Add an Interpreter to a Telehealth App with VOYCE and Twilio Programmable Video
Time to read: 6 minutes
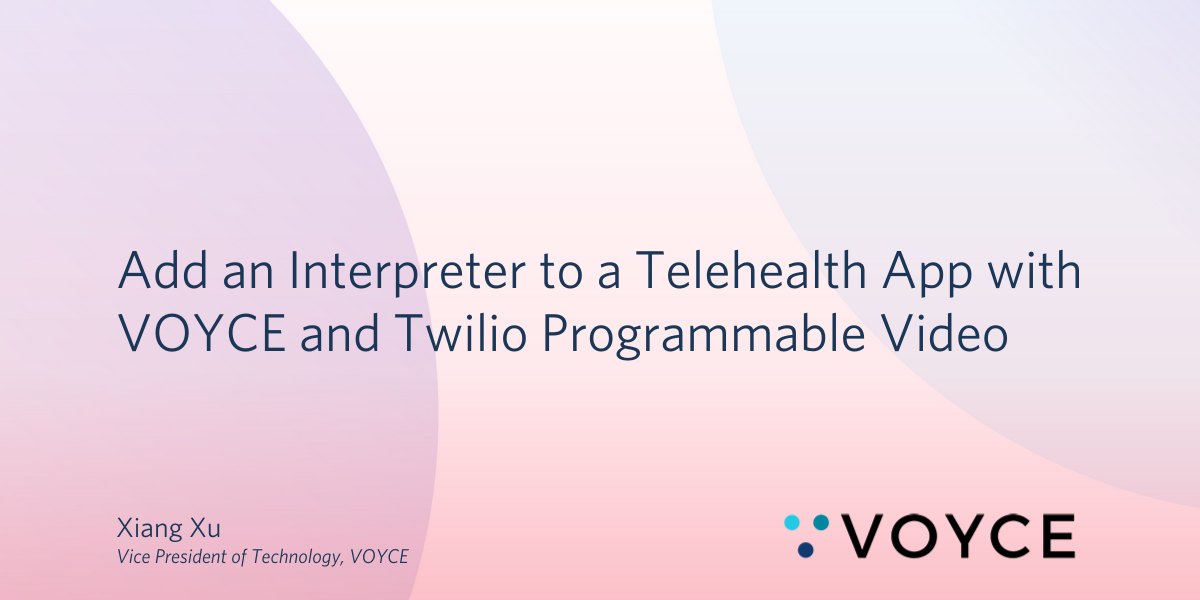
Xiang Xu from VOYCE joins us today for this guest post on how to add VOYCE’s interpreter capabilities to a telemedicine waiting room and video chat app.
VOYCE provides remote video interpretation services to the health care, legal, and business communities. Our professional, qualified, and medically trained interpreters service 220+ languages and dialects. Our platform can tightly integrate with Twilio Programmable Video and Programmable Voice.
This post will show you how to add VOYCE’s interpretation services to a Twilio Programmable Video telemedicine room. A medical provider can add an interpreter to the room with a single click of a button.
At a high level, here are the steps we’ll be following:
- Generate a Twilio Video token for a VOYCE interpreter
- Set up a basic front end user interface for the VOYCE service
- Build an integration with the VOYCE service related core APIs
If you’d like to skip straight to the code, the whole project is on GitHub.
Prerequisites
Before you can complete the build today, you'll need to have a VOYCE sandbox account and a telemedicine app built.
- Twilio video telemedicine waiting room constructed (follow the instructions inside before proceeding)
- A VOYCE Sandbox Account - Please contact us for a free one
Once you have your sandbox account and the telemedicine app is working correctly, you're ready to add the interpreter integration.
Add interpreter integration to the telemedicine app
Once you’ve finished setting up your telemedicine waiting room with Twilio Programmable Video, these steps will help you add the VOYCE interpretation service into your telemedicine waiting room.
