Build a Wake Word Detection Assistant in PHP using Ratchet for WebSockets and Twilio SMS
Time to read: 3 minutes
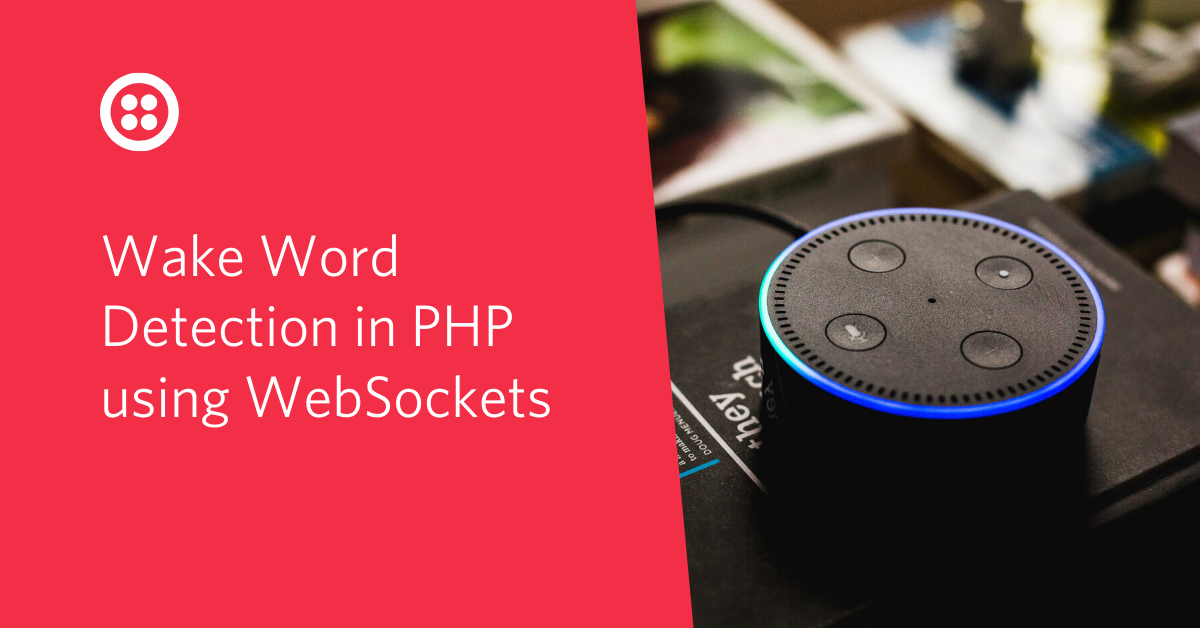
Due to their data transmission speed and low latency, WebSockets open up exciting possibilities for real-time apps. Many of your favorite apps such as Google Docs, Instagram, and Facebook are already using them to keep us in sync with each other using live data.
We’re going to dive deeper into the world of PHP WebSocket development by building a wake word and hot word detection assistant that communicates with us via SMS, similar to an Alexa.
To begin, we’ll use the introductory tutorial, “How to Create a WebSocket Server in PHP to Build a Real-Time Application”. We’ll modify the HTML to create a <textarea>
that streams the input value to our WebSocket. Our Socket()
class will listen for a list of predefined keywords and upon a match, send an SMS to the user.
If you haven’t already done so, download the repo here.
Tools Needed to Complete This Tutorial
To complete this tutorial we will need the following tools:
- The starter repo cloned to our local environment
- A free Twilio account
Install the Twilio PHP SDK in the project
Twilio provides a near-seamless experience to incorporate voice, SMS, and video into your application. For this project, we’ll use the Twilio PHP SDK to connect to the Twilio SMS API. The Twilio API will be used to send SMS to the client users later in the tutorial.
To install the SDK, run the following command:
Next, we’ll need a way to securely store our Twilio account credentials. We’ll build on the work of the phpdotenv project by Vance Lucas. The dotenv package will create a mechanism to load environment variables from a .env
file to getenv()
, $_ENV
and $_SERVER
. Let’s install it by running the following command:
Our Twilio installation is now almost complete. The last thing we’ll need is to update the .env
we just generated with our Twilio Credentials.
Add the following variables as seen below.
Navigate to your Twilio account dashboard and copy your “Account SID” and “Auth Token” into the respective variable above. Then, navigate to the Twilio Phone Numbers section of the console and copy your incoming number into the TWILIO_PHONE_NUMBER
variable. Be sure to paste it in E.164 format.
