Using C# and Azure to Build a SMS Representative Directory
Time to read: 7 minutes
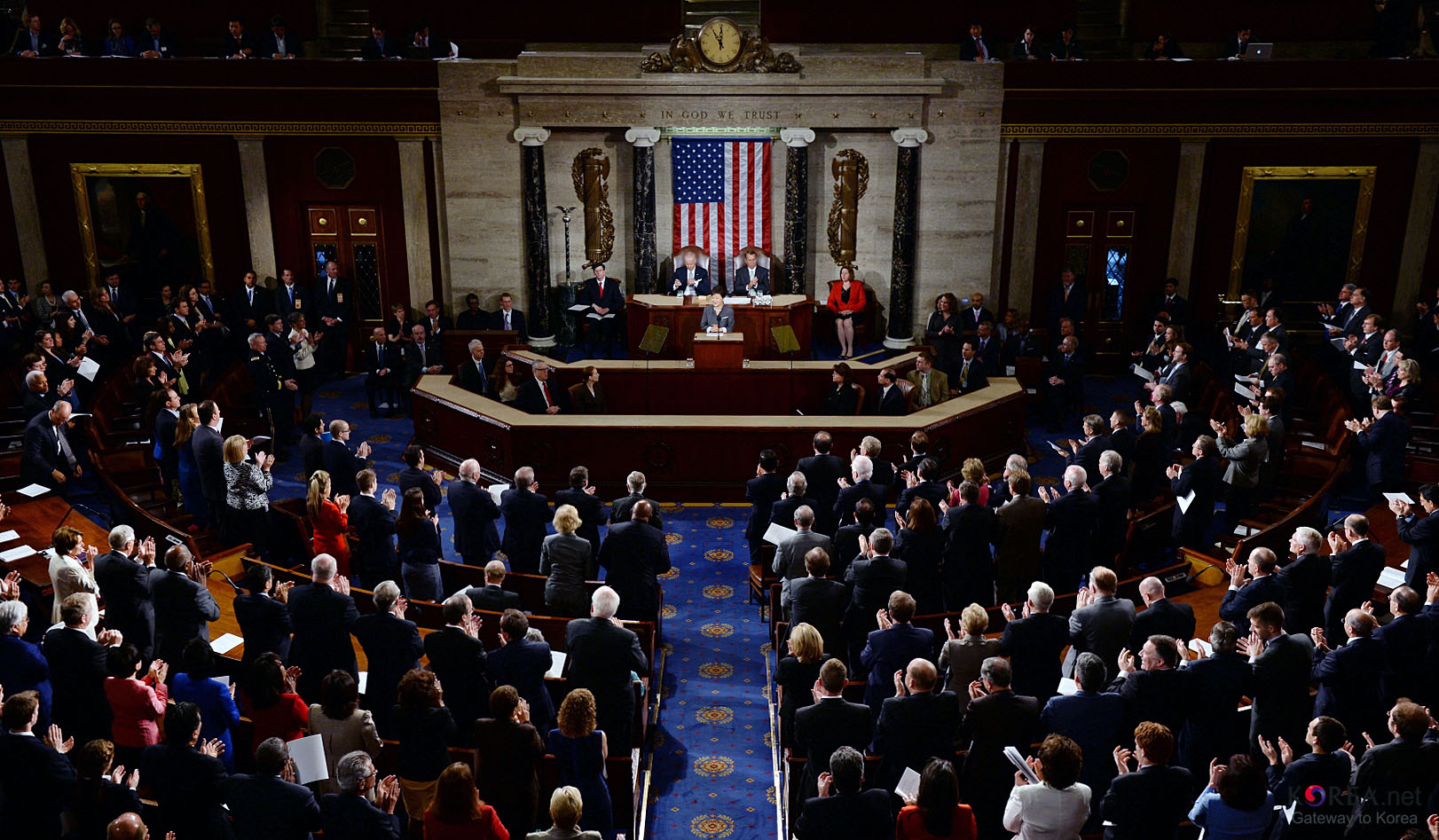
Earlier this month, you heard about Ian Webster, who built an app to call your federal representatives. Communication can be a powerful force for change. Today we’ll learn how to empower others by building an app similar to Ian’s with SMS.
