3 Things You May Not Know About Twilio Programmable SMS
Time to read: 2 minutes
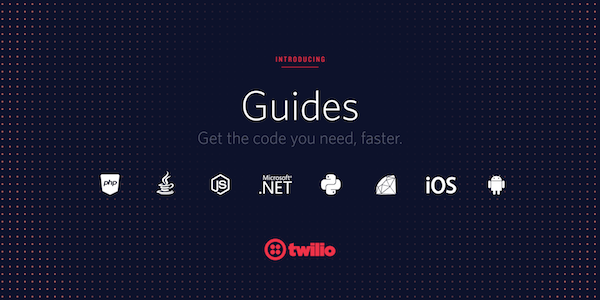
Twilio Quickstarts make it easy to get started with Programmable SMS. But where do you go to learn more after you send and receive your first text messages?
Twilio Guides are the documentation you need after “Getting Started.” Guides answer common questions and come replete with code snippets in all the most popular languages so that you can build your Twilio app faster.
Here are three lessons you’ll find in the Programmable SMS guide. We’ll show off the PHP examples here, but the guides offer code snippets in C#, Java, Python, Ruby, and Javascript as well.
Use cookies to track SMS conversations
SMS interactions typically span more than one text. Twilio offers an easy way to track SMS conversations without spinning up a datastore.
When someone texts your Twilio phone number, Twilio makes an HTTP request to your app and expects an HTTP response in return. Much like the HTTP request-response cycle that happens with browsers, you can use cookies to temporarily store data about a session — only in this case, a “session” represents a conversation.
Here’s what conversation tracking looks like in PHP:
Any time there are more than two messages between your app and the user, you’ll probably want to track state. A couple real world examples:
- An SMS based trivia game uses cookies to store a player’s score and the correct answer to their previous question.
- mRelief, a Y Combinator backed non-profit, uses cookies to track what step its users are on when taking an SMS survey.
Check out the conversation tracking guide for more details.
Use Delivery Status to Know If Your Message Made It
There are a number of reasons why a text message may not arrive at its destination. For example:
- The phone was turned off
- The phone was out of service range
- The recipient asked not to receive messages from your number
- The number was a landline
These edge cases aren’t always apparent when developing your app and sending texts to the phone on your desk. But as your app scales, it’s important to confirm that messages hit their target so that you can resend the SMS or move the conversation to a different medium (e.g., email) as appropriate.
To track delivery status, simply add a statusCallback parameter to the code used to fire off the SMS:
Twilio will send updates to the statusCallback webhook with statues of queued, failed, sent, delivered, and undelivered as the message passes through the delivery cycle.
You can also use delivery receipts when replying to an SMS with the <Message> tag. And if you’d like to help us in our quest to continually improve our service, you can send us feedback via the Twilio Message Delivery Feedback API.
Retrieve and Modify Message History
You’ll often want to revisit the details of messages you’ve sent and received. The Programmable SMS guide tells you how to retrieve and modify message history.
For example, here’s how you list your SMS logs using the PHP helper library:
For privacy’s sake, you may want to erase the contents of a message stored on Twilio servers:
Alternatively, you can remove all traces of the SMS from Twilio’s logs simply by changing that last line:
What else can you do?
We’ve covered only half of the topics in the Programmable SMS guides. Whether you’re a long-time Twilio user or just getting started, the guides will level-up your Twilio game so that you can build features faster.
If you have any questions, or if there are any other topics you’d like to covered in guides, please let us know in the comments or drop me a line at gb@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.