How to View Twilio SMS Notifications on Your Desktop with Python and FastAPI
Time to read: 4 minutes
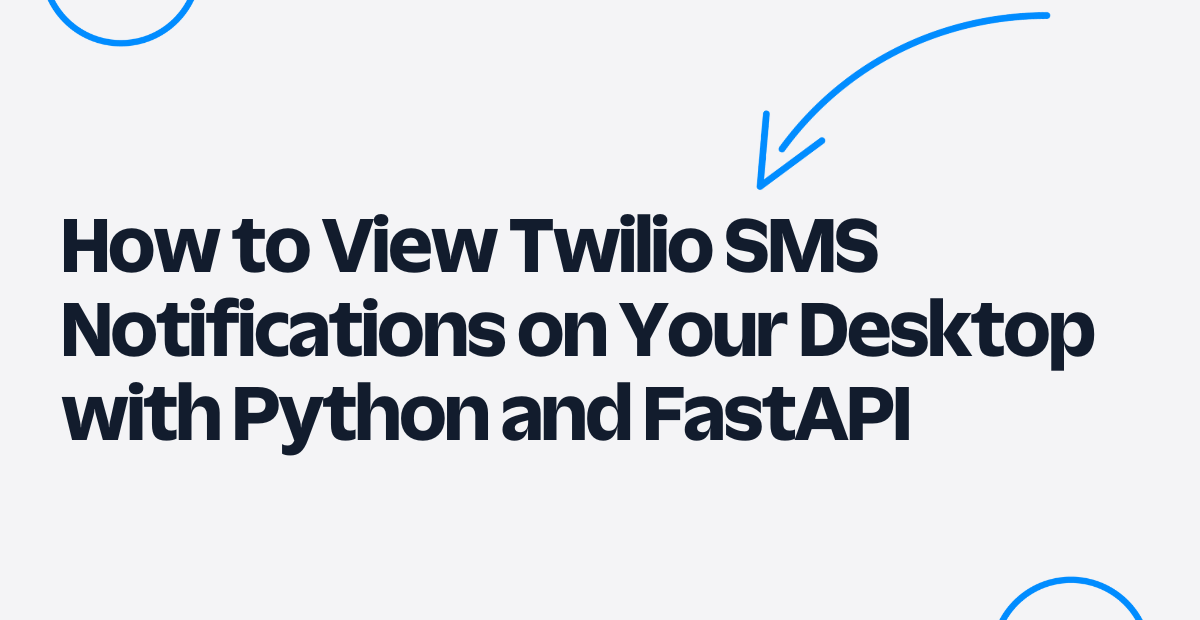
If you are building a project with Twilio SMS, there are times when it would be convenient to see the messages that come in on your Twilio number as desktop notifications. For example, if you are tracking a package or a food delivery, you may want to see these notifications appear without having to look away from your device.
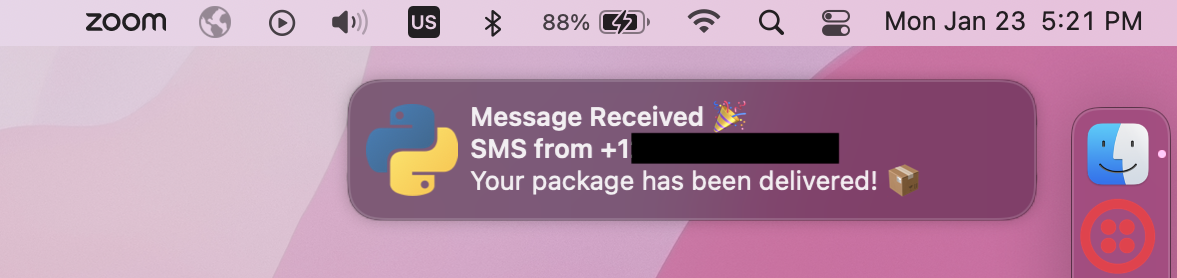
In this short tutorial, you will learn a quick way to use Python and FastAPI to create a tiny app that will display the messages you receive to your Twilio number as notifications on your desktop — in real time.
If you are looking for a quick and efficient way to manage your SMS notifications, then this tutorial is for you.
Let's get started!
Prerequisites
- A Twilio account (sign up for free here)
- A Twilio phone number with SMS capabilities (learn how to buy a phone number here)
- Python 3.7 or newer
- ngrok installed and set up on your machine (learn more about using ngrok here)
- A phone that can send SMS
- A laptop or desktop computer
Set up a virtual environment and install dependencies
To start, open a new terminal window and navigate to where you would like to set up your project. Once you have done this, run the following commands to set up a new directory called desktop_notifications and change into the directory:
Next, you'll need to create and activate a Python virtual environment. There, you can install the dependencies for this project. If you are working on a macOS or UNIX machine, you can do this by running the following commands:
However, if you are working on Windows, run these commands instead:
For this project, you will be using the following Python modules:
- FastAPI, to create the web application
- Uvicorn, to run the application
- notify-py, a cross-platform desktop notifications library
- python-multipart, to work with incoming data from the request body
Run the following command in your terminal to install these modules in your virtual environment:
Now you're ready to start building your FastAPI application.
