Building Video Applications on Android
Time to read:
This post is part of Twilio’s archive and may contain outdated information. We’re always building something new, so be sure to check out our latest posts for the most up-to-date insights.
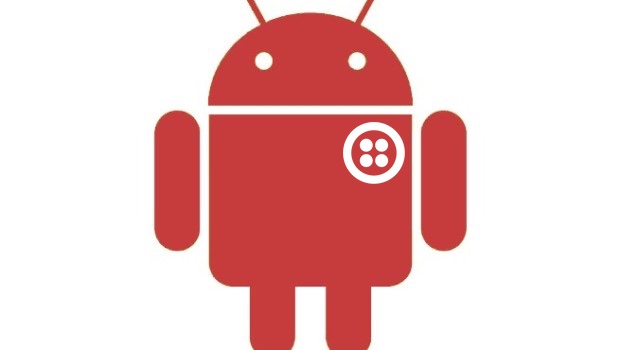
Context is everything, and there’s nothing more annoying than using a mobile application and then being told you need to switch to a different app to communicate with other users or technical support. In my last blog post I showed you how to add IP communications into an existing application using the Twilio IP Messaging SDK for Android.
Twilio also offers a Video SDK which can be used to add video communications into the apps you’re already building.
In this blog post I will show you how to use the Twilio Video SDK and how to video applications on Android that let you have a video conversation between a device and a browser.
What you’ll need
- A free Twilio account, which comes with access to the Twilio Video public beta
- A server-side quickstart and access token generator. I’ll use the .Net quickstart in this tutorial but there are server options for six major programming languages and you can use any of them.
- Ngrok to provide a tunnel to our backend. You can read more about ngrok here.
Once you have downloaded the quickstart of your favourite programming language and followed the instructions to get your access tokens, make sure you open up a couple of browser windows and check that you can see yourself on both windows.
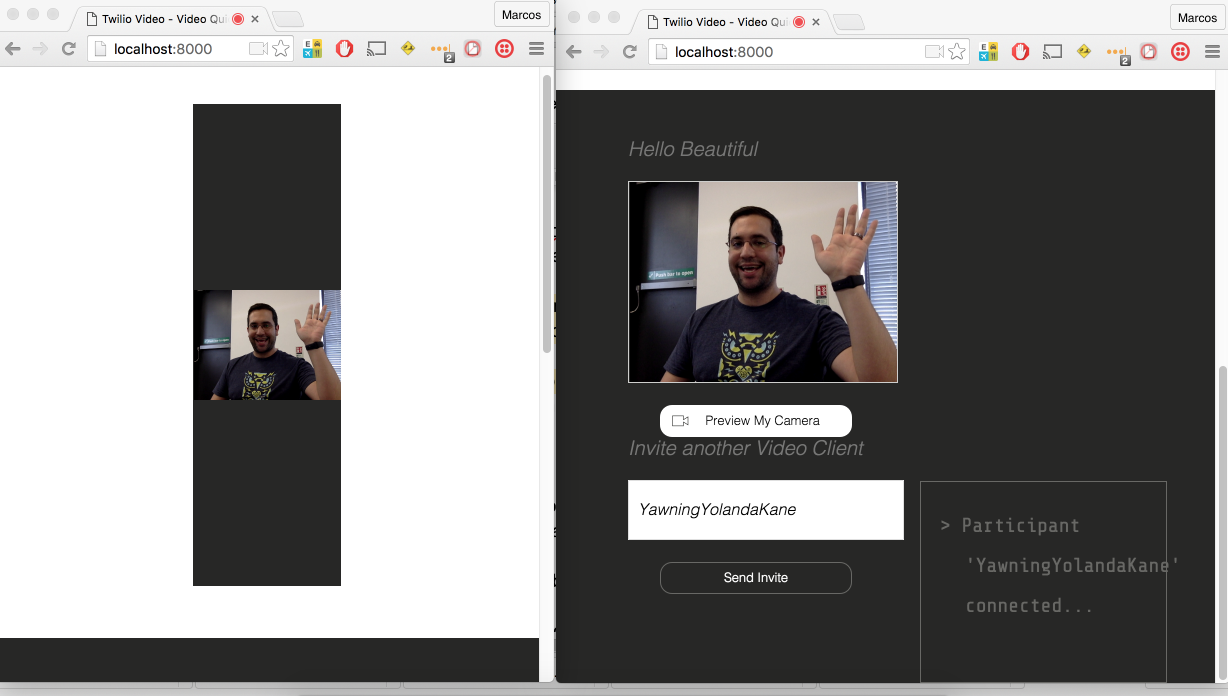
This is also a good time to start ngrok and check that you get access to your application using the ngrok URL. Make a note of it as we will be using it soon.
