How to Send an SMS With Node.js Using Twilio
Time to read: 2 minutes
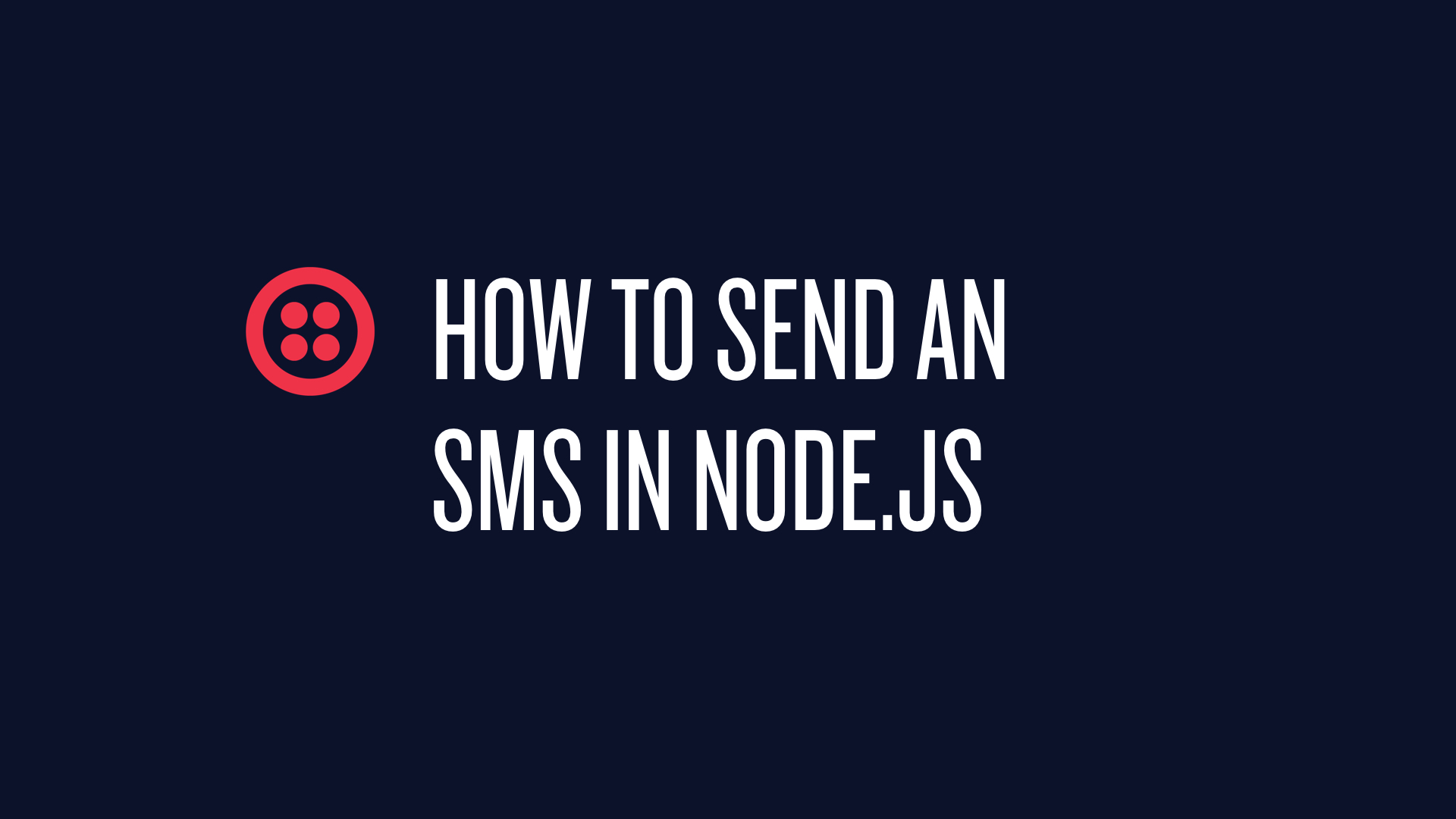
Ten lines of code (including whitespace!) is all you need to send an SMS with Node.js using Twilio:
If you’d like a short explanation about how this works, check out this short video:
Prefer a Walkthrough Instead?
