How to Capture Call Tracking Metrics in Google Analytics with Twilio Programmable Voice
Time to read: 5 minutes
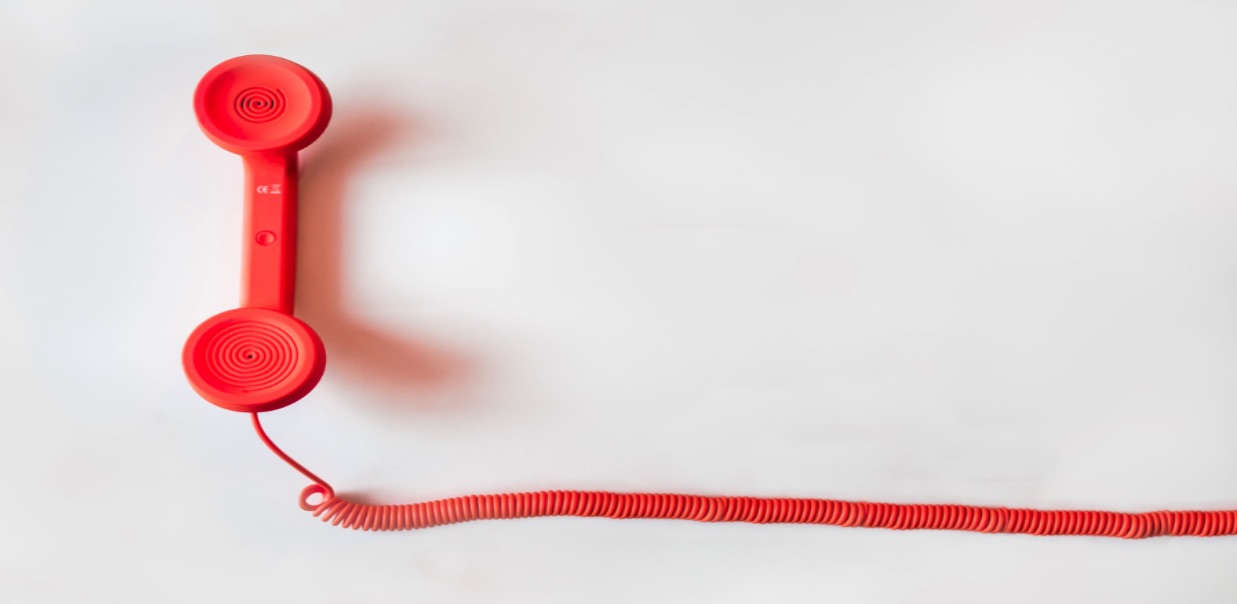
Call tracking involves saving a phone number and recording related the information about the calls made by that number. The metrics can then be used to measure the impact of marketing efforts on lead generation and sales.
Say that you are an entrepreneur or the marketing head of an organization and your main source of leads are phone calls. To spend your marketing dollars wisely, you will have to know which of your campaigns generate the maximum number of calls. This can be done using a call tracking system.
Call tracking systems used to be very expensive and cumbersome. However, in this blog post we will create an easy call tracking system that combines the powers of Twilio and Google Analytics to give you a very detailed picture of the calls and locations of these calls.

Configuring Google Analytics
Start by logging into your Google Analytics account or signing up for a new one.
Go to Admin->create account-> give the specification for website tracking. In the required column enter any website name and url (for example, www.example.com), also select the category as industry. To get the Tracking Id Go to Admin -> property ->Property Settings->you can now see the Tracking ID. Take a note of the tracking ID because it is needed to connect a Twilio Function to your Google Analytics property.
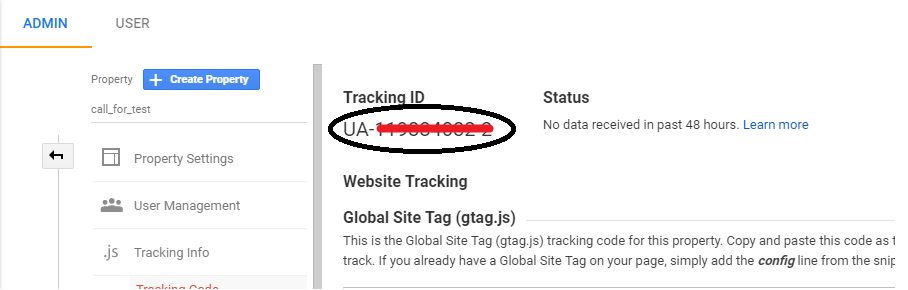
Log in to your Twilio account or sign up for a new account.