In my terminal I just ran:
If you don’t feel like going through the entire build, feel free to download and run the app from my GitHub repository.
Setting it up
In Android Studio create a new project called Twilio Video and choose a location for it. I usually store my Android apps in /Users/mplacona/Projects/Android/ but feel free to put it anywhere you like. Choose API 16 as the Minimum SDK.
Choose Basic Activity as your starting point for this application. This will come with a pre-baked Floating Action Button which we will modify and use later on.
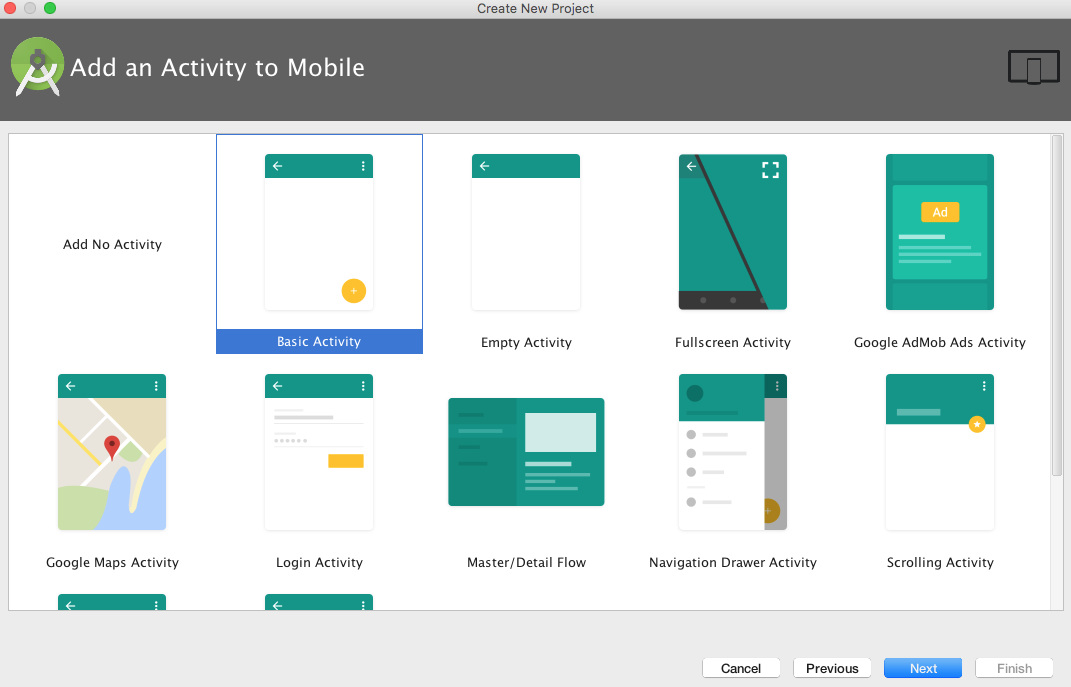
Click Finish on the next screen and you’ll be ready to start building the application. If you want to make sure your build is working correctly, now is a good time to plug in your device and run the application.
