How to: dotfiles
Time to read: 4 minutes
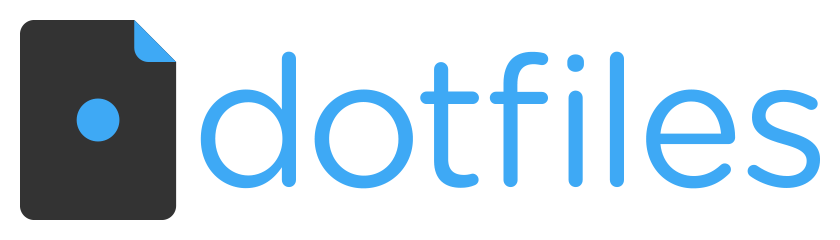
Want to learn how you can setup your workstation and start developing as quickly as possible? Want all of your configurations saved somewhere?
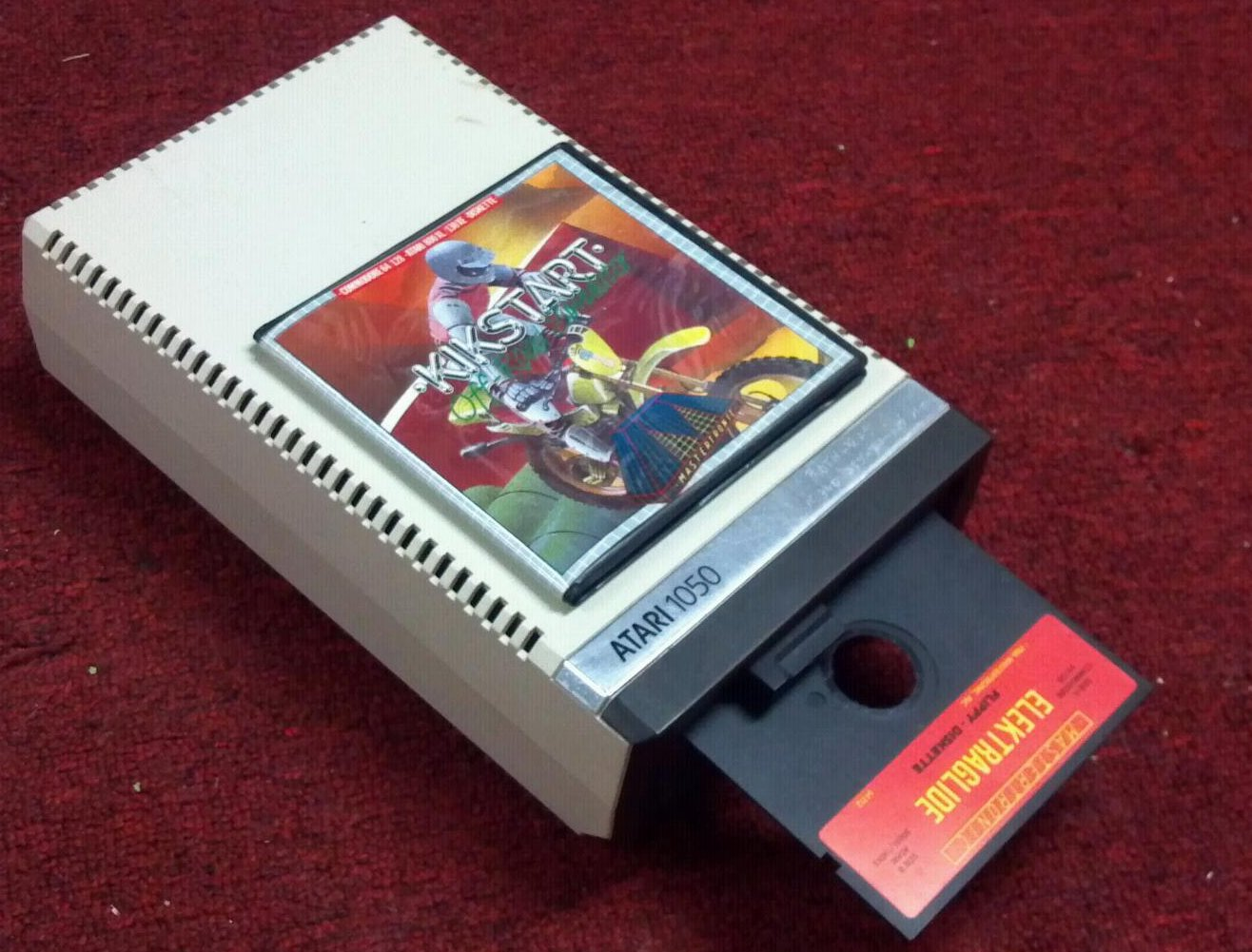
What are dotfiles?
