Instant Lead Alerts with Ruby and Rails
Time to read: 2 minutes
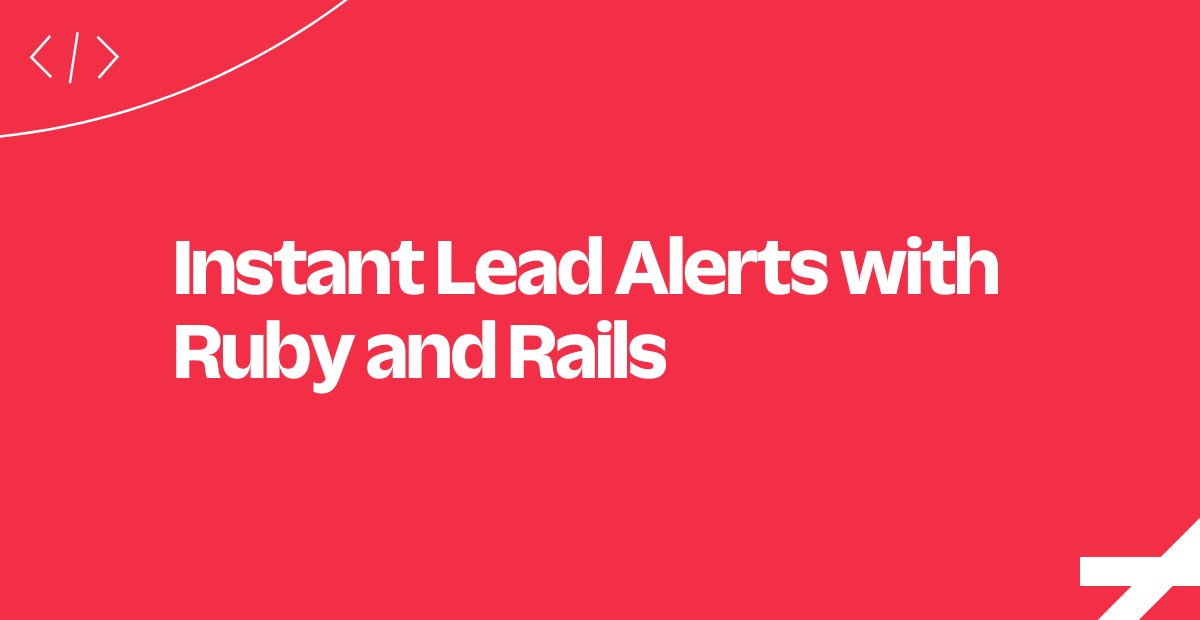
You probably already have landing pages or product detail views which you're using to generate some excellent leads for your business. Would you like to let the sales team know when you've got a new qualified lead?
In this tutorial, we'll use Twilio Programmable SMS in a Ruby on Rails application to send a message when a new lead is found.
In this example, we'll be implementing instant lead alerts for a fictional real estate agency.
We'll create a landing page for a house and notify a real estate agent the moment a potential customer requests information.
Let's see how it works!
Landing Page Data
To display a landing page for our fictional house we need some data to display to web surfers.
For demonstration purposes we've created a hard-coded hash containing the information we need.
Now that our route is ready, let's see how to render the Landing Page.
Render the Landing Page
In our HAML template we insert our hard coded data about the fictional house. We also add a form in the sidebar so the user can request more information and send in their contact info.
Now that our landing page is ready, let's see how to set up the Twilio REST Client.
Creating a Twilio REST API Client
Now we need to create a helper class with an authenticated Twilio REST API client that we can use anytime we need to send a text message.
We initialize it with our Twilio Account Credentials stored as environment variables. You can find the Auth Token and Account SID in the console:
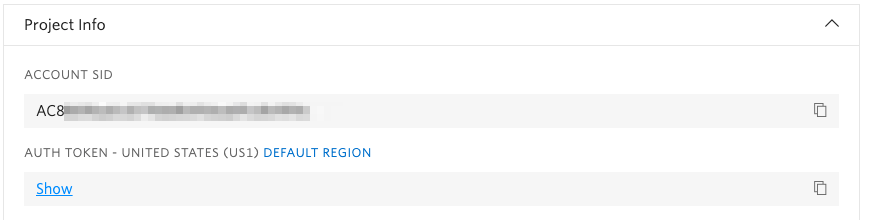
Now that our Twilio Client is ready, let's have a look at how to handle an incoming lead.
Handle the Lead POST Request
This code handles the HTTP POST
request issued by a user's form on our landing page. It uses our MessageSender
class to send an SMS message to the real estate agent's phone number, which is stored in an environment variable.
We include the lead's name, phone number, and inquiry directly in the body of the text message sent to the agent.
Now the agent has all the information they need to follow up on the lead.
That's it! We've just implemented an application to instantly route leads to sales people using text messages.
In the next pane, we'll look at some other easy to add features for your application.
Where to next?
Ruby, Rails, and Twilio - such a great combination! Here're a couple other great examples of integrating new features in a Ruby on Rails application:
Twilio Client allows your users to make and receive phone calls in their web browsers.
Call Tracking helps you measure the effectiveness of marketing campaigns.
Did this help?
Thanks for checking out this tutorial!
Tweet @twilio to let us know what you're building.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.