Danger JS: Helping Paste Focus on Paste
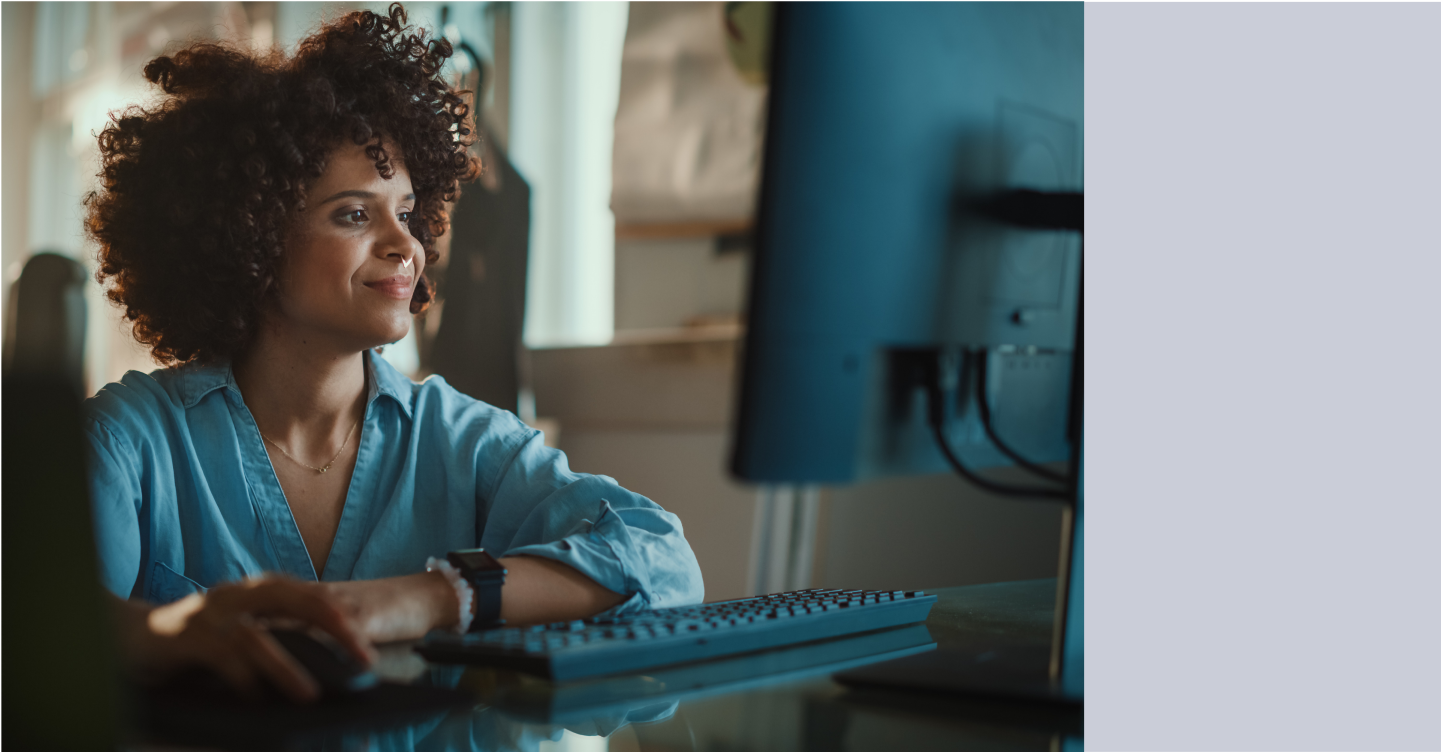
Time to read: 3 minutes
There’s always a checklist. Whether you’re writing code or reviewing it, every repo has a checklist of what should be included in a PR – and sometimes what shouldn’t. But what happens when that list becomes unwieldy as a project grows? Automating important parts of that checklist is vital to efficiency and consistency. That’s where Danger JS comes in.
Danger JS allows you to create custom rules about what your team’s PRs should look like. Its flexibility makes replacing many types of error-prone tasks possible. In the Danger team’s words:
You can:
- Enforce CHANGELOGs
- Enforce links to Trello/JIRA in PR/MR bodies
- Enforce using descriptive labels
- Look out for common anti-patterns
- Highlight interesting build artifacts
- Give warnings when specific files change
Danger provides the glue to let you build out the rules specific to your team's culture, offering useful metadata and a comprehensive plugin system to share common issues.
Twilio’s Paste Design System helps us build accessible, cohesive, and high-quality customer experiences. However, as our design system grows, so does the possibility of stray errors. So how does Paste take advantage of the power of Danger? Although we’ve written a few rules, our first – and most powerful – has been a rule to enforce accurate versioning for our package updates. Let me show you how the team works with Danger to keep our packages versioned accurately with the least amount of effort!
Our Danger JS rules, explained
Enforce versioning (missing Changesets check)
For our versioning and changelog generating tool, Changesets, to work best, every PR that modifies one of our public packages should include a Changeset file. We got tired of telling each other “Oy! This needs a Changeset” in reviews, so now we let Danger do it for us!
Our process is (if you prefer, you can follow along in the source here):
1. Compare the list of changes in the PR to Paste’s public packages (the ones that need versioning)
Using our package crawling utilities and a danger
object typed to Danger’s DangerDSLType, we can determine which of our packages need a Changeset associated with them. We also check to see if any Changeset files have been added or changed.
2. If changes come from public packages, does any Changeset file include it?
We wrote a getMissingPackagesFromChangesets
utility that uses the two pertinent pieces of information, our modified Changeset files and our modified, relevant packages, and returns an array of packages that have gone rogue (have been changed but do not have an associated Changeset file).
3. If we have a rogue package, our Danger check will fail
If anything is returned from our getMissingPackagesFromChangesets
utility, Danger will fail
and output the specific reason for failing into a HTML table. To be as user friendly as possible, our plugin includes the package name in this message, and an idea on how to fix it.
That’s it! Since we have implemented this Danger rule, we haven't had issues with missing Changeset files, or having to bug anyone to add one. It also inspired us to write more, like the next one about external dependency versioning.
Pin external dependencies
We use external dependencies in our repo, but to protect our work from unexpected changes, we pin them. How do we make a rule that enforces this?
Our process (follow along in the source here):
1. Find the package.json files that need inspecting
Again, Danger lets us know which files were modified and created in the last commit. We run through this list and filter for package.json
files.
2. Search package.json files for unpinned external dependencies
After excluding Paste dependencies, we look for any starting with the ^
character, which indicates that this version accepts a range of versions, which we don’t want.
3. If we have an unpinned external package dependency, our Danger check will fail
If anything is returned from our function and is an unpinned external dependency, Danger will fail
and indicate both the problem and its solution. All set!
The Danger effect
As Twilio’s design systems team has grown, we’ve benefitted immensely from Danger JS automatically alerting us to simple but impactful errors, such as missing versioning information and unpinned external dependencies. Since we have implemented these Danger rules, they’ve allowed us to focus on solving design system problems, as opposed to human memory hiccups. And we keep finding new places to create useful rules all the time! Check out our .danger
folder to see them all 😎.
Like how we’ve used our tools? Learn more about our design system, tooling, and tracking from our post on insights and metrics!
Glorilí Alejandro is a UX Engineer for Twilio’s Paste design system. She is passionate about creating a more human centered world through thoughtful design and craftsmanship. She also stress bakes, and is always looking for her next, great dessert. Reach her at galejandro [at] twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.