Set up your environment with a VOYCEToken
You will have a .env
file already in the directory if you finish building the Twilio Video Room. Please add another variable, VOYCE_TOKEN
, into the file to store the VOYCE credentials for the back end.
You should have a file that looks something like this:
TWILIO_ACCOUNT_SID=ACXXXXXXXXXXXX
TWILIO_API_KEY=SKXXXXXXXXXXX
TWILIO_API_SECRET=XXXXXXXXXXXXXXXXX
VOYCE_TOKEN=XXXXXXXXXXXXXXXXX
Add an interpreter request button on the provider page
Let’s start with a front end change. Please copy the following code to replace the code currently in the provider.html
file.
Here, we import Twilio’s client-side Video SDK and the jQuery Library as well as the JavaScript file from the telemedicine app build. Then, we implement all the click handling functions for the buttons to do the right thing when the provider enters and leaves.
Different from the previous app, we also add a button to add an interpreter to the call. This we'll eventually wire up to VOYCE.
Add a VOYCE interpreter into the video room
This section will show you how to add the VOYCE functionality to index.js
, needed for the HTML file we just edited.
Open up public/index.js
and replace what’s in there with the code from the following blocks – I’ll explain what’s going on in turn. (You can also find the code in the repo).
Twilio room related setup
This section at the top of the file will set up the Twilio video room, and wire up the buttons with their advertised behavior.
In the code above, we implement functions including joining the room, and setting up a callback for a participant joining and leaving the video room. The user can join the video room by clicking the join button, and when other participants join the video room their audio and video track will be added to the web page.
VOYCE related setup
In the next step, we’ll add the interpretation service into our project. In the following code, we will generate a video token for VOYCE’s interpreter to join the video room and send the token, video room name and other related information to VOYCE API calls.
Paste this code after the code from the above section.
In addition to the things I described above the code block, we also implement a mute/unmute and a pause/resume function using Twilio’s SDK. This will let you keep your test clean and quiet.
Now that you have the code in index.js
, you can see the whole service lifestyle. These are the steps the app will work through:
- Set up a Twilio Programmable Video Room
- Generate a Twilio video token for the VOYCE interpreter
- Initialize a VOYCE request by sending a Twilio video token and the room name.
- Poll the VOYCE request status to display necessary information to the customer
- Finish the VOYCE request when you don’t need it anymore
(Optional) Understand the VOYCE API
Optionally, I'm going to explain how the VOYCE API works here. Feel free to move onto the next section if you're working through the implementation.
Every VOYCE API call needs to use https://www.voyceglobal.com/APITwilio
as a root url. You can check the detailed documentation via this link.
All VOYCE API calls require a VOYCEToken
parameter in the request headers. (You would have received everything you need for authentication with your sandbox account.)
Sample Header:
VOYCE Core Service Related APIs
In this section, I’m going to explain the 3 main API calls we’ll be using – one to invite a translator, one to get the call status, and one to finish a call (and cleanup).
Invite an interpreter to a room
POST Request/InviteWithoutLanguage
POST DATA:
RESPONSE DATA SAMPLE:
This call starts an interpretation request by inviting an interpreter to the room.
Check the status of an interpretation request
GET Request/StatusByPreInviteToken?PreInviteToken={PreInviteToken}
This is the API call to get the status of a service request.
Close a translation session
POST Request/FinishByPreInvite?PreInviteToken={PreInviteToken}
RESPONSE DATA SAMPLE:
This is the API call to finish a request when the connection should be concluded.
Add VOYCE API calls from the server
Now that you understand the VOYCE API, next let’s set up all the API calls from server.js
.
Open up server.js
and replace it with the following code (I’ll explain what’s going on in each block). As before, you can also find the code in the repo.
Twilio and boilerplate setup
In this section, we scaffold our server and add the necessary code for Twilio Programmable Video.
VOYCE API Setup
This section adds the code to make the calls described in the "Understand the VOYCE API" section. I'll cover each in turn – but in your server.js
, paste these blocks one after the other at the end of the file.
