Twilio + PHP to Call and Text
Time to read:
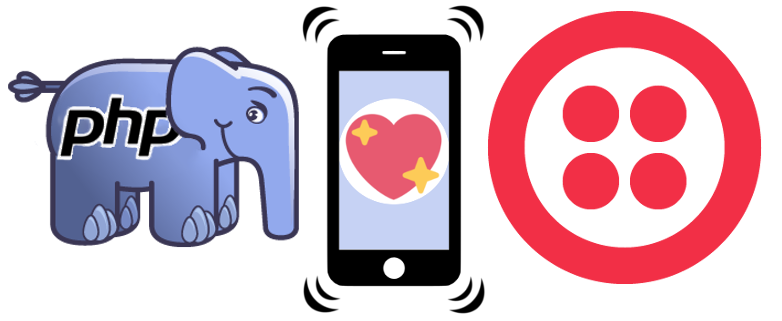
Twilio is all about communication. To help us communicate with a wider range of learning styles, on our YouTube channel you can find out how to get started with many of our APIs and services across a variety of languages, with new videos being added all the time.
Here we will step through making a phone call and sending a text message using PHP and the Twilio REST client. Each of these has a corresponding video on our YouTube channel for those who prefer to watch.
Twilio SetupIn order to make a phone call, we will need to own a Twilio number. If you don’t already have one, you can get one here.
To make our call or send our text, we will send information to Twilio using the PHP sdk and specifically the REST client. We will send both the information to authenticate our account and our instructions for the API.
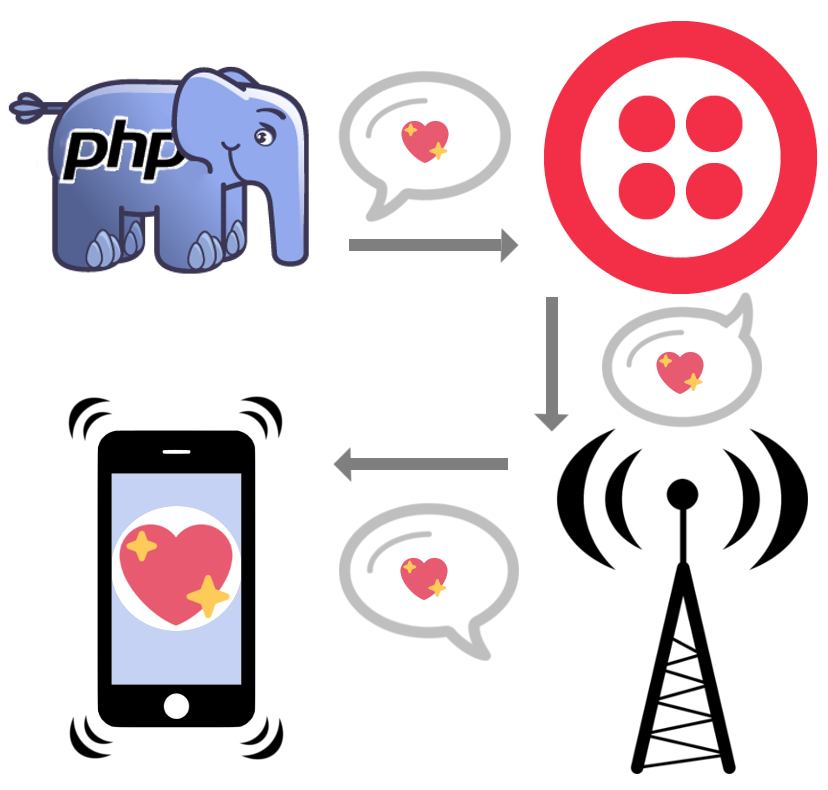
Twilio then instructs the relevant telecom systems to make our call or send our text, and somewhere a phone gives a buzz.
The REST client allows us to compose instructions using PHP and then create and transmit those instructions to the Twilio API using TwiML, a subset of XML.
Our phone call will rely on the TwiML instruction.
Making a CallCreate a new project with the Twilio sdk using composer:composer require twilio/sdk
We will need the project to be able to access our account credentials. For this example code let’s assume these are stored as environment variables and are retrievable using getenv(). If you prefer to use a .env
for environment variables, you can install vlucas/phpdotenv. Remember when storing phone numbers in environment variables that they must be prefixed with a plus sign “+” and their country code.
Create a new file and open it in an editor:pico phonecall.php
Add the following code to include the REST client, authenticate, and to send instructions to the Twilio API:
Save the new file and execute the script.php phonecall.php
What we hear when we receive the call is dictated by the TwML found in voice.xml at the URL we specified in the calls create method parameters:
The tag specifies a voice and the text which that voice will speak. The
tag instructs Twilio to play a sound file.
We could customize the content of the call by instead specifying a url where different instructions are located. It can be useful when experimenting with custom instructions to be able to have a url point to a file on your local machine without having to deploy it. Ngrok is a useful tool for that purpose.
Watch how to make a phone call using PHP and Twilio here:
Create and open a new PHP file in the same directory where we have already installed the Twilio PHP sdk.pico sendtext.php
Add the following code to include the REST client, authenticate, and to send instructions to the Twilio API:
Here we’re creating a text message instead of a phone call. Our create parameters start with the destination phone number. Next it takes an array where we give the body of the message, our Twilio phone number, and a url for an image to send too.
Save the file, run the script, and enjoy your sparkle unicorn dance party!
Watch how to send a text using PHP and Twilio here:
Congratulations on setting up outgoing calls and texts with Twilio! Now we’re are equipped to communicate more effectively with notifications, updates, reminders; and we’ve just scratched the surface of programmable communications with Twilio.
Be sure to subscribe to the Twilio Youtube channel and catch the great new content being added there. You can find me at Seattle meetups, various tech conferences, and of course here on the internet any time.
- Email: mstaples@twilio.com
- Twitter: @dead_lugosi
- Github: mstaples
I look forward to seeing what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.