Protecting Your Passwords with the .NET Secret Manager
Time to read: 4 minutes
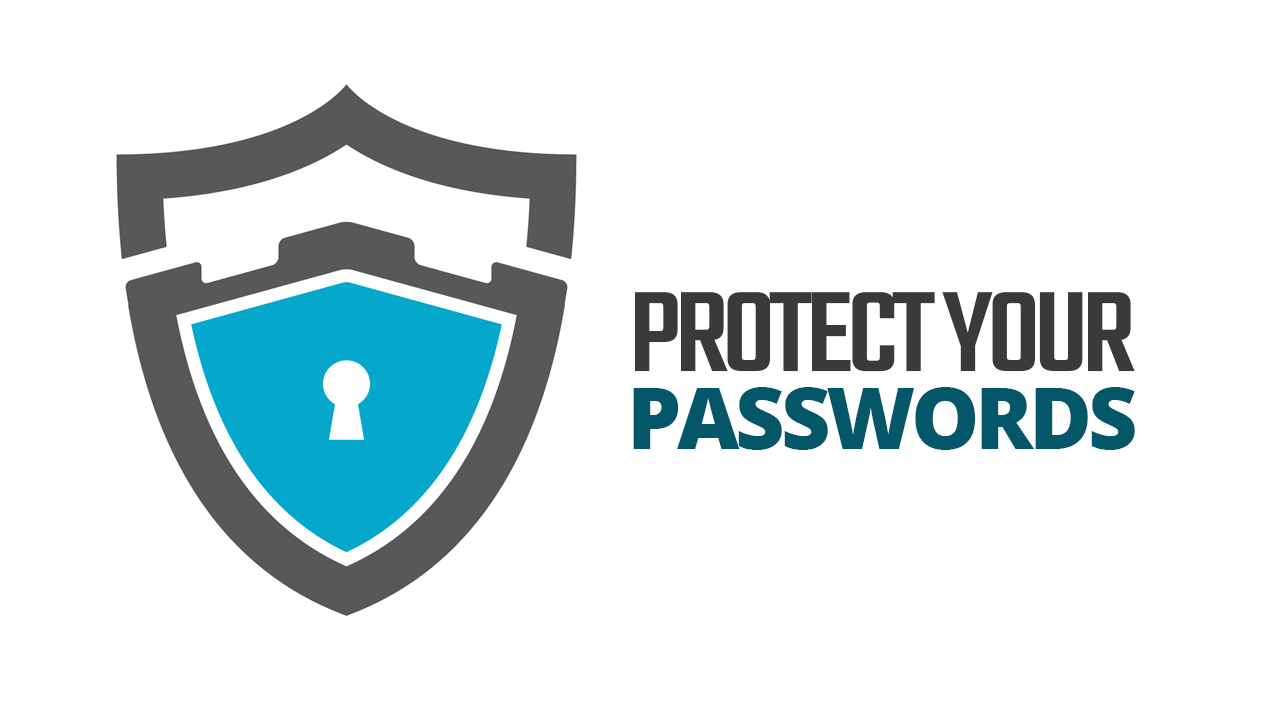
As developers, we’ve all done this at least once. We’ve spent time building a cool project that uses a third party API, and then checked it into Github only to realize our API password is there in plain text for all to see. Do a search in Github to see how frequently this still happens.
When I’m writing code for the first time I hard code those values in my code to get things working and then go to commit it to source control. That’s about the time I remember saving passwords into source control is one of the seven Deadly Sins of Security.
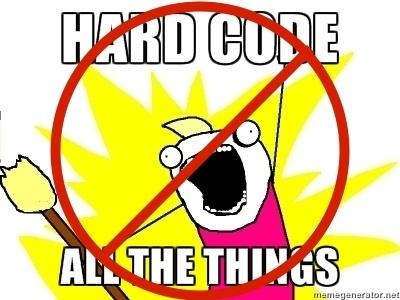
If you’re coding in .NET, the Secret Manager makes it easy to remove these sensitive details before checking your code in. It saves those passwords in your environment variables on your machine.
Let’s say you find yourself building an app that searches for music on Spotify. To search Spotify’s API, you’ll need a client ID and secret. We will replace the hardcoded passwords in our project with the Secret Manager to keep those sensitive details out of source control. When you’re done with this tutorial, you’ll have a functional SMS jukebox that uses the Spotify API.
Ingredients for This Recipe
We’ve got a few tools to install in order to complete this challenge (skip 1-4 if you already have Visual Studio Core setup):
Add Secret Manager to an ASP.NET Core Web API Project
Let’s grab a copy of an existing project from my Git repo. This project is an SMS Jukebox that uses the body of incoming SMS to lookup music data. To get started, use this to clone the project locally: git clone "https://github.com/clw895/SpotifySMS.git" SecretProject
. I’ve cloned it to ~/Code/SecretProject but it is totally cool to save it in a different path! Next open the folder in Visual Studio Code and try out the steps below:
- Update the SpotifySMS.csproj file to add the highlighted XML right above the Project closing tag:
- Open an integrated terminal and run
dotnet restore
in the terminal. This adds the Secret Manager to our project - Get a GUID which will be used to save our secrets locally on our machine and copy that to your clipboard
- In the csproj file, add the GUID to the PropertyGroup at the top of the csproj file like the below:
We’ve just added Secret Manager to your project and created a secret store on your machine. Now let’s protect some passwords!
