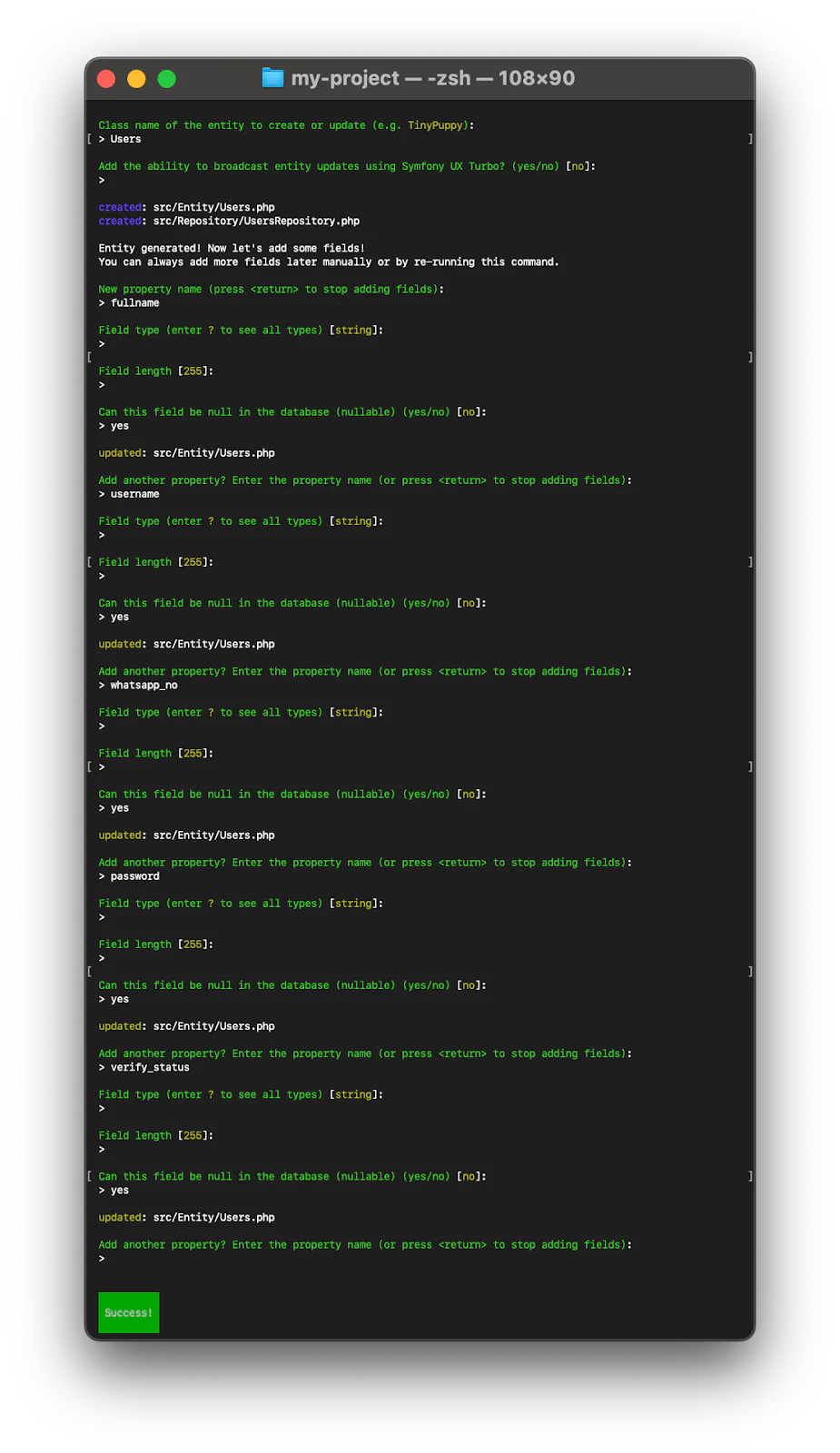
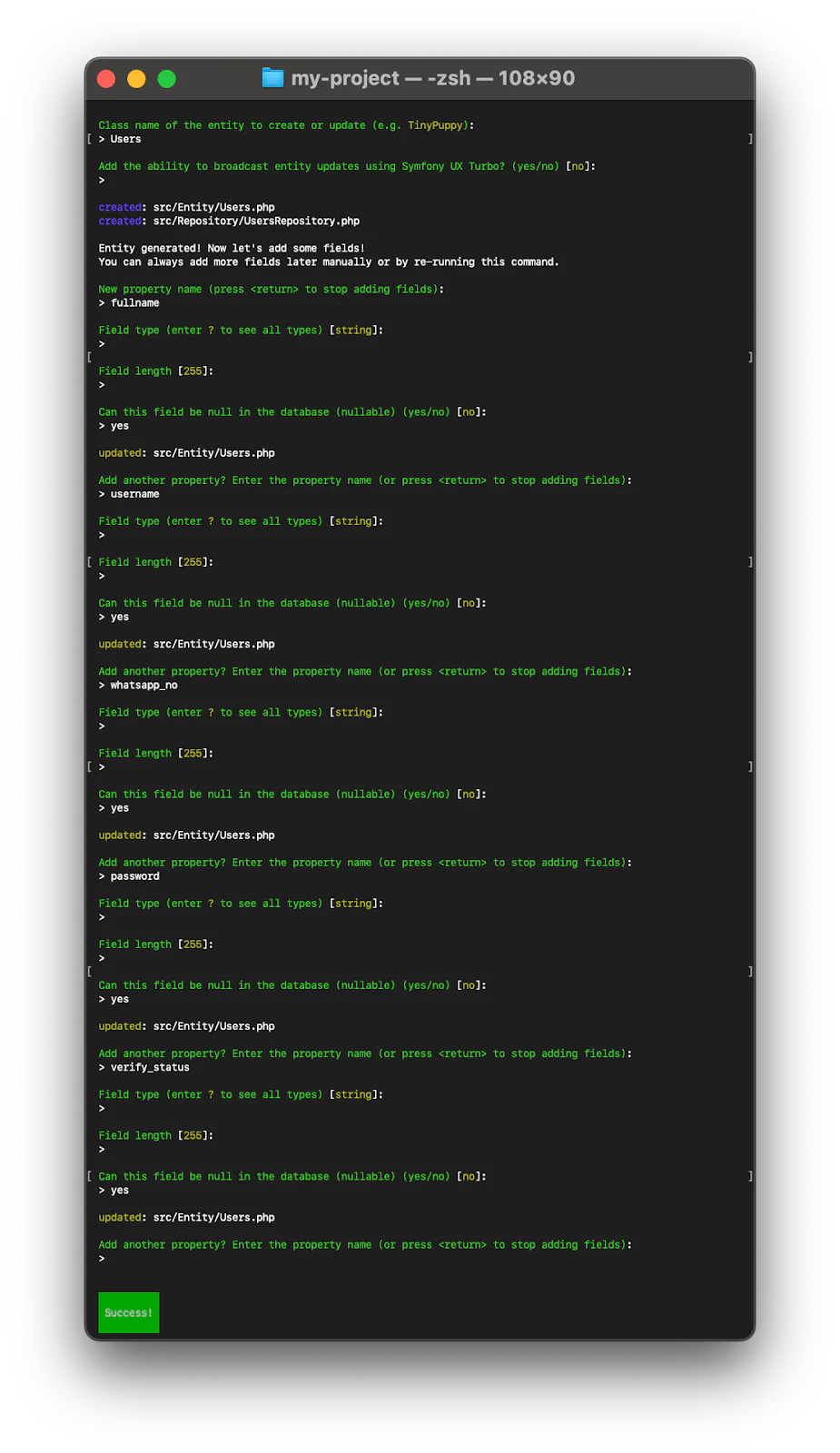
Now, you need to run the migration commands below to migrate the database with the newly created entity.