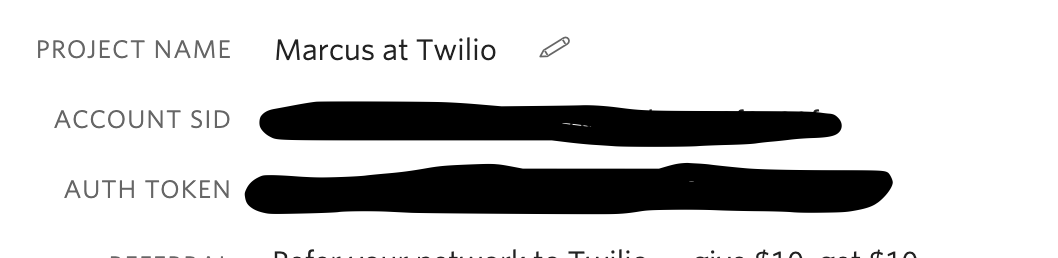
Finally, open app.php
and add the following highlighted lines:
This will load the .env
file and expose the values of the variables we defined to getenv()
.
Add Wake Word Detection to the Socket Class
There are many philosophies and engines on wake-word or hot-word detection. We’ll ignore those today and focus on exploiting WebSockets for real-time data processing.
Open app\socket.php
and replace the file with the following code:
You’ll notice that the onMessage()
method now processes our input in real-time. Upon detecting a phone number, it sends a message to the client.
Now that our wake-word detection is in place, we can move forward to updating our client-side application to test things out.
Create the Client-side Application
Open the index.html
file and replace the code:
This form accepts the phone number of the client along with a message to send to our bot.
Every keystroke is transferred to the WebSocket for real-time processing. Whenever the wake word “Hey Bot” is transmitted, the interface will respond accordingly, followed by a response for each subsequent command.
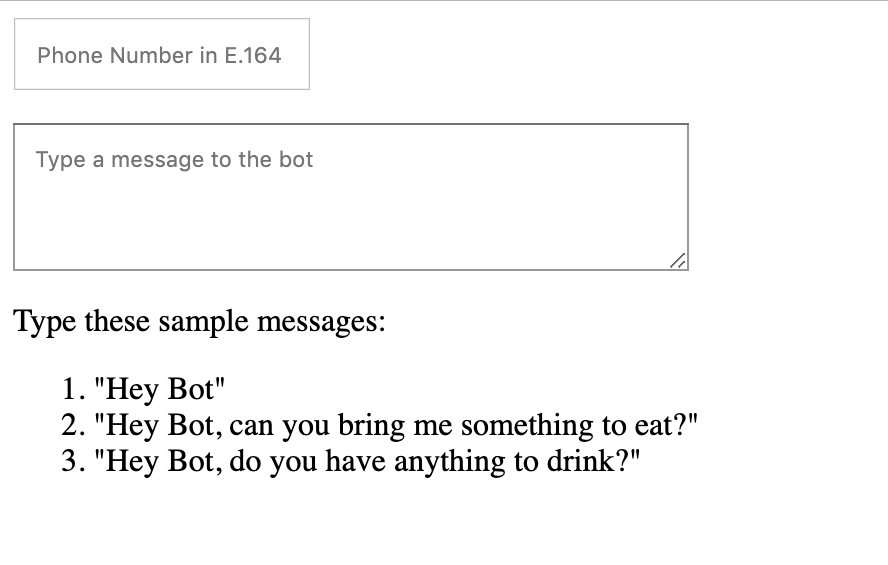
Test the Wake Word Assistant
In your terminal, start the WebSocket server by running:
In a separate terminal run ngrok to expose the HTTP server to the internet.
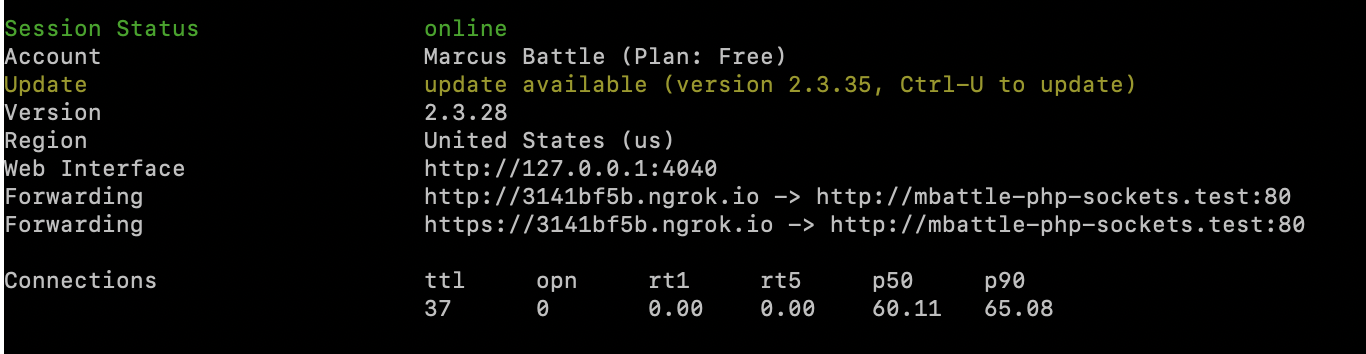
Copy the HTTPS Forwarding address and paste it into your browser.
