How to Send a Picture on WhatsApp Using Twilio and JavaScript
Time to read: 3 minutes
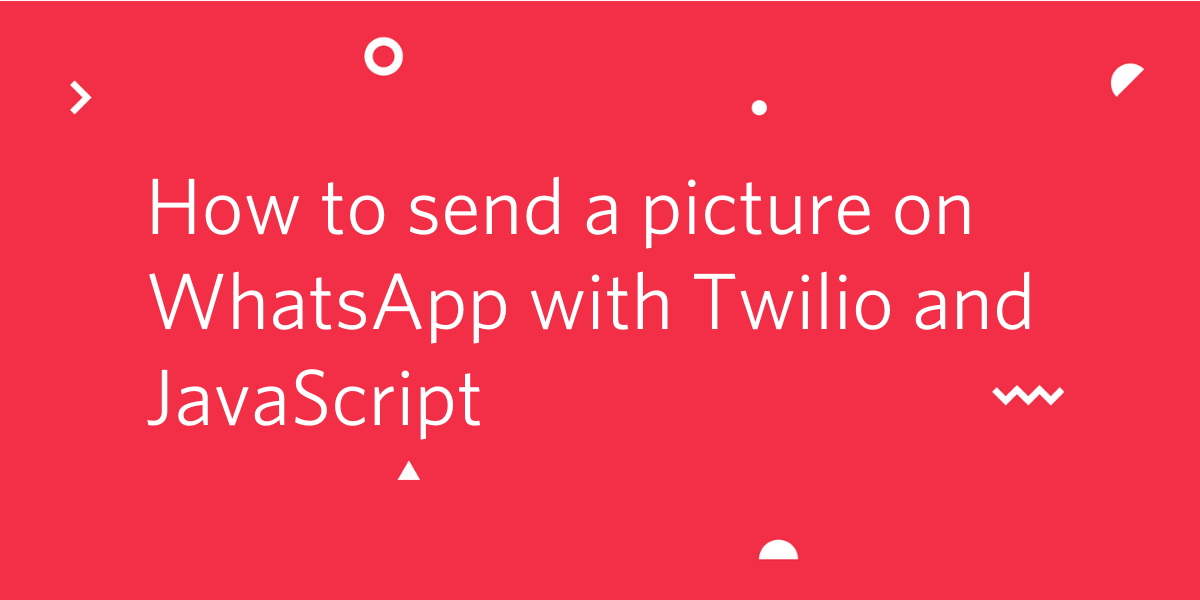
The WhatsApp Business API from Twilio is a powerful, yet easy to use service that allows you to communicate with your users on the popular messaging app.
In this article, we’ll walk you through how you can develop a functional JavaScript program to send an image to a user through WhatsApp.
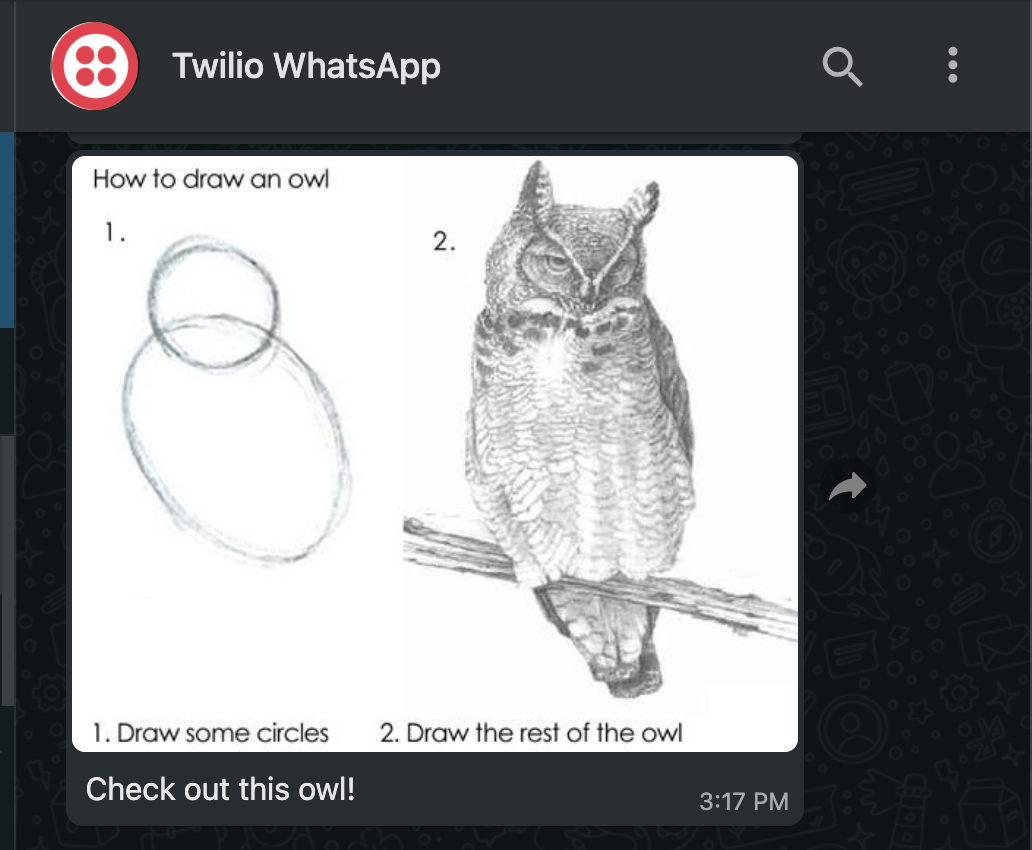
Prerequisites
To get started with this tutorial, you’ll need the following:
- Node.js installed on your machine.
- A free Twilio account (sign up with this link and get $10 in free credit when you upgrade your account).
- A smartphone with an active WhatsApp account, to test the project.
The Twilio WhatsApp sandbox
