Automating Call Center Management with Go and Twilio TaskRouter
Time to read: 9 minutes
Automating Call Center Management with Go and Twilio TaskRouter
Effective call center management is critical for ensuring smooth customer service operations. Whether you’re running a customer support line or managing sales inquiries, directing calls to the right agent quickly and efficiently is essential for keeping your customers satisfied and your business running smoothly. With Twilio's TaskRouter, you can automate call routing based on predefined rules, ensuring each call is directed to the most suitable agent.
By integrating TaskRouter with Go, we’ll build a simple yet powerful system for managing customer service calls. From configuring a workspace and creating tasks, to handling missed calls and enabling agents to update their availability, this tutorial will walk you through the entire process.
What you'll learn
- How to set up a TaskRouter workspace programmatically
- How to handle incoming calls and route them to agents using TaskRouter workflows
- How to handle missed calls by redirecting them to voicemail
- How to enable agents to update their availability status using SMS commands
By the end of this tutorial, you’ll have a fully functional Go-based backend that integrates seamlessly with Twilio’s TaskRouter API, making call management easier than ever.
Prerequisites
To follow along, you’ll need:
- A basic understanding of and experience with Go (version 1.18 or newer)
- A Twilio account (free or paid). Sign up for a free trial account, if you don’t have one already
- A Twilio phone number capable of sending SMS
- A phone that can send and receive SMS
- Ngrok or a similar tool to expose your local development server to the internet
Set up the project
To begin, create a new project directory, wherever you create your Go projects, and change into it by running the following commands:
Initialize a new Go module
Within the go-twilio-taskrouter folder, create a new Go module by running the following command.
This will create a go.mod file in the root of your project which defines the module path and lists all dependencies required by your module.
Install the project's dependencies
This project will require two packages:
- Twilio's Go Helper Library: This significantly simplifies interaction with the Twilio API
- GoDotEnv: This is a popular package for managing environment variables
Install them using the following command:
Set the required environment variables
Next, create a .env file in the project directory to securely store your Twilio credentials and host URL. Then, add the following keys to the file:
Then, run the following command from another terminal to expose your app to the internet; the app is expected to run on port 8080:
The command will generate a Forwarding URL like "https://abcd1234.ngrok.io". Copy this URL and replace <NGROK_URL>
in your . env file with the forwarding URL.
For your Twilio credentials, replace the placeholder values in .env with the details retrieved from your Twilio Console, by following these steps:
- Log in to the Twilio Console
- Copy the Account SID and Auth Token from the Account Info panel
- Replace
<YOUR_TWILIO_ACCOUNT_SID>
and<YOUR_TWILIO_AUTH_TOKEN>
with the corresponding values
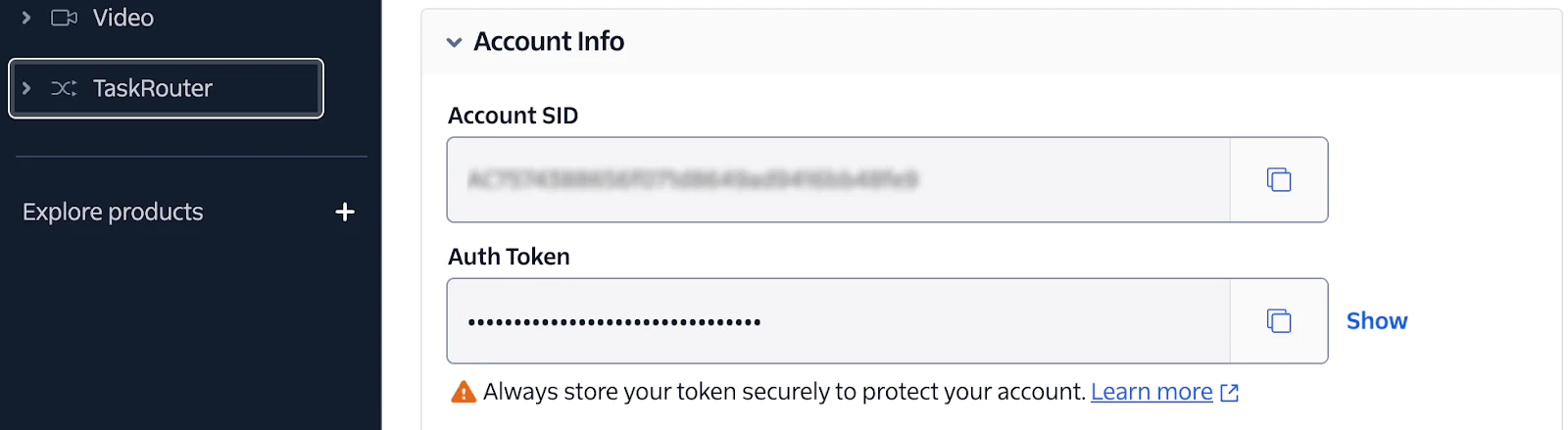
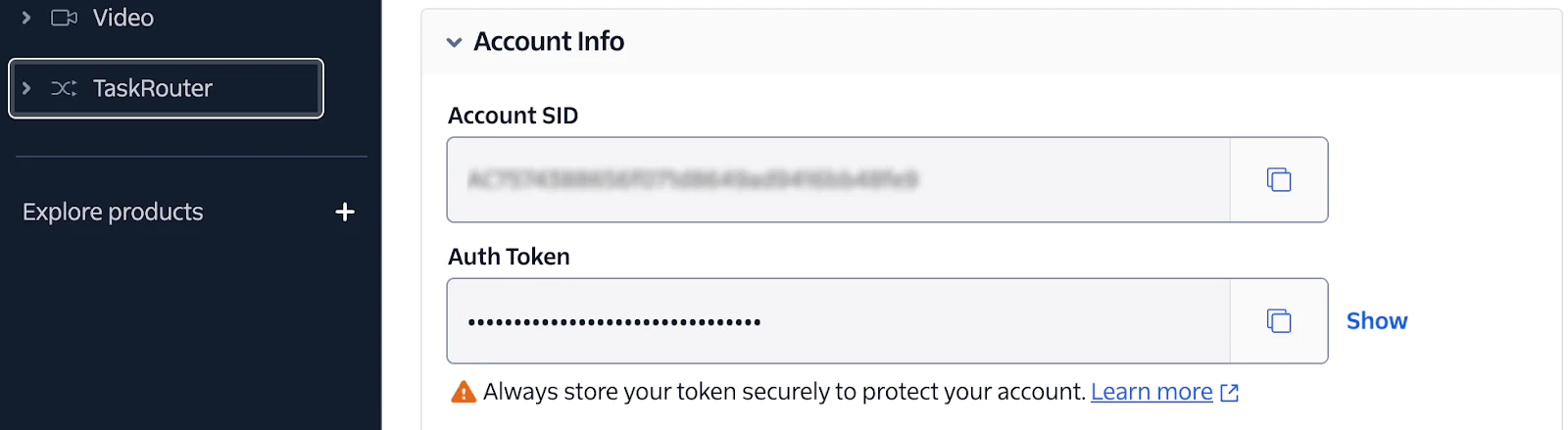
Load the environment variables
Next, to load the environment variables defined in the . env file and make them accessible throughout the application, you will create a helper function. To do this, create a folder named config, and a file called config.go within it. Following that, paste the following code into the new file:
This file handles loading environment variables, ensuring sensitive information like Twilio credentials is securely managed. In addition, the program will log an error and terminate if the file is not found.