Answer "yes" when prompted while running the second command.
Create the Form Type
Firstly, Let's create a Form Type that will be used to define the input fields for the registration form. To do this, follow these steps:
- Navigate to the src folder.
- Create a Form subfolder within the src folder.
- Inside the Form subfolder, create a file named RegistrationType.php.
Now, open the RegistrationType.php file and add the following code:
Next, let's create the login Form Type with username and password as the input fields. Inside the src/Form folder, create a file named LoginType.php and add the following code to it:
Install the Twilio PHP SDK
Before you can use the Twilio WhatsApp API, you must install the Twilio's helper library for PHP into your project using the command below:
Set the necessary environment variables
Log in to your Twilio Console Dashboard to obtain your Twilio Account SID and Auth Token, as shown in the image below:
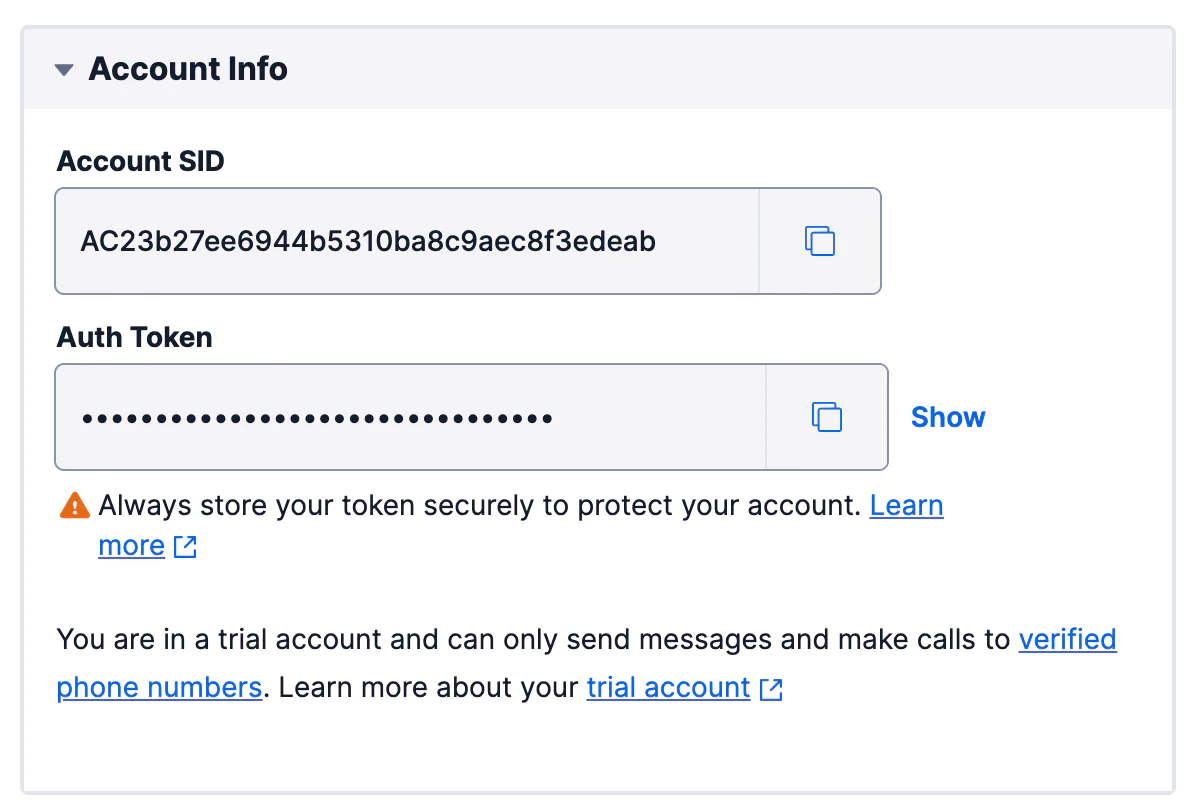
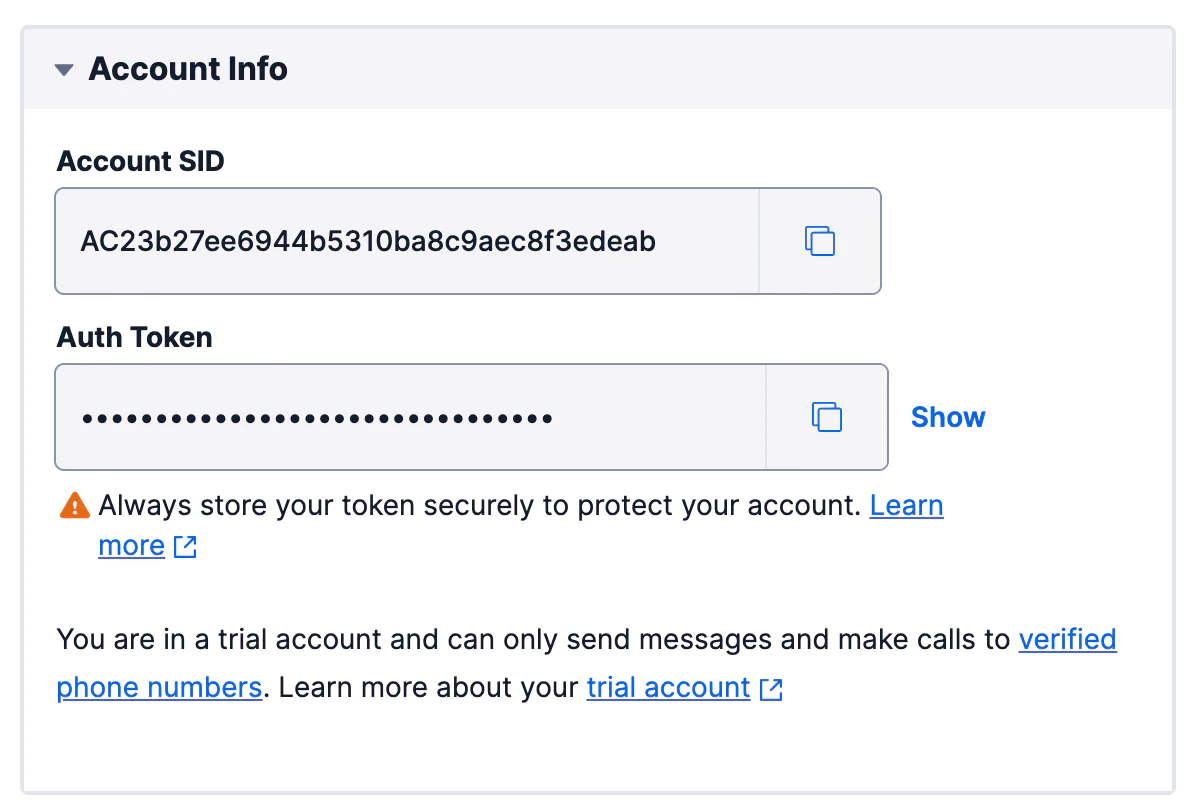
After obtaining them, open the .env file and set them as follows:
Set up your Twilio WhatsApp number
Twilio provides a WhatsApp Sandbox that allows developers to test the twilio WhatsApp API during development. From your Twilio Console dashboard menu, navigate to Explore Products > Messaging > Try it out > Send a WhatsApp message option, as shown in the screenshot below:
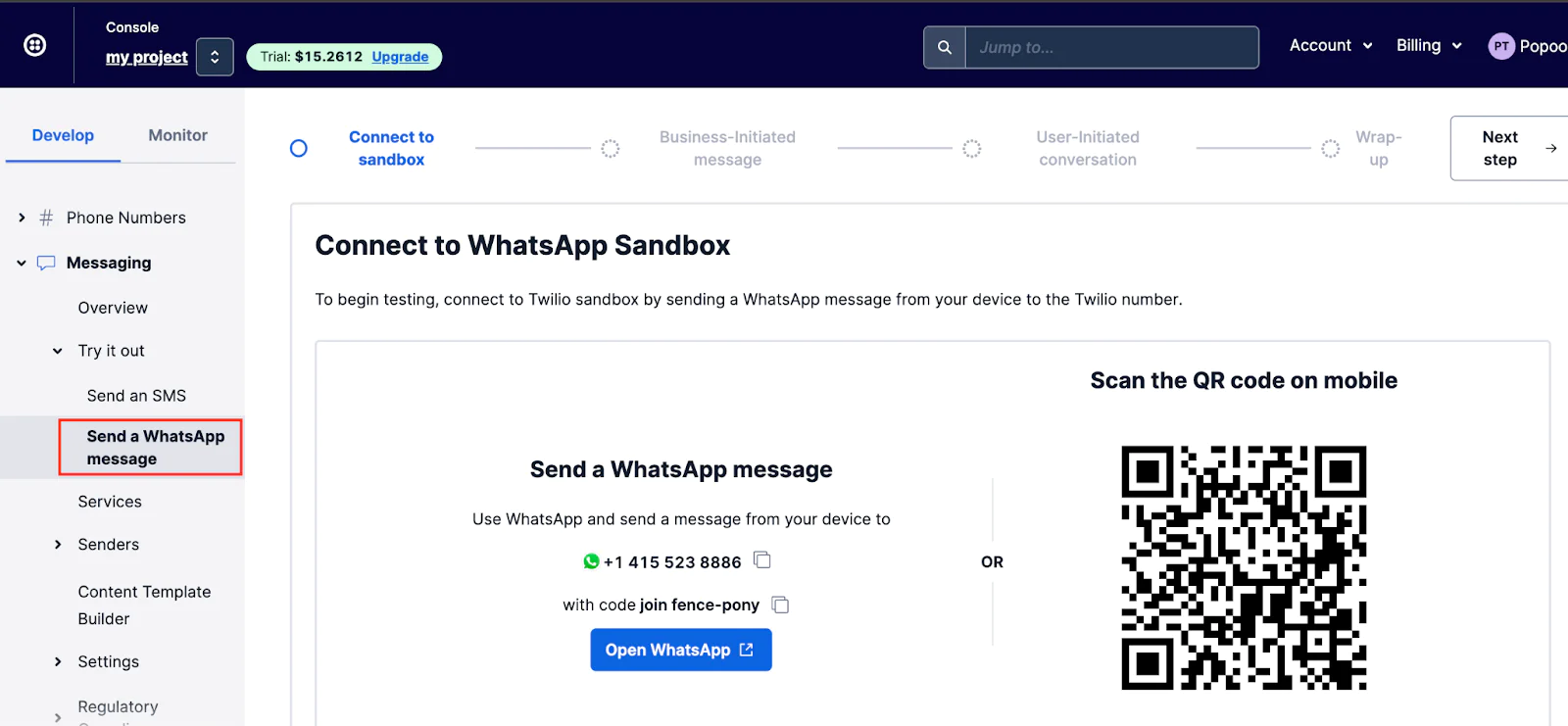
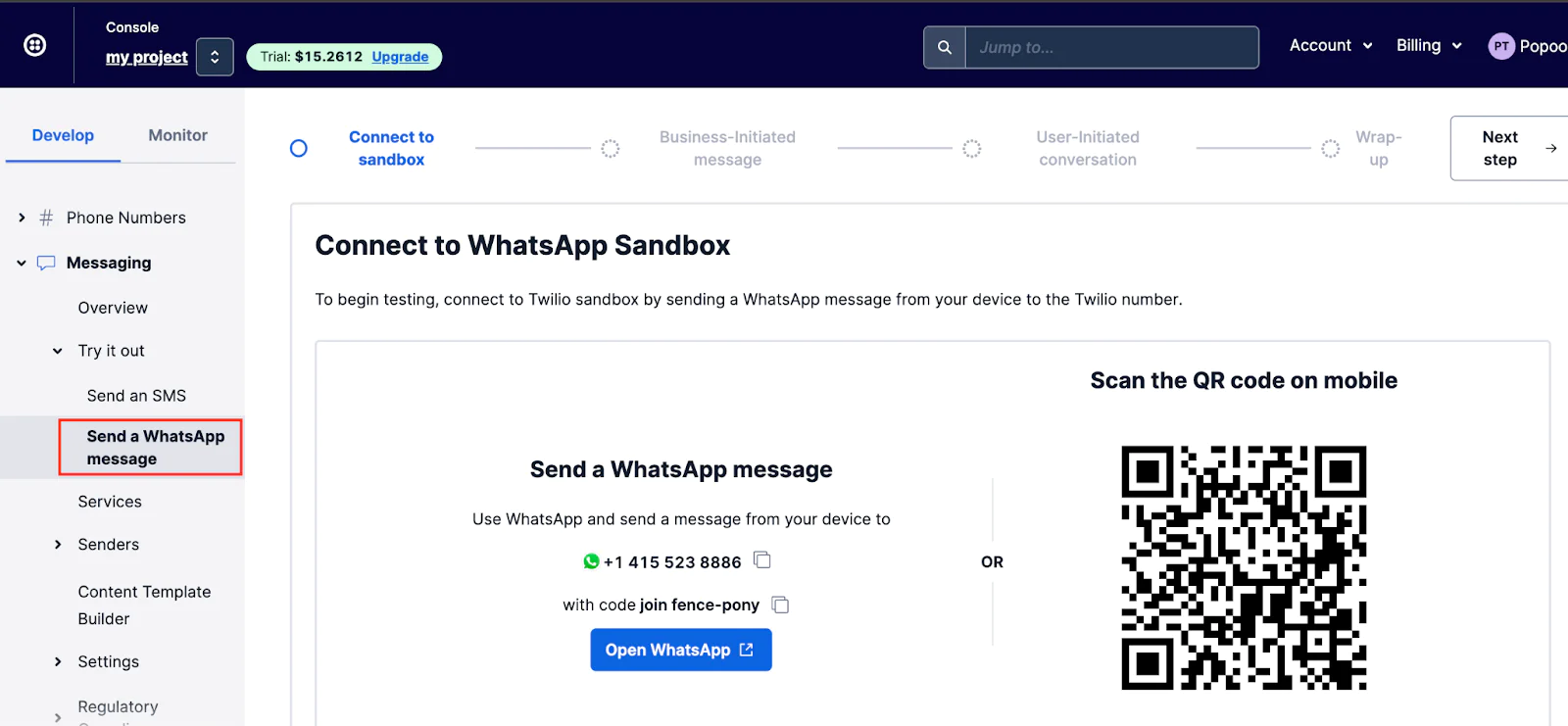
Next, you will be required to send a join message from your phone to the phone number shown in the WhatsApp Sandbox. Send the join message, as shown in the image below.
