How to Send SMS without a Phone Number using C# .NET and an Alphanumeric Sender ID
Time to read: 4 minutes
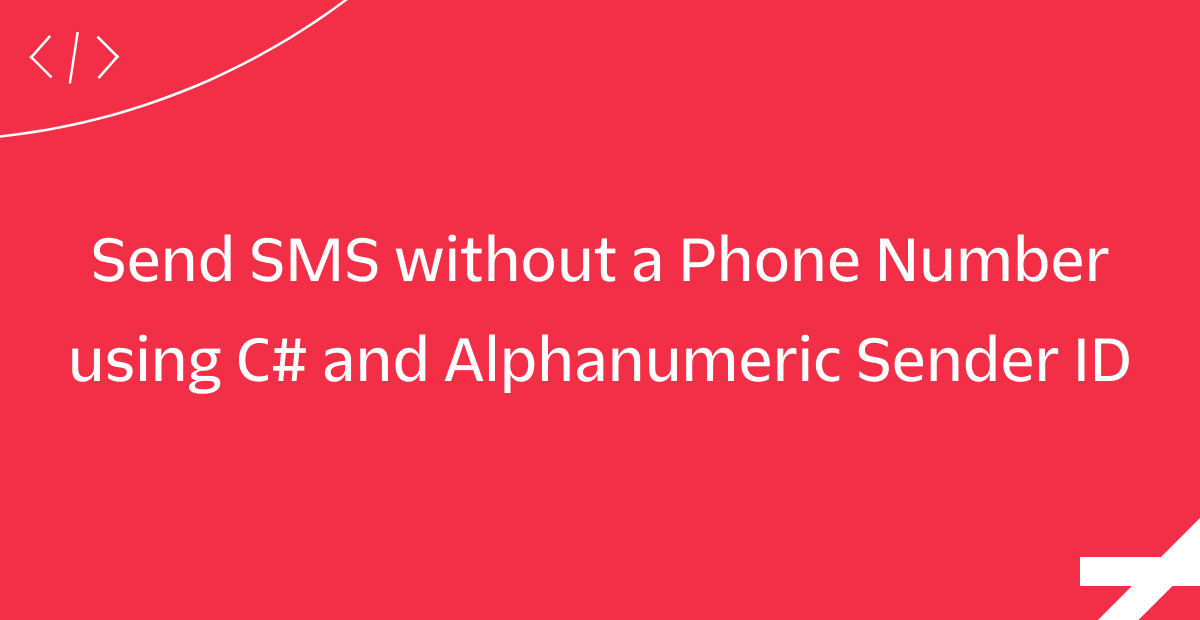
You can quickly buy a phone number using Twilio's Super Network and then use that phone number to send and receive SMS. However, not all applications require two-way messaging. What's more, there are a lot of use cases where one-way messaging is a better fit, for example: notifications, alerts, and verifications. For these kinds of scenarios, you should consider using an Alphanumeric Sender ID.
In this tutorial, you will learn how to send SMS messages from an Alphanumeric Sender ID with C#, .NET, and Twilio Programmable SMS; but first…
What is an Alphanumeric Sender ID
An Alphanumeric Sender ID (or Alpha Sender) lets you send SMS messages from a sender ID that consists of a set of alphanumeric characters, instead of from a phone number.
The biggest benefit of using Alpha Sender is that you can use an ID that is more recognizable to your users than a phone number. The example below shows how an Alpha Sender (AUTHMSG) would look instead of the standard number.

There are a couple of things you should know about Alpha Sender:
- The ID can contain up to 11 characters, composed of upper and lower case letters (A-Z), numbers (0-9), and spaces.
- A single Alpha Sender can be used across many countries without needing a dedicated phone number for each — but not all countries support Alpha Sender, and some countries require pre-registration.
- Recipients cannot respond to an SMS sent from an Alpha Sender, making it a one-way conversation from the Alpha Sender to the recipient.
