How to Send SMS in 30 Seconds with VB.NET
Time to read: 3 minutes
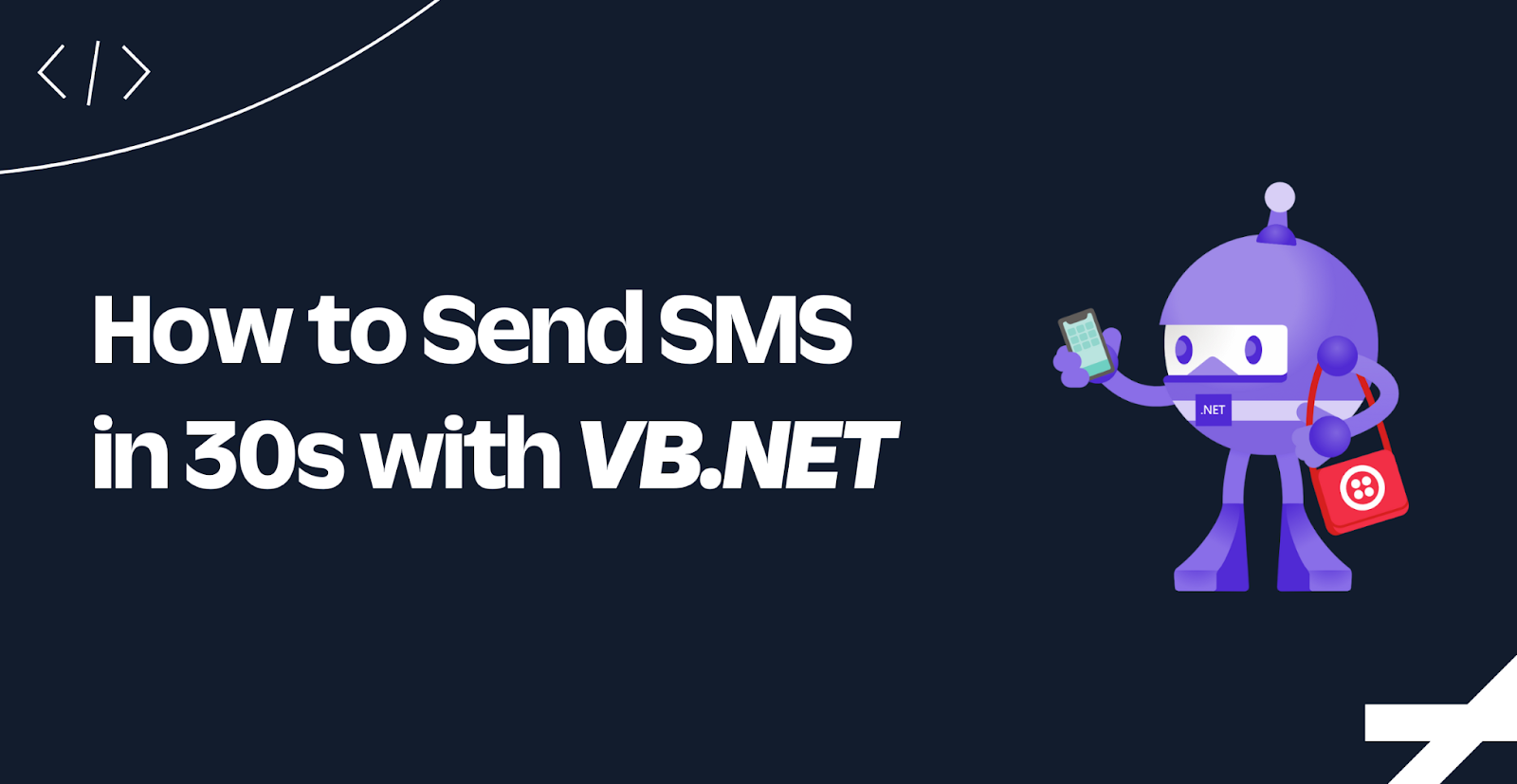
Twilio empowers developers like you to add communication technology to your applications. You can use Twilio's APIs and SDKs to send SMS and MMS, WhatsApp messages, and emails, or make phone calls and video calls.
In this tutorial, you’ll learn how to send an SMS from a VB.NET console application.
Prerequisites
Here’s what you will need to follow along:
- The .NET 7 SDK (earlier and newer versions may work too)
- A code editor or IDE (I recommend JetBrains Rider or Visual Studio)
- A Twilio account (sign up with Twilio for free)
You can find the source code for this tutorial on GitHub. Use it if you run into any issues, or submit an issue if you run into problems.
Buy a Twilio Phone Number
If you haven't done so already, you'll need to buy a Twilio phone number. To do that, log in to the Twilio Console, select Phone Numbers, and then click on the “Buy a number” button. Note that if you have a free account, you will be using your trial credit for this purchase.
On the “Buy a Number” page, select your country and check SMS in the “Capabilities” field. If you’d like to request a number that is local to your region, you can enter your area code in the “Number” field.
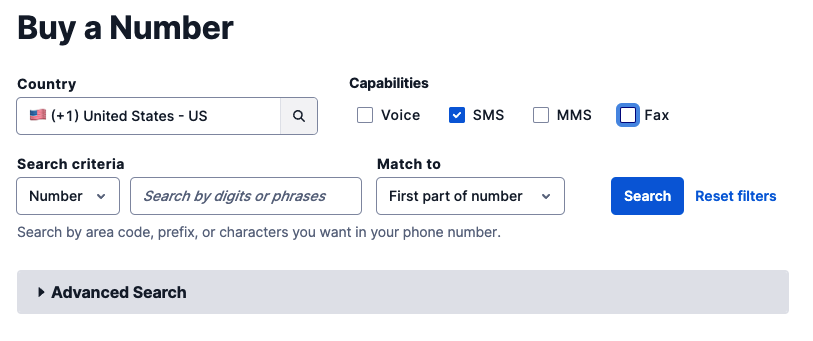
Click the “Search” button to see what numbers are available, and then click “Buy” for the number you like from the results. After you confirm your purchase, click the “Close” button.