Twilio provides a WhatsApp sandbox, where you can easily develop and test your application. Once your application is complete you can request production access for your Twilio phone number, which requires approval by WhatsApp.
In this section you are going to connect your smartphone to the sandbox. From your Twilio Console, select Messaging, then select Try it Out on the sidebar. Open the WhatsApp section. The WhatsApp sandbox page will show you the sandbox number assigned to your account, and a join code.
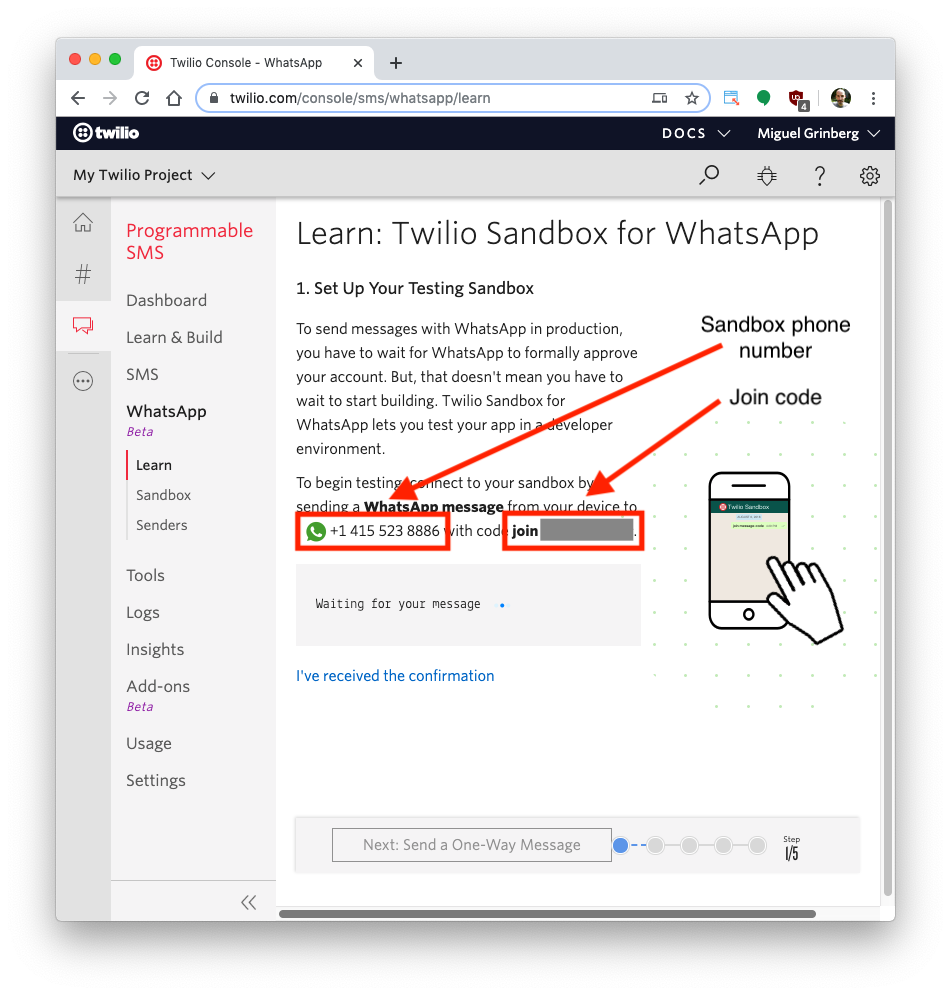
To enable the WhatsApp sandbox for your smartphone send a WhatsApp message with the given code to the number assigned to your account. The code is going to begin with the word "join", followed by a randomly generated two-word phrase.
