Open Sourcing BankersBox
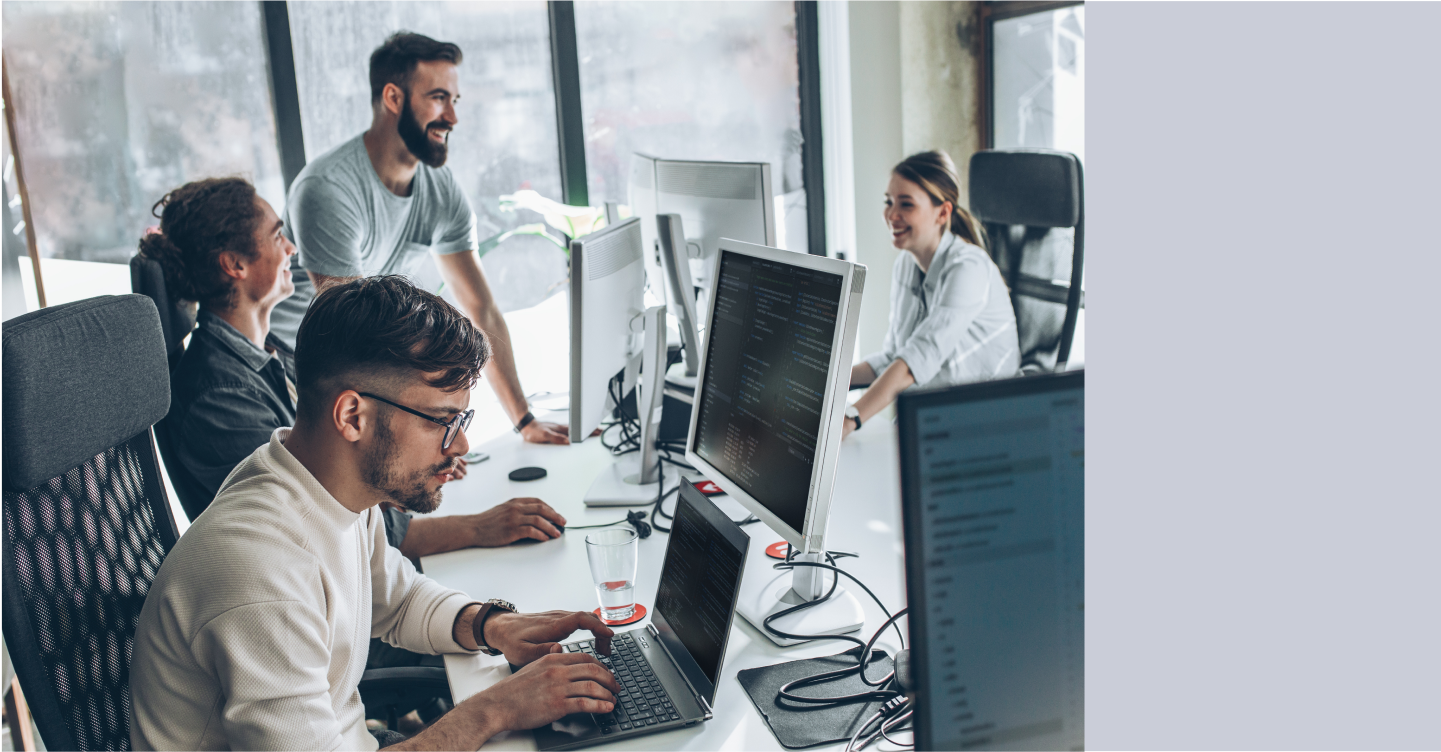
Time to read: 2 minutes
At Twilio, we like to play with new and fun technologies. One technology we have been exploring recently is client-side storage in web applications. There are a few available options, but the one that has gained the most adoption in modern browsers is javascript's localStorage
.
At its core, localStorage is a simple key-value store which uses strings as keys and can only store strings as values. Even if you try to store a non-string object into localStorage, it will be converted into a string before it is saved. This means that the developer has to worry about coercing the data back into the intended type before it is used in code again. Still, localStorage's ability to save data between sessions and browser restarts without relying on massive cookies makes it an appealing storage mechanism.
We have a lot of experience with Redis at Twilio, and we thought, "Wouldn't it be nice to have a Redis-like interface to client-side storage in javascript?"
Thus BankersBox was born.
BankersBox is a javascript library that injects Redis-like steroids into javascript data storage and uses localStorage as the default persistent data-store. There is a pluggable storage adapter system to allow other means of persisting the data stored in BankersBox.
Here is some example code:
var bb = new BankersBox(1);
bb.set("foo", "bar");
bb.get("foo"); // returns "bar"
bb.set("myobject", {greeting: "hello", thing: "world"});
bb.get("myobject"); // returns the object
bb.set("count", 10);
bb.incr("count"); // sets "count" to 11, returns 11
bb.incr("newcount"); // sets "newcount" to 1, returns 1
bb.lpush("mylist", "hello");
bb.lrange("mylist", 0, -1); // returns ["hello"]
bb.rpush("mylist", "world");
bb.lrange("mylist", 0, -1); // returns ["hello", "world"]
bb.sadd("myset", "apple");
bb.sadd("myset", "oragne");
bb.smembers("myset"); // returns ["apple", "orange"]
bb.sismember("myset", "apple"); // returns true
bb.sismember("myset", "lemon"); // returns false
bb.scard("myset"); // returns 2
Now you can store and retrieve any sort of native data type with localStorage!
The implementation is not complete (notably Hashes and Sorted Sets are not there yet), but we are actively working to add functionality. There is a full suite of unit tests written in CoffeeScript that run with the Expresso test framework for node.js.
Over the past few weeks, we have been developing several interal web apps to test and dogfood our own products. Using BankersBox has made working on these apps fast and fun.
We think that BankersBox can be useful for several different applications:
- Rapid prototyping of rich client-server applications
- Creation of useful one-page javascript applications.
- Mobile HTML5-powered apps.
- Many more we can't even think of yet.
BankersBox is now open source at http://github.com/twilio/BankersBox with an extensive README and an MIT license, so feel free to fork and contribute!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.