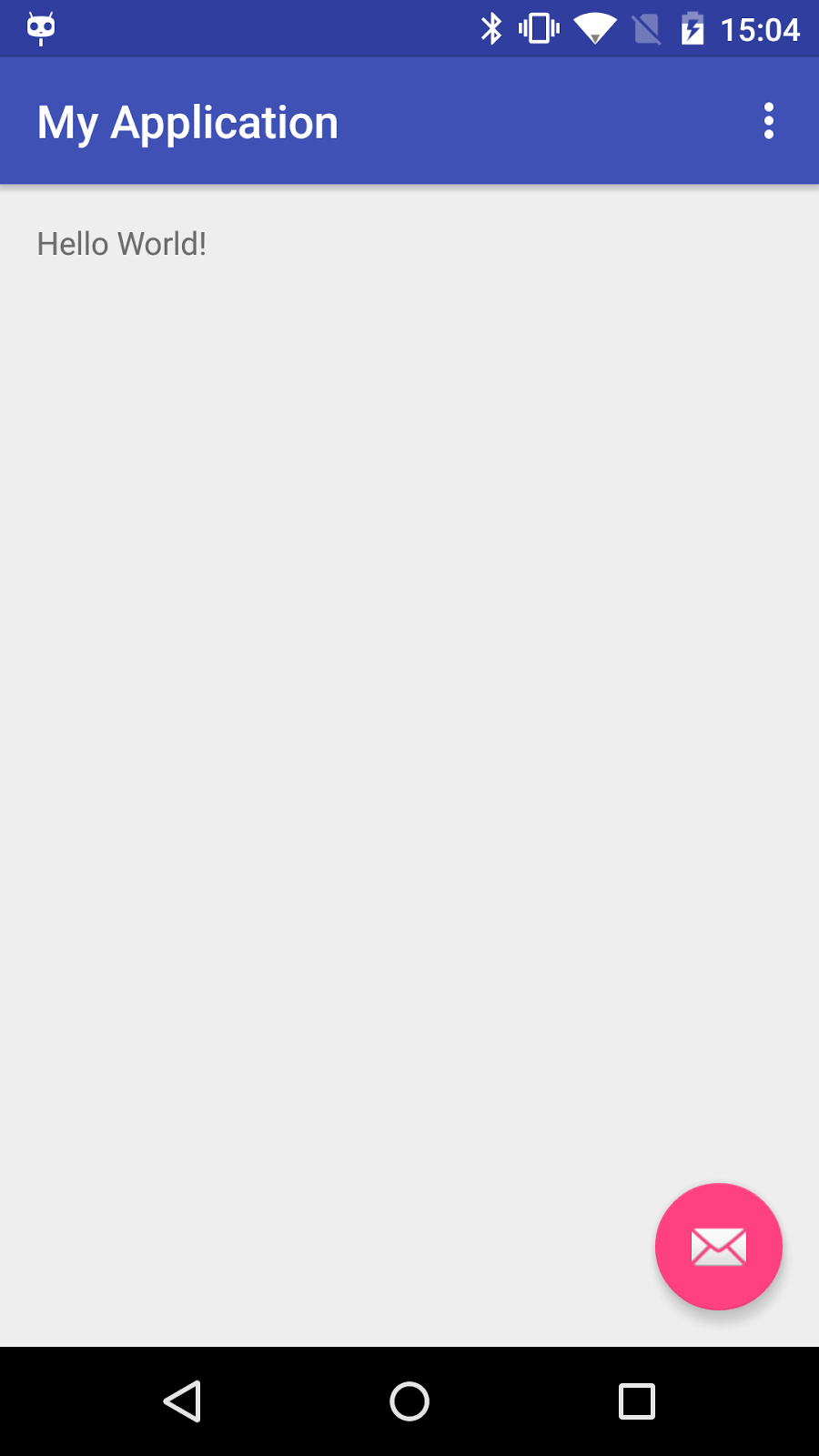
Building the layout
Now that we’ve created the app and its initial files let’s make a few changes to the layout to accommodate our video conversations.
Open TwilioVideo/app/src/main/res/layout/activity_main.xml and change the icon and background colour of your floating action button as follows:
In TwilioVideo/app/src/main/res/layout/content_main.xml delete the existing TextView, create a FrameLayout and add a couple of Relative Layouts inside. These will be our containers where we will later see a video of ourselves and one of our friends.
Permissions & Dependencies
Now that our application has a layout we can download and reference the necessary dependencies. We will also ask for the required permissions to access the device’s hardware.
In order to get access to the device’s camera, we need to install and initialise the Twilio SDK. To install it, change TwilioVideo/app/build.gradle to have the following added to it:
At this point Android Studio will tell you that “Gradle files have changed since last project sync”. Click Sync Now, and all the dependencies will be downloaded and properly referenced.
Open TwilioVideo/app/src/main/AndroidManifest.xml and add permissions for camera, audio and internet access.
Open TwilioVideo/app/src/main/java/com/twilio/twiliovideo/MainActivity.java and create a condition to check that the permissions have indeed been given. We can do that by creating a couple of new methods inside the class to check and then request when the permissions have not been granted.
