Detect Robocalls with Twilio Lookup and the Nomorobo Spam Score Add-on
Time to read: 2 minutes
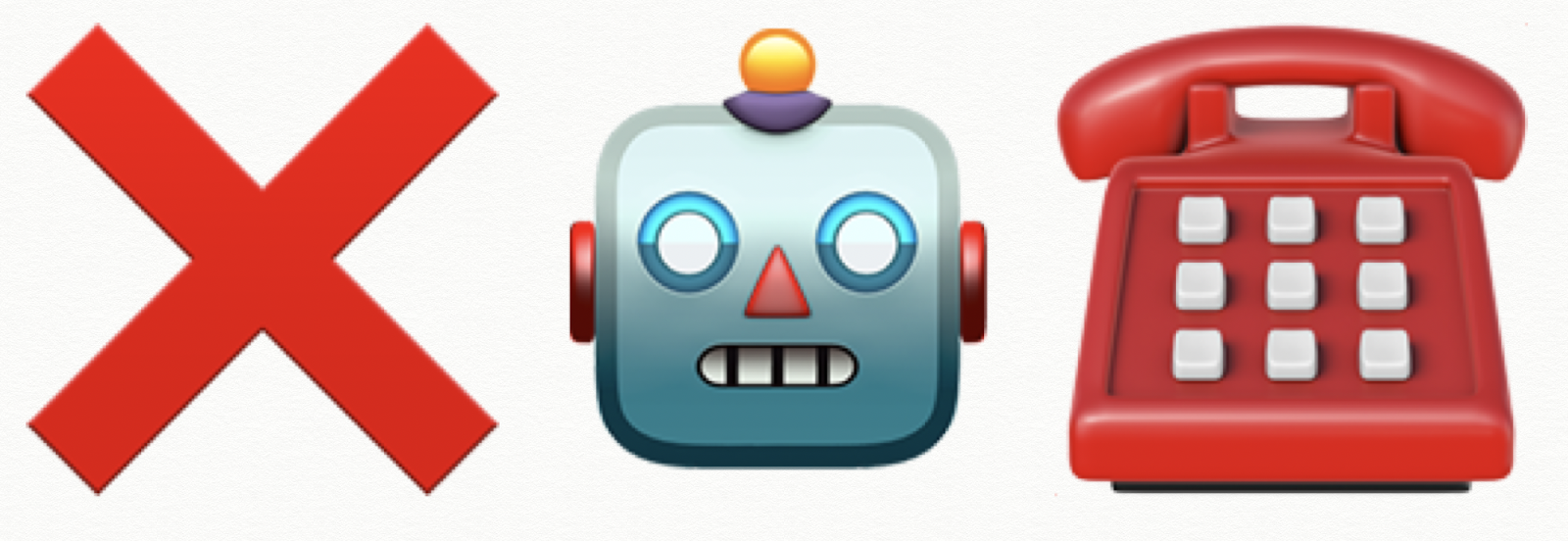
Twilio's CEO Jeff Lawson recently wrote about the history of robocalls and what we're doing to eliminate them. Until that happens, we can build a tool that will help us identify a robocall with a bit of Python, the Twilio Lookup API, and the Nomorobo Spam Score Add-on.
Set Up
In order to code along with this post you'll want to start with the following:
Head to the Twilio Console and install the Nomorobo add-on. Look for the yellow logo and click through to "Install".
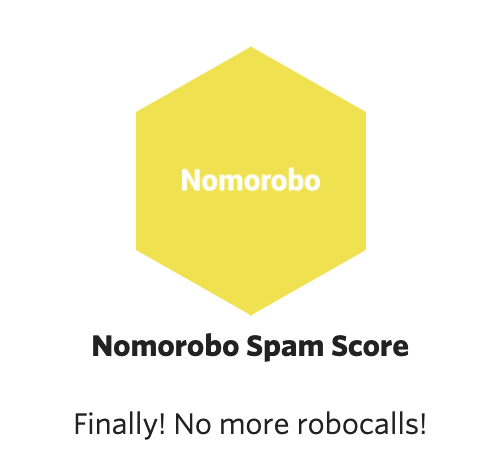
Leave the name as nomorobo_spamscore
and "Save" the Add-On.
Create a new file called nomorobo.py
and add the following code.
Replace the placeholder credential values
Swap the placeholder values for your_account_sid
and your_auth_token
with your personal Twilio credentials. Go to https://www.twilio.com/console and log in. On this page, you’ll find your unique Account SID
and Auth Token
, which you’ll need any time you use the Twilio API like this. You can reveal your auth token by clicking on the 'view' link:

You should see output like this. A score
of 1 means this is most likely a robocall.
If you get a response that says AddOn not found
double check you have installed the Nomorobo AddOn in the Console.
How to tell if a phone number is a Robocaller
This code gave us some useful information, but let's create a reusable function so our application can be used as a handy script. In nomorobo.py
, add the following function:
The possible values for score
are 0 and 1. Next, replace the original phone_number = …
and print
statements in nomorobo.py
with the following:
Make sure the function is_robocaller
is defined before this code.
This includes a bit of error handling to make sure we provide a phone number when we run the script. Now our program can take a phone number as an argument and return its line type!
Try running it again with a new phone number, maybe something from your missed calls or your personal cell phone. Don't forget to put the number in E.164 format.
🎉Ta da! Now we have a handy script we can use to identify robocalls.
What's next for using Lookup?
Maybe you want to filter calls in your call center or identify the line type (mobile vs. landline) of a phone number. With Lookup you can also prompt customers to add SMS (or voice!) based 2FA, clean up your database, or segment your users.
You may also be interested in:
- Identifying a Phone Number's Line Type with Twilio Lookup and Python
- Build Simple SMS Phone Verification with Twilio Verify and Python
- Identify Unknown Phone Numbers with Python 3, AWS Lambda, Twilio Lookup and SMS
Have you built something that takes advantage of Twilio Lookup? Let me know in the comments or on Twitter @kelleyrobinson.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.