Optimizing JavaScript Application Performance with Web Workers
Time to read: 7 minutes
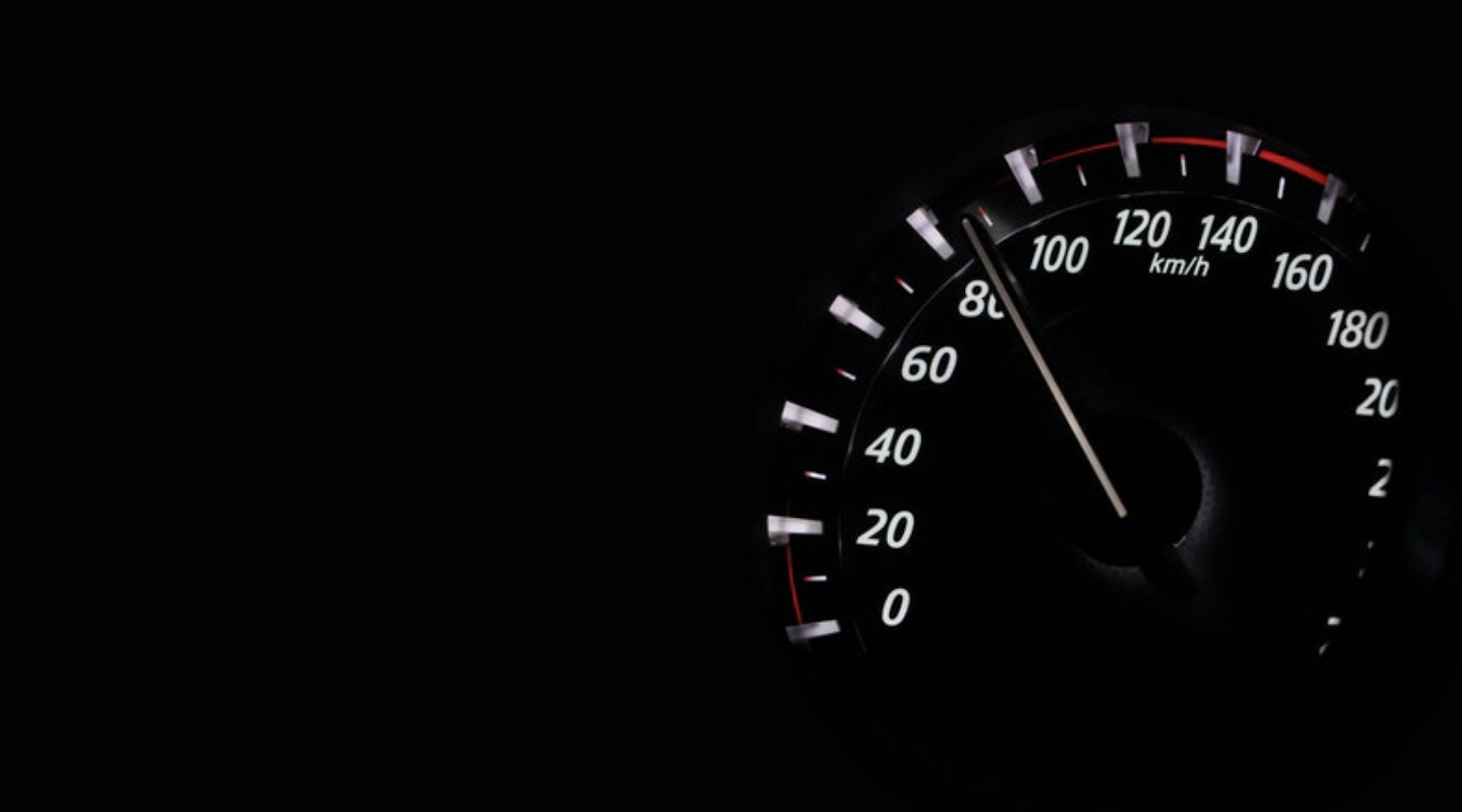
JavaScript has come a long way from being just a simple scripting language to being the de facto standard programming language for the Web. It's also being widely used to build server-side applications, mobile applications, desktop applications, and even databases.