Initialize the Twilio Client
To access Twilio services, initialise the Twilio RestClient
with your Twilio credentials. To do this, create a folder named setup and a file called client.go within it, then paste the following content into the file:
This helper function sets up the Twilio RestClient
, allowing the application to interact with the TaskRouter API.
Configure the TaskRouter
We’ll continue by setting up a TaskRouter workspace, defining activities, and creating workers. These components form the backbone of your call routing system.
To handle the TaskRouter configuration, create a new file named workspace_setup.go in the setup folder and paste the following content in it:
The ConfigureWorkspace()
function is responsible for the setup of a Twilio TaskRouter workspace. At a high level, it does the following:
- Initializes the Workspace: Ensures a workspace exists by either creating a new one or retrieving an existing one using the
findOrCreateWorkspace()
helper. - Registers Workers: Defines a list of workers, each associated with a specific product and contact number. It then registers these workers via the
findOrCreateWorker()
helper. - Configures Task Queues: It sets up task queues for routing tasks based on products (e.g., SMS or Voice). The
findOrCreateTaskQueues()
helper ensures these queues are properly configured. - Defines a Workflow: Creates or retrieves a workflow that binds tasks to queues based on routing logic. The
findOrCreateWorkflow()
helper encapsulates this logic.
Add helper functions for workspace configuration
As mentioned in the preceding section, the ConfigureWorkspace()
function relies on several helper functions to handle the detailed logic of creating or retrieving resources like the workspace, workers, task queues, and workflows.
Paste the following content at the end of the workspace_setup.go file, just after the ConfigureWorkspace()
, to define those functions.
Handle incoming calls
Next, create an HTTP handler to process incoming calls, using Twilio’s TwiML to guide callers through selecting a service. To do this, create a handlers folder and a file named call.go within it. Paste the following code into the file:
The code defines the VoiceResponse
struct which defines the TwiML response structure for Twilio’s Voice API, supporting fields like <Gather>
, <Say>
, <Pause>
and <Hangup>
. Next, it defines two functions, IncomingCall()
and EnqueueCall()
, which handle incoming calls and route them based on user input.
The IncomingCall()
function generates an XML response for Twilio's Voice API, prompting callers to press "1" for Programmable SMS, or "2" for Programmable Voice. It uses the <Gather>
tag to collect a single digit and forwards the input to the "/enqueue" endpoint.
The EnqueueCall()
function handles the input by determining the selected product. For invalid selections, it responds with an error message and hangs up. For valid choices, it checks for available agents with the required skill using the FindAvailableWorkerBySkill()
helper function.
If no agents are available, the caller is redirected to voicemail. Otherwise, the caller is connected to the agent with a brief message, pause, and the agent's name before ending the call. This setup leverages Twilio's TaskRouter and helper functions to dynamically retrieve workspace and worker details.
Add helper functions for handling calls
The EnqueueCall()
function used a couple of helper functions to retrieve workspace details, find a worker skilled in the selected product, and retrieve an agent’s name. To define these helper functions, create a new file named worker_utils.go within the setup folder and paste the following code in it.
The code above defines the following helper functions:
GetWorkspaceSID()
: This retrieves the SID of a workspace based on its friendly name. If the workspace is not found, it returns an error.GetWorkerSID()
: This function retrieves theSID of a worker based on their phone number. It parses the worker's attributes to find the matching contact URI. If no worker is found, it returns an error.UpdateWorkerActivity()
: This function updates the activity status of a worker (e.g., "Available" or "Offline") based on the specified activity name. It first retrieves the activitySID corresponding to the name and then updates the worker's activity.FindAvailableWorkerBySkill()
: This function finds an available worker based on a required skill (e.g., product type). It checks the worker's attributes and activity status, returning theSID of the first match found.GetWorkerName()
: This function fetches and returns the friendly name of a worker using theirSID. If the worker details cannot be fetched, it defaults to "Unknown".
These helper functions provide the core utilities for interacting with Twilio's TaskRouter API, enabling workspace and worker management while maintaining code modularity and clarity.
Handle missed calls
To redirect unanswered calls to voicemail when agents for a specific product are offline or unavailable, create a file named voicemail.go in the handlers folder and add the following code in it:
The RedirectToVoicemail()
function prompts callers to leave a brief message, with a limit of 10 seconds or the option to finish by pressing "#". After recording, it transitions to the VoicemailComplete()
function, which thanks the caller and ends the call.