We can also remove some of the unnecessary methods in this class so it looks like the following code.
We’re now going to add a few member variables to the class, and replace the whole of onCreate with the following.
This will make use of our new permission methods to check whether access to the camera and microphone have been granted, then create references to our layout widgets which we will use later on. Lastly, it will make a call to the method getCapabilityToken which we’re just about to create.
Initialising the Twilio SDK
Make sure the quickstart application you downloaded earlier is still running, and that you have its ngrok URL. We will initialise the Twilio SDK by giving it a Capability Token that is returned by the quickstart.
Very soon you will be wondering which are the correct imports to use because you will have a lot of red squiggles under your code. To make things easier, just copy all the imports from this file and replace what you currently have.
In MainActivity.java create a new method called getCapabilityToken inside the class. This will make use of our ngrok URL.
Notice that we have also created a method called run, which uses OkHttp – one of our dependencies – to make an HTTP request to our backend application. Make sure you replace the contents of [your-ngrok-url] with the URL you got from ngrok.
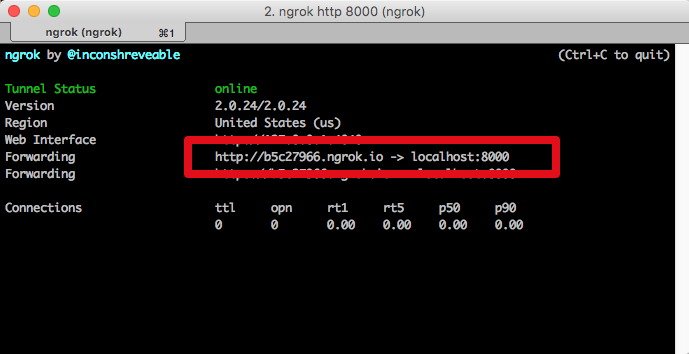
The new method overrides OnResponse with a call to initializeTwilioSDK which we will create in a minute. That method will only be called when we get a response from the server with a capability token.
There are four important things to notice on the code above. We first get access to the TwilioAccessManager interface which will authenticate the application using the capability token we got earlier and listen to any expirations or errors on that. Once we have that, we pass this information to a listener.
We then create a new conversations client which takes an event listener called conversationsClientListener and listens to things like new invites to the conversation.
Once the conversation is established we get access to the camera and when that’s granted, we start previewing it to the screen.
Let’s add these event listeners to the bottom of the class and a method to start and stop previewing the camera on the screen. We will bind the camera to the previewFrameLayout we created earlier.
