How to Send a vCard with Twilio WhatsApp and Node.js
Time to read: 5 minutes
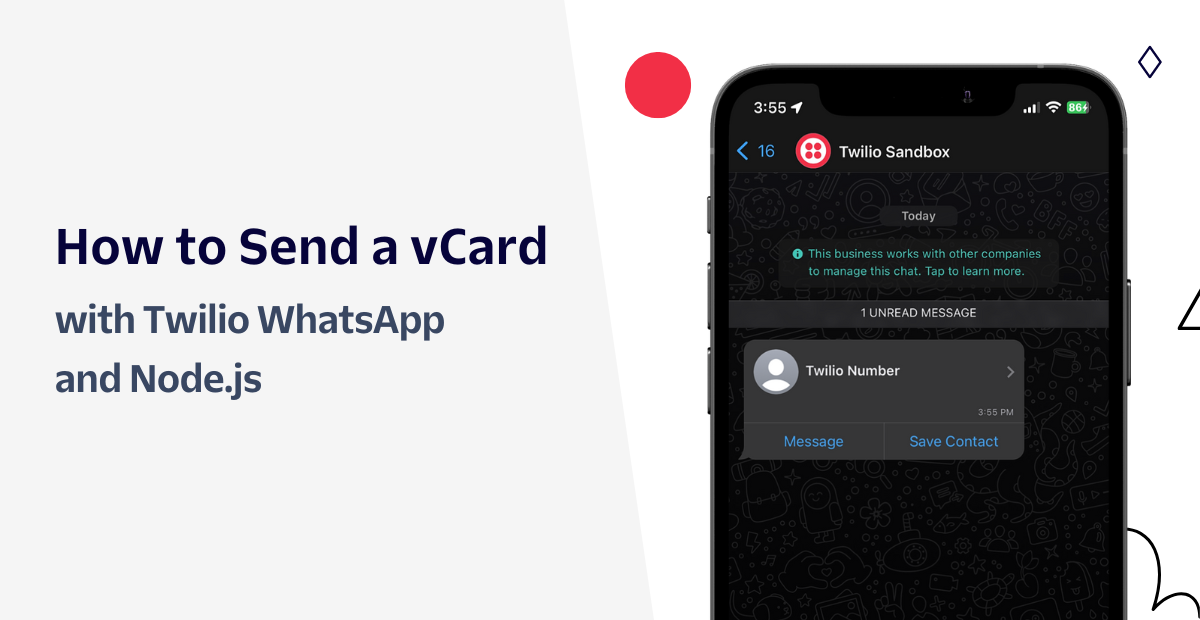
Enhance your customers' interactions with your business and improve their overall experience by sending them vCards. vCards are an electronic contact card in the standardized VCF file format. By using vCards, your customers can seamlessly import your business information into their contact book. In this article, you will learn how to create vCards and send them through the WhatsApp Business API using Node.js.
Prerequisites
- Node.js v16.x installation
- An ngrok account
- ngrok installation that is connected to your ngrok account
- A free Twilio account - Sign up here
- A Twilio number - Read our docs here on how to obtain a Twilio number
What is a vCard?
The vCard file format (.vcf) is specifically created to simplify the smooth sharing of contact information among individuals, applications, and devices. It is a commonly used standard for the exchange and storage of personal information, including name, phone numbers, email addresses, job title, company name, and physical address. By utilizing vCard, it is effortless to transfer your contact details to another person's address book or add a new contact to your own address book within seconds.
With Twilio, vCards can be sent as a media message using the MediaUrl
parameter when creating a message resource.
Set up your Twilio Number with WhatsApp
For demonstration purposes, you’ll be setting up your Twilio number with WhatsApp using the Twilio Sandbox for WhatsApp.
Navigate to your WhatsApp Sandbox settings on your Twilio Console. To head to this section, click on Messaging on the left sidebar of your Console (if you don't see it on the sidebar, click on Explore Products, which will display the list with the available products and there you will see Messaging). Then, click the Settings dropdown and click WhatsApp Sandbox settings within the dropdown.
