Send SMS with PHP and Twilio
Time to read: 1 minute
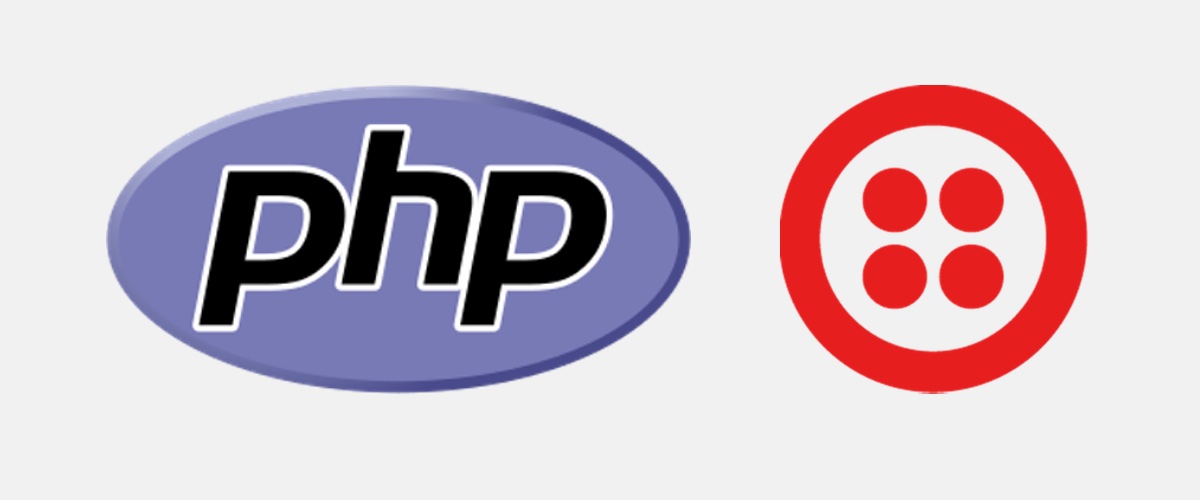
It takes just a few lines of code and even fewer minutes to send your first text message with PHP and Twilio. Here’s how:
Sign up for a free Twilio account and buy a phone number.
Install the PHP helper library with Composer: composer require twilio/sdk:5.* then put require "vendor/autoload.php'; at the top of your file.
Find your account SID and auth token in your console dashboard, and your phone number in your phone numbers list.
Create a file called send-sms.php and paste in the code below to:
- Include the helper library
- Create a Twilio client using our account credentials
- Create a new message from our Twilio number, to our cellphone
Save your file and run it:
Boom! Your phone should light up with a text!
Next Steps
We’re just scratching the surface of what’s possible with PHP and Twilio. If you’d like to explore more, check these out:
- The Twilio PHP documentation
- The Twilio PHP quickstarts
- How to build an SMS trivia game in ~40 lines of PHP
- Call Tracking in PHP
- Getting started with Twilio and Laravel
- The production ready Twilio powered PHP apps over in the tutorials
If you build something sweet using PHP and Twilio, or if you have any questions, I’d love to hear about it. Please drop me a line at @greggyb or gb@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.