Invite an interpreter functionality
This code calls Request/InviteWithoutLanguage
, which will kick off inviting an interpreter to the room.
Check interpreter session status
This code calls Request/StatusByPreInviteToken
to check on interpreter status.
Close an interpreter session
Finally, here is the code to call Request/FinishByPreInvite
and close out a session.
And that's all you need! Now let's try it out and see how everything comes together.
Try out your new translation service
Ready to try it all out and see it working?
Navigate to http://localhost:1337/provider in your browser and click the “Join Room” button:
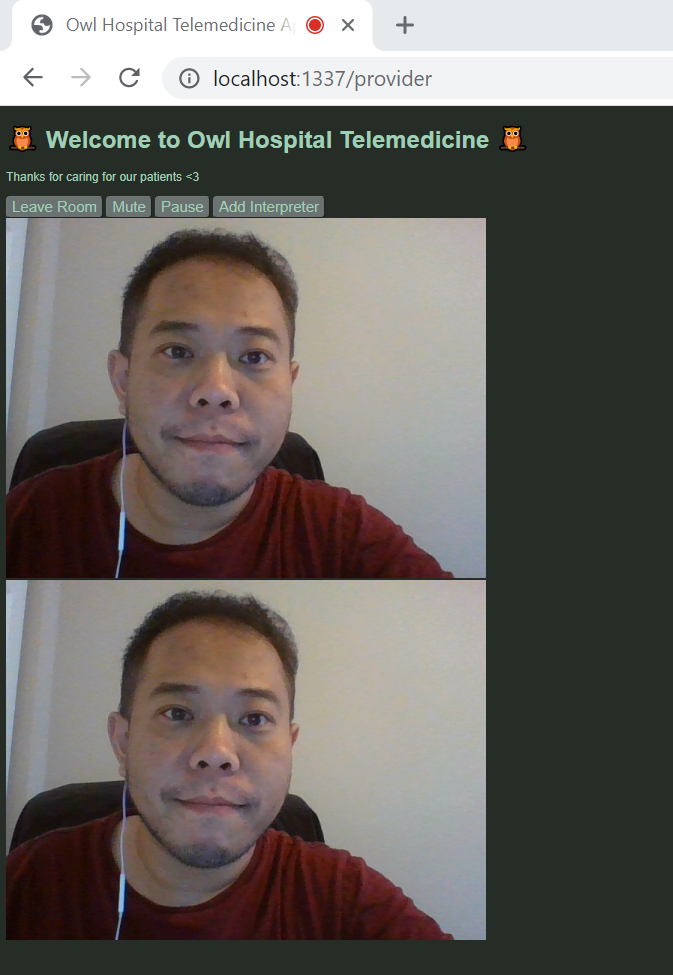
Then open a new tab to http://localhost:1337/patient in your browser and click the “Join Room” button:
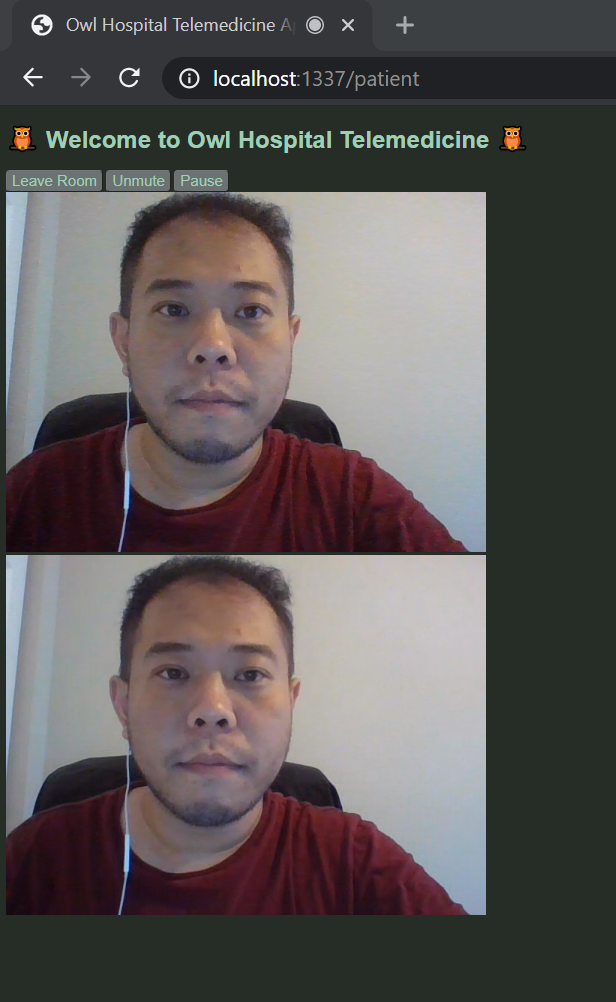
Then, open the interpreter portal in a new tab: https://www.voyceglobal.com/providerstaging/?Company=TwilioSandbox.