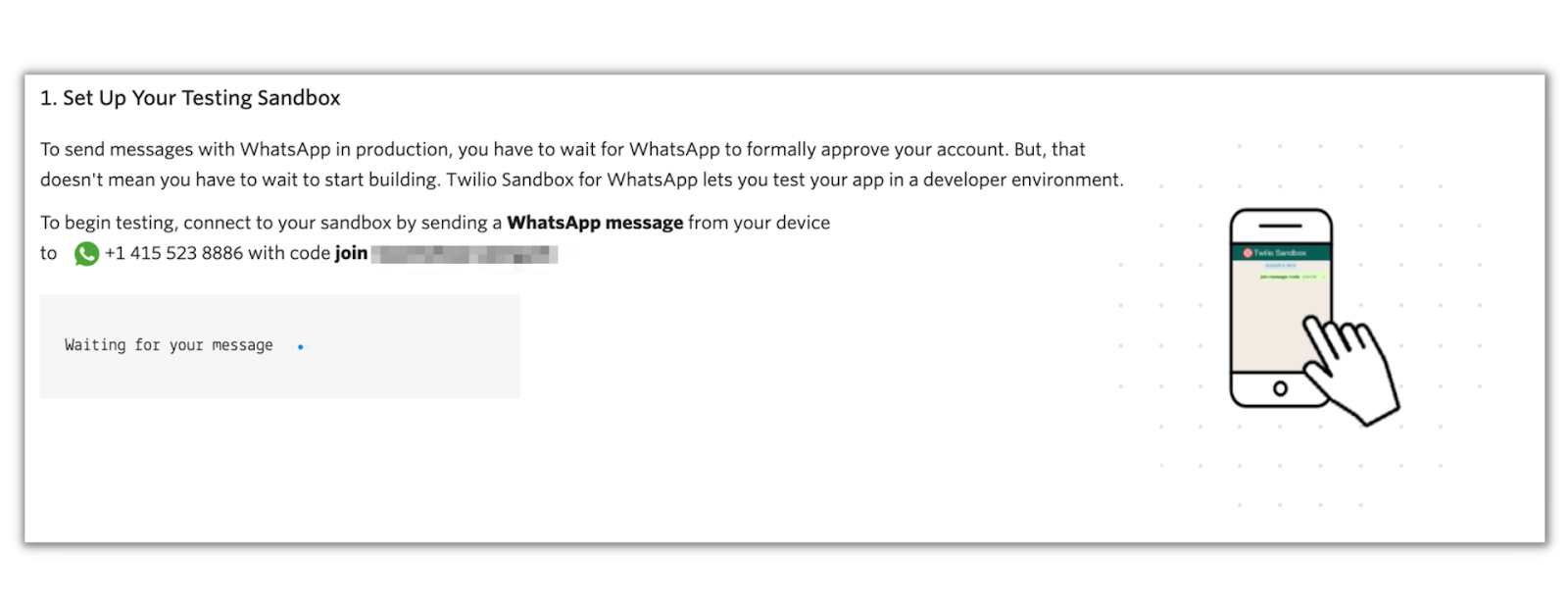
Follow the steps on screen to connect your WhatsApp account to your Twilio account. Upon joining the sandbox, you’ll be able to send or receive WhatsApp messages to yourself.
Set up the Node.js App
Before you create and send out a vCard, you’ll need to set up your application.
Inside your terminal or command prompt, navigate to your preferred directory and enter:
Then create an index.js file with the following command:
This file is where all of your code will be placed.
Install dependencies
Next, you’ll need to initiate a brand new Node.js project and to install the dependencies required for this project:
You will need the twilio
package to send the vCards through WhatsApp. The vcards-js package is used to programmatically create vCards. dotenv
is used to access environment variables, which is where you will store your Twilio SID and Auth token which is needed to interact with the API.
