Using Twilio with Classic ASP and VBScript
Time to read: 2 minutes
April 14, 2010
Written by
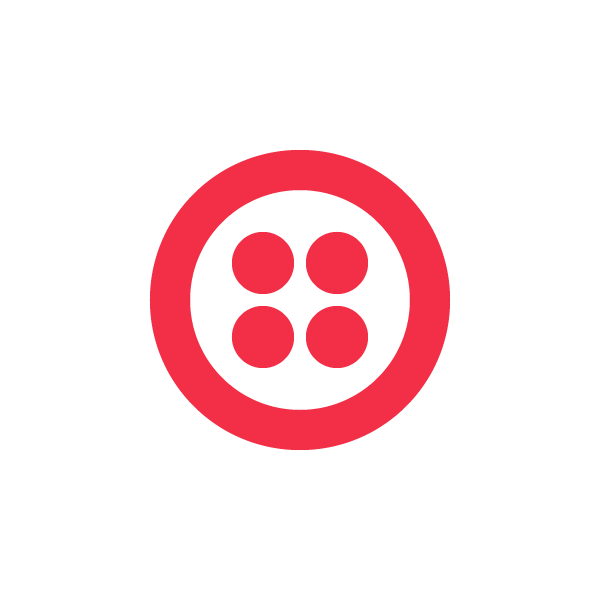
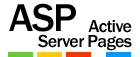
We’ve recently had an influx of requests for examples showing how to use Twilio with Classic ASP and VBScript. Here are two short examples to demonstrate how to initiate call and send a text message. You can download both files here.
Placing a Call via the REST API with Classic ASP
Let’s start off with an example for placing a call via the REST API. Initiating a call requires making a simple POST request. Let’s take a look at the code to make that happen.
<%
' replace with your account's settings
' available in the Dashboard
accountSid = "ACxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
authToken = "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
' setup the URL
baseUrl = "https://api.twilio.com"
callUrl = baseUrl & "/2008-08-01/Accounts/" & accountSid & "/Calls"
' setup the request and authorization
Set http = Server.CreateObject("MSXML2.ServerXMLHTTP")
http.open "POST", callUrl, False, accountSid, authToken
http.setRequestHeader "Content-Type", "application/x-www-form-urlencoded"
' call parameters
caller = "your-number-here" ' the number to call from
called = "recipient-number-here" ' the number to dial
url = "call-handler-url-here" ' URL handler for the call, should return TwiML
postData = "Caller=" & Server.URLEncode(caller)
postData = postData & "&Called=" & Server.URLEncode(called)
postData = postData & "&Url=" & Server.URLEncode(url)
' send the POST data
http.send postData
' optionally write out the response if you need to check if it worked
' Response.Write http.responseText
' clean up
Set http = Nothing
%>
Sending an SMS text message via the REST API
Sending an SMS is straightforward as well:
<%
' replace with your account's settings
' available in the Dashboard
accountSid = "ACxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
authToken = "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
' setup the URL
baseUrl = "https://api.twilio.com"
smsUrl = baseUrl & "/2008-08-01/Accounts/" & accountSid & "/SMS/Messages"
' setup the request and authorization
Set http = Server.CreateObject("MSXML2.ServerXMLHTTP")
http.open "POST", smsUrl, False, accountSid, authToken
http.setRequestHeader "Content-Type", "application/x-www-form-urlencoded"
' message parameters
from = "your-number-here" ' the number to send the message from
recipient = "recipient-number-here" ' the number to send the message to
body = "Sending SMS is easy with Twilio!" ' message contents
postData = "From=" & Server.URLEncode(from)
postData = postData & "&To=" & Server.URLEncode(recipient)
postData = postData & "&Body=" & Server.URLEncode(body)
' send the POST data
http.send postData
' optionally write out the response if you need to check if it worked
' Response.Write http.responseText
' clean up
Set http = Nothing
%>
There you have it! If you have any questions about these examples, head on over to our forums or send us an email at help@twilio.com and we’ll be glad to help!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.