Send a Slack Notification When Laravel Jobs Fail in Laravel 6 using Queue Failing
Time to read: 2 minutes
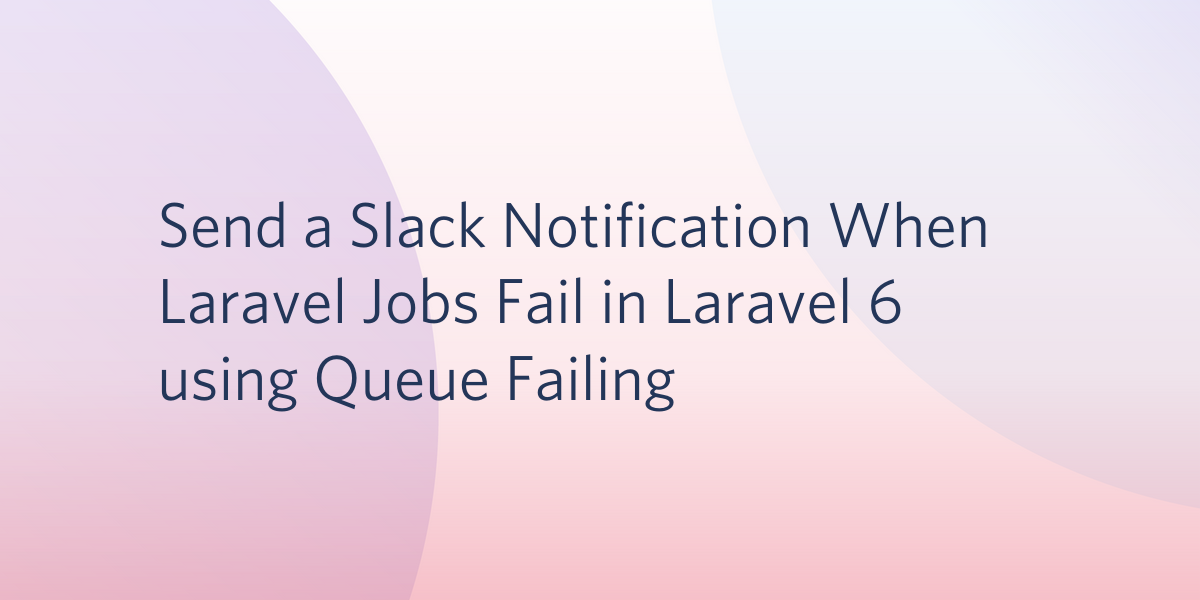
Laravel provides a queue interface to help you defer long-running tasks such as sending emails/SMS, file processing, etc. As much as you try to avoid it, your queue will fail sometimes and because these failures are ignored by default, you need a way to be notified. In this article, we will explore how to send a Slack notification each time a queue fails.
Pre-requisites
Before Laravel 5.8.x, the Slack notification channel was pre-packaged into the framework. If your Laravel version is < 5.8.0, feel free to skip the installation (although you should look into upgrading as Laravel is currently on version 6.0). From Laravel 5.8.x and above, the Slack notification channel is packaged separately from the framework so we have to import it via:
Next, update your .env
file to include your Slack webhook URL. This URL will be used by the Laravel notification to send our message to the associated Slack channel.
Next, generate a new notification with:
Open the newly created file located at APP-FOLDER/app/Notifications/QueueFailed.php
and paste in the following:
Now that our notification and queues are set up, update the boot()
method in our app/Providers/AppServiceProvider.php
file to the one below:
The Queue::failing()
condition is trigged whenever the handle()
method of your Laravel Job fails. Failure could be the result of an exception being thrown, but regardless of the reason, this will be how we test the Slack notification.
That’s it! You should now get a notification in the #general channel of your Slack group each time a queue fails.
Testing
To test the Slack notification, simply run your job with a condition that would cause the job to fail.
If you’ve used the tutorial “Create a Database Queue to Send SMS in PHP with Laravel Queues and Twilio SMS”, setting the To
field in the database to empty will cause the job to fail.
Conclusion
If you're planning to handle your failed queues separately, you might want to start from the official queue documentation.
In any case, feel free to reach out to me on Twitter @firechael if you have questions or suggestions.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.