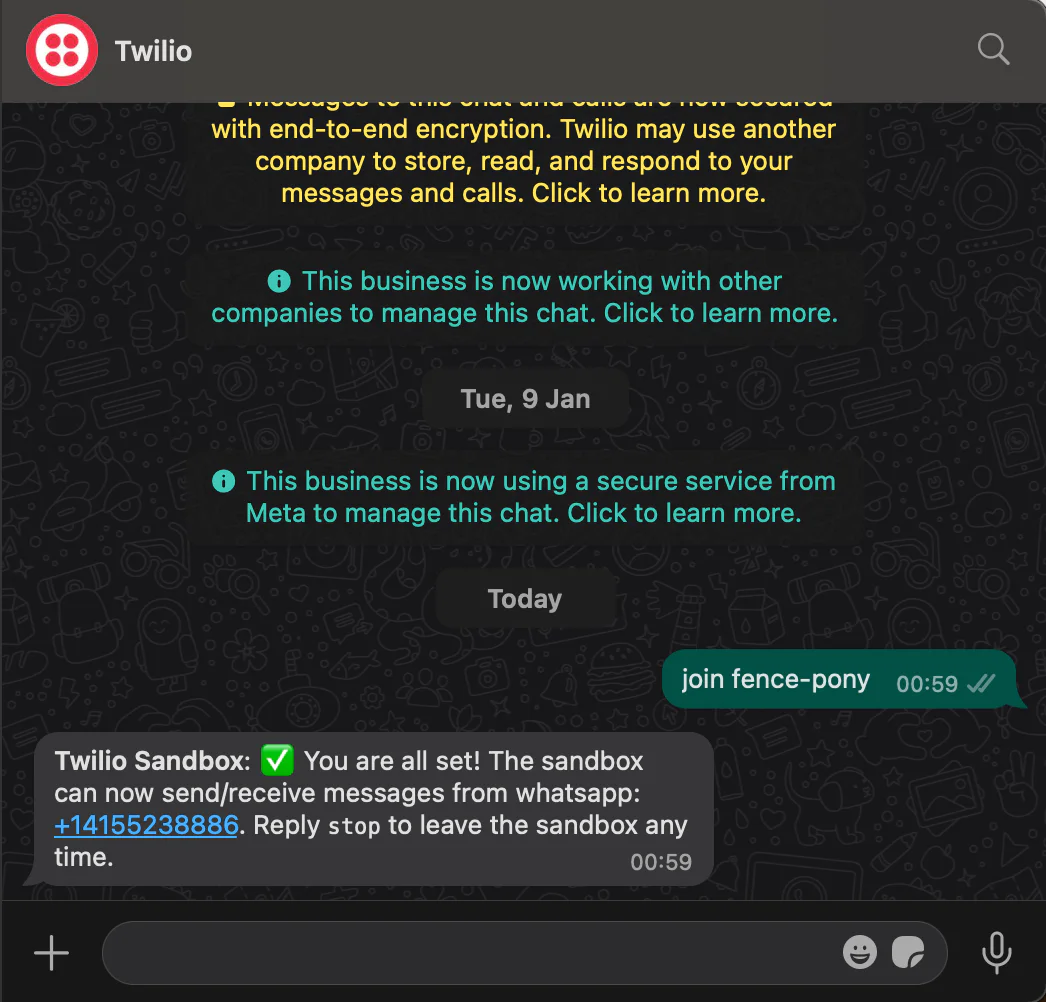
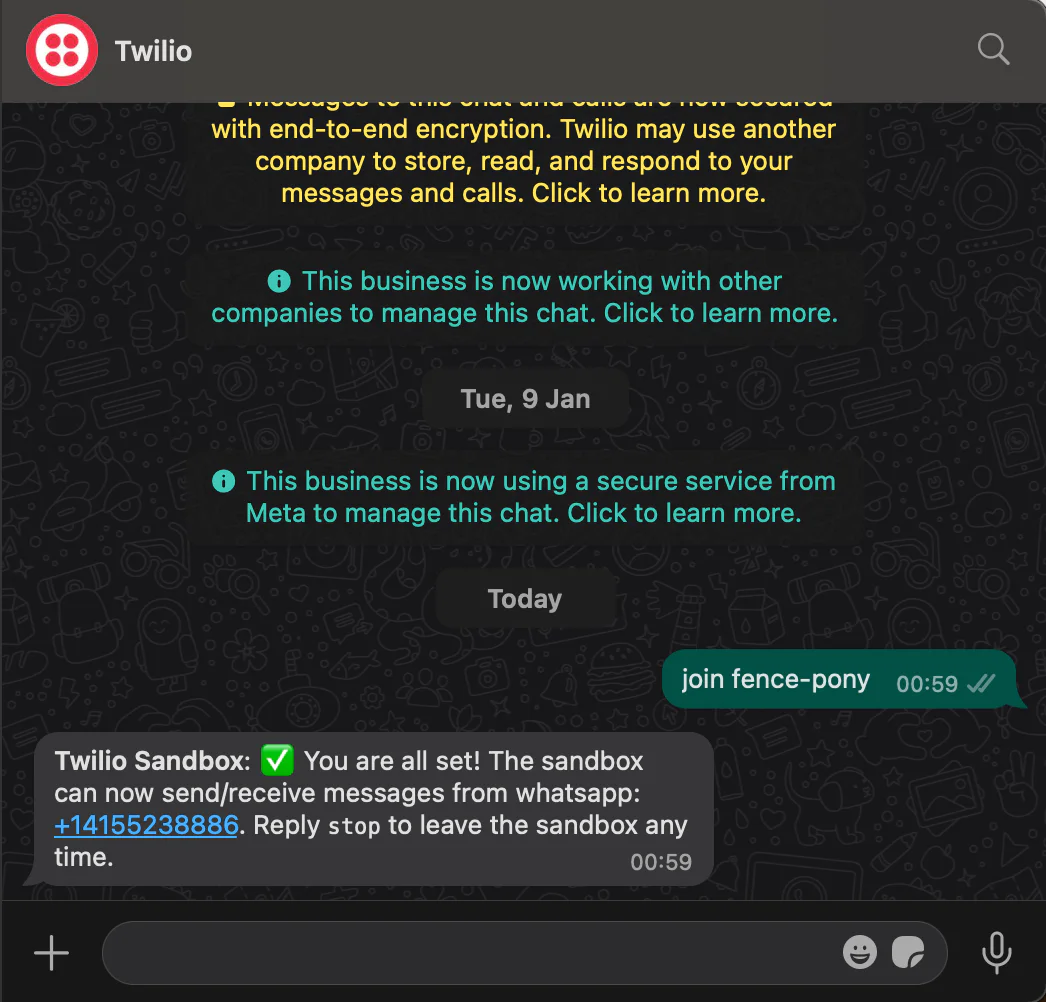
Next, add the dedicated Twilio WhatsApp number (without the "whatsapp:" prefix) to the .env file as follows:
Create the registration Controller
A Symfony controller is used to define the application's logic and can also define an application's routes. Run the command below to create a controller named Registration.
The command will create two files: src/Controller/RegistrationController.php and templates/registration/index.html.twig.
Implement a service to send WhatsApp messages
Now, let's create a service class for sending OTP messages to users via WhatsApp. To create it, navigate to the src folder and create a folder called Service. Then, within the src/Service directory, create a file named TwilioService.php and add the following code to it.
In the code above:
- The sendWhatsappOTP() function receives two arguments, $recipientWhatsappNumber and $otpCode. The $recipientWhatsappNumber variable represents the recipient's WhatsApp number, where the OTP will be sent, and The $otpCode variable holds the actual OTP generated for the user's phone number verification.
- The Client Twilio method is then used to send the WhatsApp OTP to the user's WhatsApp number.
Create the registration route
To create the registration route, navigate to the src/Controller directory, open RegistrationController.php, and replace its code with the following.