Using C#, ASP.NET, and Azure, we will build a messaging application that responds to a ZIP code with a list of federal representatives for that area:
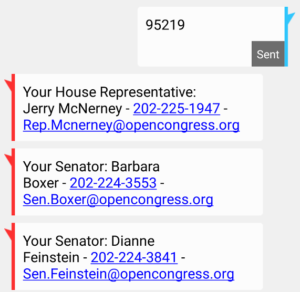
Tools
The tools we’ll be using are the staples of the Microsoft stack along with the Twilio API and Sunlight Foundation’s Congress API. Here’s what you’ll need to get right now:
- A Twilio Account
- Visual Studio 2015 – Free Community Edition is fine
- An Azure Account
- An API Key for Sunlight Foundation congress API
We’ll also be using ASP.NET MVC 5 and the Twilio C# Helper Library.
Getting Started
First, create a new, empty ASP.NET MVC project that can handle incoming SMS text messages from Twilio. For step-by-step instructions, please see our documentation on how to setup a new project.
Name your project TwilioCongressBot.Web and the solution TwilioCongressBot . Once you see that your Azure Web App is ready, you can proceed.
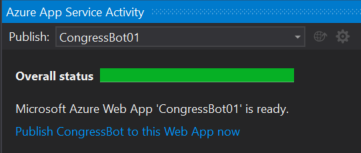
NuGet Packages
To finish off the framing for our project, we need to install a couple dependencies via the NuGet Package Manager. In the menu, choose Tools > NuGet Package Manager > Package Manager Console. Then, run the following commands:
Creating a Legislator Controller
When Twilio receives a SMS message at a phone number that you own it will call a special URL called a Webhook. Let’s create an empty MVC 5 Controller and name it LegislatorController . Our previously mentioned walkthrough has details on creating a new controller.
Update the namespaces that you import into your controller to be as follows:
And then make sure your LegislatorController inherits from TwilioController :
Using the Congress API
If you don’t have your API key yet, head over to the Sunlight Foundation and register for a free one. Their website also contains documentation for the API.
Storing the API Key
We need somewhere to keep track of this API key, and the typical place for doing that in an ASP.NET app is the Web.config file, specifically the appSettings section:
Add the highlighted line to your web.config file and use the API key you obtained during the registration process.
Calling the API
We will be calling the /legislators/locate endpoint, passing it a ZIP code as well as our API key. The template for the URL we’ll be calling can be assigned to a private field on our controller like so:
We’ll use C#’s String.Format() function to replace {0} with the ZIP code and {1} with our API key from the web.config file. Once we’ve built the URL, we can use RestSharp (already included as a dependency of the Twilio helper library) to make the call and Json.Net to parse the result:
The CongressApiResult class defines all of the expected properties of the API result. We need to create a source file to hold this class and dependent classes. Place it in the “Models” folder of our solution.
Pro-tip: This class was auto-generated by Visual Studio by copying the expected JSON result (obtained here) from the congress API and using: Edit… Paste Special… Paste JSON as Classes. The generated Result class was then manually renamed to Legislator:
Determining a ZIP Code
As you can see here, Twilio will pass us a Body parameter containing the contents of whatever was sent to us as a text message. It also may send us a FromZip parameter if it can identify geographic data based on the source phone number (not geolocation). For example if the text message was sent from the 415 area code, Twilio can tell you that area code is located in San Francisco, CA.
Let’s check the Body first, to see if the user specified a ZIP code and fallback to the FromZip:
Notice we’re adding a help message if we fallback to the FromZip letting them know they may need to text us their specific ZIP code if the one associated with their phone number isn’t where they reside.