The first thing we need for the above code to work is a Twilio account. Sign up for your free trial account here.
We also need an SMS-enabled phone number. You can search for and buy one in the Twilio console.
Sending an SMS using Twilio is as simple as making an HTTP POST request to the /Messages
resource in the Twilio API. Twilio makes this super simple by providing a helper library. Install the twilio-node library from the terminal using npm
:
Next, create a file named sms.js
and open it in your favorite text editor. At the top of the file require
the twilio-node helper library and use it to create a Twilio REST client. You can find the credentials you need for this step in the Twilio Console. Store these credentials in system environment variables for safe keeping. If you’re unsure how to set environment variables click here for Windows or here for Mac OS X.
Use the client
to send an SMS message from your Twilio number to your cell phone. Make sure to replace the phone number placeholders with your Twilio and cell phone numbers:
Head back to the terminal and run the script:
In just a few seconds you should receive your text message!
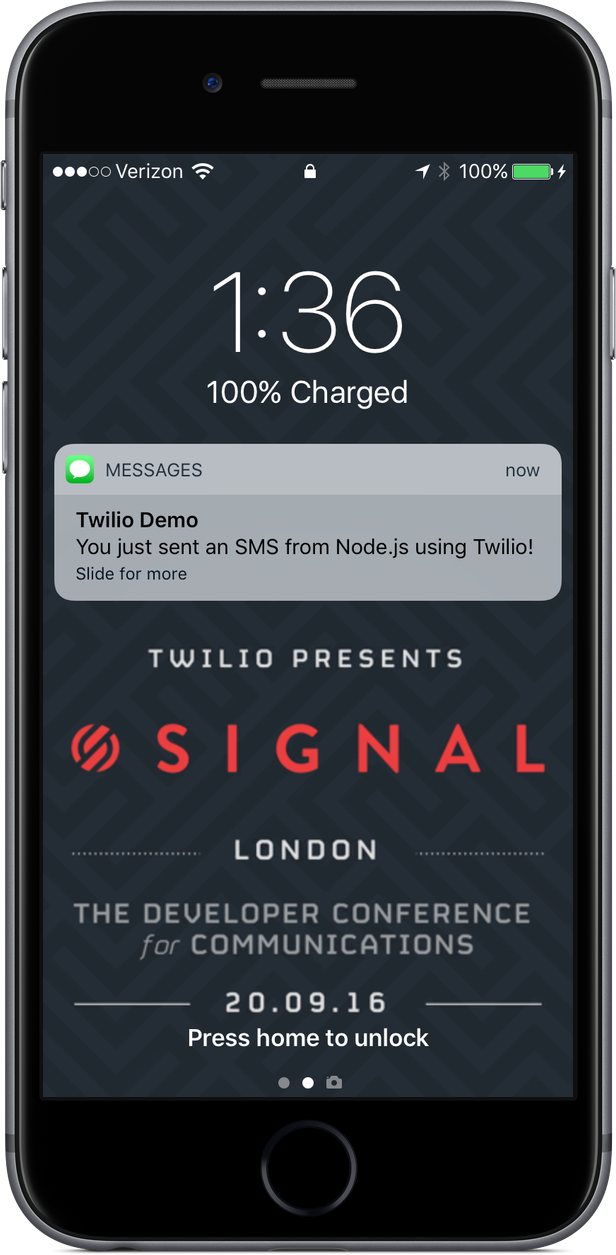
Conclusion
If you’d like to learn more about using Twilio and Node.js together check out:
- The Twilio Node.js Helper Library
- Node.js ETA Alerts Tutorial
- Marketing Notifications Using SMS in Node.js
What are you building? I’d love to hear about it. You can reach me on Twitter @brentschooley or drop me an email at brent@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.