Even though JavaScript is a great language for building complex and engaging software on the Web, it's possible performance inefficiencies can be introduced into these applications due to the nature of the JavaScript language.
In this post you will learn how to fix performance issues caused by long-running scripts in web applications by using web workers. A web worker is a JavaScript script that runs in the background, independently of user interface scripts executing from the same web page.
Prerequisites
To follow along, you will need a development server. If you don't already have one installed you can install the Web Server for Chrome extension for Google Chrome.
This post also assumes a basic knowledge of HTML, CSS, and JavaScript.
The case study project code for this post is available in a repository on GitHub.
The JavaScript Main Thread
JavaScript is single threaded, which means only one line of code can be executed at any given time. Tasks such as UI updates, user interactions, image transformation, and others that need to be performed are added to a task queue and executed one at a time by the browser's JavaScript engine.
This single-threaded pattern gives rise to a performance issue known as blocking. Blocking occurs when a particular task on the main execution thread takes a very long time to complete, thereby blocking every other task from being run. This problem manifests itself in web applications as a slow or sometimes frozen application, which is a huge turn-off for users.
Knowing that blocking poses a huge performance concern, JavaScript offers an API for running script operations in a background thread separate from the main execution. It is called the Web Workers API.
Web Workers
According to the Mozilla Developers Network (MDN) documentation: “Web Workers makes it possible to run a script operation in a background thread separate from the main execution thread of a web application. The advantage of this is that laborious processing can be performed in a separate thread, allowing the main (usually the UI) thread to run without being blocked/slowed down.”
Web Workers allow you spawn new threads and delegate work to these threads for efficient performance. This way, long running tasks which would normally block other tasks are passed off to a worker and the main thread can run without being blocked.
Spawning A Web Worker
A Web Worker is simply a JavaScript file. To spawn one, create a JavaScript file that contains all the code you want to run in the worker thread and pass the path to the file to the Worker constructor:
The code snippet above creates and assigns a Worker to the worker
variable. With this, the worker is ready to send and receive data from the application’s main thread.
Communicating With A Web Worker
Web Workers and the main thread send data to each other via a system of messages. This message can be any value such as a string, array, object, or even a boolean.
The Web Worker API provides a postMessge()
method for sending messages to and from a worker and an onmessage
event handler for receiving and responding to messages.
To send a message to or from a worker, call the postMessage()
method on the worker object:
To receive data from a worker or the main thread, create an event listener for the message
event and access it via the data
key:
Note that data passed between a worker and the main thread is copied and not shared.
Terminating A Web Worker
Creating a Web Worker spawns real threads on the user’s computer which consumes system resources. It’s therefore good practice to terminate a worker when it has served its purpose. A worker can be terminated by calling the terminate()
method on the worker. This immediately terminates the worker regardless of whether or not it’s performing a task. A worker can also be terminated from within its scope. To do this, call the close()
method from within the worker:
Limitations Of Web Workers
The Web Workers API is a very powerful tool, but it has a few limitations:
- A worker can’t directly manipulate the DOM and has limited access to methods and properties of the
window
object. - A worker can not be run directly from the filesystem. It can only be run via a server.
Setting Up The Demo Application
A demo application will be created to demonstrate how long running scripts affect performance in web applications. Ensure you have the Web Server for Chrome extension installed in Chrome before continuing.
Create a new folder, web_workers, on your computer and create an index.html file in the web_workers folder. Add the following code to the file:
The code above contains a basic HTML document with three <i>
tags, each holding a space shuttle icon, and also two buttons. On clicking the first button, the space shuttle icons should move from left to right. Clicking the second button should run a CPU-heavy calculation.
Create a styles.css file and add the complementing styles for the markup above:
To see the demo application, open Web Server for Chrome, either by clicking the Apps shortcut in your Chrome bookmarks bar or by navigating to chrome://apps.