For other benefits, limitations, and guidance, read the support article about Alphanumeric Sender ID for Twilio Programmable SMS
Prerequisites
You will need these things to follow along with this tutorial:
- An OS that supports .NET (Windows/macOS/Linux)
- .NET 6 SDK
- A code editor or IDE (I recommend VS Code with the C# plugin, Visual Studio, or JetBrains Rider)
- A free or paid Twilio account
- A phone number from a country that supports Alphanumeric Sender IDs, to test your application
You can find the source code for this tutorial on GitHub. Use it if you run into any issues, or submit an issue if you run into problems.
Configure an Alphanumeric Sender ID in Twilio
The recommended way to send SMS from an Alpha Sender is to use a Messaging Service in Twilio. To create a Messaging Service, log into the Twilio Console and click Explore Products in the left-hand side navigation menu. On the Explore Products page, find and click the Messaging product. Then, in the left-hand side navigation menu, click on Services under the Messaging submenu. On the Messaging Services page, click Create Messaging Service, which leads you to a multistep wizard.
On Step 1, enter a meaningful name into the Messaging Service friendly name, and click Create Messaging Service.
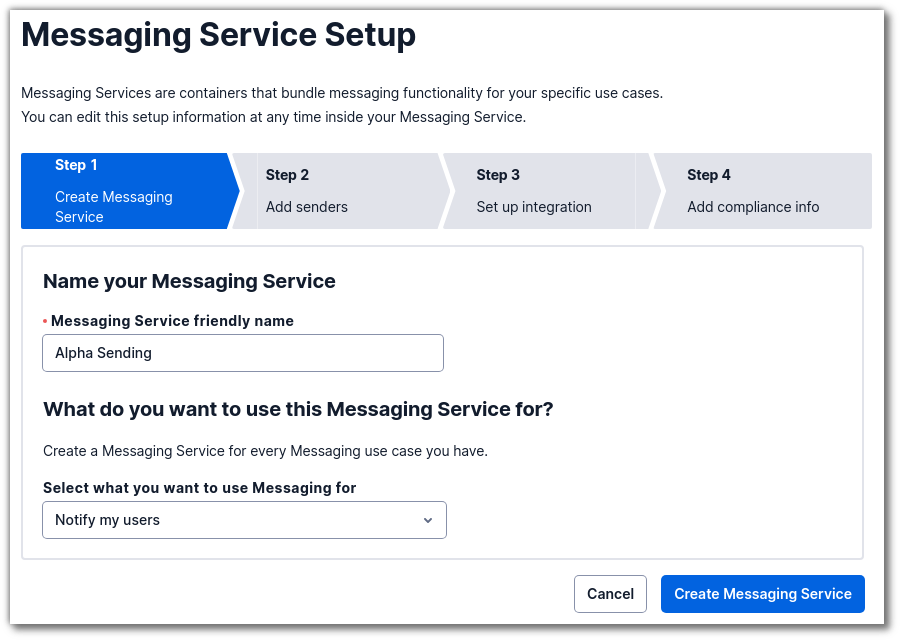
On Step 2, select the "Alpha Sender" option for the Sender Type dropdown, and click Continue. Next, enter your desired ID into the Alpha Sender ID field. This will be the ID shown to the recipient instead of a phone number.
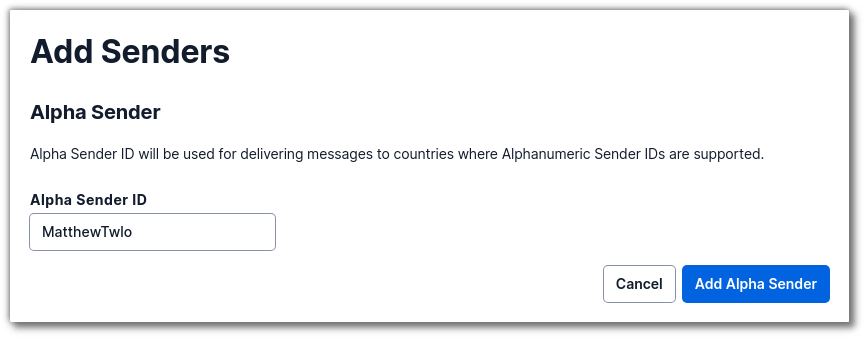
Click Add Alpha Sender and then click "Step 3: Set up integration".
