Make Spooky Automated Calls at Halloween With Twilio Voice and Go
Time to read: 4 minutes
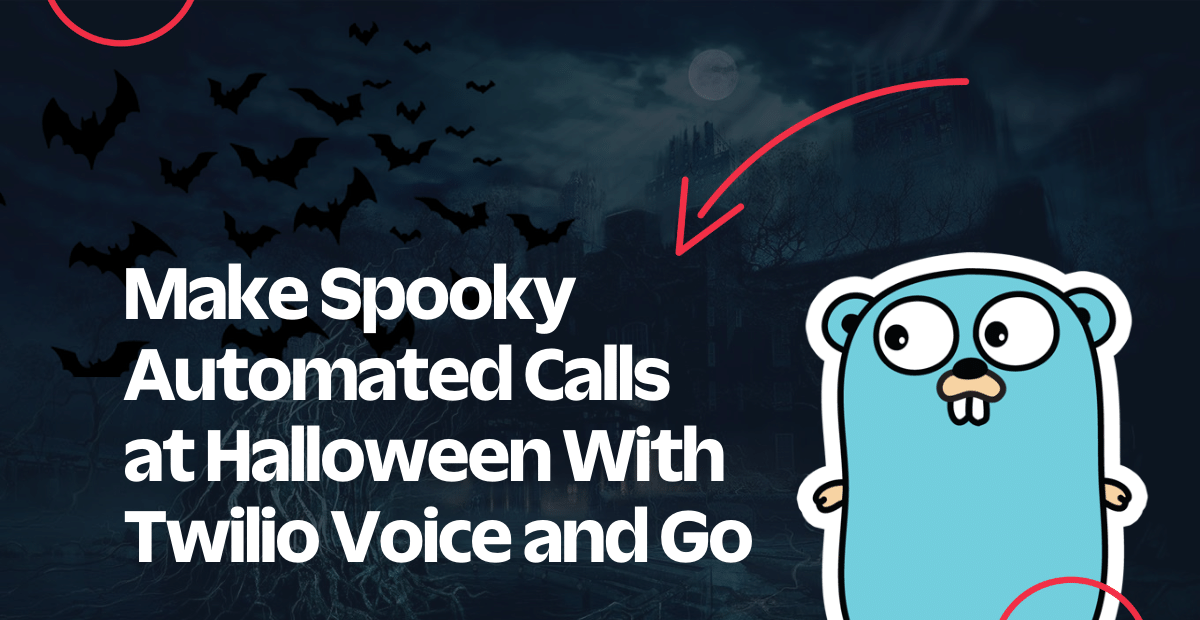
Make Spooky Automated Calls at Halloween With Twilio Voice and Go
Automation is essential to improving the efficiency of any process. By harnessing technology solutions for monotonous tasks, human resources are freed up for use in more intricate aspects of the process. An excellent example of this is automating notifications and reminders using phone calls.
In this article, I will show how to build a Go web application which, when provided with a phone number, will make an automated phone call to the provided phone number and play one of three pre-recorded audio files. But keeping with the spirit of the season the caller will be greeted with a spooky Halloween message.
Prerequisites
To follow this tutorial, you need the following:
- A free Twilio account
- A Twilio phone number
- A phone that can make and receive phone calls
- A basic understanding of Go
- Go 1.19
- Git
Set up the project
The first thing you need to do is to create a project directory and change into it. To do that, run the following commands.
Next, create a new Go module by running the following command
Then, add the project dependencies:
- GoDotEnv: This will help with managing environment variables
- Twilio Go Helper Library: This simplifies interacting with the Twilio API
To install them, run the two commands below.
Next, create a new file named .env to store the environment variables which the application requires. Then, in the new file paste the following code.
After that, create a local version of the .env file using the following command.
Make your Twilio credentials available to the application
The next thing you need to do is to retrieve your Twilio Auth Token, Account SID, and phone number. To do that:
- Login to the Twilio Console
- Copy the details from the "Account Info" panel
- In .env.local, replace
<<TWILIO_AUTH_TOKEN>>
,<<TWILIO_AUTH_TOKEN>>
, and<<TWILIO_PHONE_NUMBER>>
respectively, with the copied details
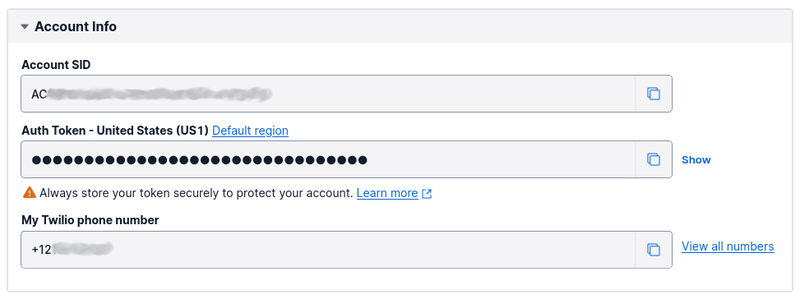
Add the ability to make a phone call
Create a new directory named helper in the application's top-level directory. Then, in that directory create a file named caller.go. In that new file, add the following code.
After specifying the package name and imports, the Call()
function is declared. This function is used throughout the application whenever a phone call is to be initiated. Using the phone number provided as an argument, a request is made to the Twilio API passing the following parameters:
- The recipient’s phone number
- The Twilio phone number to call from, which is retrieved from the environment variable specified earlier
- The TwiML instruction (generated in the
playTwiml()
function) for Twilio. The TwiML instruction uses the<Play>
verb to play an audio file back to the caller. Twilio retrieves the file from one of the three bit.ly URLs provided in therandomAudioClip()
function
If the call was successful, a response will be returned. Otherwise, an error will be returned with the corresponding error message.