The methods accessManagerListener, conversationsClientListener and capturerErrorListener only listen for requests coming back from the access manager, conversations or errors. startPreview and stopPreview will turn your local camera on or off upon the start or end of a conversation.
Stop the application and run it again.You should see that the local camera starts and you can see yourself on it.
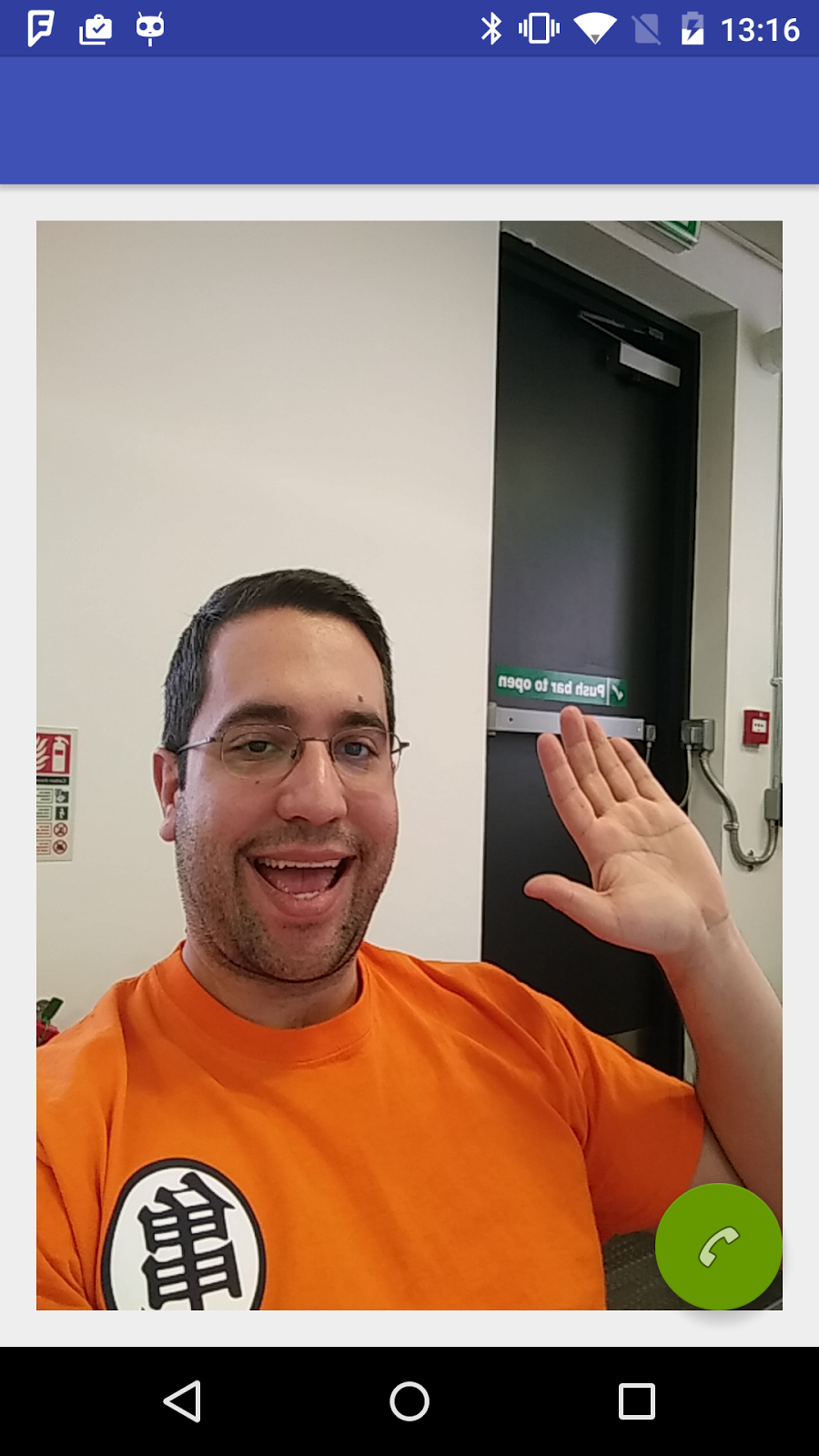
Now change the onIncomingInvite method so it handles new invites coming to this conversation.
We’ve introduced five new methods in the code above. The hangup, reset and setHangupAction methods will disconnect a call and reset the view to remove any active widgets. Paste the following to the bottom of the class:
The listeners give us information about when a conversation starts, ends or when a participant connects or disconnects. Then there’s setupLocalMedia which will create an instance of local video and audio by calling LocalMediaFactory.
In conversationListener we’ve introduced three more listeners.
- participantListener for when a new participant joins.
- localMediaListener for listening to our local media, that’s the camera and microphone.
- hangupActionFabClickListener for when the hangup button is pressed.
Add these to the bottom of the class.
Back in the reset and hangupActionFabClickListener methods we introduced a call to setCallAction, which does the opposite of setHangupAction. By clicking the hangup button, your Activity should reset and the widgets should be ready to display video for both parties.
The last piece of the puzzle is to handle clicks on the Floating Action Button when it’s ready to make a call. At the bottom of the class create a new method called callActionFabClickListener.
When the button is clicked, we call stopPreview so the local camera stops showing us on the big frame. We hard code a participant we want to invite and set up our layout so it’s ready to display both ours and the invitee’s camera.
We then send the invite and upon acceptance reset the button to enable us to hangup the call and disconnect.
We need to make sure our button is ready to be clicked when the application starts. Scroll to the top of the onCreate method and add the following to it.
Test this out by loading up the quickstart on the browser and getting the username assigned to that tab. Copy that name into participants.add(“WhateverNameYouWant”) in Android Studio and run the application.
Click the green button and you should see yourself on both screens now. The top one is your local camera, and the bottom one is from your browser. Click the red button to hangup.