Dotfiles are the colloquial name for a user's configuration files. Historically many of them started with a period, making them invisible in *nix environments.
These files preserve your favorite settings on different programs that you use on your system. If you get a brand new system (or your current system is wiped out), you can just pull your dotfiles from the cloud, a USB stick, or even a floppy disk and restore all of your applications, binaries, and configurations for important applications and tools. A perfect example for developers who use git is your ~/.gitconfig
:
If you do use version control: dotfiles that don’t have any sensitive information are great to commit to your own repo. Git is great for tracking system changes, just like it’s great for tracking code. As you go about your work, you can update your dotfiles and be ready to reinstall your system at a moments notice.
Dotfiles are great for teams as well, who work on many of the same projects and require many of the same tools. A team dotfiles repository can be created with member forks that contain personal configurations.
With shared dotfiles, we can draw the owl once, and everyone can benefit.
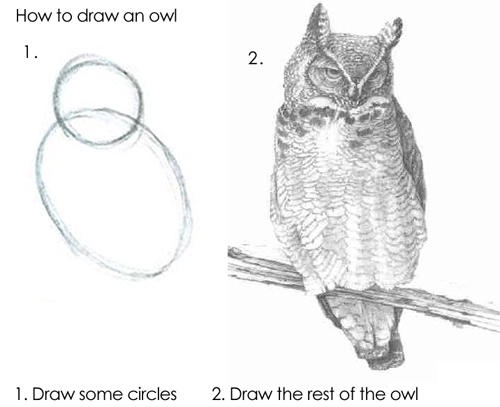
How can you create useful dotfiles?
You can:
- Find templates/ideas from other peoples dotfiles: https://tinyurl.com/yypxf5g3
- Or fork my dotfiles: https://github.com/earlonrails/dotfiles
- Start tracking the changes to your own dotfiles
Once you have a place to start, look through the template that you have chosen and get familiar with it.
What does the install script do? The install script should be something like: source install.sh
or ruby install.rb
.
Let's break down the install script we use for the API team at Twilio (run using ruby install.rb
) looks like this:
First we import some gems. The first is readline
, which will help us get the username. With ERB we can have some templates for files that we will customize (now we can put their username in some files).
Here we are prompting the user for their username. We could ask if they want certain packages installed that are optional, or what their favorite color is to theme their terminal.
Setup some constants for use later in the script:
Here is the super fancy erb
rendering, where we can put their username and email in their gitconfig
and use the username for the HOME
name for log rotate setup on Mac.
Check if the user responded yes to questions that you asked about:
Setup a clone repo
function to help clone many repos later:
It is good to check if things are installed, either by checking if the directory or file exists (like we do here) or by checking if a command exists.
Here we update Homebrew and then call brew bundle
which will use the Brewfile to install all the taps, casks, binaries and App Store applications we have specified:
To start contributing for your team, you need to create an SSH key and then paste it up on github. This will create your key and put it in your clipboard, then open Github to the page where you can paste your key.
Using asdf
we will install python
at a specific version and set it to the default.
Now, we will clone repos and run setup tasks on certain repos:
Finally, there is an optional step here where we take a file that is filled with different Mac defaults we would like to set.
For instance: defaults write com.apple.print.PrintingPrefs "Quit When Finished" -bool true
will quit the printer app when printing completes.
https://www.defaults-write.com/ is a good place to find things that you can customize. This macos file could be a dotfile, but it is only run once and only by this install script so lives in your dotfiles repo.
One thing that we do on the API team is make symbolic links to the files in the dotfiles directory to paths on our systems. This way you can edit the files where they live, and your changes will simultaneously be updated in your ~/.dotfiles
as well. This makes it easy to keep track of what has changed as well as commit new changes.
Useful tools to compliment dotfiles
Another thing you’ll like is the Brewfile
. (I learned about the Brewfile
recently myself!) Before Brewfile
I was using Ansible, which has a Homebrew task.
The thing I like about the Brewfile
is how easy it is to update: brew bundle dump
will create a new Brewfile
based on the things that you have already brew install
ed!
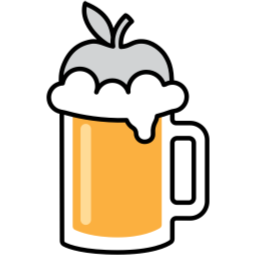
Next we have asdf
, another thing that I recently discovered. Many times I’ve thought: “Why do I have rvm
, gvm
, nvm
, pyenv
... Why isn’t there just one version manager to rule them all?”
