SMS and MMS Marketing Notifications with PHP and Laravel
Time to read: 4 minutes
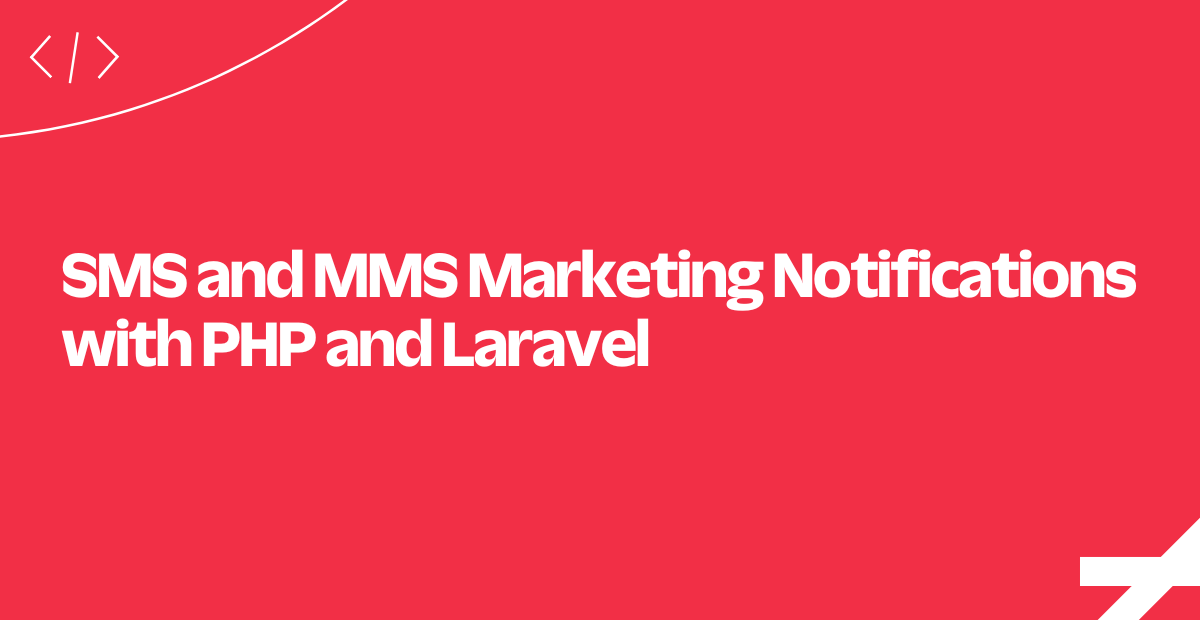
Ready to implement SMS and MMS marketing notifications? Because of the high read-rate and the always-present nature of mobile devices, marketing through SMS is an excellent choice. Today we'll build notifications with PHP and Laravel.
Here's how it'll work at a high level:
- A potential customer sends an SMS to a Twilio phone number you've advertised somewhere
- Your application confirms that the user wants to receive SMS and MMS notifications from you
- An administrator crafts a message in a web form to send to all subscribers
Basic Twilio building blocks
For this tutorial we'll be working with the following tools:
- TwiML and the <Message> Verb: We'll use TwiML, the Twilio Markup Lamguage to manage interactions initiated by the user via SMS.
- Messages Resource: We will use the Twilio REST API to send marketing messages out to all subscribers.
Let's get started!
Making the Subscriber class
In order to track who wants our marketing messages, let's start at the beginning and provide the right model:
phone_number
stores where to send the marketing messages.subscribed
is a boolean which tracks if aSubscriber
is opted in. Unsubscribed users will not receive messages.
We can use the command line to create the scaffolding for this model with a command similar to:
php artisan make:model Subscriber -m
Now that we have a model, let's create the migration.