Go to Runtime, Select “Function”, and then click “+” symbol and select the “Call Forward" Function template. Press "Create".
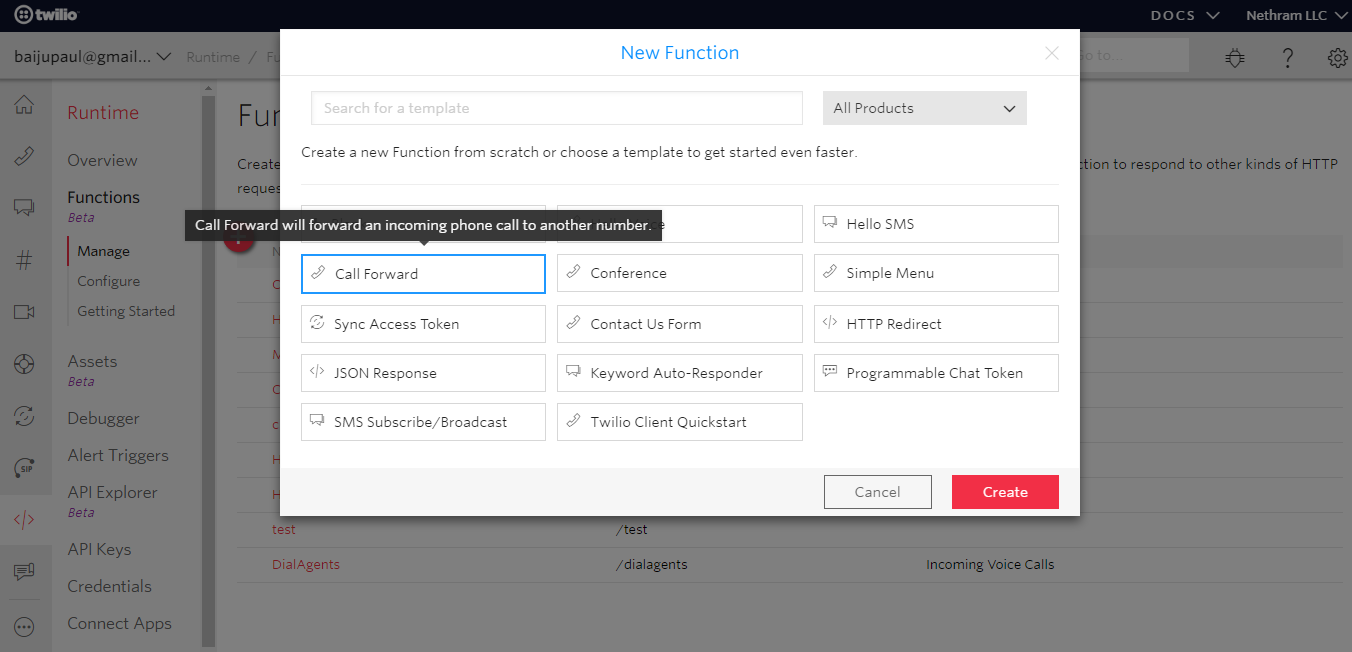
Open the Function and give the function name and the path as your choice. This Function path is needed later so, copy this path and save the Function.
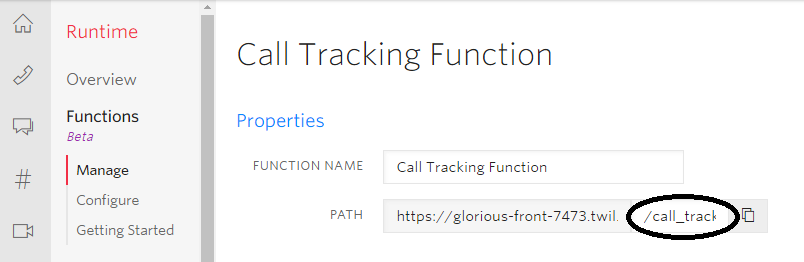
We can now associate our Twilio number with the Google Analytics tracking ID.