Take note of your Twilio Phone Number, as you'll need it later.
Create and set up your VB.NET console project
Open your preferred shell and run the following commands to create a new VB.NET console project:
You'll need to store sensitive secrets to authenticate with the Twilio API. You'll use the .NET Secret Manager, aka user secrets, to store your secrets.
Start by initializing user secrets for your project using this command:
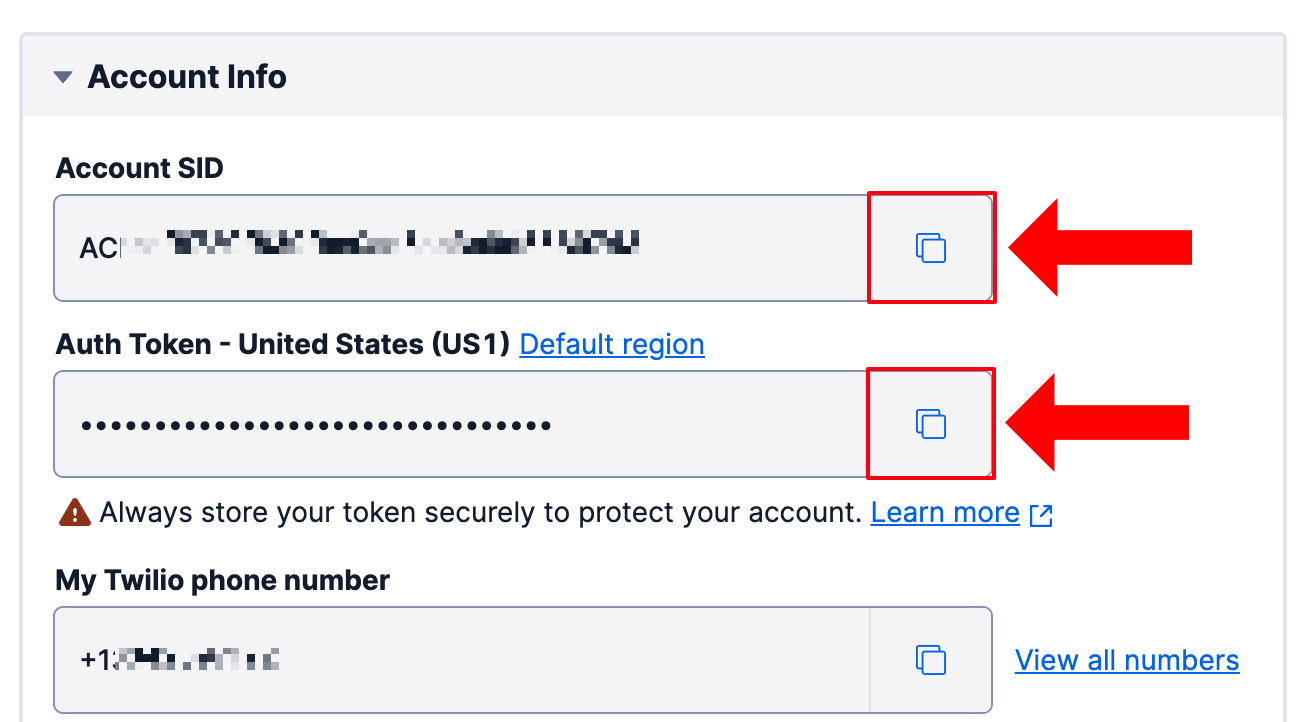
Then, go back to the Twilio Console and find your Account SID and Auth Token in the Account Info section. Use them to replace the respective placeholders in the commands below before running them; they store the Account SID and Auth Token as user secrets.
To load these secrets into your .NET application, you'll need to add the user secrets configuration package. Add it by running the following command:
Now, open the project in your preferred IDE and update the Program.vb file with the following code:
The program:
- Loads the user secrets for the project and puts them into the
configuration
variable - Retrieves the two secrets from configuration
- Prints the Account SID to the console and displays whether the Auth Token has been configured or not.
Try it out by running the following command:
Send an SMS message
Twilio has a helper library for .NET to make sending SMS more convenient. Add the helper library using the .NET CLI, with the command below:
After that, update the Program.vb file to import the following Twilio namespaces:
Then, add the following code at the end of the Main
method:
Replace [YOUR_TWILIO_PHONE_NUMBER]
with your Twilio Phone Number and [YOUR_PERSONAL_PHONE_NUMBER]
with your personal phone number. Both phone numbers need to be E.164 formatted. For example, a US phone number, E.164 formatting would look like +14155552671.
Run your project again using dotnet run
. You should now receive an SMS saying "Ahoy!" and see the following console output:
Next steps
Great job! You now know how to send SMS from VB.NET, but you can do a lot more! You can send MMS, WhatsApp messages, or respond to incoming SMS using the SMS webhook. Give them a try.
We can't wait to see what you build. Let us know!
Niels Swimberghe is a Belgian American software engineer and technical content creator at Twilio. Get in touch with Niels on Twitter @RealSwimburger and follow Niels’ personal blog on .NET, Azure, and web development at swimburger.net.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.