In the code above:
- All the necessary dependencies are imported
- The route for the user's registration page is defined
- In the registration route, once a user is successfully registered, an OTP is sent to the user's WhatsApp number
- Then, the user is redirected to the verify page where they can enter the OTP
Next, let’s create the registration form template. Navigate to the templates/registration directory and create a new file named register.html.twig. Inside the created file, add the following code to it:
Create the verify route
To create the verify route, where the user can enter their OTP to verify their account, add the following function to RegistrationController.php.
In the code above, the OTP code entered by the user is verified to check if it is correct or not. If the OTP code is correct, the user's verify_status is then changed from Pending to Verified in the database.
To create the verify form template, in the templates/registration directory, create a verify.html.twig file, and add the following code:
Create the login route
To create the login route, add the following function to RegistrationController.php.
In the code above,
- The user's username and password are checked to see if they are correct.
- The user's account verification status is checked to determine if the user has verified their account or not.
- If the user's account verification status is 'Pending', they will be redirected to the account verification page. Otherwise, a message will be displayed to show that the account is verified.
Next, let’s create the login form template. To do that, in the templates/registration directory, create a login.html.twig file, and add the following code:
Wrapping up
Now, let’s style the application. To do so, in the templates directory, open base.html.twig file and replace its code with the following.
Testing the application
To test the application, let’s first start the application server by running the code below:
By default, the application will run on port 8000. To open the user's registration page, navigate to http://localhost:8000/registration in your browser.
On the registration page, create a new account as shown in the image below:
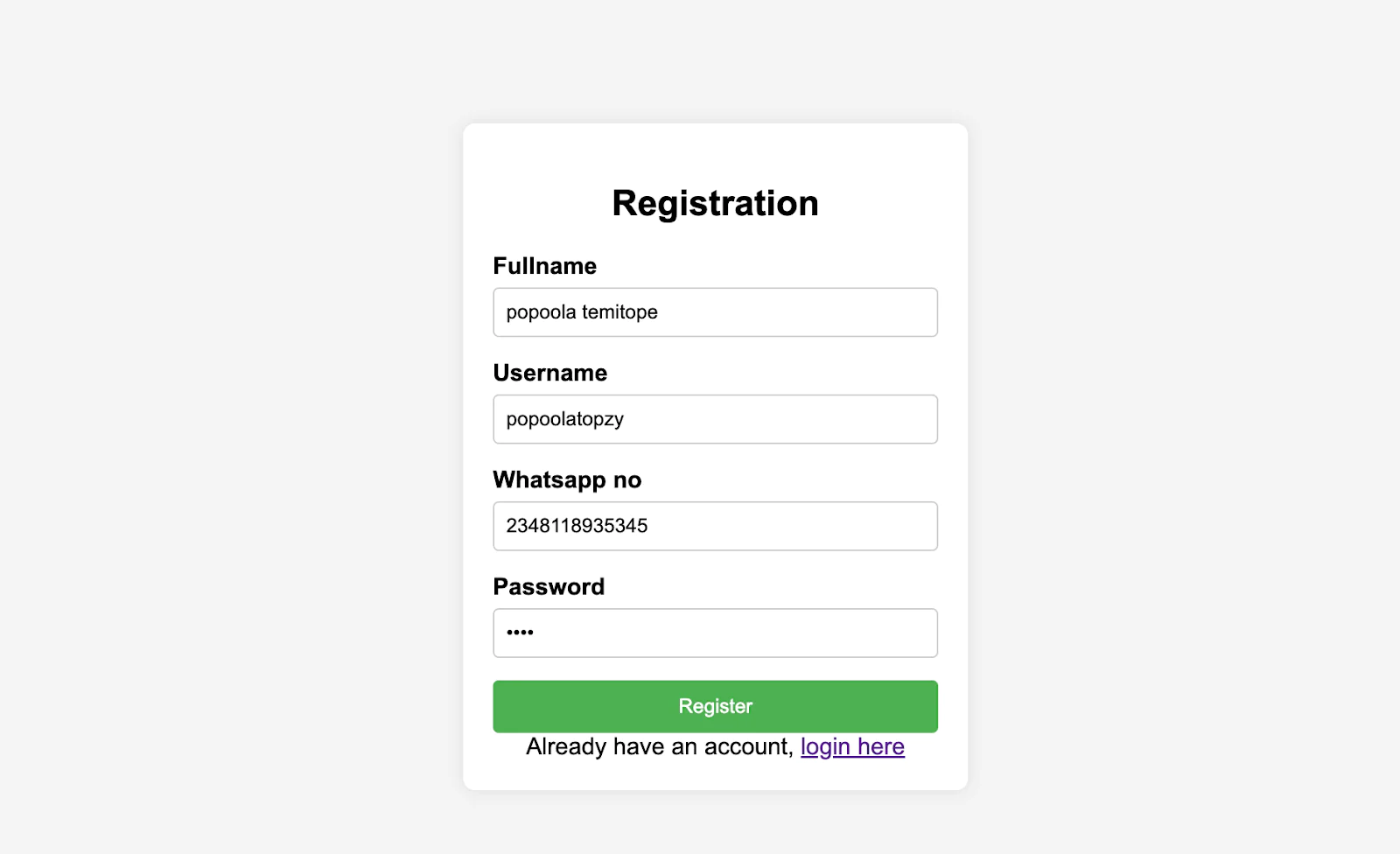
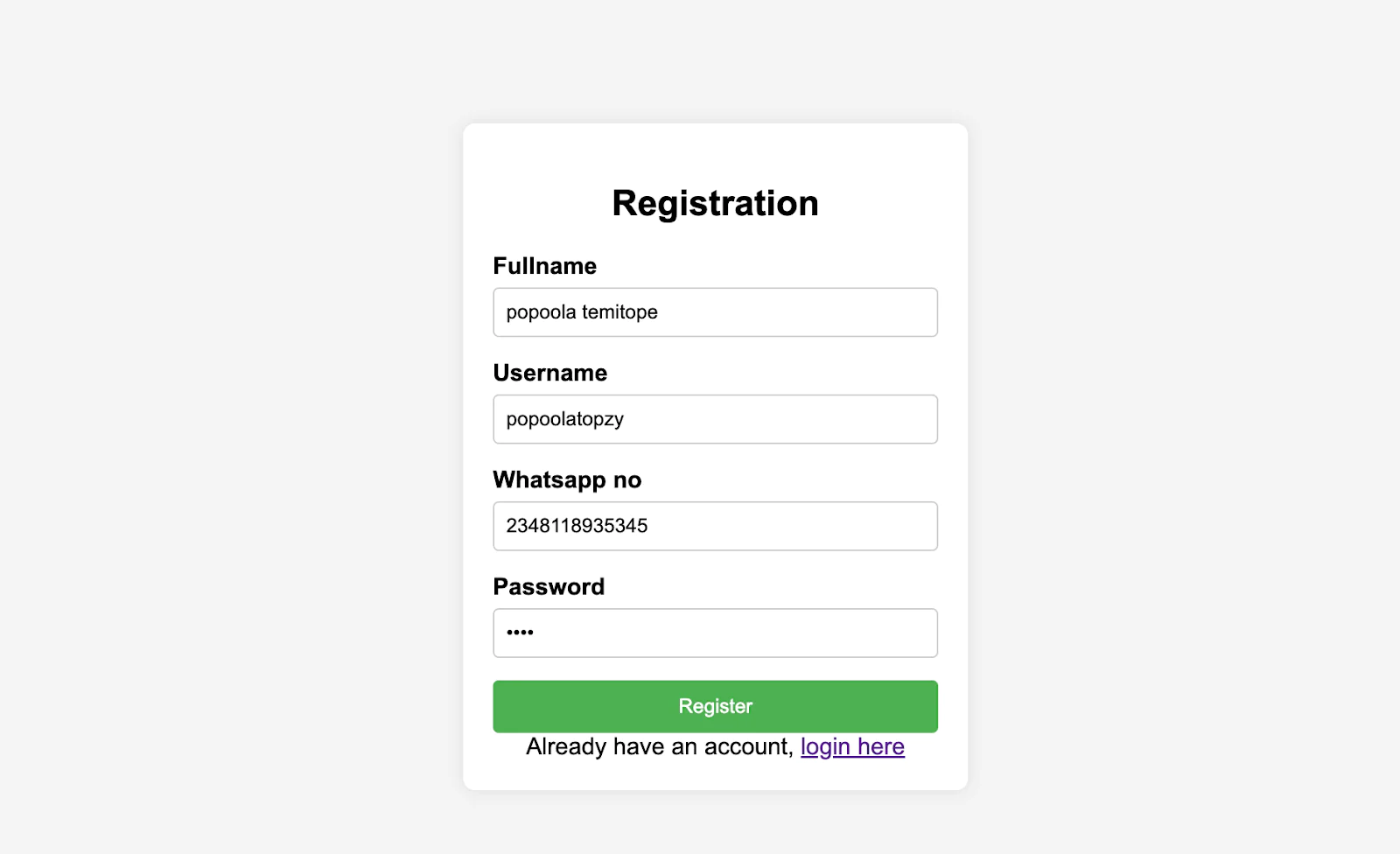
The application login page can be accessed at http://localhost:8000/login. During the login, the user will be redirected to the verify page if their account is not verified. You can login to your account as shown in the image below.