Associating Phone Numbers with Tracking IDs
Go to Phone Numbers (# icon in menu) in your Twilio account and select a Twilio number from the list of active numbers. If you don't yet have a number, buy a number and then go to Phone Numbers and select the number from the list of active numbers. After selecting the number, go to Voice & Fax option and select Voice calls. In the “A CALL COMES IN” box, set it as webhook and provide the details in the empty box beside it, in the following format:
Paste your function path followed by a question mark then "PhoneNumber=(here set the actual phone number which you need to be forward all the calls". Remove the spaces and hyphens in the number) then "&campaign=(here set campaign name of the number you have currently selected))&trackingId=(here set the tracking Id you got from google analytics)".
It's much easier to see in an example:
Save the changes. You can also change the phone numbers for different campaign for the same tracking ID.
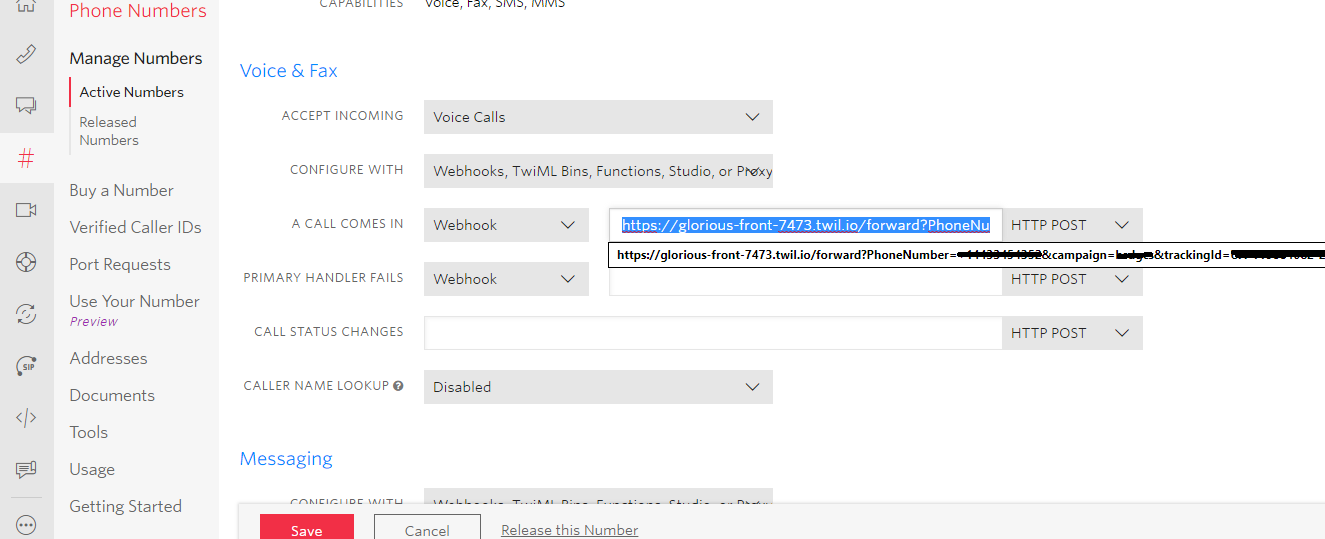
Go back to Runtime ->Go to Twilio function and Create Another blank function similar to the one that you created for call forward function. Give the function name as Call Action
and path as /call_action
and save the function.
Coding Call Forwarding
Go back to the call forward function. This function is used to forward calls that are received by your twilio campaign numbers to the actual phone number of your business through Webhook(HTTP post). The call forward function accepts three parameters i.e., campaign, actual phone number and tracking Id of your google analytics account. Paste the following code in the box provided and save the function.
Here is the code for Call Forward function:
The above function is used to forward calls to actual phone number. When the call ends, call action function is called by passing parameters such as phonenumber, campaign and tracking ID through action URL.
Linking the system with Google Analytics
Go back to the call action function and paste the following code in the box provided and save the function. This function is used to get a call details and callers geo location and report to google analytics (by your tracking ID of your analytics account) through the Events API.