Create a migration for the Subscriber model
To create the table where we’ll store subscriber info, we’ll create a migration and then execute it. First, create a basic migration file by running the command:
php artisan make:migration subscribers
Next, open the new file and add info on the fields we need this table to have. It will be located in the database/migrations
directory and named with the current timestamp and the table name we provide. For example, it might look something like 2018_02_01_012345_create_subscribers_table.php
Inside the file we’ll find the class CreateSubscribersTable which contains two methods: up()
and down()
for creating or destroying our table. We just need to edit the up()
method to add the phone_number
and subscribed
fields we need for our model.
Here's how the migration should look:
To create that table in our database, our database name, database user, and database password will need to be setup to match the local database installation in our Laravel project’s .env file.
Next, run the command:
php artisan migrate
... and the migration should be successful.
Simple, right? Next let's look at how to handle incoming messages from people opting into the service.
Handling incoming text messages in PHP and Laravel
Here we create the endpoint that Twilio calls every time our number receives an incoming message from a new subscriber.
It relies on a createMessage
method that produces the message that will be returned, resulting in a warm and fuzzy welcome message.
Next we'll show how the createMessage
method works.
Create a subscriber from an incoming message
We begin by getting the user's phone number from the incoming Twilio request. Then we try to find a Subscriber
with that phone number.
If there's no subscriber with this phone number, we create one, save it, and respond with a message asking them to text the word "add". By default, users won't be subscribed; users will need to confirm the subscription with an additional text.
To recap: we've created a Subscriber
model to keep track of the people that want our messages, and need to implement subscribing and opting-out.
Let's look at the subscribe/unsubscribe logic on the next pane.
Manage user subscriptions
We want to provide the user with two SMS commands to manage their subscription status: add
and remove
.
These commands will toggle a boolean flag for a Subscriber
record in the database and will control that customer's marketing message preference.
To make this happen, the controller logic handles incoming messages from known Subscribers
like this:
- If it is a
add
orremove
command, create/update their subscription with the right status in the database. - If it is a command we don't recognize, send them a message explaining available commands.
We'll visit the message creation form next.
Set up the business logic for marketing messages
From the site's frontend, we retrieve the message text (and/or image URL) first. Next we loop through all subscribers and call the sendMessage
method to send the message out.
When the messages are on their way, we redirect the submitter back to the notifications.create
route with a Flash Data message containing feedback about the messaging attempt.
Ready to see how we send SMS and MMS themselves?
Send SMS or MMS marketing messages
In the method sendMessage we create a Twilio REST API client that can be used to send either SMS or MMS messages.
The client requires your Twilio account credentials (an Account SID and Auth Token), which can be found in the Twilio Console:
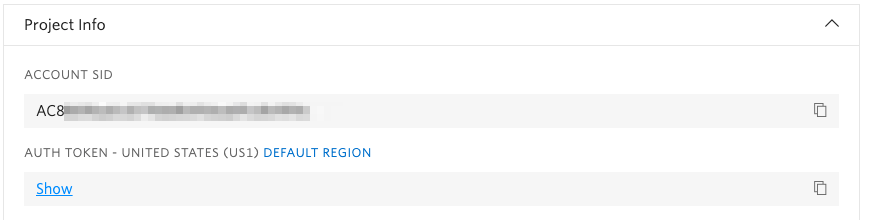
You'll want to set those in the .env file. (Additionally, you'll need to add a SMS-capable number there from the Phone Number console.) Once the app is running, then all we need to do is call create
on the client->messages
object in order to send our message.
The Twilio Message API call requires a to
parameter and an array containing a from
number, the message body
and an optional mediaUrl
.
And with that, the application is completely wired. Next up, we'll look at what other features we can easily add with Twilio and a little guidance from our tutorials.
Where to Next?
That's it! We've just implemented a an opt-in process and an administrative interface to run an SMS and MMS marketing campaign.
Now all you need is some killer content to share with your users via text or MMS (That's for you to find!).
PHP and Twilio are a great mix. Here are just a couple of other features we think you'll enjoy:
Easily route callers to the right people and information with an IVR (interactive voice response) system.
Instantly collect structured data from your users with a survey conducted over a voice call or SMS text messages.
Did this help?
If you have any feedback to share with us please tweet to us @twilio. We love to hear what you've built and what you're building!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.