Click the Choose Folder button and select the web_workers folder wherever it is located on your computer. Click the toggle button to start the server and visit the web server URL shown in the Web Server for Chrome interface. You should see something similar to the image below:
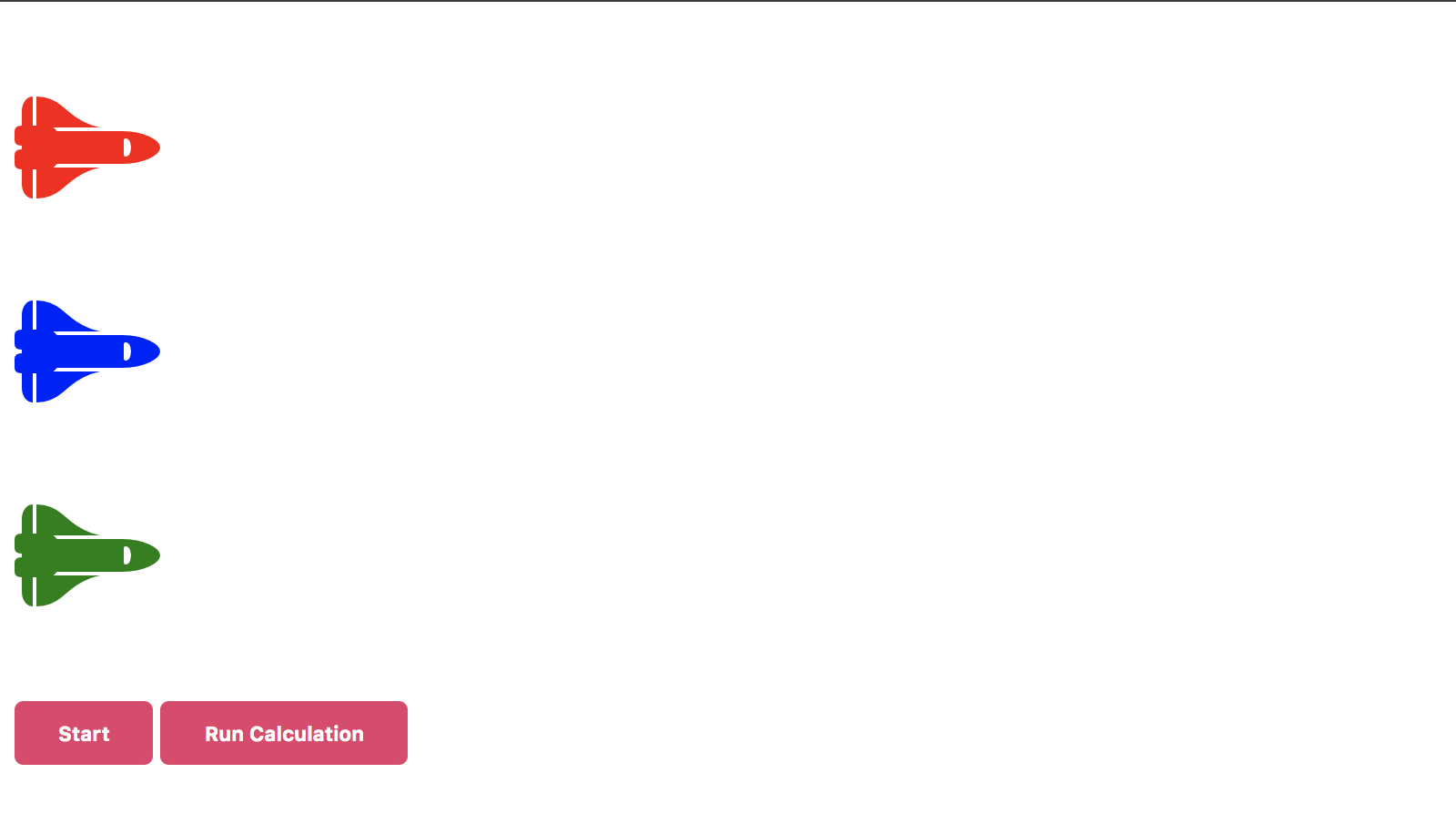
To make the buttons functional, create an index.js file in the web_workers folder and add the following code to it:
The code above contains three functions; a move
function that moves the shuttle images on the page forward by 1px every 5 milliseconds, a calculate
function that returns the 40th number in the Fibonacci sequence, and a fibonacci
function which holds the logic for calculating the index value of the provided number in the Fibonacci sequence using recursion. Calculating the 40th number in the Fibonacci sequence is resource intensive and will take a few seconds to run.