Follow the prompts in the interface and look for an SMS to be sent when you trigger the “eat” and “drink” keywords.
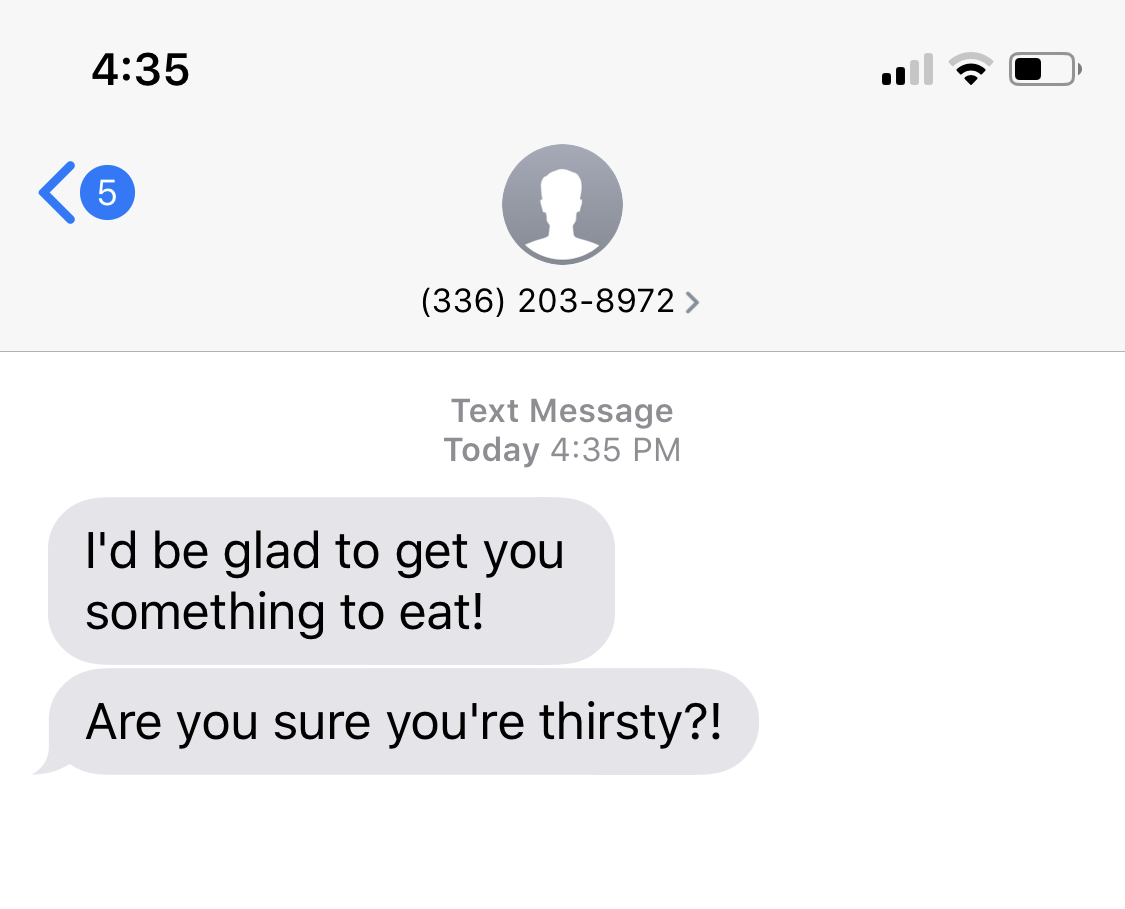
Conclusion
Because we capture the phone number of each client, this tutorial could be extended to send SMS messages to each connected client in real-time.
Marcus Battle is the PHP Developer of Technical Content at Twilio. He is committed to prompting and rallying PHP developers across the world to build the future of communications. He can be reached on Twitter at @themarcusbattle and via email.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.