Model the phone number
Next, create a struct to model a phone number. This struct will simplify parsing of the JSON request. Additionally, it will hold the logic for validating the provided phone number before a request to the Twilio API can be made.
Create a new directory named model in the top-level directory of the project, and in that new directory create a file named PhoneNumber.go. Then, paste the following code into the new file.
This struct has a single field named Value
which corresponds to the phone number.
The Validate()
function takes a PhoneNumber
struct and matches the value against the regular expression for a valid E.164 phone number. In the event that the provided phone number is invalid, an error is returned with an appropriate error message.
Create the template for the application's default route
The application's default route will render an HTML page with a form which contains two elements:
- An input field for the recipient's phone number
- A submit button
To build it, first create a new directory named static in the application's top-level directory. Then, in the new directory create a new file named index.html and paste the following code into the new file.
Bootstrap is used to style the template, and several Javascript functions validate the submitted form and handle the submission process for valid input.
When the submit button is clicked, the provided phone number is sent to the backend encoded as a JSON string. Following that, the response received from the request is rendered in an alert above the form.
Create the main function
All that is left is a main()
function to serve as the entry point for the application to serve the index file and handle incoming requests.
In the application's top-level directory, create a new file named main.go and paste the following code into it.
In the main()
function, four key things take place:
- The environment variables are loaded.
- The files in the static directory are served to handle requests to the index route.
- The
callHandler()
function is declared to handle POST requests to the/call
route. - The application is served on port 8000.
Test that the application works
First, start the application using the following command.
Then, view the application in your browser by navigating to http://localhost:8000/.
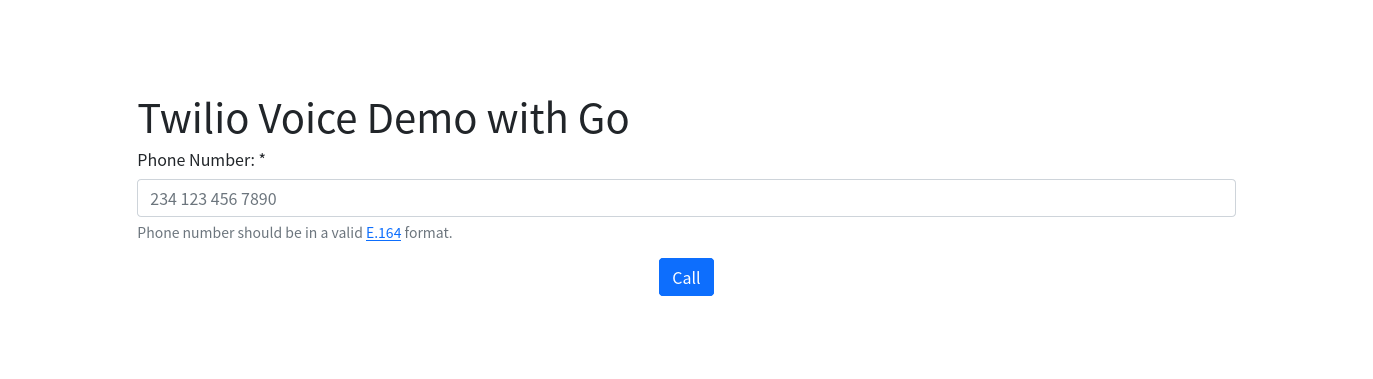
Fill in your best friend's number, click Call, and wait for Twilio to deliver some shivers down their spine.
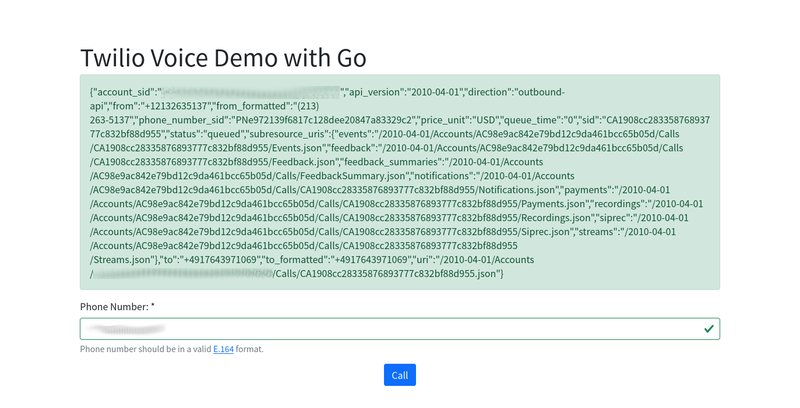
That's how to make automated calls with Twilio Voice and Go
You've now learned how to build a Go web application that can make an automated phone call to a friend's phone and play a pre-recorded audio file.
Want to have even more fun? Refactor the app to use the Twilio Voice API to record their reaction to the surprise call. That way, the moment will last forever.
If at any point you get stuck you can review the final codebase on Github. Until next time, make peace not war ✌🏾
Joseph Udonsak is a software engineer with a passion for solving challenges – be it building applications, or conquering new frontiers on Candy Crush. When he’s not staring at his screens, he enjoys a cold beer and laughs with his family and friends.
Image credits
- Spooky Scenes by Wendy is licensed under CC BY 2.0
- "Bats", castlevaniac.tumblr.com, https://castlevaniac.tumblr.com/post/614362124555255808/the-tepes-family-netflix-ayami-kojima-official
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.