How to use Twilio SMS and Voice with a .NET 6 Minimal API
Time to read: 5 minutes
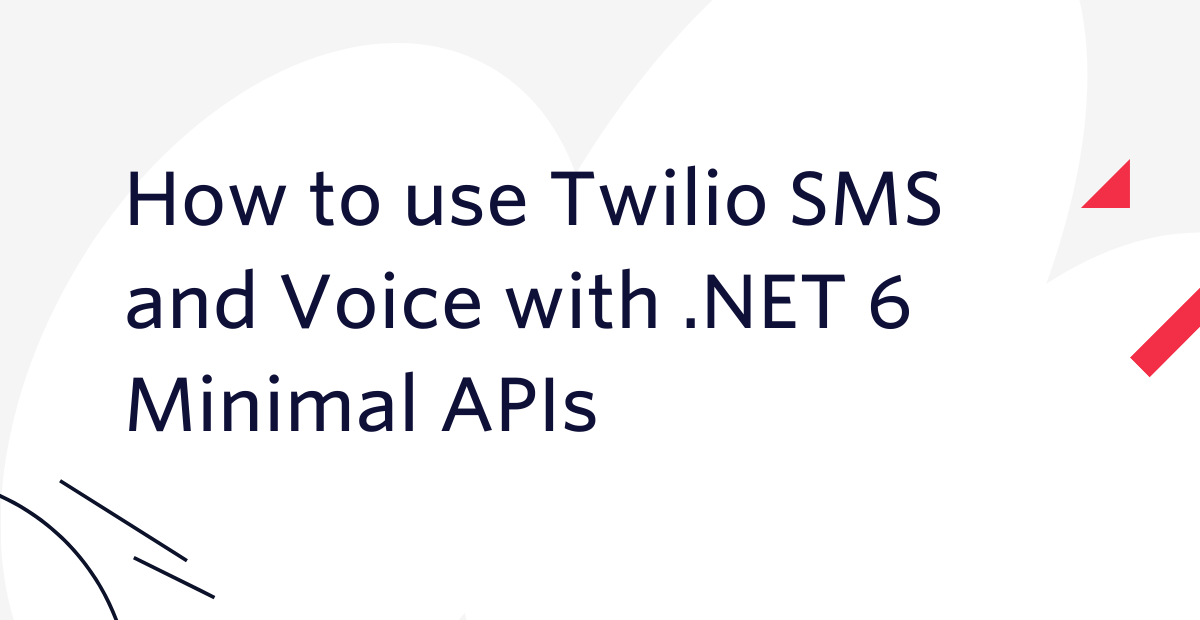
With the release of .NET 6, ASP.NET Core introduced a new feature called Minimal APIs. Minimal APIs give you the ability to create HTTP APIs with minimal code. This is ideal for developers who want to build applications or microservices with the minimum amount of files and code possible. In this tutorial, you will learn how to build a Minimal API and use it with Twilio to respond to SMS messages and voice calls.
Prerequisites
You will need these items to follow along:
- OS that supports .NET (Windows/Mac/Linux)
- .NET 6 SDK
- A code editor or IDE (Recommended: VS Code with C# Plugin , Visual Studio , or JetBrains Rider)
- A free Twilio account
- Install Ngrok
- Web Browser of your choice.
You can find the source code for this tutorial on GitHub. Use the source code if you run into any issues, or submit an issue on this GitHub repo if you run into problems.
Get started with Twilio
In order to get started with Twilio, you will need a few things:
- If you don’t already have a Twilio phone number, go and buy a new phone number. Make sure you keep track of your Twilio phone number to use later on!
- If you are using a trial Twilio account, you can only send text messages to Verified Caller IDs. Verify your phone number or the phone number you want to SMS if it is not on the list of Verified Caller IDs.
Preparing your .NET 6 Minimal API Project
To set up your .NET 6 Minimal API project, you will create a new project using the .NET CLI. In the command line or terminal window, navigate to the folder you want your project to be created in, and run the following command:
This command will create a new empty .NET Web project in a folder called TwilioMinApi.
Navigate to the new folder:
Out of the box, your new project will contain one C# file, named Program.cs:
This program creates a new web application with a single endpoint responding with “Hello World”. You can run this by using the .NET CLI:
Create a SMS Endpoint
You need to create an endpoint for a POST request called “sms”. This endpoint is where Twilio will send an HTTP request when a message is sent to your Twilio phone number. Our sms endpoint will be configured as the webhook URL in the Twilio Console. Check out this article for a better understanding of webhooks.