Update workers status
Workers can update their availability status by sending SMS commands (e.g., "on" to become available). To handle the SMS commands, create a file named sms.go within the handlers folder and paste the code below in it:
This function allows workers to update their availability status via SMS. It retrieves the sender’s phone number and message body, identifies the corresponding worker in the TaskRouter workspace, and updates their status based on the message (i.e., "on" for available and "off" for offline). If any errors occur, an appropriate response is returned.
Put it all together
Create a main.go in the project’s root directory and then paste the following code in it:
This is the entry point of the application. It initializes the Twilio configuration, defines HTTP routes, and starts the server.
Run the application
If you haven’t already exposed the application to the internet, do so now by running the following command:
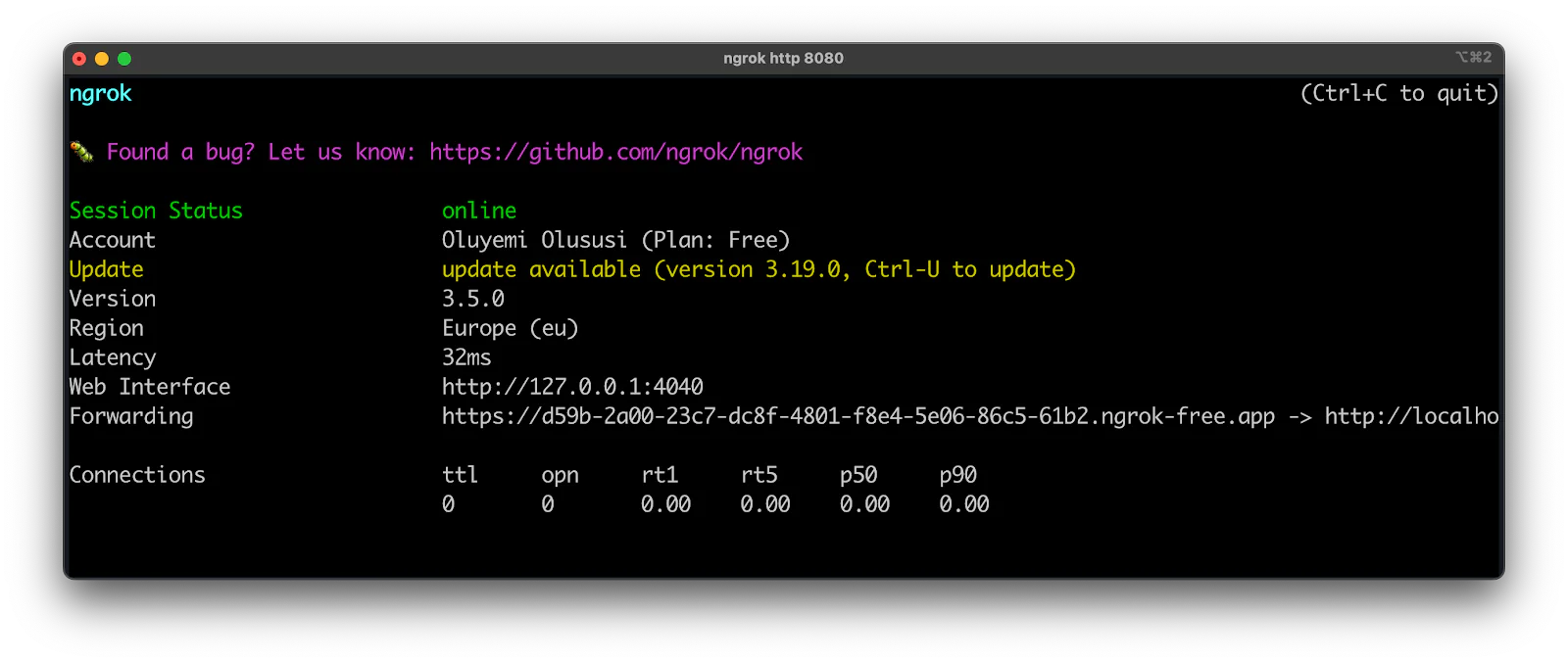
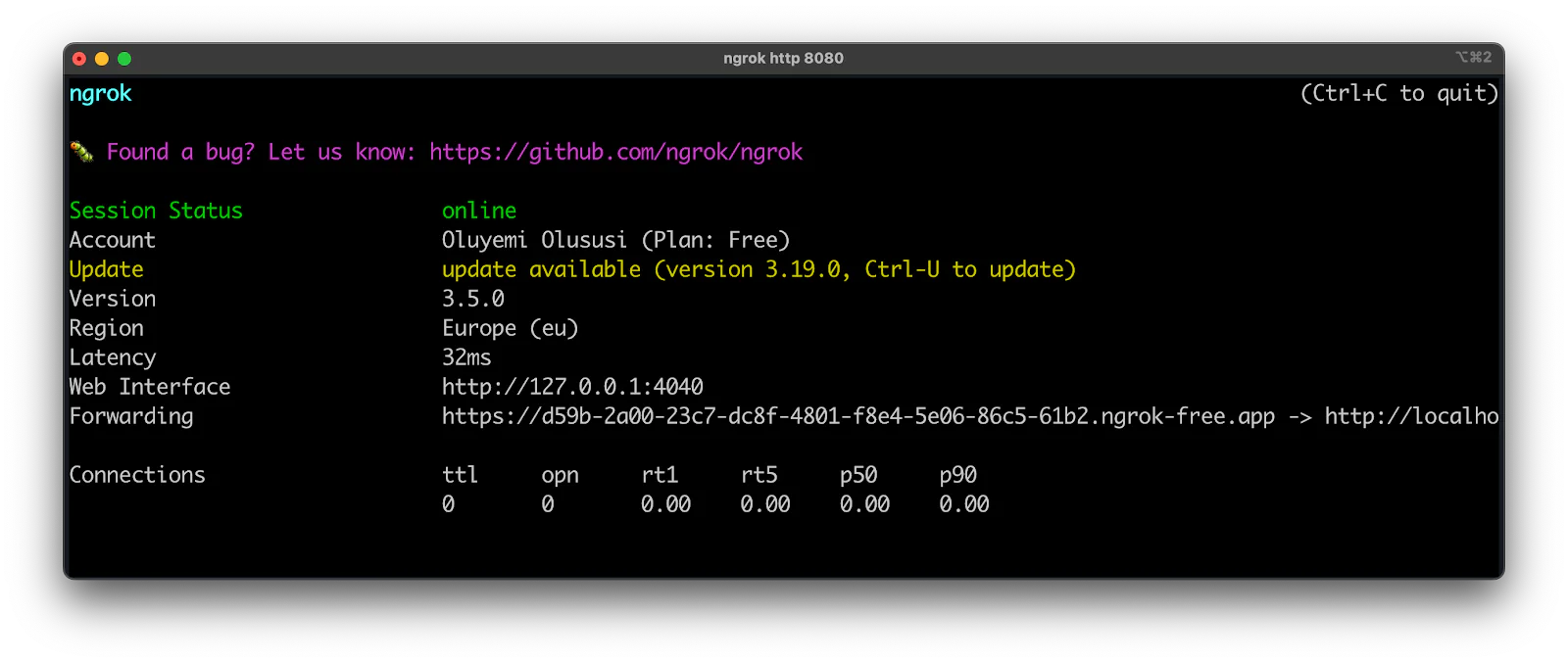
Copy the generated Forwarding URL from the terminal output, setting it as the value for HOST_URL
in your .env file. Then, in the Twilio Console Dashboard, configure the webhooks for your Twilio phone number as follows:
- Go to Explore Products > Phone Numbers > Manage > Active Numbers in your Twilio Console.