Here is the code for updating events API:
In the above code you can see,
sendAnalyticsEvent
function, which is used to report events(call duration, call status and callers location) to analytics through events api.GeoCode
function, which is used to find callers location. It takes country code from the callers number. The country code varies from one to four digits. The country code is mapped to a Google Map’s geocode for placement in the map. There are four separate mapping tables, each corresponding to the number of digits in area code. First, the country code is assumed to be 4 digits and the mapping is obtained from theget4DigitGeoCodeMapping
function. If no map is obtained, it is assumed to be 3 digits and the mapping is obtained fromget3DigitGeoCodeMapping
function. This process is continued until theget1DigitGeoCodeMapping
. If no map is found, it maps to Gambia country by default.getDigitGeoCodeMapping
functions are used to map country code to google geocode.getAreaCodetoGeoCodeMapping
function is used to map area code of US and Canada to geo-caller Id.
Note that calls are tagged at the country level. However, calls from US and Canada are further resolved to the state or province in Google Analytics. Calls from any unknown country are marked as Gambia.
After completing these steps, you can test it by calling to the Twilio number you have setup. The call will be forwarded to the actual number.
Viewing Results in Google Analytics
The results can be viewed in the Google Analytics account you created in step 1. Login to Google Analytics, go to real-time, select events there you can see the live results of call tracking as given in the below figure:
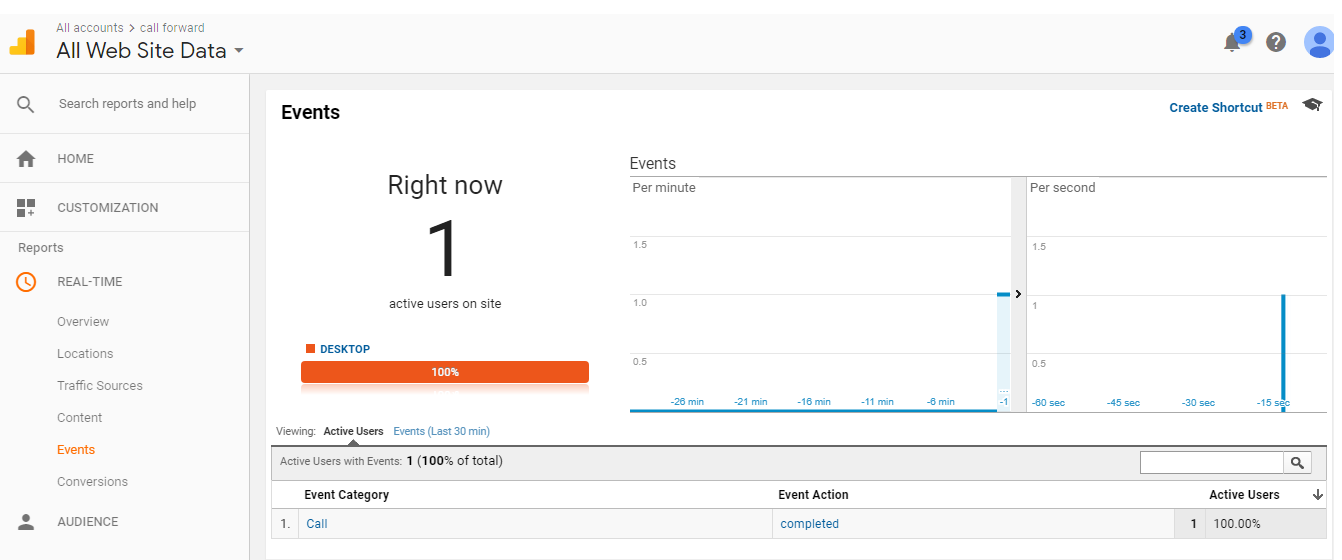
To see the location of the caller. Go to Audience -> geo -> location.