Next, click the add interpreter button on the provider page. A new window will open. Please follow the instructions:
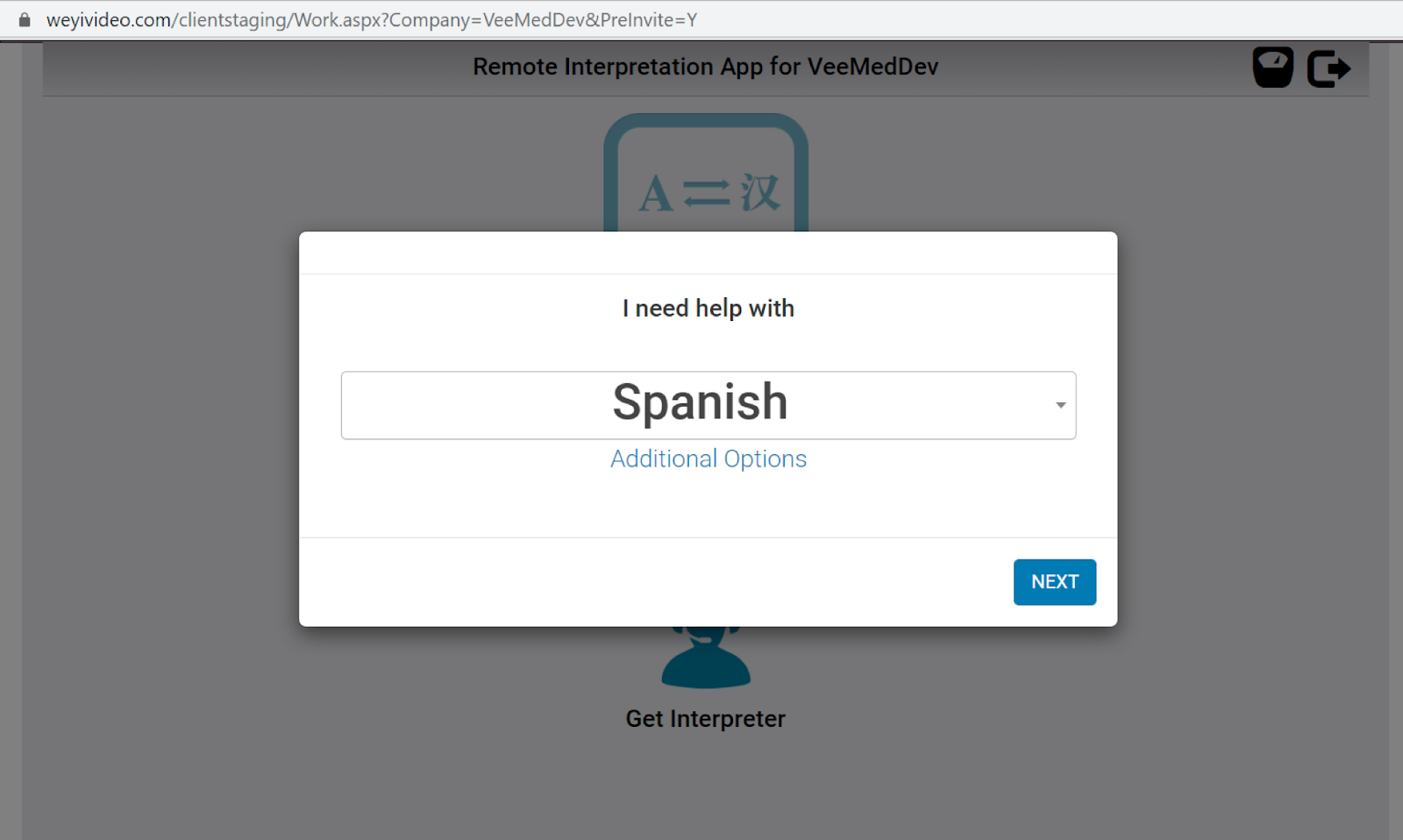
You will receive a new request in the interpreter portal. Click the accept button to join the service, then you will be able to see that all three parties are all in the room.