- Click on the phone number that you want to use for this tutorial.
- Under Voice Configuration:
- Set "Configure with" to "Webhook, TwiML Bin, Function, Studio Flow, Proxy Service"
- Set " A call comes in" to "Webhook"
- Set the webhook URL to
<your ngrok Forwardingurl>/incoming
- Under Messaging Configuration
- Set " Configure with" to "Webhook, TwiML Bin, Function, Studio Flow, Proxy Service"
- Set " A call comes in" to "Webhook"
- Set the webhook to
<ngrok_url>/sms
- Then, click Save configuration.
Once these updates are complete, you’re ready to run the application. Start it with the following command, in a new terminal tab or session:
The command above will run the application on port 8080 and perform the following setup automatically:
- Create a new workspace: Sets up the core TaskRouter environment
- Add workers: Configures workers with specific skills and contact details
- Create task queues: Defines queues based on product types for task routing
- Create a workflow: Establishes the logic for routing tasks to the appropriate workers
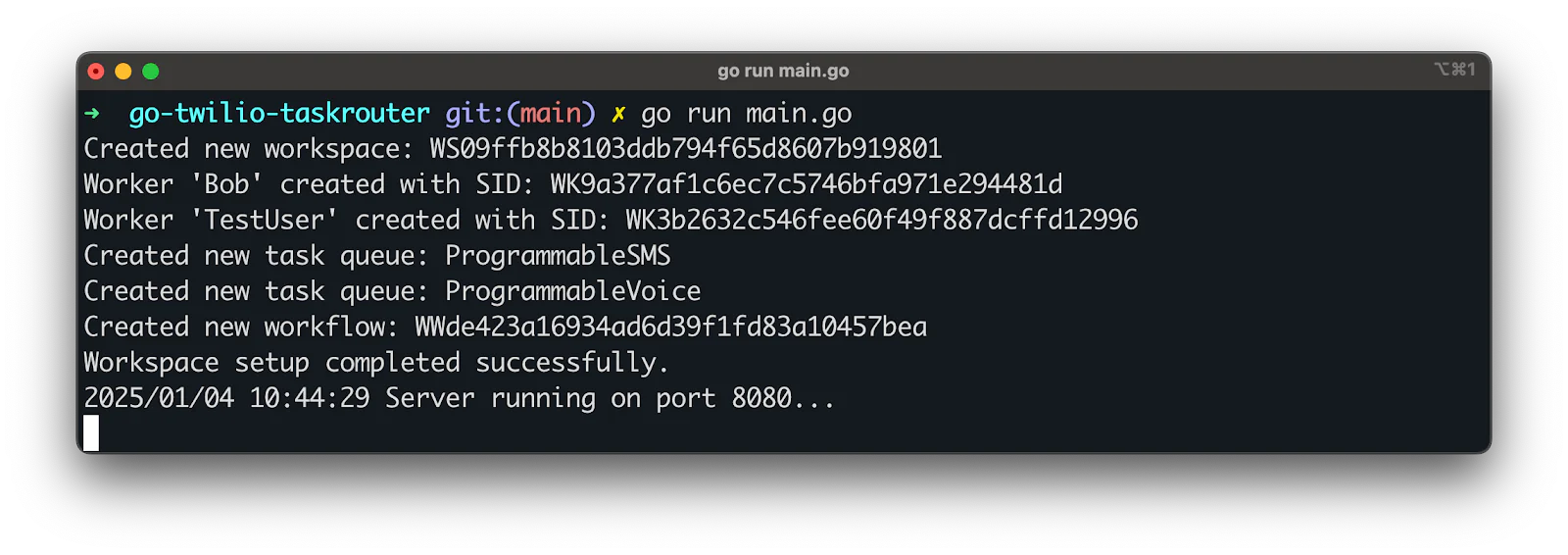
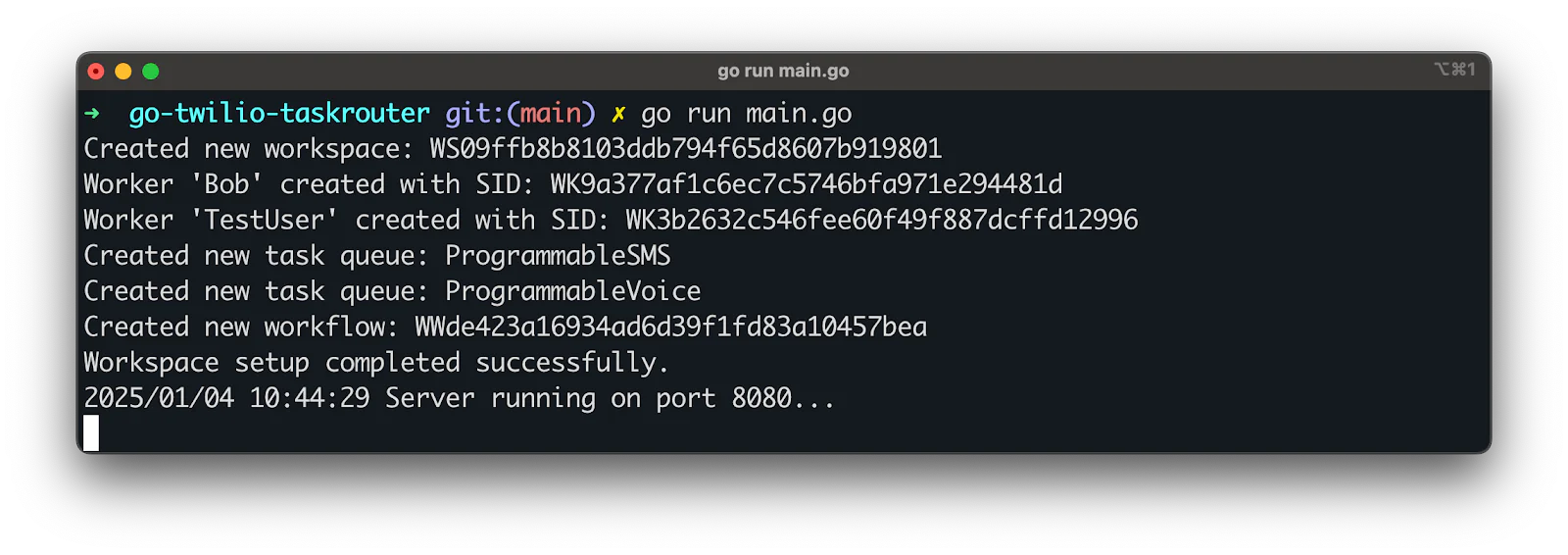
Test the Application
Once the application is running, follow these steps to test its functionality. Dial your Twilio phone number. You will be prompted to press "1" for Programmable SMS or "2" for Programmable Voice. Follow the prompt by pressing the corresponding number on your dial pad.
Based on your selection:
- If an agent is available, you'll hear a message mentioning the agent's name.
- If no agents are available, the call will redirect to voicemail. Leave a brief message after the beep, which will automatically end after 10 seconds or upon pressing "#".
At the moment, all workers should be offline because they were just created. This means you will be redirected to voicemail.
Update agent availability