The following code creates an endpoint handling HTTP POST requests to /sms. This endpoint returns TwiML or Twilio Markup Language to tell Twilio how to respond to the incoming message. You can do this in a number of ways. First, you can simply write the TwiML by hand like this:
In this code, you create a TwiML response to send back a message that says “Hello from a .NET Minimal API!”. Next, you are telling the application to return the response
text as XML. Another way you can achieve this is by using the Twilio SDK for C# and .NET. The Twilio SDK has APIs to build the TwiML for us. To add the SDK, you need to add the Twilio NuGet package.
You can install the NuGet package with the .NET CLI using the following command:
Add the following using statements at the top of the Program.cs file:
Now you can write the endpoint this way:
Run ngrok
In your command prompt or terminal window, start the website using the .NET CLI by running the following command:
The output will look like this:
Running the ngrok CLI tool on your machine will tunnel the local URL to a public URL. Every time you start a new tunnel using ngrok, the subdomain will be different.
You want to start ngrok using the following command and replacing [YOUR_HTTP_SERVER_URL] with the web server URL starting with http://localhost. In this instance, I will be using port 5118. This will start a new tunnel to a new public URL that you can find in the displayed output.
You can navigate to one of the Forwarding URLs listed followed by /sms. Some browsers may warn you that this is a deceptive site, which you can disregard in this case. The browser should return the TwiML that was created by our sms endpoint.
You can also tunnel HTTPS URLs, but you need to sign up for an ngrok account (free) and authenticate your ngrok CLI tool. Once you have done that, you can also run the ngrok https command with the URL starting with https://localhost instead.
The Forwarding URL that starts with https in the session details is what you will need to configure your phone number in the Twilio Console.
Configuring Your Twilio Phone Number
Now you need to configure your Twilio Phone Number, the one you purchased during the Getting Started with Twilio section, to send HTTP requests to your server. Navigate to Phone Numbers > Manage > Active Numbers. Select the number you purchased earlier. Then scroll down to the Messaging section to configure what happens when a message comes to our Twilio number. Update the section that says When a Message Comes In. Make sure Webhook is selected, and paste your ngrok HTTPS Forwarding followed by /sms URL in the text box to send webhook HTTP requests to the /sms endpoint. Make sure the far right drop down has HTTP POST selected, and save your changes to the phone number configuration. Now, try sending a message to your Twilio Phone Number from your cell phone. Did you get a message back saying “Thanks for messaging my dotnet minimal api!”? If so, great job! If not, here are a few things you can check.
- Double-check your ngrok URL in the Twilio Console where you configured your Active Number and make sure that you saved your changes.
- Look at your ngrok window to see if your local server received a request .
- Verify that your local project is compiled and running.
- Check the Messages Log tab where you configured your Twilio Phone Number.
Create a Voice Endpoint
Creating a voice endpoint is very similar to the SMS endpoint. First, create the voice endpoint writing the TwiML by hand.
You can also utilize the Twilio SDK to build the TwiML for you. Both of these code examples will have the same response.
Again, you need to navigate to Phone Numbers > Manage > Active Numbers. Select the number that you purchased earlier. Then scroll down to the Voice section to configure what happens when a call comes to your Twilio Phone Number. Update the section that says When a Call Comes In. Make sure Webhook is selected and paste your ngrok HTTPS Forwarding followed by /voice in the text box to send webhook HTTP requests to the /voice endpoint. Make sure the far right drop down has HTTP POST selected, and save your changes to the phone number configuration. Now, try calling your Twilio Phone Number. You should hear a voice saying “Thanks for calling my dotnet minimal api!”.
Conclusion
.NET 6 gives us a quick and easy way to set up a minimal API and use it with Twilio to respond to SMS messages and voice calls. If you’re a JavaScript developer and you’ve used the Express framework this should feel very familiar. There is a lot more that you can do with TwiML, check out the TwiML Messaging and TwiML Voice docs to learn more!
Additional Resources
Check out these other great .NET resources on the Twilio Blog!
- Send Emails with C# and .NET 6 using the SendGrid API
- Send Scheduled SMS with C# .NET and Twilio Programmable Messaging
Chris Gargotta is a Developer Evangelist who works primarily with Enterprise Developers at Twilio. Get in touch with Chris on Twitter @TheToeFrog, find him on Twitch, and follow Chris’ personal blog at ToeFrog.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.