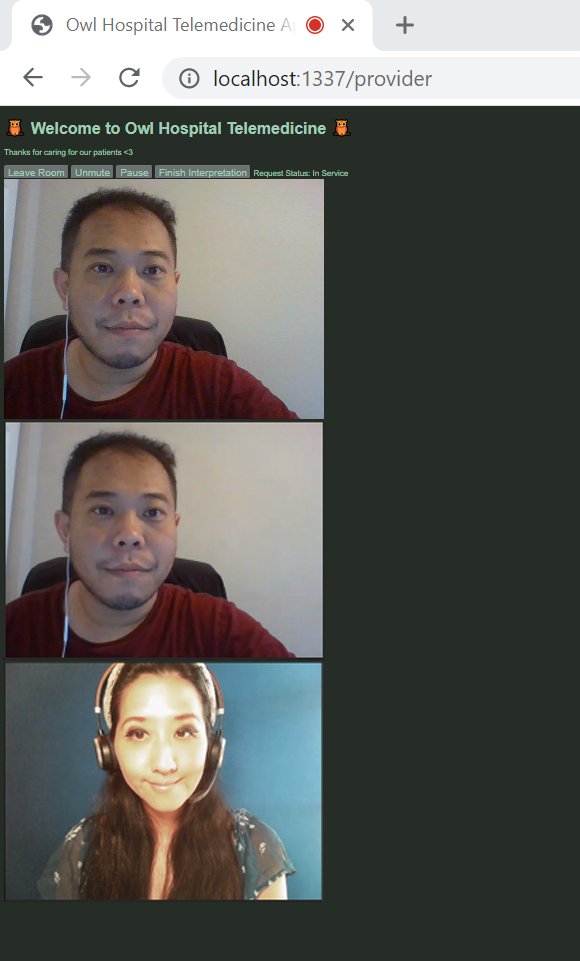
And, congratulations, you've now added translation services to a telemedicine app using VOYCE and Twilio Programmable Video. You're now ready to start productizing your app!
Adding a translation service to a Twilio Programmable Video telehealth app
Let’s review what we’ve learned today:
- How to add a VOYCE interpretation service
- How to show the VOYCE request status on a page
- How to show a remote participant’s audio and video elements on a page
- How to show and hide elements on the page when participants enter and leave a video room
This sample code to add a VOYCE interpretation service is admittedly pretty basic. There are so many add-ons you could imagine to make it truly innovative and awesome, such as:
- You could design a better looking interpretation related UI of the service options for your customers
- Design and implement the process to request and end the interpretation service request and provide a simple and better user experience
But either way, you've got the makings of a great telemedicine app. Let us know how you expand it!
Xiang Xu is the Vice President of Technology at VOYCE. He's currently working on improvements to provide more professional, stable, and better quality interpretation services to our customers. To discuss a partnership with VOYCE for interpretation services, reach out to us on our site.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.