All the workers are initially either offline or unavailable. Check the Workers Dashboard, which you can find in the Twilio Console under Explore Products > TaskRouter > Workspaces > Twilio Center Workspace, and click on Workers for details. You will see a page similar to the screenshot below:
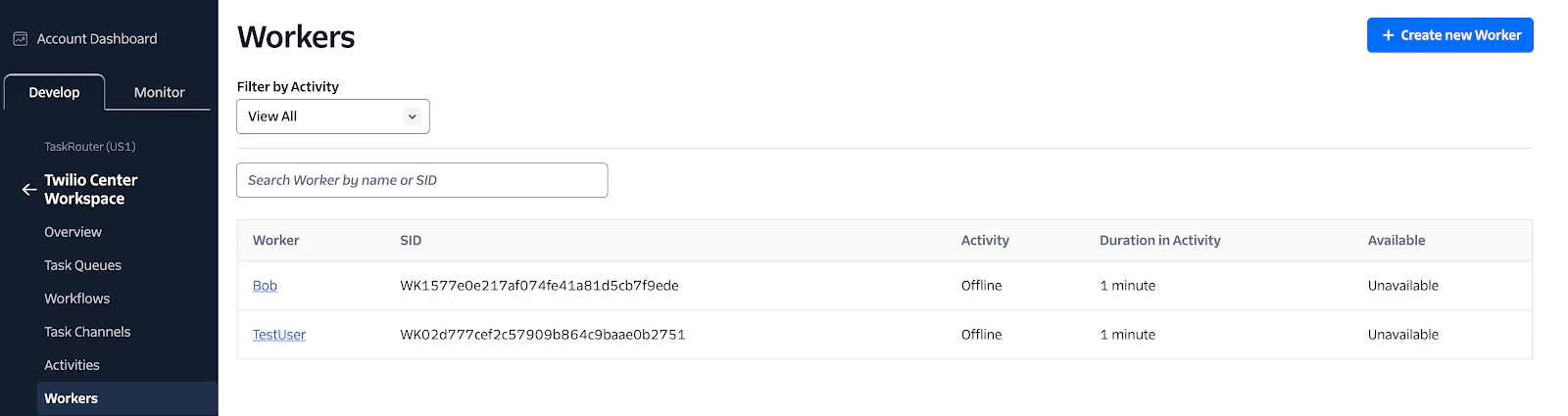
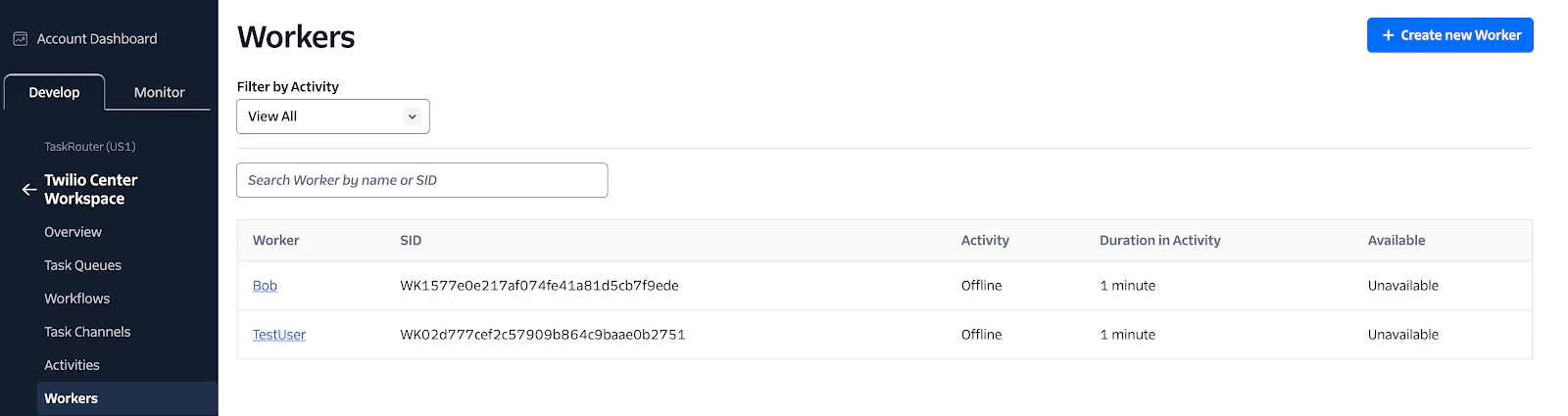
If you have added your phone number as the test user from the workers created programmatically earlier, send an SMS from your personal phone number to your active Twilio’s phone number. Start with an invalid unrecognized command such as “Hello”:
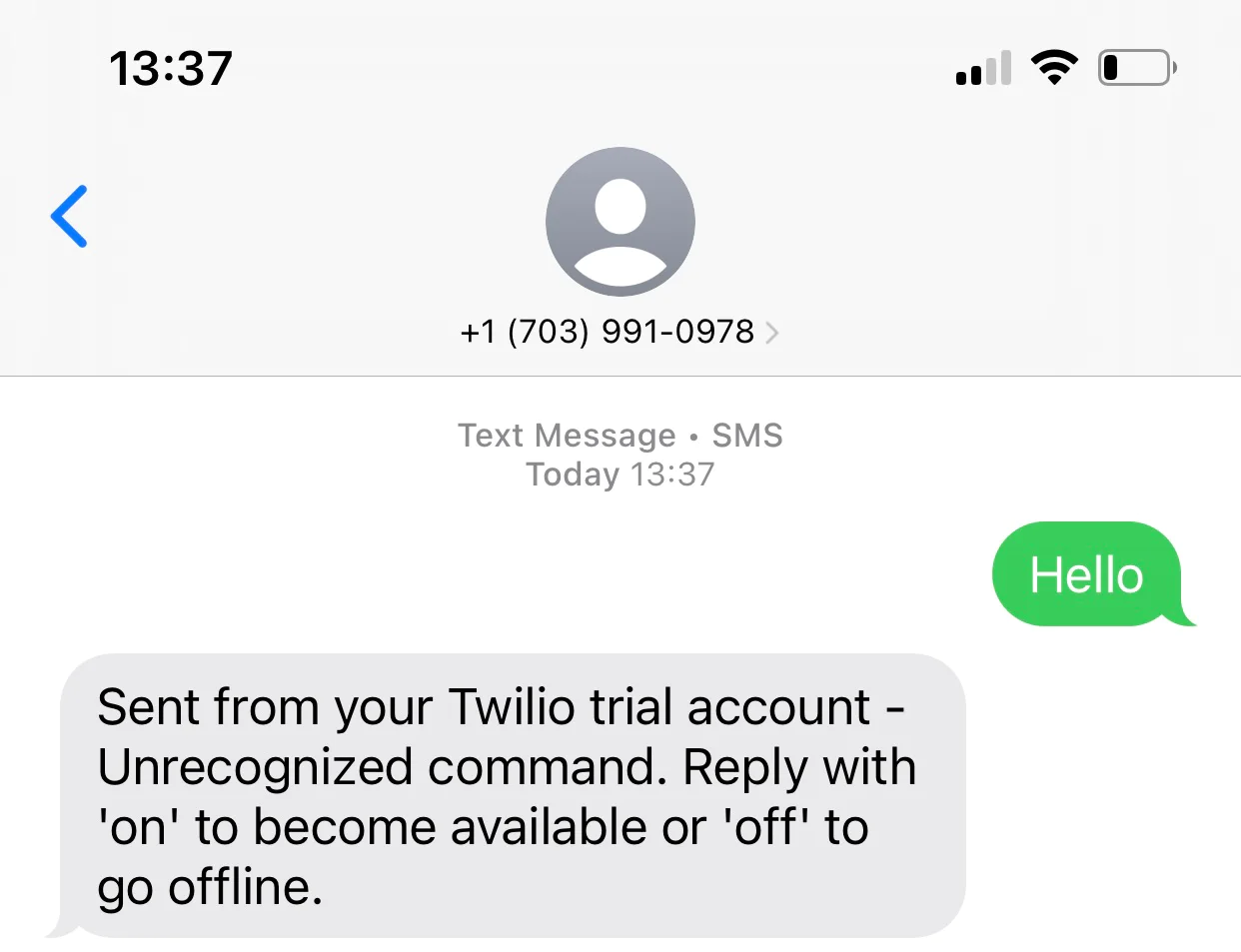
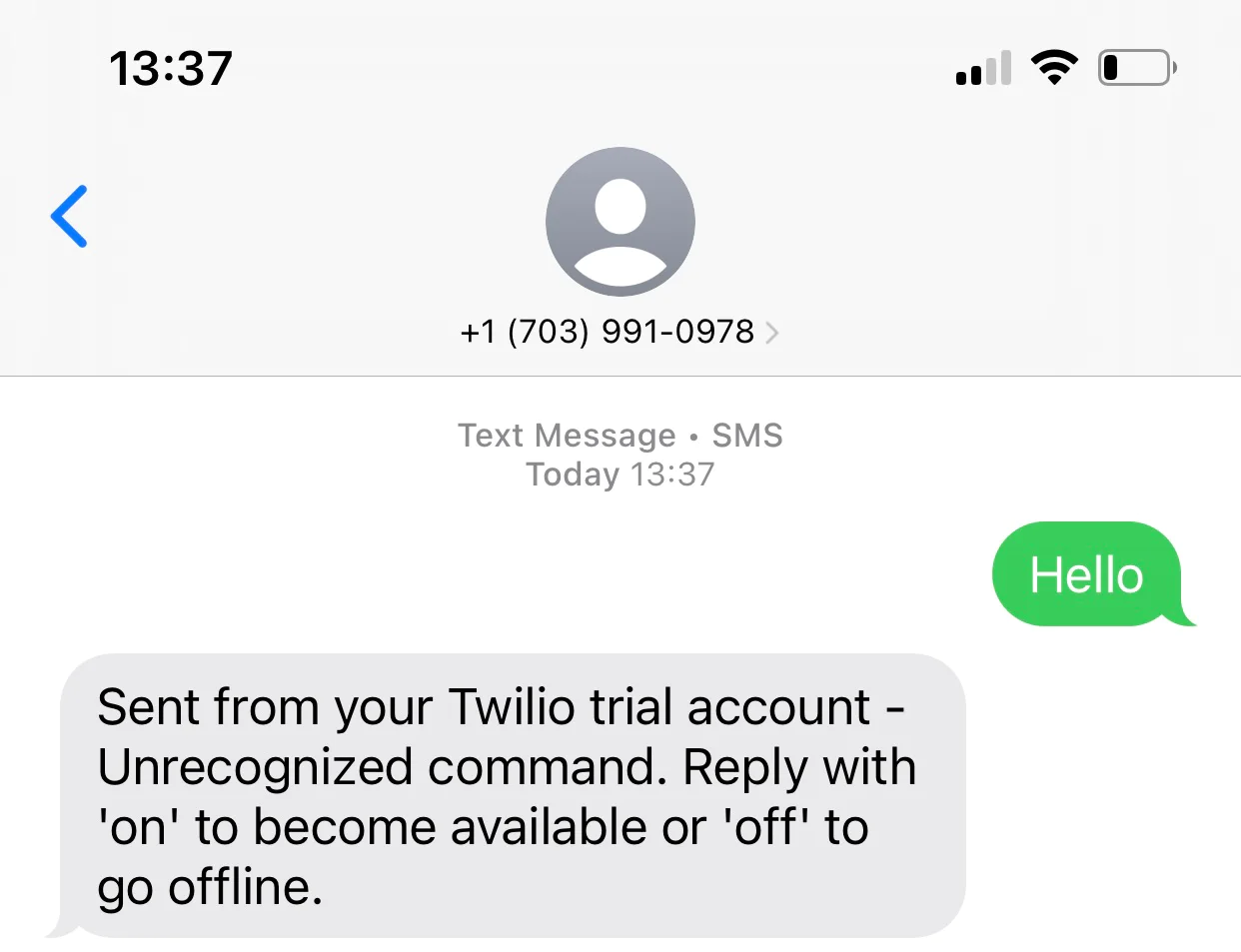
Now, change that and send a recognized command such as “on”, to set the worker as available.
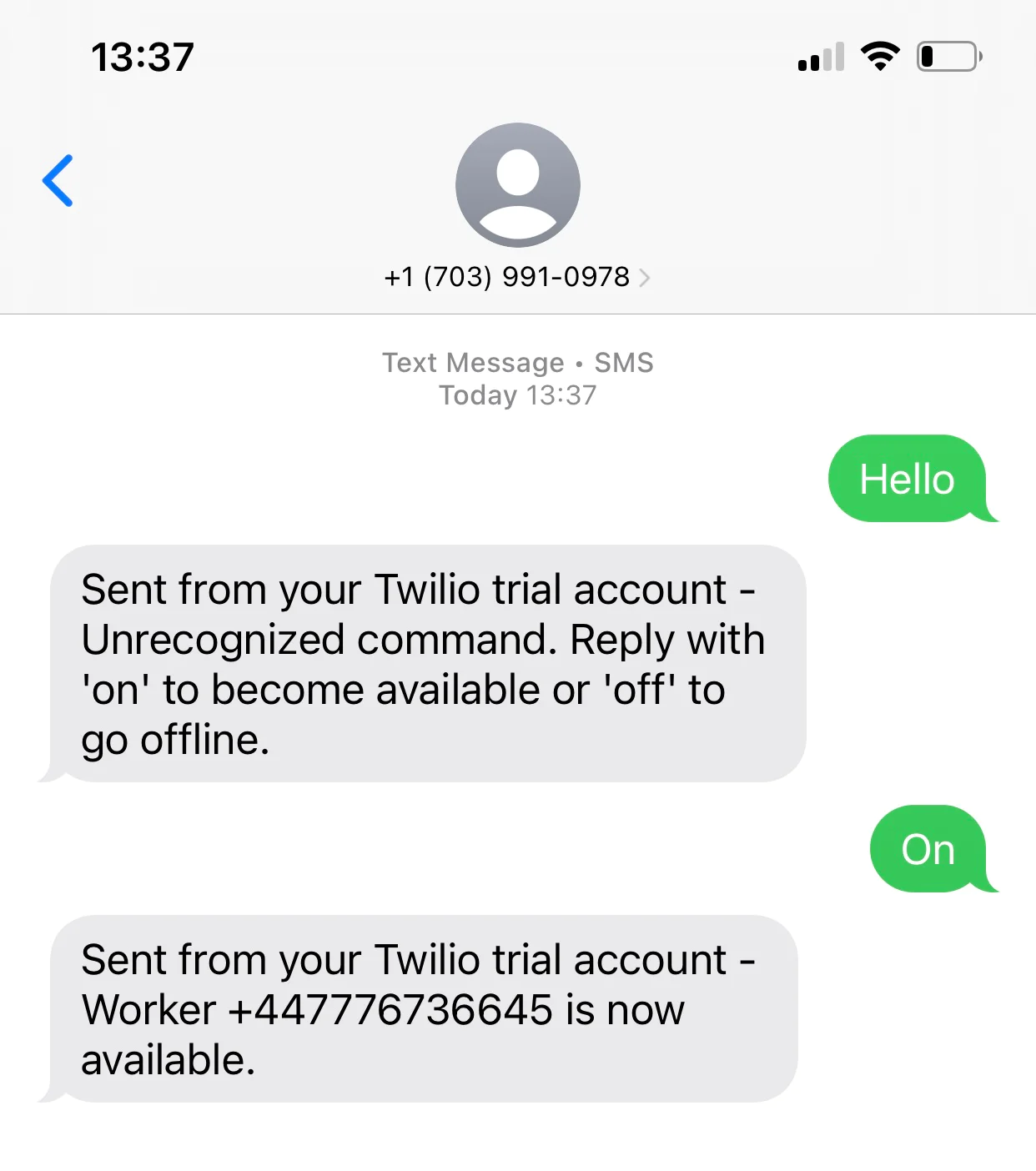
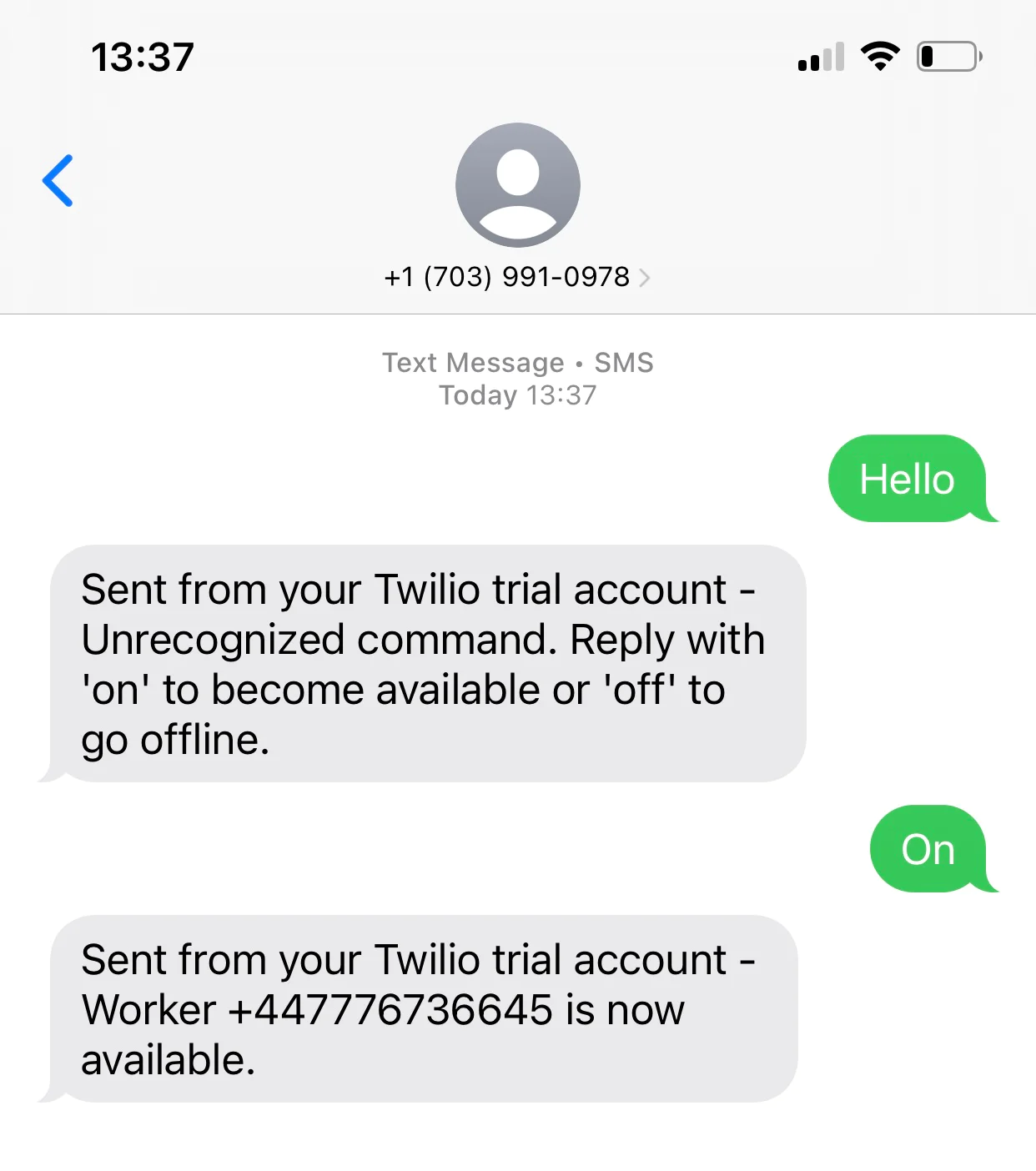
