Using the Twilio REST API in Windows 8 Metro-style applications
Time to read: 5 minutes
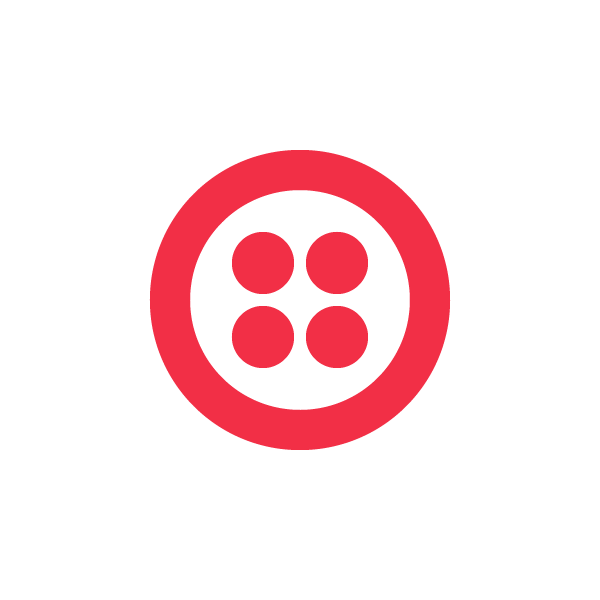
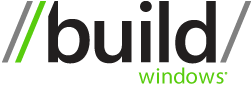
What about twilio-csharp?
WinRT uses a subset of .NET Framework 4.5 that doesn’t include some of the dependencies our current twilio-csharp library requires. Because of this you are not able to use it within a Metro-style Windows 8 app. We’re working on adding support for Metro-style apps in a future release but for now, we’ll use the new System.Net.Http namespace and classes to make our requests to the Twilio REST API.
Getting Started
The heart of our request revolves around System.Net.Http.HttpClient, a new and improved replacement for HttpWebRequest with a simple API and full async support. In the example below we’ll use HttpClient to make our POST requests needed to send a message.
If you haven’t yet, download and install the Windows 8 developer preview. Once installed, open Visual Studio and create a new Visual C# Windows Metro Style Application project.
Open up the MainPage.xaml.cs and create a method with parameters for the number to send from, the number to send the message to and the contents of the message. Lastly, create a new instance of an HttpClient so we can configure it to make the request. Here’s what we have so far:
Next, because Twilio uses HTTP Basic authentication on calls to our API, you need to add authentication headers to the HttpClient. The new System.Net.Http.Headers.AuthorizationHeaderValue class gives you a clean way to represent the different HTTP authentication headers and exposes a simple constructor that accepts the authorization scheme authorization value.
Create a separate method to encapsulate the creation of the AuthenticationHeaderValue class and return the instance.
Once the AuthenticationHeaderValue instance is returned from the CreateBasicAuthenticationHeader method, assign it to the HttpClient instance. The client exposes a property called DefaultRequestHeaders which represents the default set of headers included with the request, including the Authorization header.
Next, create the actual content to POST to the API. Sending an SMS requires three parameters: a From number, a To number and the Body. To send the content the HttpContent requires you to encapsulate content into a type derived from the HttpContent object, so for simple string content, you can create a instance of the StringContent class and pass it the assembled POST request body.
Note that by default the StringContent object will send its content with a Content-Type of text/plain, but you need to let Twilio know that is actually form data. To do that you need to set the StringContent object’s ContentType property.
Once the StringContent object is created and its Content-Type set, you’re ready to make the POST request to the API which is done by calling the Post method of the HttpClient with the URL to post to and the content to include. The fully assembled method is shown below:
The Post method returns a HttpResponseMessage object which contains a number of useful properties, including the IsSuccessStatusCode property, which will tell you if the request succeeded (a status code from 200-299 was returned) or failed (any other status code was returned).
The HttpResponseMessage.Content property contains the data the REST API sends back, which for successful requests will be the XML representation of the message we just created. You can easily parse this response with LINQ to XML.
To use the method in an app, you simply call the SendMessage method from somewhere in your app:
Going Async
One of the major aspects of the experience of Metro style apps in Windows 8 is the requirement that they remain responsive to the end user. To help you create apps that don’t lock the UI, Microsoft has added new asynchronous programming features to .NET.
To leverage these new features in the SendMessage method, you need to make a few simple modifications. First decorate the method with the new async keyword and change the method to return a Task<HttpMessageResult>. Next change the Post method call to use the PostAsync method and decorate that call with the await keyword.
Now when the app is run the request will be made asynchronously, keeping the UI nice and responsive. The remaining code in the method will continue to run once the asynchronous Post method call completes.
Build Your Own App
Sending SMS is just the start of what you can do with the Twilio REST API. Now that you’re armed with the skills to integrate Twilio into your application, enter our developer contest for a chance to win a Samsung Windows 8 Developer Preview tablet. Hurry, the contest ends this Sunday October 1st at 11:59pm PT!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.