Detect browser support for WebAuthn
Time to read: 2 minutes
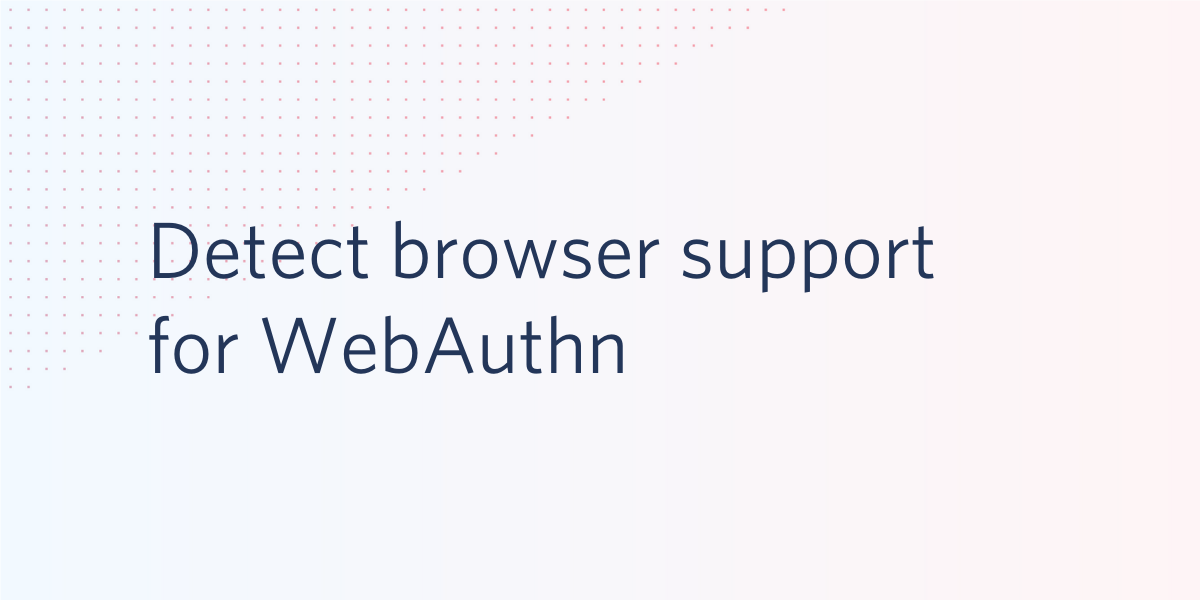
Browser support for WebAuthn is growing rapidly. As of writing, 87.39% of internet users should have support. You can check for WebAuthn support by checking for PublicKeyCredential
in JavaScript:
What is WebAuthn?
WebAuthn (short for Web Authentication) is a relatively new browser API for strong, scoped, passwordless authentication. Instead of a password, an authenticator uses public key cryptography to create a key pair (known as a credential) for a website. It's part of the FIDO2 specification written bythe W3C and the FIDO Alliance, a group of security researchers interested in abolishing passwords.
WebAuthn is an incredibly promising API because it reduces the web's reliance on passwords. Because credentials are tied to a website, it also prevents phishing attacks. Once a credential is generated, only the public key is sent to a website's servers. This means that databases are less vulnerable to attacks since public keys are useless without the corresponding private key.
How to detect Platform Authenticator support
FIDO2 and WebAuthn support two types of authenticators, platform authenticators and roaming authenticators.
Platform Authenticators are authentication mechanisms built into devices. This could include things like Windows Hello, Apple's Touch ID or Face ID.
Roaming Authenticators are separate authentication hardware keys like Yubikeys or Google's Titan Keys.
Because roaming authenticators are expensive and require a special device, I expect platform authenticators to be the main way we see WebAuthn adoption in consumer identity.
You can detect if a platform authenticator is available with the PublicKeyCredential.isUserVerifyingPlatformAuthenticatorAvailable() method. Run the following in a browser console:
Getting started with WebAuthn
I recommend checking out https://webauthn.guide/ as a starting point for learning more. If you want to play around with some more code, Google has a step by step tutorial on how to get started.
What's next for WebAuthn?
Even a couple years after release adoption is still pretty low. While we wait for adoption to pick up while more people get access to platform authenticators, you should support additional factors like Push, TOTP, SMS and email. These methods can also be used for account recovery if the user only registers one platform or roaming authenticator.
Check out Twilio's Verify API for a wide range of authentication channels.
Are you building WebAuthn support? Let me know how it goes! I can't wait to see what you build.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.