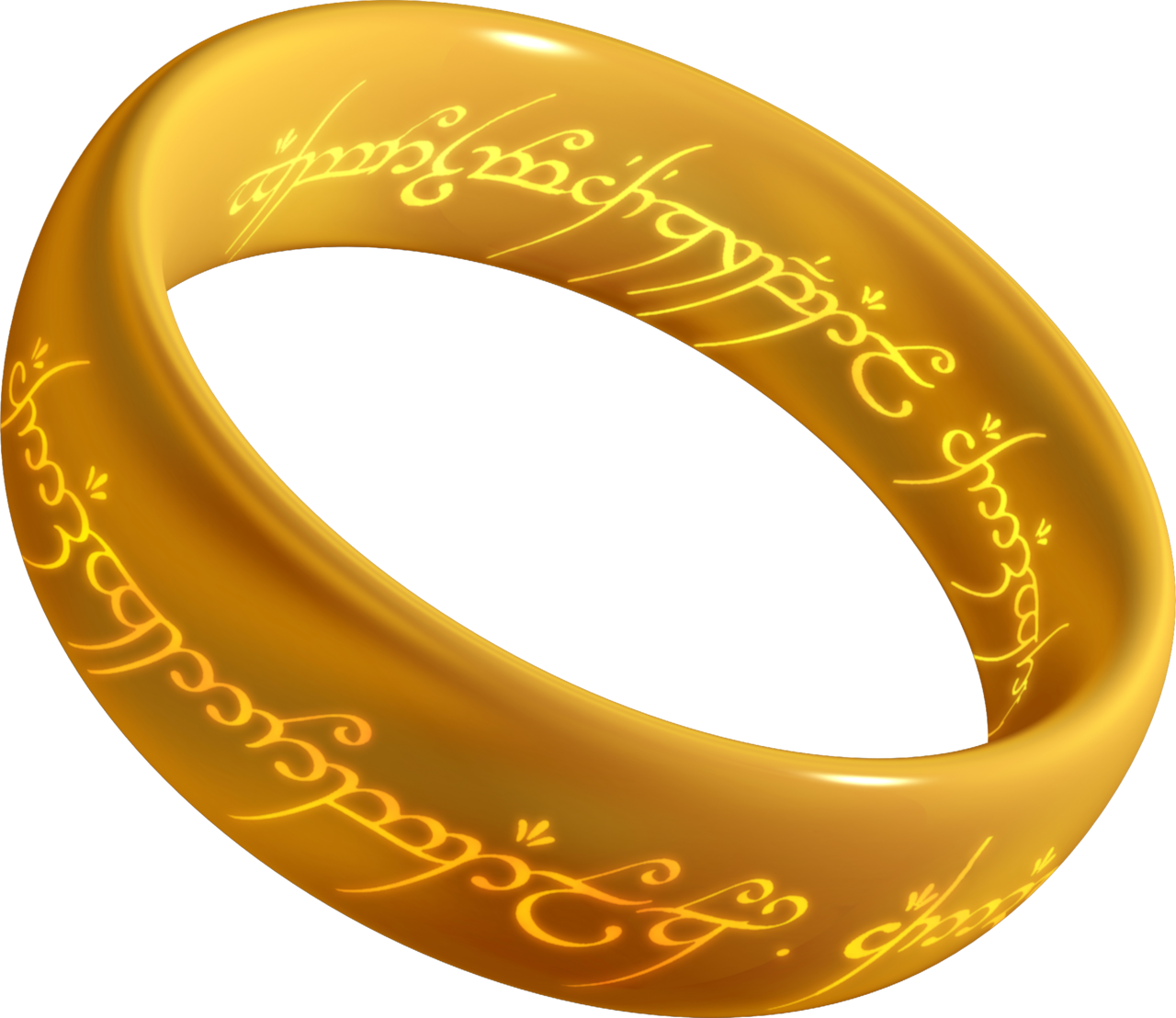
After a tireless journey through distant lands, I'm happy to report that such a thing exists! The one ring: asdf
With asdf
, I can install all the language and version combinations I want, in the same way. No longer will I lose my mind figuring out how to install X-language at version Y while managing my path.
Finally, we simply create an SSH key for the user, copy it to their clipboard, and open Chrome with the url where they can paste their key. After that we clone some repos (foo
, bar
, and baz
in our example) and perform make install
on those repos, which does the rest of the job of making sure each program has all its dependencies and is ready to run.
The cool thing is if you have complex setup tasks, replicating the same environment across multiple different workstations is straightforward.
Conclusion: start to use dotfiles
Dotfiles are awesome and everyone should use them. They help simplify your setup, make team collaboration easier, and recover from computer problems. For a similar rant (and to see where my dotfile journey began) visit https://driesvints.com/blog/getting-started-with-dotfiles/
Kevin Krauss is an engineer on Twilio's API team and is currently working on setting up edge services for websockets. He enjoys fishing and foraging. If you have any questions, feel free to contact him kkrauss@twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.