Send Daily Animated Gifs Using Firebase, Giphy, Node.js and Twilio MMS
Time to read: 3 minutes
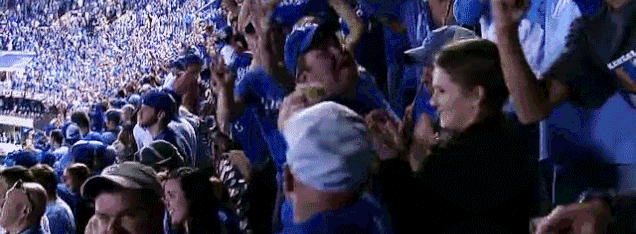
Last month I showed you how to send daily SMS reminders with Firebase, Node.js and Twilio. A week later we launched MMS on long codes in US and Canada and I thought what better way to take advantage of this than to update my app to include a picture in my daily message. What kind of picture would I want to get sent every day? A random gif from Giphy of course! Let’s get to work updating the SMS code to do this. If you haven’t already worked through the previous post, go back and knock it out. Don’t worry, I’ve got plenty of gifs to watch while you’re working.
#BeRoyal
Want to see this app in action? Well I hope you’re as excited about the Kansas City Royals as I am because I built an app that will text you a random Royals gif every afternoon. Want to subscribe? Text the word ‘subscribe’ to (816) 844-6184.
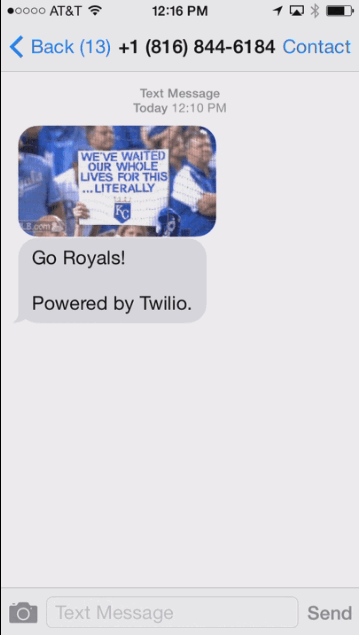
Sending a Gif On Signup
If someone is subscribing to have a gif sent to them everyday, obviously we should send them a great gif to congratulate them when they sign up. To send an MMS using TwiML we want to nest and nouns inside our original verb. If you remember, we’re using the Twilio node helper library to generate our TwiML. With our node library we can nest verbs or nouns by passing a callback function to the function that creates our initial verb. For the sake of clarity, I’ll show the entire /message route and highlight the lines we’re changing to accomplish this:
The body will contain the text we want to reply with. Media contains a url to the image we want to reply with. Be sure to pick a great gif from Giphy to send. I went with this one. Text ‘subscribe’ to your Twilio number and get a virtual high five!
MOAR GIFS!
There’s one major problem with our app right now – there aren’t enough gifs! Fortunately for us we can tap into the Giphy API to access more gifs than we would ever need. To interact with Giphy from our app we’ll be using the giphy-wrapper node library. Let’s install it using npm:
Now let’s instantiate the library at the top of our app.js file:
The giphy-wrapper lets us easily search Giphy for gifs with a certain tag. Let’s add the code that pulls gifs tagged puppy to our app. Replace the code that was in our textJob callback from the last post with this code:
We’re using the giphy-wrapper to pull 100 puppy gifs from Giphy. If there’s an error, we’ll log it to the console. Otherwise, we’ll pull a random gif from our response and log it to the console. Run this code and see it in action. Don’t forget to update the time on your textJob to a time in the near future so you’re not stuck waiting hours to test your code.
Someone Put a Picture in My Message
Now that we’re pulling a random gif from Giphy, let’s add the code that sends that to everyone who is subscribed to get updates. Right after we pull our random gif add the following code:
This code is almost identical to the code we previously used to send SMS messages. We use the sendMessage function provided by the Twilio node library to send our message. The one difference is the we’re also passing a mediaUrl as an argument when we call sendMessage. This mediaUrl is a publicly accessible url to the gif we want to send. Giphy gives us many versions of the gif to retrieve but we’ll be using the downsized image.
Run your app, subscribe and see it in action.
Let There Be Gifs!
You can now send daily animated gifs. Who will be the first to build an animated gif Chuck Norris app? I can’t wait. It’s been almost 1 full month since we opened up MMS. Have you already built a killer MMS hack? I’d love to see it! Questions/Comments? Hit me up on twitter or drop me an e-mail.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.