Step 3 lets you configure how to handle incoming messages. Alpha Senders can't receive messages, so you can ignore this step and go to "Step 4: Add compliance info".
In Step 4, follow the described steps to add compliance info, if required for your use-case.
Finally, click Complete Messaging Service Setup.
You will be prompted to test from the console; you can also get to this by navigating the console menus: Messaging > Try it out > Send an SMS.
You may see a warning message that no phone numbers are configured for this messaging service, which you can safely ignore.
There will be a cURL command that you can copy/paste to your terminal. If you use the test interface or the cURL command to send an SMS to your phone number, you should receive an SMS from your Alpha Sender ID. Notice that you can't reply.
Get Your Twilio Configuration
You'll need some configuration elements from Twilio to send text messages from C#. Back in the Twilio Console, click on the Properties link under your Messaging Service in the left navigation menu, then take note of your Messaging Service SID. You will need it later.
Then, click on your account link on the top left to navigate back to the Twilio Console start page, and take note of your Twilio Account SID and Auth Token located at the bottom left of the page.
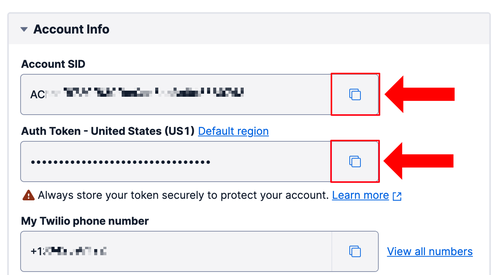
You need to configure this Twilio configuration as environment variables because the project will need them. Open your preferred shell, and set these environment variables using the following commands.
In each case:
- Replace
[RECIPIENT_PHONE_NUMBER]
with the phone number you want to text. This phone number has to be from a country that supports Alphanumeric Sender IDs. If you're sending a text to a number in another country, make sure to enable the country in the Messaging Geographic Permissions. - Replace
[TWILIO_ACCOUNT_SID]
,[TWILIO_AUTH_TOKEN]
, and[TWILIO_MESSAGING_SERVICE_SID]
with the Account SID, Auth Token, and Messaging Service SID you took note of earlier.
SMS from Alphanumeric Sender ID with C# and .NET
With that configuration done, it's time to write some C# code! and create a new console project using the .NET CLI, by running the following command:
This will create a new console project in a new folder named SendAlphaSms. Navigate to the SendAlphaSms folder by running the following command:
The best way to start using Twilio in .NET is to add the Twilio SDK for C# and .NET. Add the Twilio NuGet package using the .NET CLI:
Open the project in your preferred .NET editor and open the Program.cs file. Then, replace the existing code with the following:
The code grabs the configuration from the environment variables, then authenticates with Twilio, and finally sends an SMS using your Messaging Service, which will use your Alpha Sender.
Try it out by running the application using the .NET CLI:
If you entered your phone number, you will now receive a text message from your Alpha Sender! Great job 🎉
Next steps
Congratulations on learning how to send SMS from an Alpha Sender with .NET and C#! If you run into any problems, you can refer to the source code for this tutorial on GitHub, or you can submit an issue on the GitHub repository. In addition to the above sample code, the source code also contains a sample for sending SMS from an Alpha Sender without a Messaging Service.
We can't wait to see what you'll build with Twilio and Alphanumeric Sender IDs!
Niels Swimberghe is a Belgian American software engineer and technical content creator at Twilio. Get in touch with Niels on Twitter @RealSwimburger and follow Niels’ personal blog on .NET, Azure, and web development at swimburger.net.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.