Shortly after you send the message you should receive a reply from Twilio indicating that your mobile number is connected to the sandbox and can start sending and receiving messages.
If you intend to test your application with additional smartphones, then you must repeat the sandbox registration process with each of them.
Set up your development environment
In this section you are going to set up a brand new JavaScript project. Open your terminal and run the following commands:
The next step is to initialize a new Node.js project by running the following command:
After initializing your new Node.js app, you can install the two required dependencies: the Twilio Node Helper Library and dotenv
, to load your environment variables:
Create two new files, index.js and .env, inside your whatsapp-pic directory:
Open the .env file and add two environment variables to store your Twilio Account SID and Twilio Auth Token. Leave the values for each variable blank for the moment, as you’ll collect these credentials in a moment.
Head to your main dashboard within the Twilio Console and find your Account SID and Auth Token.
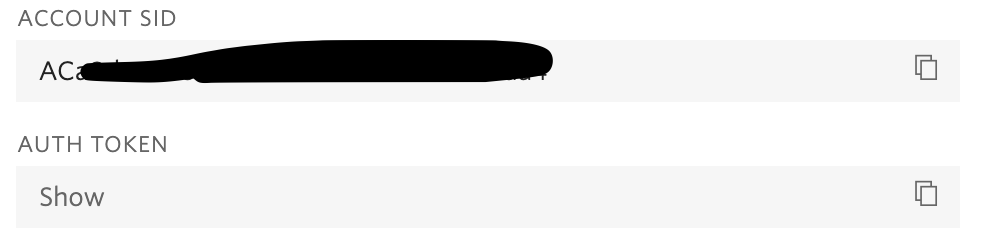
Copy and paste these values into the .env file you just created as the values for the variables TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
respectively. You can save and close this file now.
Send a picture message with Twilio
Open your new index.js file and copy and paste the following code, taking care to replace the placeholders with your WhatsApp sandbox number and your personal WhatsApp number using the E.164 format, on lines 5 and 8 respectively.
This code creates a new Twilio client using the Twilio credentials stored in your .env file.
It then creates a new message
instance of the Message
resource as provided by the Twilio Messaging API for WhatsApp in order to send a WhatsApp message to your personal phone number from the Twilio sandbox number.
The message
instance also allows you to include the body
of a message as well as a URL of an image you want to send. Feel free to change it to another image URL as you wish.
After the message is sent, the message SID is printed on your terminal for your reference.
To run this code, save your index.js file and head to your terminal. From your whatsapp-pic directory, run the following command:
In just a moment, you will see a ping from WhatsApp on your personal phone sent directly from your computer!
Let me know what else you’re building with WhatsApp, Twilio, and JavaScript on Twitter!
Ashley is a JavaScript Editor for the Twilio blog. To work with her and bring your technical stories to Twilio, find her at @ahl389 on Twitter. If you can’t find her there, she’s probably on a patio somewhere having a cup of coffee (or glass of wine, depending on the time).
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.