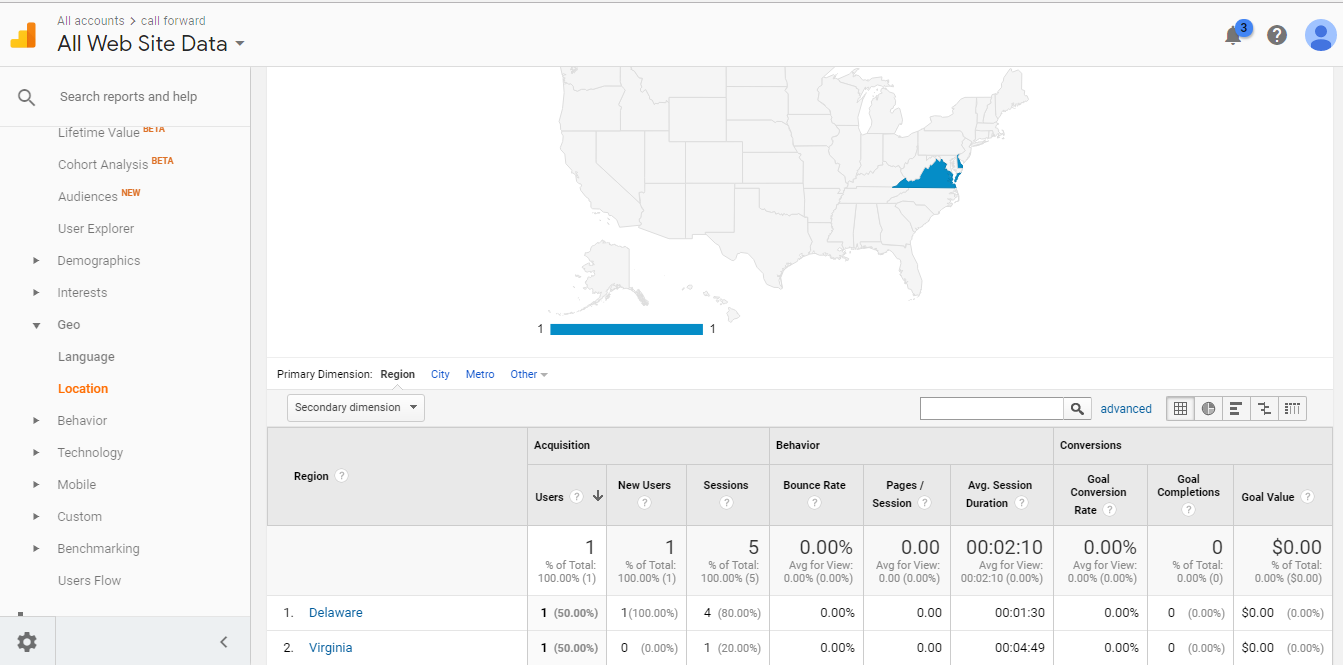
To see the accumulated results of the call tracking, Go to Behaviour ->Events->Overview.
This Overview represents the call tracking data over a month. The present day details are noted in real-time->events. You can select the range of the days at the right top of your window.