Refresh the demo application in the browser and click the Start button to move the shuttles. At any point in time, click the Run calculation button to run the fibonacci calculation. You’ll observe the shuttle animation freezes for a few seconds. This is a visual representation of how long-running scripts affect performance in web applications.
To identify the cause of the freezing animation, reload the browser tab, open the Developer tools (F12 or Ctrl + Shift + I), and switch to the Performance tab. Click the Record button (Ctrl + E) in the Performance tab to start JavaScript profiling, then click the Start button in the application, followed by the Run calculation button.
After the animation in the application freezes, click the Stop button in the Developer tools Performance tab to end the profiling. You should get a chart similar to the one in the image below:
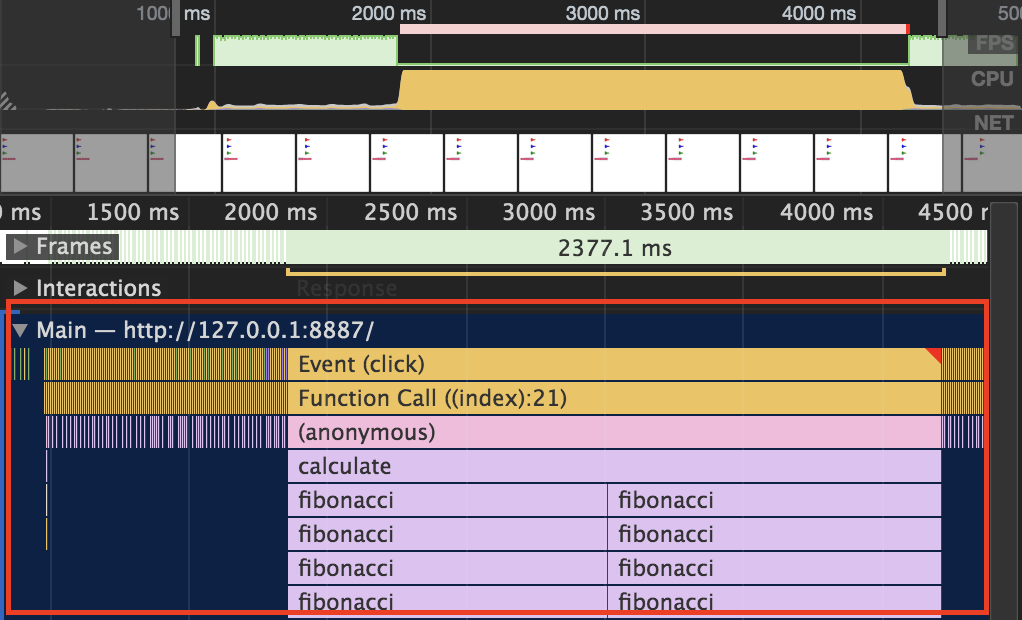
The highlighted section in the image above shows the activity on the main thread represented by flame charts. It shows a click event with a red triangle in the top right corner. The click event causes a function call on line 21 in the index.js file, which in turn calls the fibonacci
function a couple of times.
The red triangle on an event is a warning that indicates a performance issue related to that event. This shows that the fibonacci
function is directly responsible for the frozen animation on the page.
Improving Performance with Web Workers
To ensure the animated shuttles in the demo application are not affected by the fibonacci calculation, the recursive logic for the fibonacci calculation needs to be moved off the main thread so it is not blocked.
Create a file, worker.js in the web_workers folder and move the fibonacci
function there from the index.js file:
Now that the calculation logic has been moved to a worker, it needs to be called whenever the calculate button is clicked. In the index.js file, create a new worker instance and link it to the worker.js file by replacing the fibonacci
function with the following statement:
Update the calculate
function in the index.js file to send the number we want to calculate its index value in the Fibonacci sequence to the worker:
Whenever the calculate function is called, the number 40 is sent to the worker to calculate the 40th number in the Fibonacci sequence. To access this number in the worker, add an onmessage
event listener the top of the worker.js file with the following code.