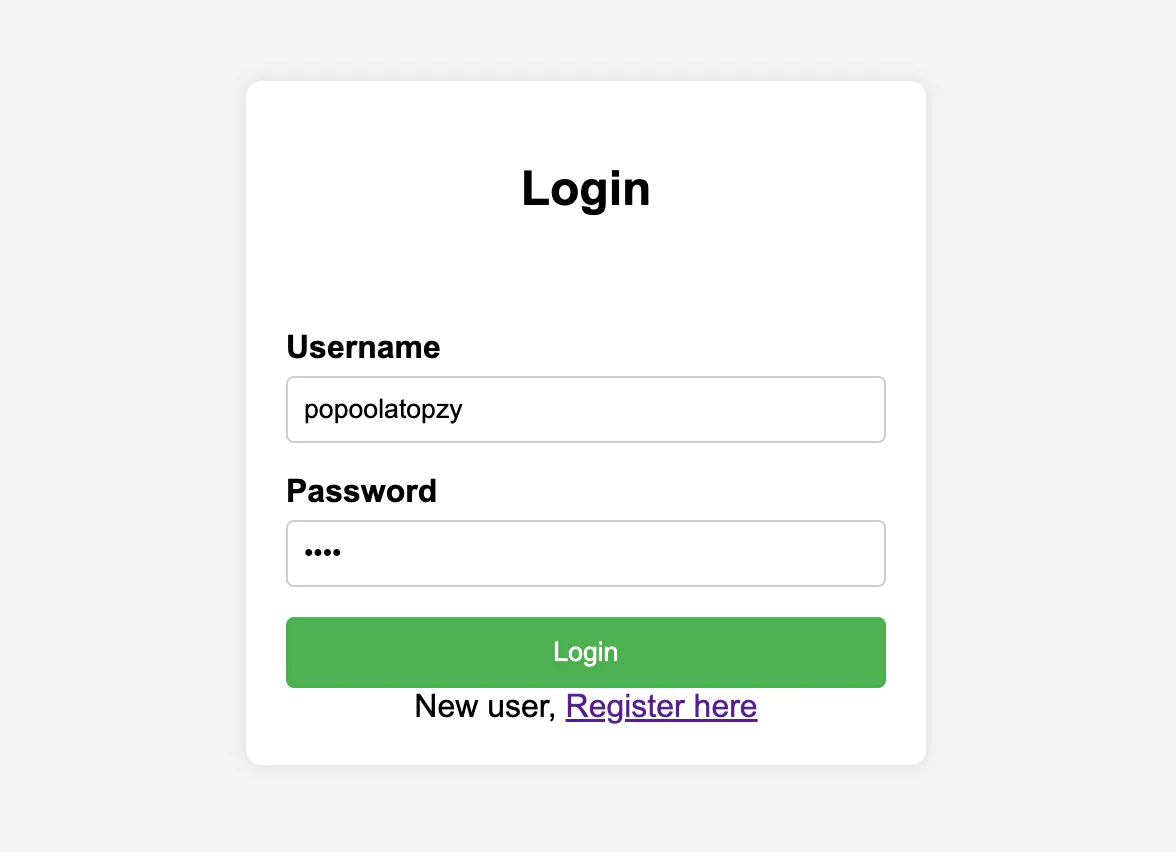
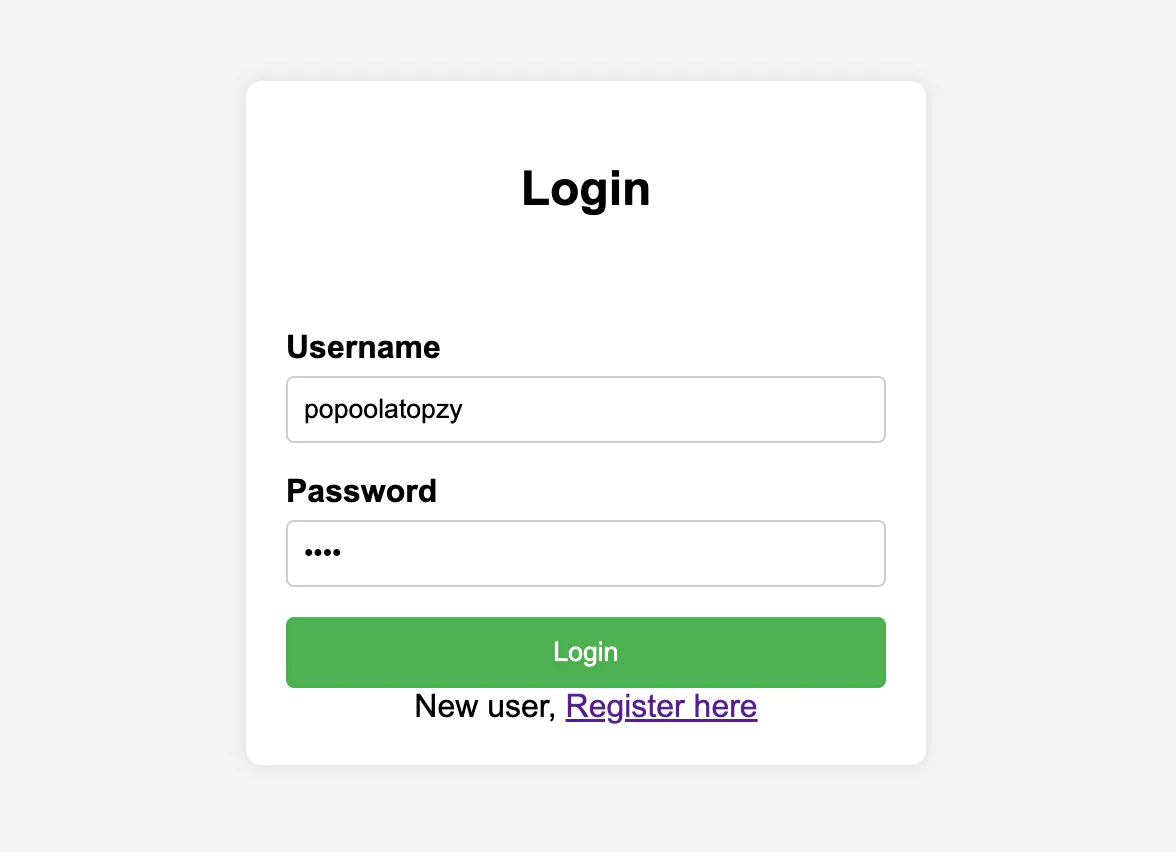
When redirected to the verify page, enter the OTP sent to your WhatsApp number, as shown in the image below:
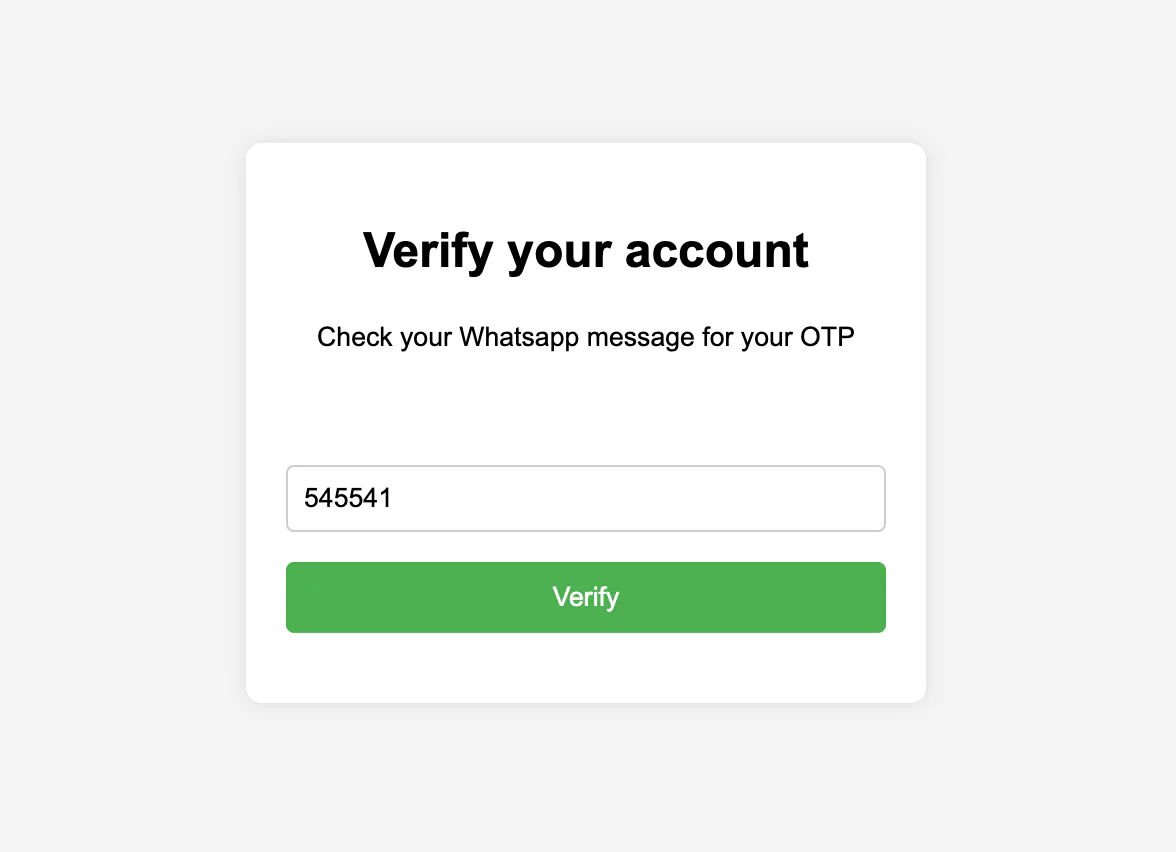
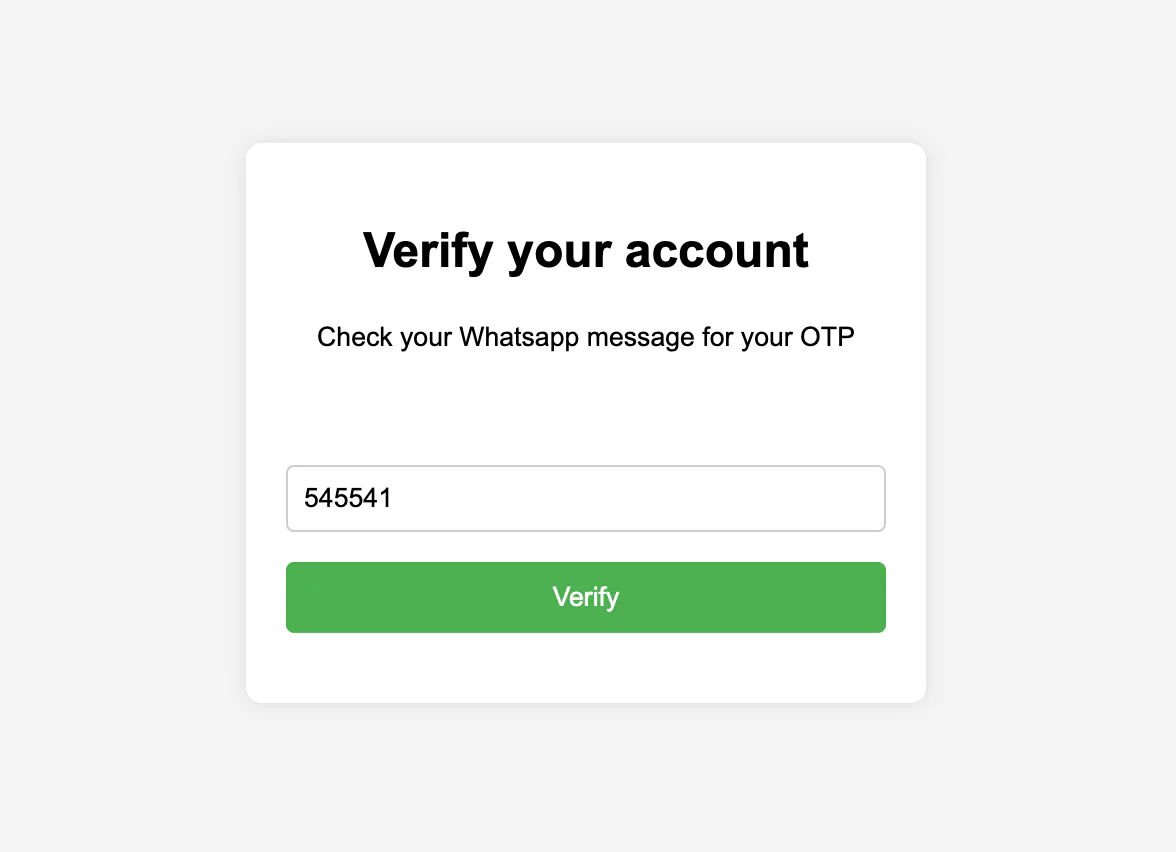
Once the entered OTP is correct, a success message will be displayed, as shown in the image below.