That would change the status of the “Testuser” on the dashboard. With the updated worker status, call the Twilio phone number again and follow the prompt. If a worker is available for the selected product, the system will announce the worker’s name.
That's how to automate call center management with Go and Twilio's TaskRouter
This tutorial provides a foundation for building a call management system with Go and Twilio TaskRouter. From setting up a workspace to handling calls and missed interactions, you now have a functional demo that can be extended with additional features like database integration or advanced reporting.
For further enhancements, consider adding:
- Event Logging: Implement the
/callback/events
endpoint to capture and log TaskRouter events. This can be useful for monitoring, debugging, and even triggering custom business workflows based on real-time updates. - Missed Call Management: Extend the missed call functionality by logging unanswered calls into a database and notifying agents for follow-up actions, ensuring no customer inquiry goes unresolved.
These additions can further streamline your call center operations, providing deeper insights and improving customer service efficiency.
I hope you found this tutorial helpful. Check out Twilio's official documentation to explore additional products and services that can help grow your business. The complete source code for this tutorial can be found on GitHub. Happy coding!
Oluyemi is a tech enthusiast with a telecommunications engineering background who leverages his passion for solving everyday challenges by building web and mobile software. A full-stack Software Engineer and prolific technical writer. He shares his expertise through numerous technical articles while exploring new programming languages and frameworks. You can find him at: https://www.linkedin.com/in/yemiwebby/ and https://github.com/yemiwebby.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.