Open up your index.js file with your preferred text editor and enter the following code:
This code will import the environment variables from the .env file, initialize the Twilio library with your credentials and initialize the vCards-js package.
Host your vCard
The contact card you’ll be creating will be stored on a local .vcf file. However, there needs to be a way for Twilio to publicly access this file through a public URL. To achieve this, you’ll use ngrok to expose a static directory (that will hold your .vcf file) through a public URL.
This static directory will be called assets. To create this folder, enter the following command on your terminal:
If you haven't registered your ngrok CLI to your account, register your authentication token in the console:
You’ll then expose your assets folder with the following command:
Replace the <folder path>
placeholder with the full folder path of the assets folder. If you are using Visual Studio Code, you can grab the folder path by right clicking the assets folder in your project directory and then clicking Copy Path.
After entering the command, your terminal will look like the following:
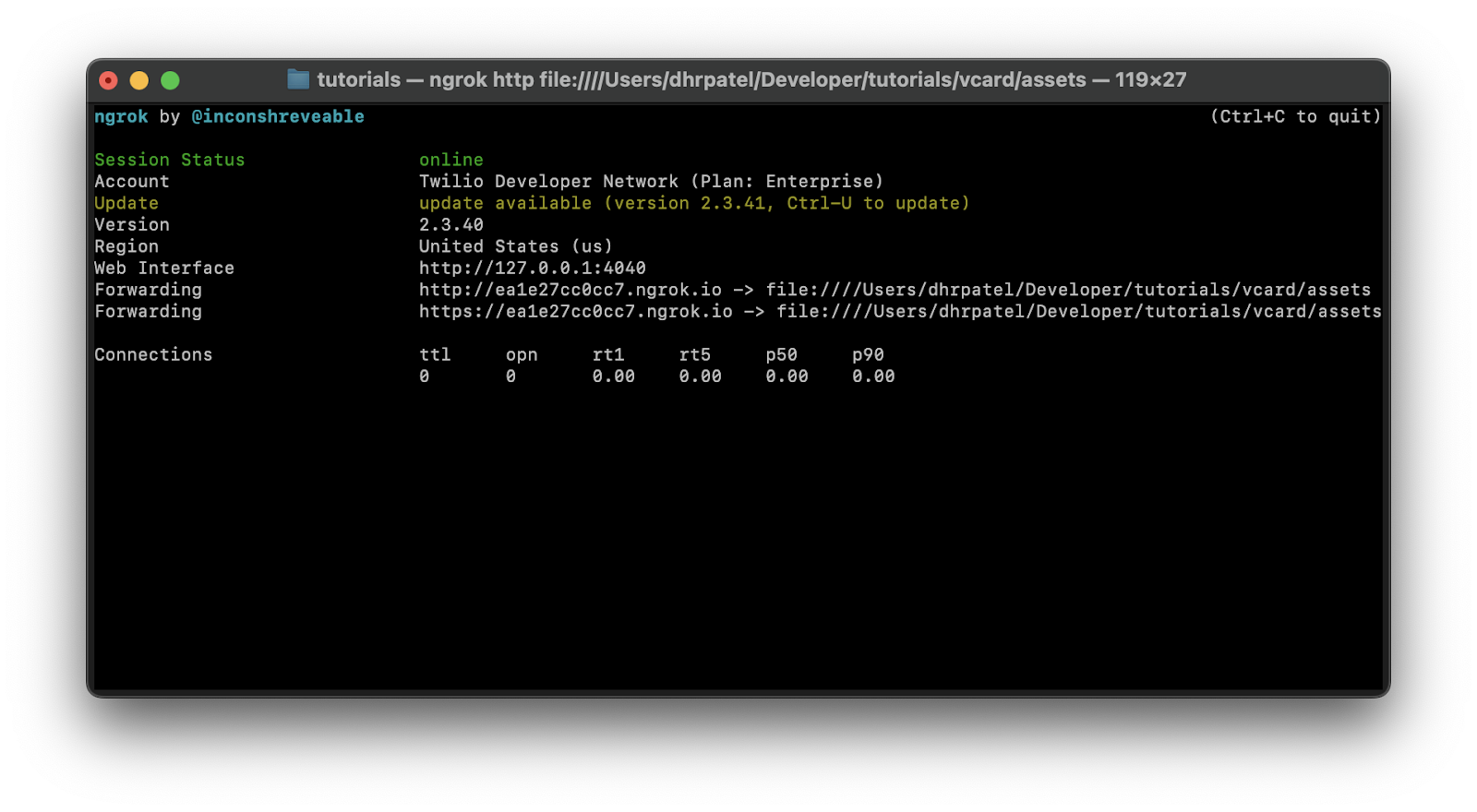
You’ll see that ngrok has generated two Forwarding URLs to your assets folder (in some cases only one URL may be shown). Copy either of the URLs – the https URL is recommended as it’s encrypted – and save it for the next section.