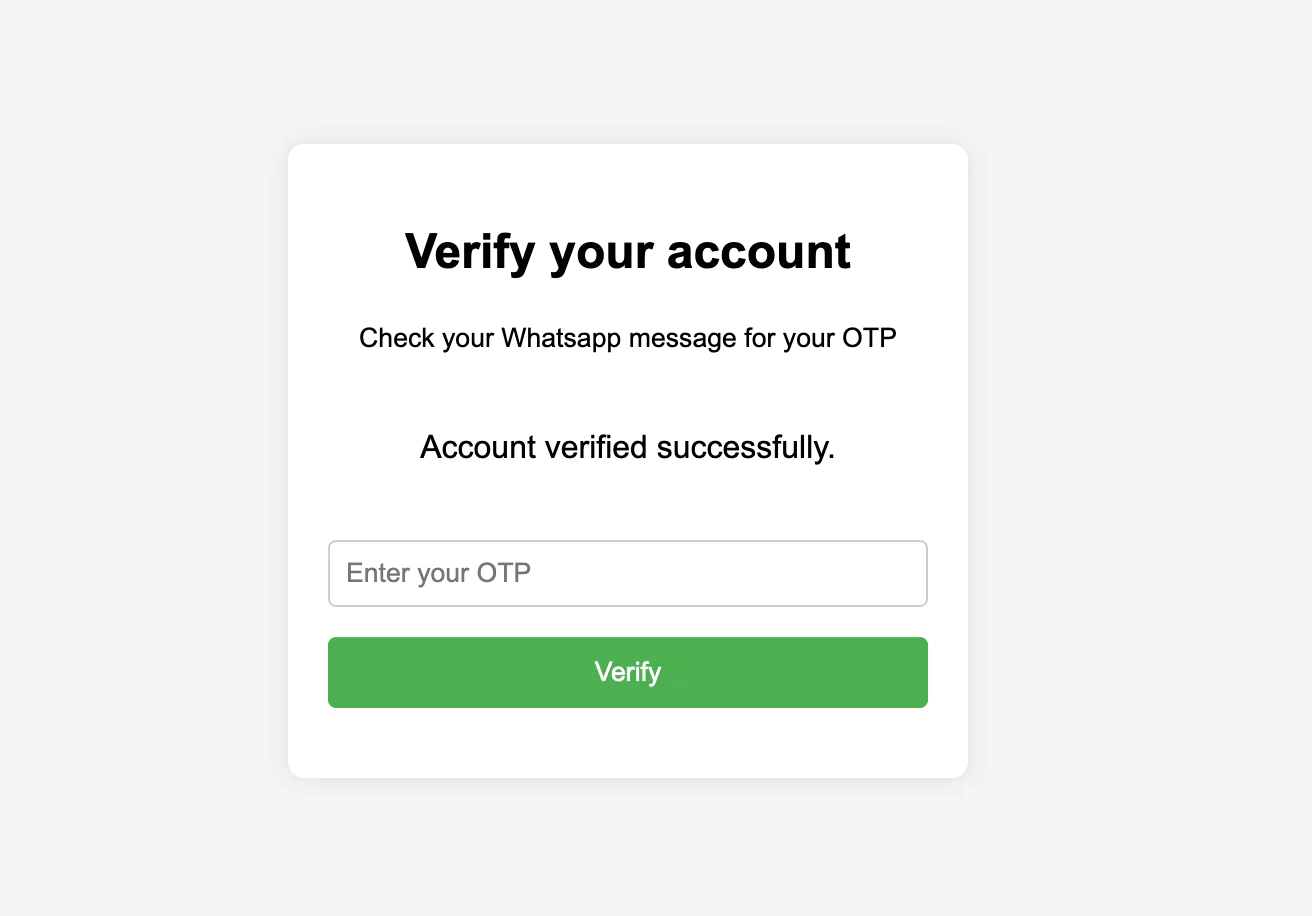
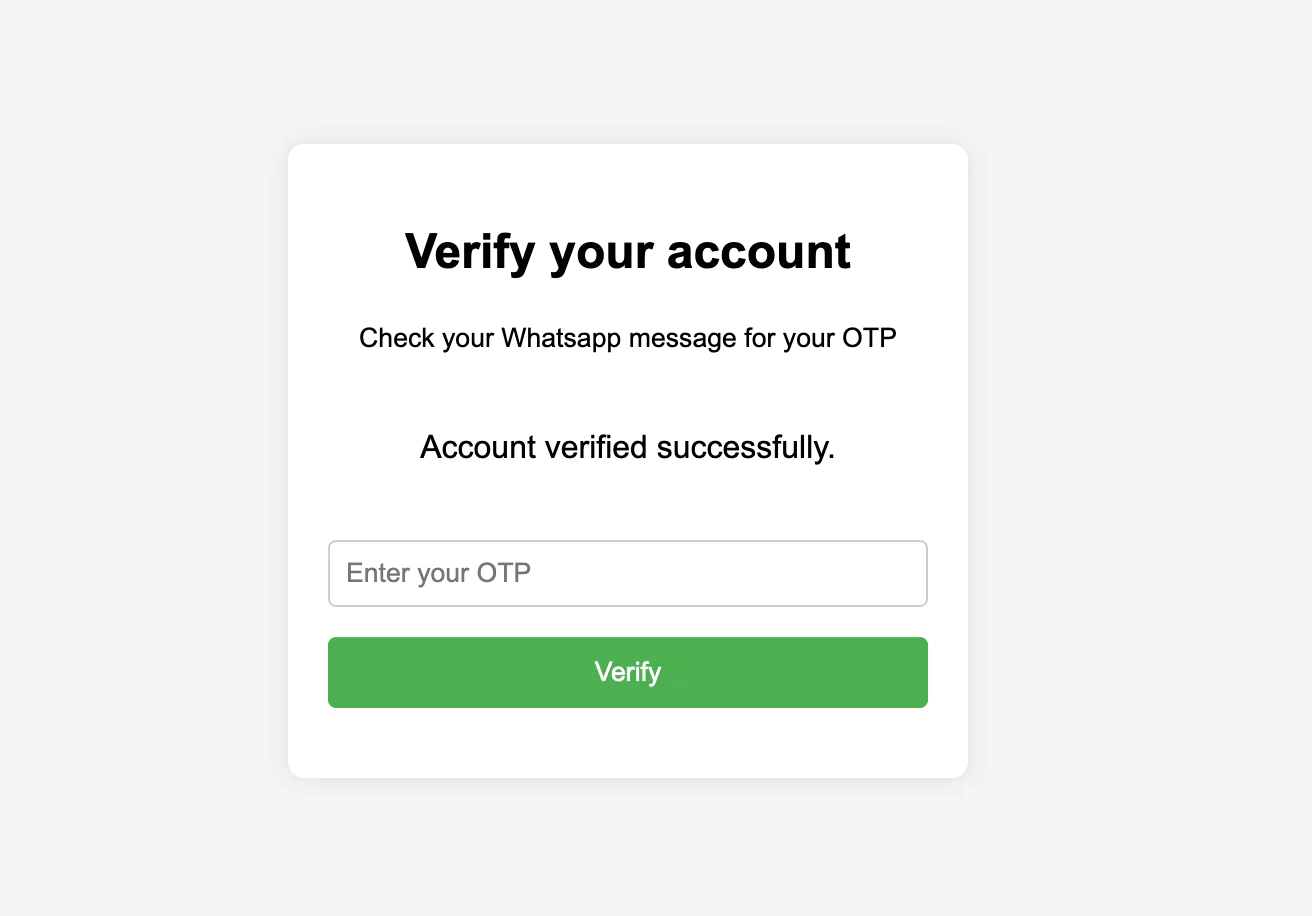
Now, you can then redirect the user to your preferred page such as dashboard or profile page.
That's how to send verification OTP through WhatsApp in Symfony app using Twilio
In this tutorial, you learned to create a secure user account verification system by sending a verification OTP to the user's WhatsApp number using Twilio WhatsApp API in a Symfony application.
To use Twilio WhatsApp API in production, you will need to upgrade your Twilio account to enjoy the full features of the Twilio WhatsApp Business API.
Popoola Temitope is a mobile developer and a technical writer who loves writing about frontend technologies. He can be reached on LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.