Agregar capacidades de voz personalizables nunca ha sido tan fácil
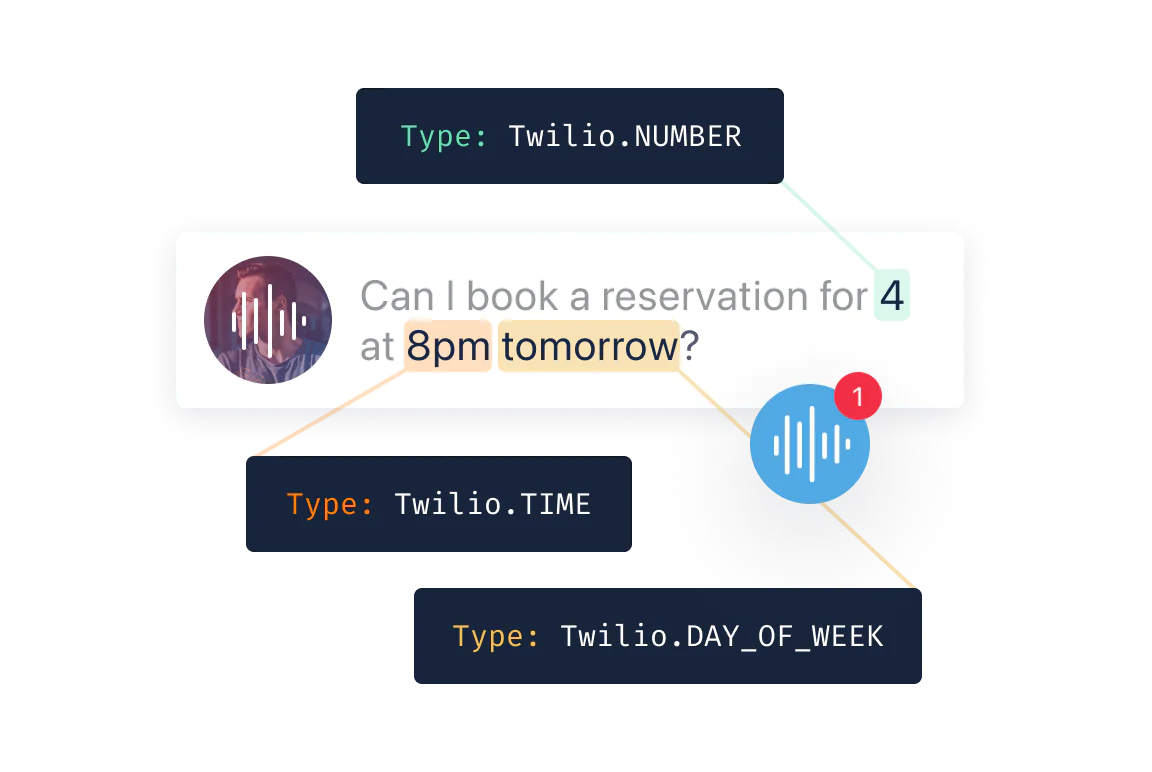
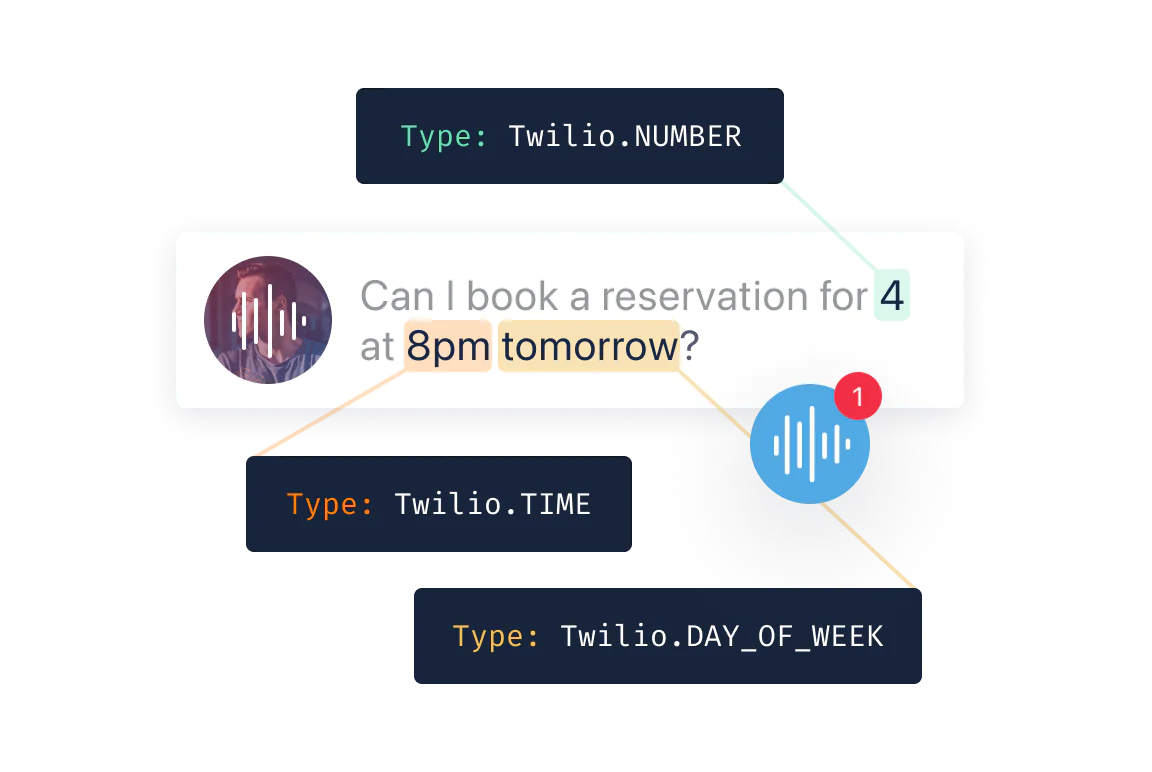
Céntrate en tus clientes, no en crear una voz desde el principio
Twilio Voice te ofrece las herramientas necesarias para crear experiencias de voz personalizadas que satisfagan y superen las expectativas de los clientes, siempre cambiantes. Gracias a nuestra infraestructura de nivel empresarial, podrás dedicar más tiempo a ofrecer experiencias memorables a los clientes y menos a crearlas desde cero.
Y con nuestras innovadoras API y SDK y recursos para desarrolladores, tendrás todo el apoyo necesario para empezar a crear una aplicación de voz memorable.