Add Twilio SMS Notifications to Your .NET Application Logging
Time to read: 4 minutes
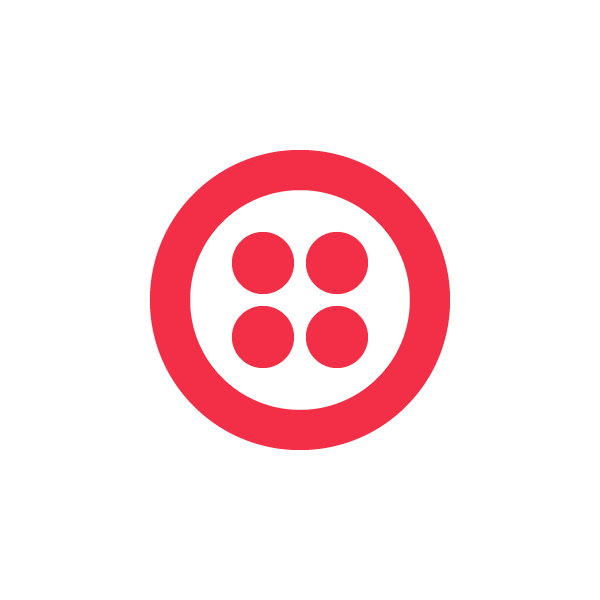

NLog is a popular logging framework for .NET applications, which by default it includes a whole bunch of different Targets, or locations where log events can be written. In this post I’ll show you how you can create a custom NLog Target that sends log messages to you as an SMS message using Twilio.
Custom NLog Targets
To get started creating my custom NLog Target, I first created a new Class Library project in Visual Studio and then added the NLog and the Twilio packages from NuGet. Once the packages were added, I create a new class called SMS in the project. The image below shows the initial project setup.

With the initial project set up, I started to write the code for the SMS target. First, in order to get NLog to recognize the Sms class as a target, I did two things. First,I modified the class to derive from the NLog.Targets.TargetWithLayout base class, and second I added the NLog.Targets.Target attribute to the class with a unique name.
Next I added properties to the class to let me specify Twilio details like my Account SID and Auth Token, and the From and To phone numbers that I am using to send the message.
I’ve indicated that each of the properties is requires a value by applying the Required attribute on each.
Next, I added to the Sms class a method that actually sends the SMS message using the Twilio .NET helper libraries SendMessage method.
Finally, I tie everything together by overriding the TargetWithLayout’s Write method, which NLog calls whenever my application writes a message to NLog. In the overriden method I render the NLog event to a string and pass that to the SendTheMessage method.
And that’s all it takes to create my custom NLog Target. Next l’ll show you how to use this Target in a simple console application.
NLog Target Configuration and Testing
To configure and test the custom NLog Target I created in the previous section, I added a new console application project to my existing solution, and then added the Twilio.Targets project as a reference. I also added both the NLog and NLog configuration packages from NuGet. Adding the NLog package adds the NLog assembly as a project reference, and adding the NLog Configuration package adds an empty NLog.config file to my project. The NLog.config file is used to set NLog configuration information, including configuring my custom Target.

First, in order to tell NLog about the assembly that contains my custom Target, I added a new element to the extensions section.
Next, to tell NLog about the Target in my assembly I added a new target element, setting values for the properties exposed by my Target class.
Note that in the sample the Target property values replaced with placeholders. In your NLog config file you would insert your account information into the attributes shown.
Finally I added a new logger element which lets me tell NLog when it should use my custom Target.
For my sample application, I configured NLog to use the SMS target for all log events Warn level and above.
Once NLog was configured to use my Target, in order to test it I called some of the NLog event logging methods from my console application.
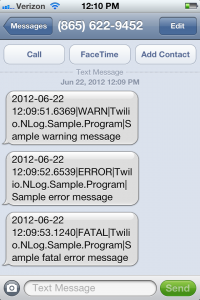
And thats all there is to create a custom NLog Target to send log events to you via SMS.
Summary
Logging is an essential part of any application and NLog is a great option for easily adding high configurable logging to your .NET application. Its also easy to use NLog to create custom log Targets, including the SMS target shown in this post.
The complete source code for my custom SMS NLog Target can be found here, and an example NLog config file can be found here.
Find more .NET Developer How To posts here. I’d love your comments or questions about building a custom NLog Target. Feel free to email me at devin@twilio.com or tweet me at @devinrader
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.