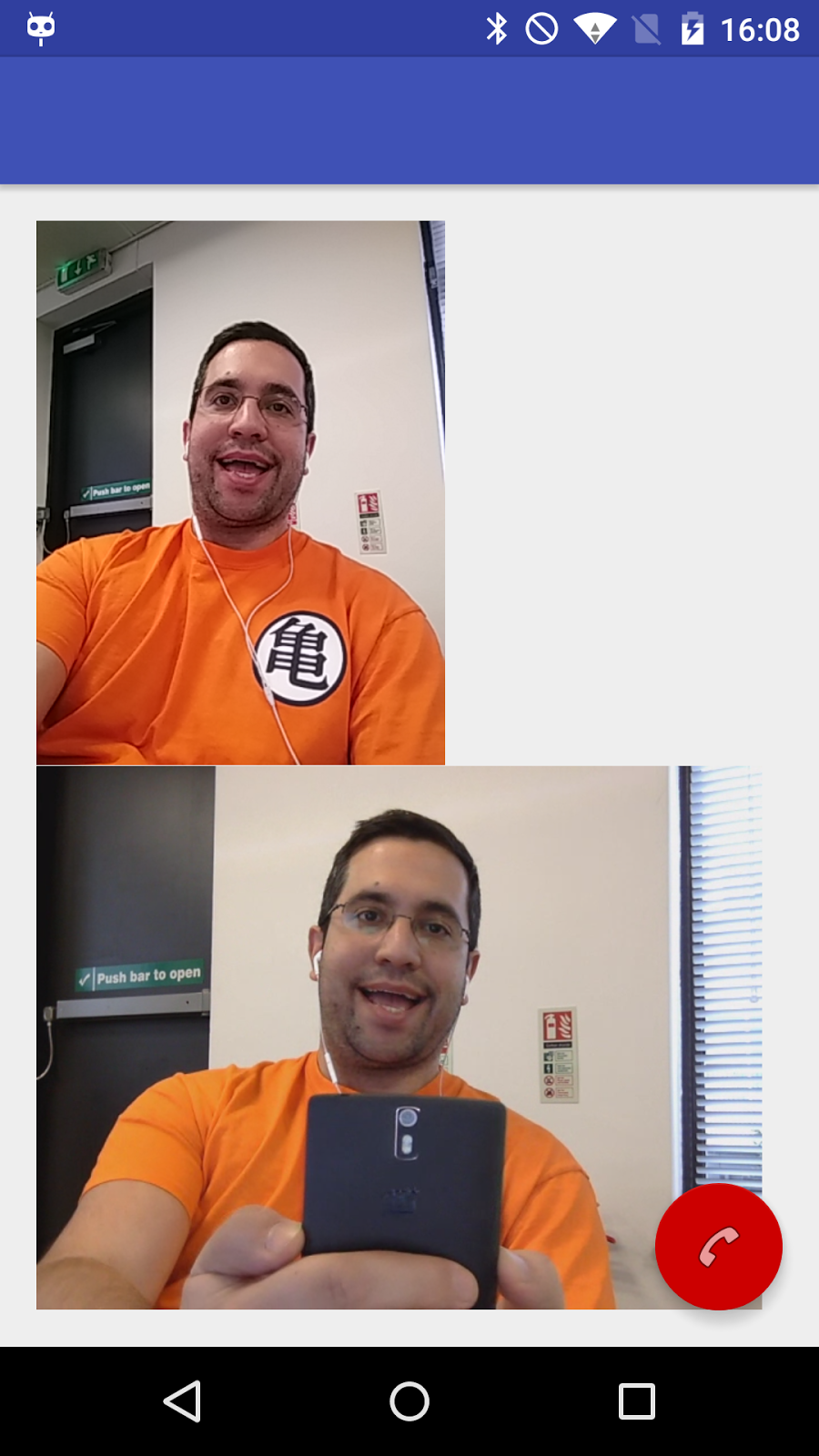
Get your friends involved
Now that you’ve built an app that lets you do video chats between your device and the browser it’s time to get your friends involved. Send your ngrok URL to a friend and just tap the call button and get chatting with of them.
Bonus points for modifying the quickstart so your friends can use their actual names. How about adding the ability for them to call you from the browser?
I can’t wait to see what you’re gonna build. Hit me up on Twitter @marcos_placona or by email on marcos@twilio.com to tell me more about it.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.