Simple Phone Verification with Twilio, PHP, MySQL and jQuery
Time to read: 3 minutes
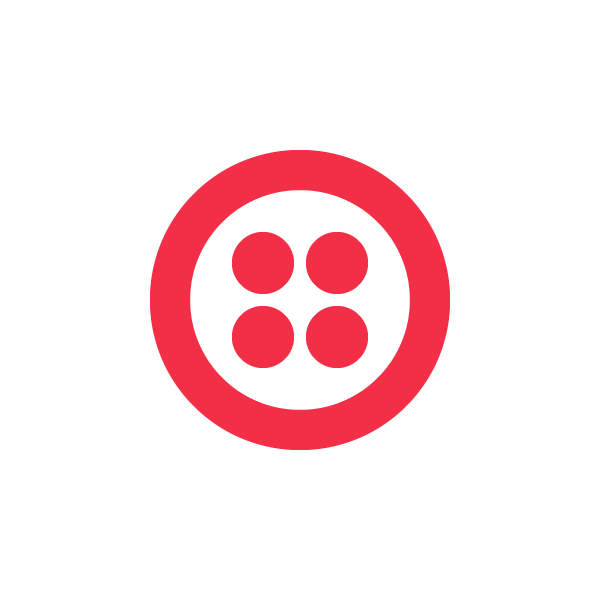


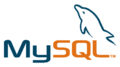
See the most updated version of these instructions and sample code here or read the tutorial on how to build phone verification in a Rails app.
As more and more applications integrate with phones there is an increasing need to verify phone numbers to help prevent spam, fraud and other dubious activities. In these cases it’s common to use an automated phone verification system to present the user a verification code in their web browser while a call is initiated to their phone. When the call is answered they are prompted to enter the code, allowing them to confirm that they are the owner of that number.
In this post I’m going to cover how to build a simple phone number verification system using PHP, MySQL and jQuery.
This is a long example so I’m only going to highlight the most important parts in this post. You can download the complete example here. To run this example on your own server, update the database settings in database.php and your Twilio account settings in call.php. This example also makes use of our Twilio PHP Helper library which you can find on GitHub.
Basic steps
- User visits verification web page and enters phone number.
- Random verification code is generated and user is called and prompted to enter code.
- If code is entered incorrectly, re-prompt to enter code.
- If code is entered correctly, update database.
- Update web page with status message.
Step One: The verification web page
We’ll start with the HTML the user will see. Create a file called index.php and add the following code to the :
When the user visits the page they’ll be presented with the form asking them for their phone number. Once entered, we’ll hide the form and show them their verification code. Here’s the jQuery needed to make that transition:
Our initiateCall() function kicks off an AJAX request to generate the random code and initiate the phone call, which I’ll discuss in more detail in Step Two later. Once we’ve got the code, we’ll update the UI to show it to the user and then start polling our database for status updates. The code to poll the server looks like this:
Once we’ve got confirmation from the server that the number has been verified, we update the UI to let the user know.
Step Two: Generate and display random code
Step Two in our walkthrough overlaps with Step One since it is initiated when the user submits the form with their phone number. We’ll pick up in our initiateCall() function which makes the AJAX POST request to call.php. Inside call.php we generate the random 6-digit verification code and start the phone call by calling the Twilio REST API using the PHP Helper Library. Once we have the code, we’ll send it back as JSON for the browser to parse and display (as covered in Step One).
We’re storing the phone number along with the generated code in a MySQL database so that we can update the verified column from our TwiML script and then retrieve it later. For this example I’m using a simple table definition:
Steps Three and Four: Collect and verify code via phone call
When initiating the call we direct Twilio to use the twiml.php file which generates the necessary TwiML to prompt the caller to enter their code. The first time this file is requested we’ll ask the caller to enter their code. Once they’ve entered 6 digits, Twilio will make another post to the same URL with the Digits POST parameter now included. With this information we look up the caller’s phone number in the database and check for a match. If the code entered is incorrect, we’ll re-prompt them to enter it again. Once they’ve entered the correct code we update the database and thank them for calling.
Step Five: Update the web page when the number is verified
Back in Step One we created the jQuery needed to begin polling the server for status updates. Our checkStatus() function makes a POST to status.php which looks up the phone number in the database and sends back some JSON containing the verification status.
Coming soon: Verification via SMS
In an upcoming post I’ll be using this example as a starting point for creating a similar system to verify phones but using SMS instead of voice. Let me know in the comments below if there’s anything you’d like to see covered in that post.
Download the complete example
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.