Build the FastAPI application
To build this tiny application, you'll only need to create one file: main.py. Create and open this new file now, at the root of your project. Then, add the following code to the file:
In the code above, you create a new web application with FastAPI, and then set up a /notify
route that will receive POST requests to the /notify
endpoint. When a POST request is made to the /notify
endpoint, your application will call the notify()
function, which creates a new notification using notify-py, sets that notification's name, title, and message, and then sends that notification to appear on your desktop. The phone number that the SMS was sent from (From
) will appear in the notification's title, while the Body
of the received SMS will become the body of the notification.
Run the server and start ngrok
To start your FastAPI application, run the following command from the terminal window within the activated virtual environment:
Once the application is running, you will see log statements like the following printed to the terminal, letting you know that the server is running on port 8000, and all is working as expected:
Now that the server is running, it's time to run ngrok as well. In a separate terminal tab, start ngrok by running the following command:
The log output from ngrok will be similar to the following:
Take note of the URL next to Forwarding
, ending with .ngrok.io
. You will need this to configure the webhook on your Twilio phone number.
Set up the webhook with ngrok
Navigate to the Phone Numbers section of the Twilio Console. There, select the phone number you would like to use, and then scroll down to the Messaging section.
Copy the forwarding URL from ngrok and paste it into the Webhook field under A MESSAGE COMES IN, adding /notify
to the end of this URL. The full URL should look something like this: https://XXXX-XXX-XX-XXX-XX.ngrok.io/notify
. Make sure the request method is set to HTTP POST
.
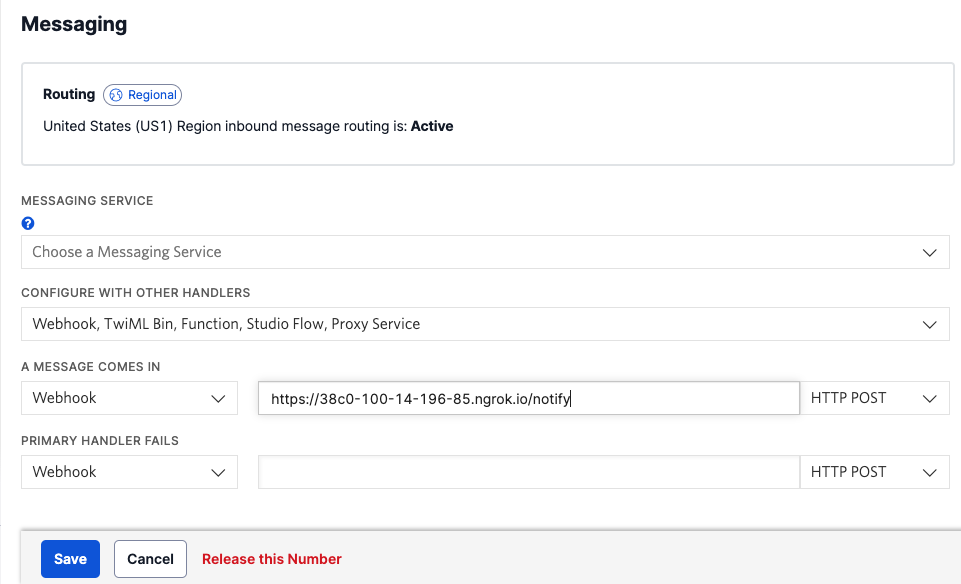
Click the Save button to save your changes. Now, every time someone sends an SMS to your Twilio number, your notifications application will receive a request to the /notify
endpoint and show you a desktop notification with the details about the SMS message you received.
Test it out
It's time to test the application. Try sending a message from your phone to your Twilio number. Maybe something like Your package has been delivered! 📦
.
You will see the notification appear on your desktop!
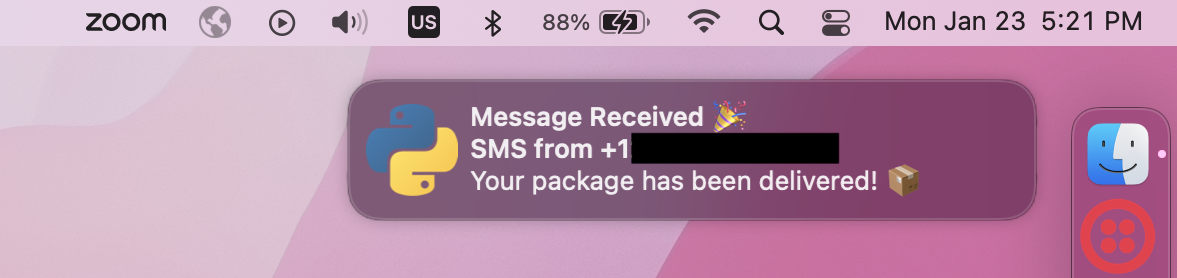
What's next for desktop notifications?
In this tutorial, you learned how to create a tiny application using Python and FastAPI, one that lets you see the SMS messages received to your Twilio number as desktop notifications.
As a next step, you could customize the notification further, perhaps changing its icon or making it play a sound when the notification appears. This project could also be a great jumping-off point for a fuller package-tracking app, or even for an application that sends SMS in addition to just reading them.
I can't wait to see what you build!
Mia Adjei is a Software Engineer for Technical Content at Twilio. They love to help developers build out new project ideas and discover aha moments. Mia can be reached at madjei [at] twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.