Environment variables
Create a file named .env and place the following lines into the file:
You’ll need to replace the XXXXXXXXXX
placeholders with their respective values. Paste the forwarding URL you just copied from the previous section into the NGROK_URL
placeholder.
To get your Twilio number, Account SID, and Auth Token, log in to the Twilio Console and it will be on your dashboard:
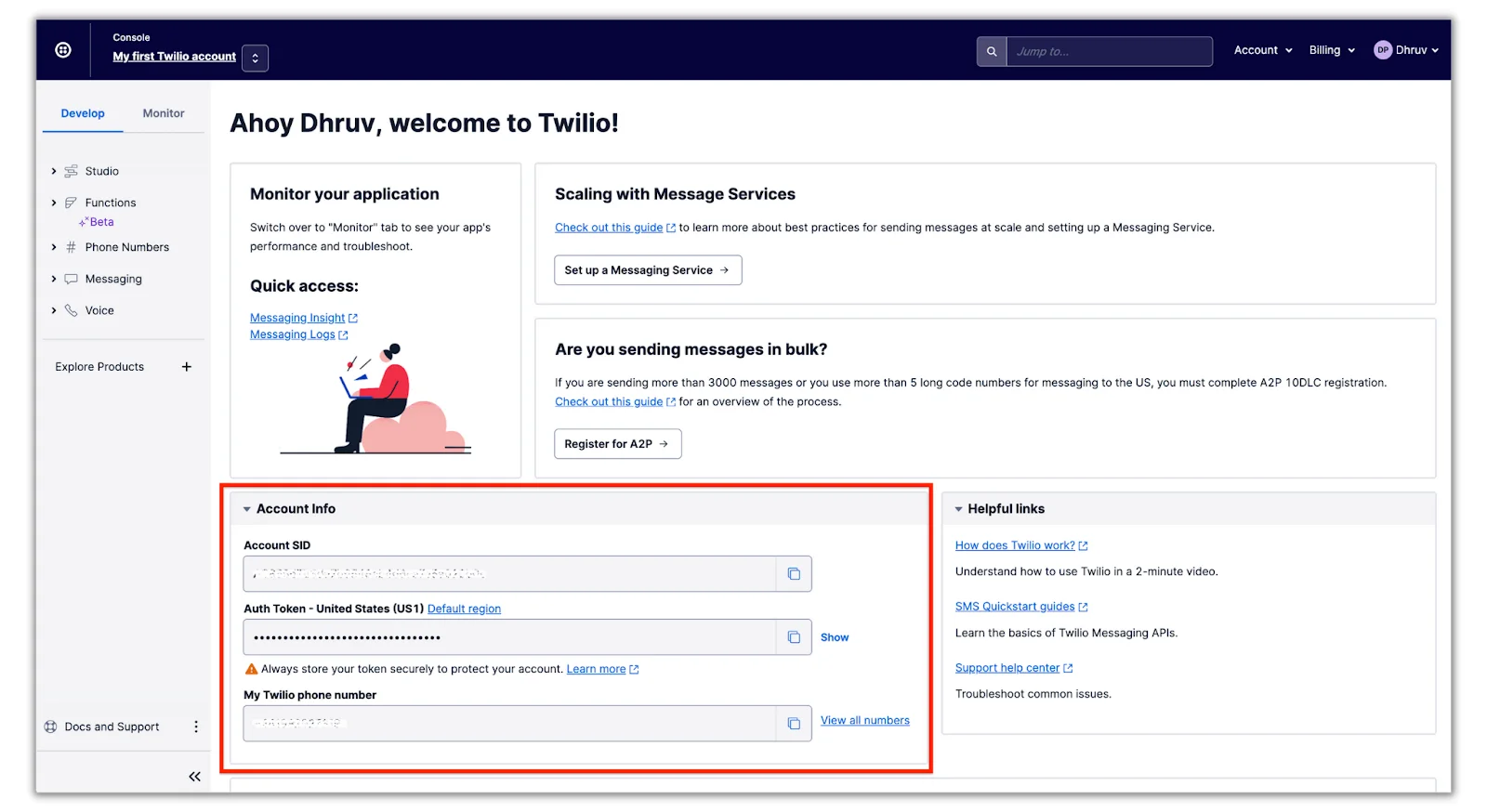
Build the vCard
If you already have a .vcf file that you want to send out, move it into the assets folder, rename it to twilio.vcf and skip to the next section. If you don’t, you’ll be using the vcards-js npm package that you installed earlier to create a vCard in this section.
Open up the index.js file and place the following code in the file:
This code creates a vCard file for your Twilio number using the different vCard properties. Take a look at this example if you want to see the full list of properties you can add to your vCard. Feel free to change the contact info to your liking.
The last line of code will save the vCard in a file called twilio.vcf within the assets folder.
Send the vCard
You’ll be using the Twilio SDK you initialized earlier to send out a WhatsApp message of your newly created vCard. At the bottom of the index.js file add in the following code:
The code above creates a Message resource with the given properties. You can see that the mediaUrl
property is using the publicly accessible URL of the vCard file which you exposed using ngrok.
Replace the <YOUR_NUMBER>
placeholder with your personal phone number and the <SANDBOX_NUMBER>
placeholder with the phone number that Twilio assigned to you in the Sandbox (this is the same number you used when you sent a message to enable the Sandbox). Ensure both of these numbers are in E.164 format.
If you already have your Twilio number registered with WhatsApp, feel free to replace the Sandbox number with it.
Run the code
The code is now ready to be put into use! Navigate back to your terminal and run the application to send the vCard using the following command:
In a few seconds, you’ll receive a WhatsApp message from your Sandbox number with the contact card!