Using Secrets in the Secret Manager Tool
Time to add the Spotify client ID and secret to our secret store. To get an ID and secret, create a Spotify app and both values will be provided to you. Back in Visual Studio Code, open an integrated terminal and run:
Those commands add our Spotify app details to the secret store. Next we are going to edit the SpotifyController.cs
file to read the secrets out of the configuration store. In the SpotifyController.cs
, start by:
- Add the below properties under the class declaration. We’re going to use these properties throughout our code to access the information in our secret store:
- Replace the class constructor with the code to read the values from our secret store. ASP.NET Core will inject the values from our secret store into the
IConfiguration confg variable in the class constructor.:
Testing Our Secret Manager Project
Start up our SMS jukebox by typing dotnet run
in the Integrated Terminal. Then in a separate terminal or command prompt window, start ngrok: ngrok http 5000
. You can test it by firing up your browser and sending an HTTP GET request to http://{YOUR_NGROK_URL}/api/spotify?body={YOUR_SONG_HERE}
. To get it working with SMS, login to Twilio (or create an account if you don’t have one), purchase a telephone number, and configure the inbound text message to send an HTTP GET request to your ngrok url as in the screenshot below:
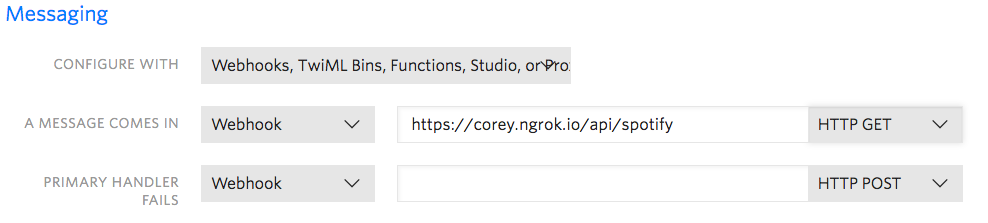
Then fire off a text message with a song name to your Twilio number and watch the magic happen!
Consider Yourself Password Protected
And just lik
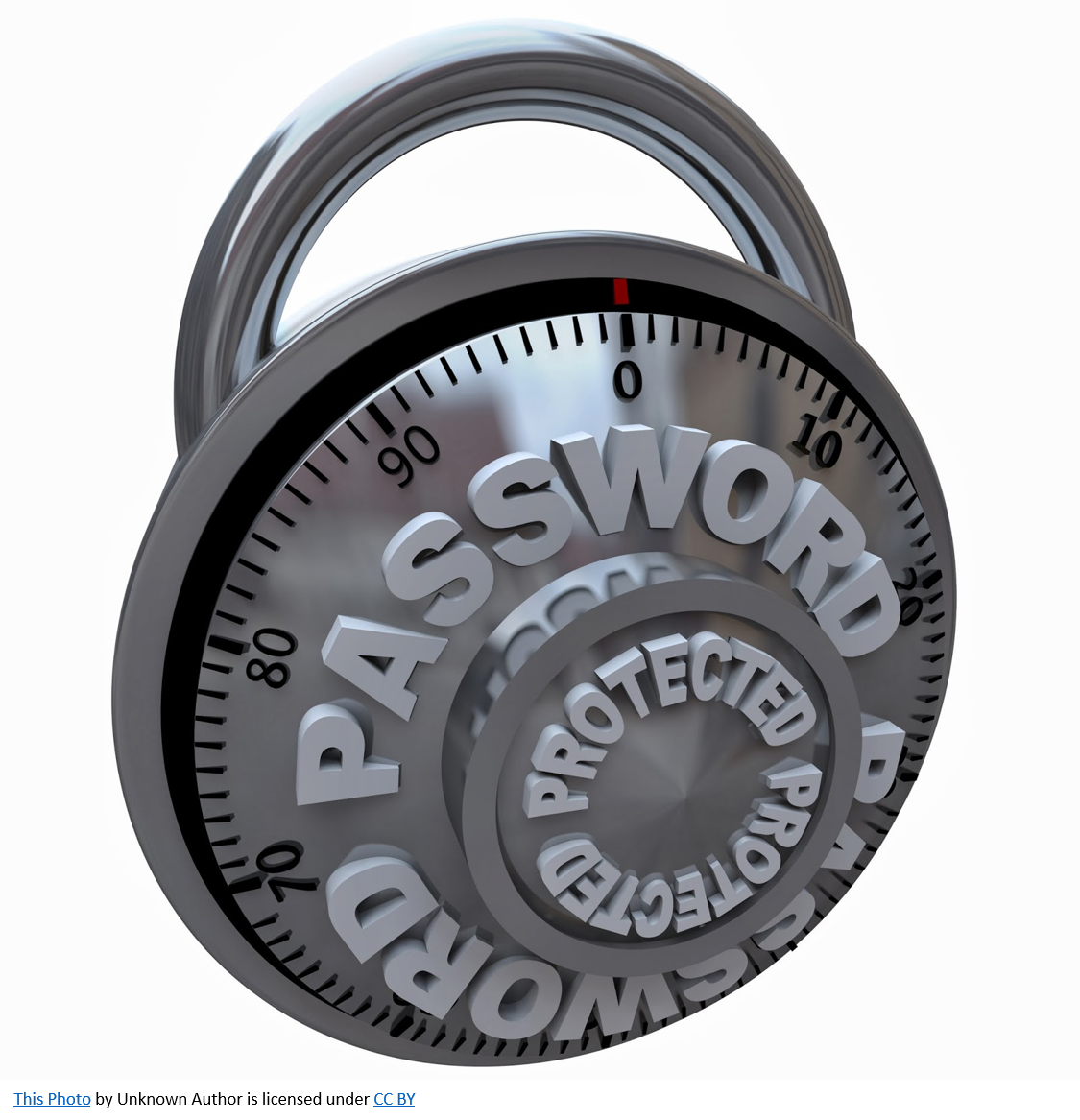
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.