Putting it Together
Now we just need our controller action to use the code we’ve written to get the ZIP code, pass it to the congress API, and format the results:
There’s two things in the formatting code above we haven’t covered yet. First, we create the following dictionary as a private field:
This helps us translate the “chamber” property from the API to a more friendly text description. Also, we have a simple helper function to concatenate the first, middle, and last name of the representative:
You can find the full source code for the controller and model on GitHub.
Testing and Debugging Locally
Run your application from within Visual Studio to view your application in the browser. In the case of an empty ASP.NET project, we don’t have a default home page for our app:
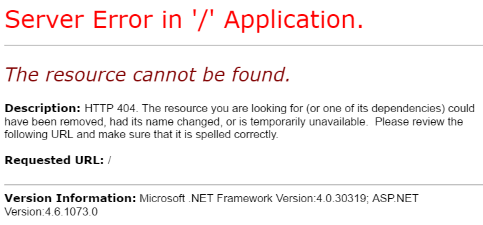
Instead of using the browser to test, open a new PowerShell window and run the following command:
Replace the XXXXX with the random port number that Visual Studio assigned to your web app. You can see this in the browser URL when Visual Studio first launched your app.
This will return a PowerShell object with the response from your controller:
You should be able to see the raw XML response we are returning in the Content property. Pro-tip: you can see just the Content property with a single command:
Deploying and Configuring the App
Publish to Azure
To publish your app to Azure, find the “Azure App Service Activity” tab in Visual Studio and click the Publish button:
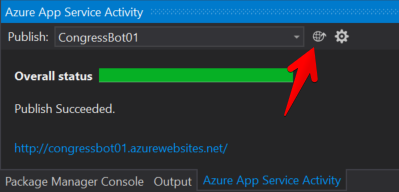
Once the publish is complete, you can test your app from PowerShell again:
Replace congressbot01 with the name you selected for your Azure Web App.
Assuming that works, we can move on to attaching your Webhook to a phone number in Twilio.
Configure a Twilio Number
If you haven’t already purchased a Twilio phone number, do so now. Once that is done, you can configure the Messaging Webhook as follows:
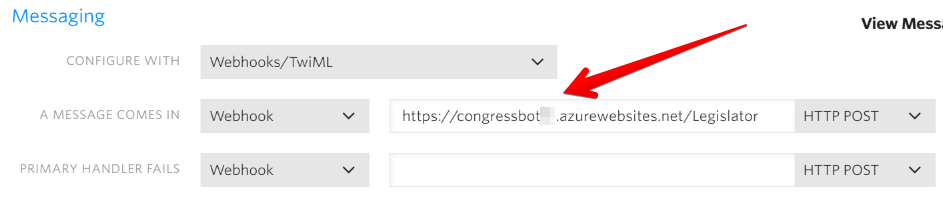
Use the same URL for your Azure Web App that you tested in PowerShell earlier. Be sure to click the SAVE button to save your configuration for the phone number.
Taking the Final Test Drive
With your Twilio phone number confirmed, you can simply send a text to test it out:
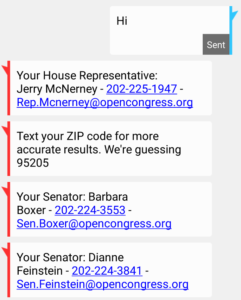
Wrapping Up
You can download the entire solution from GitHub. If you want to publish this completed app to Azure, right-click on the CongressBot web app in the Solution Explorer and choose “Publish.”
Now all you need to do is give your Twilio phone number to your colleagues, friends, family, etc. and allow their voices to be heard! Or, have them text (646) 8USA-REP (+1-646-887-2737), which is powered by this app.
I’m David Prothero and I work on the Twilio Developer Education team. If you have any questions or feedback on this hack, let me know in the comments below, hit me up on Twitter at @dprothero or shoot me an email at dprothero at twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.