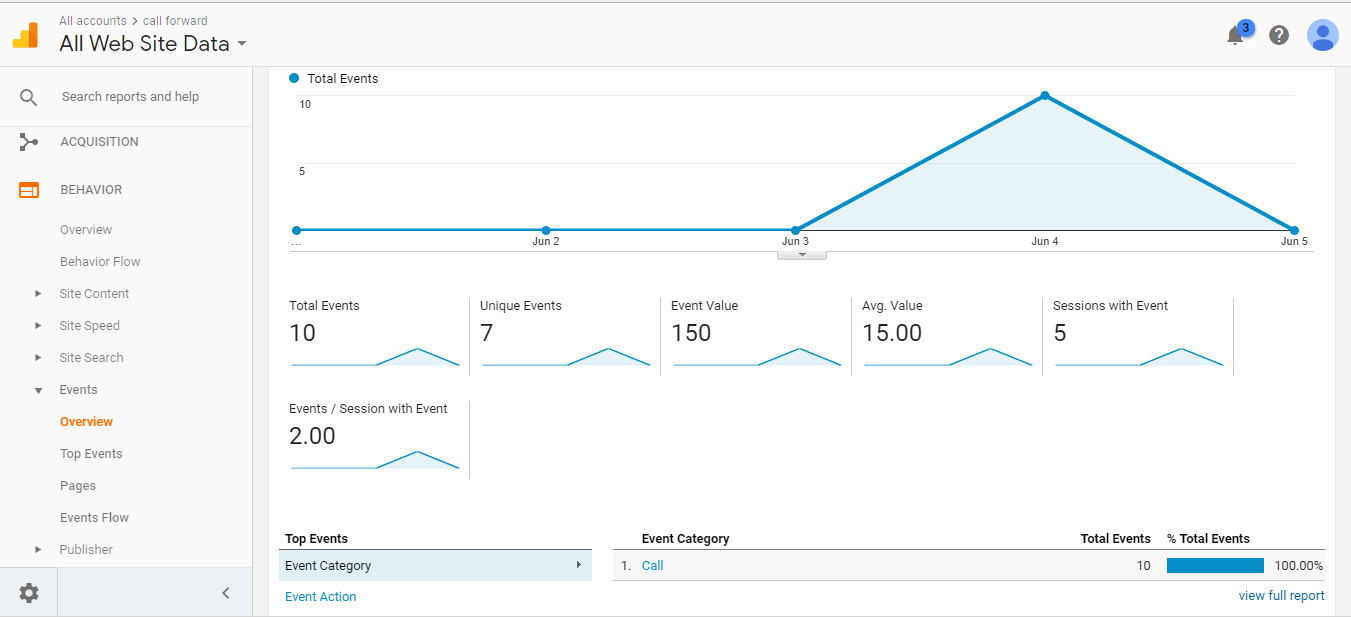
In the above events graph,
1. Total events : Total number of calls.
2. Unique events : Total number of unique calls.
3. Event value : total call duration for all calls.
4. Average value : Average call duration.
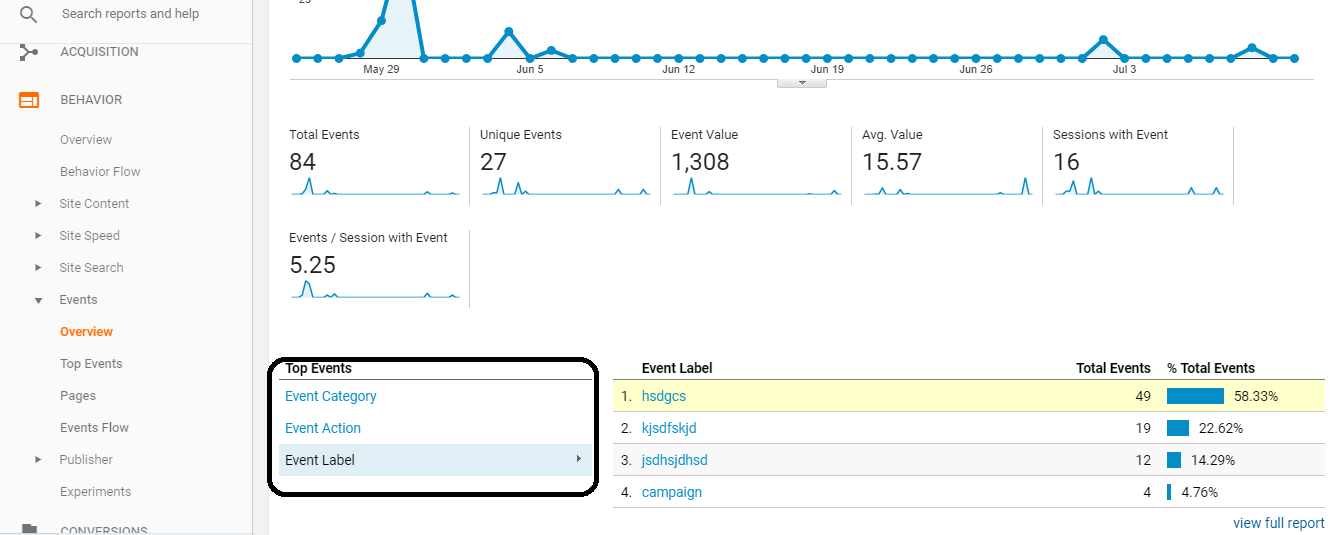
To see the results for different campaign. Click “Event Label” to choose campaign. To know Calls Status. Click “Event action”.
Keeping Track
Call tracking function allows you to tie a phone number by a campaign. Here, a phone number is programmed to forward calls to the actual phone number. The country code of the caller is mapped to a geo-location, which is used in Google Analytics. Calls from the US and Canada are further resolved to the state or province respectively. Finally, this function reports to the Google Analytics by tracking ID of your account created. In analytics you get a clear picture of the impact of your marketing efforts on lead generation and sales. You can also make your campaigns more effective and cost efficient based on the results.
There are several possibilities to expand this further. In this example, only US and Canadian numbers are mapped to State. It is possible to expand this for any other countries by area code mapping. Currently calls are not recorded. It is possible to record calls as well. It is also possible to implement IVR, and track the selected IVR option.
Happy call tracking!
This article a guest post from Nethram.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.