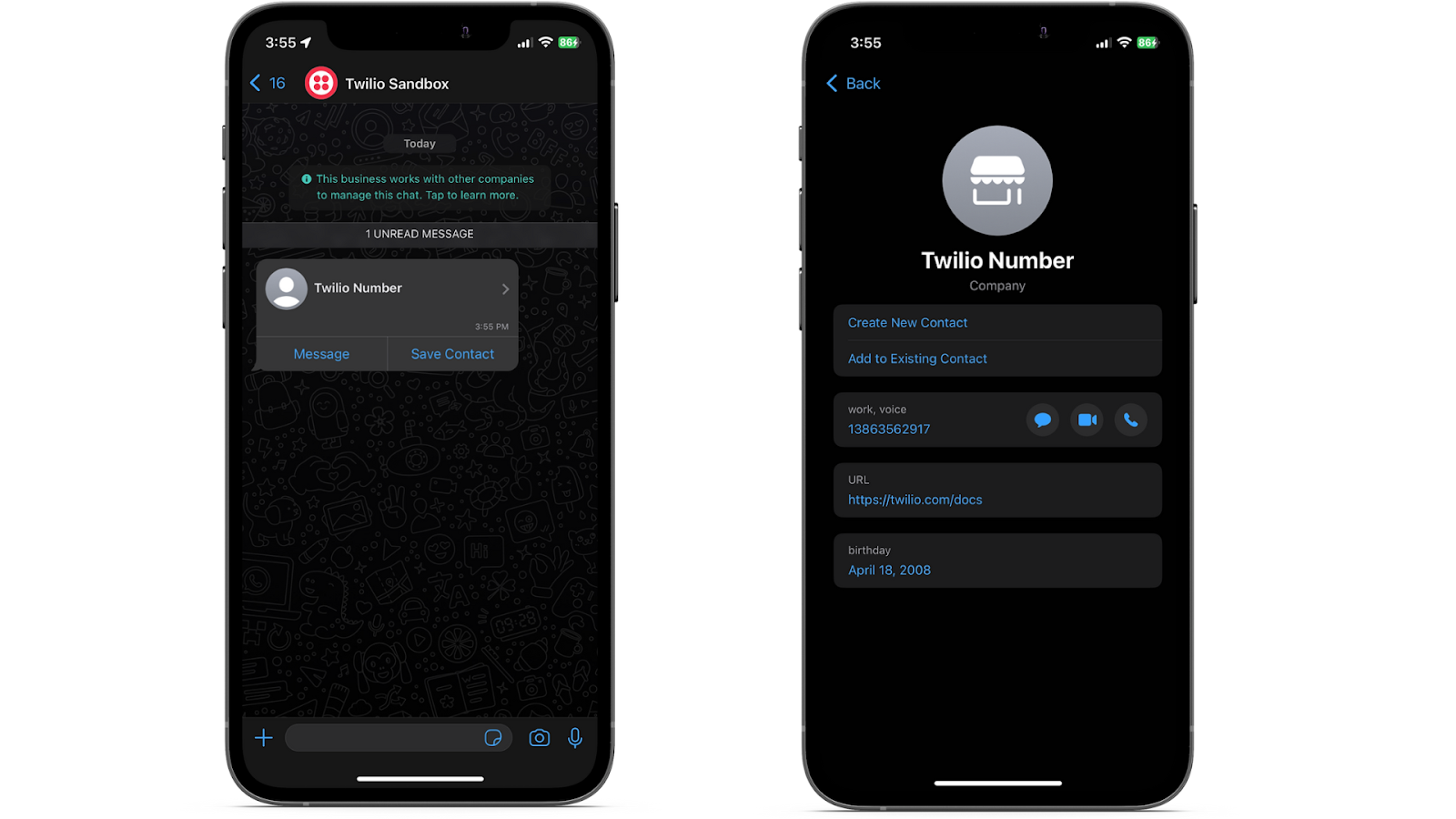
Things to note when sending media through WhatsApp
- You can send media messages to any WhatsApp user who has sent you a message within the past 24 hours. For this tutorial, you’ve already sent out your unique code to the WhatsApp Sandbox sender so you don’t need to worry about the media not going through.
- In production environments, I recommend you have the user opt-in to your messaging service through a WhatsApp message and then sending the vCard once they opt-in. Another option is to ask the user if they’d like to receive a contact card from your business.
- If you send a media message along with a message body, the user will only receive the media; this is the expected behavior when sending an audio, contact, or location message. To send a message along with any of these media types, you’ll need to send two separate messages.
For more information on this, check out the following guide: Sending and Receiving Media with WhatsApp Messaging on Twilio.
Conclusion
Tada! With Twilio and Node.js, sending vCards is possible within minutes! Moving forward, you can enhance your customers' experience by including more information in your contact cards or hosting your app to eliminate the need for serving your contact cards directory. Happy building!
Dhruv Patel is a Developer on Twilio’s Developer Voices team. You can find Dhruv working in a coffee shop with a glass of cold brew or he can either be reached at dhrpatel [at] twilio.com or LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.