The onmessage
event handler gets the number from the data
key in the event
object and passes it to the fibonacci
method to get the result of the calculation. Then it sends the result back to the main script and terminates the worker.
The fibonacci calculation is now being run on a different thread and the result is sent back to the main thread. To receive the result in the main script, add an onmessage
event handler at the top of the index.js file:
Reload the application in your browser, start the animation, and click the Run calculation button. You’ll observe that the result of the Fibonacci sequence calculation is still being logged to the browser console but this does not affect the movement of the shuttle images on the page.
To identify the performance impact of the web worker, refresh the browser tab with the Developer tools panel open and the Performance tab selected.
Repeat the steps above to start performance profiling, run the animation, and perform the Fibonacci calculation. You should see results similar to those shown below:
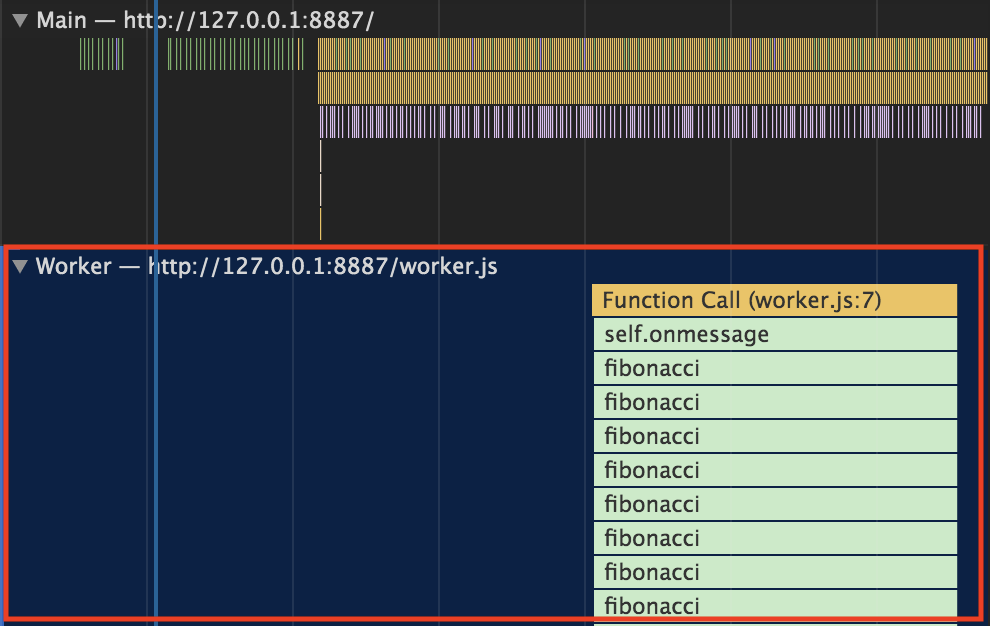
From the image above, the first obvious observation is the presence of a worker thread alongside the main thread. The worker thread shows a function call in the worker.js file with an onmessage
event, which in turn calls the fibonacci
function multiple times. This shows that the fibonacci calculation no longer happens on the main thread, hence the improved performance in the shuttles animation.
Summary
In this post you learned how long running scripts affect web performance and how to fix these performance issues using the Web Workers API. You also saw how to use the Google Chrome developer tools to profile your JavaScript application's performance so you can identify bottlenecks that might benefit from being moved to web workers.
Additional Resources
MDN’s Web Workers API documentation is a great resource that contains everything there is to know about Web Workers.
W3Schools’ Web Workers documentation is another great resource with examples on how to use Web Workers.
You can find the complete code for this post on GitHub.
Chuks Opia is a Software Engineer at Andela. He is also a technical writer and enjoys helping out coding newbies find their way. If you have any questions please feel free to reach out on Twitter: @developia_ or GitHub: @9jaswag.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.