Make Outbound Phone Calls with Delphi
Time to read: 2 minutes
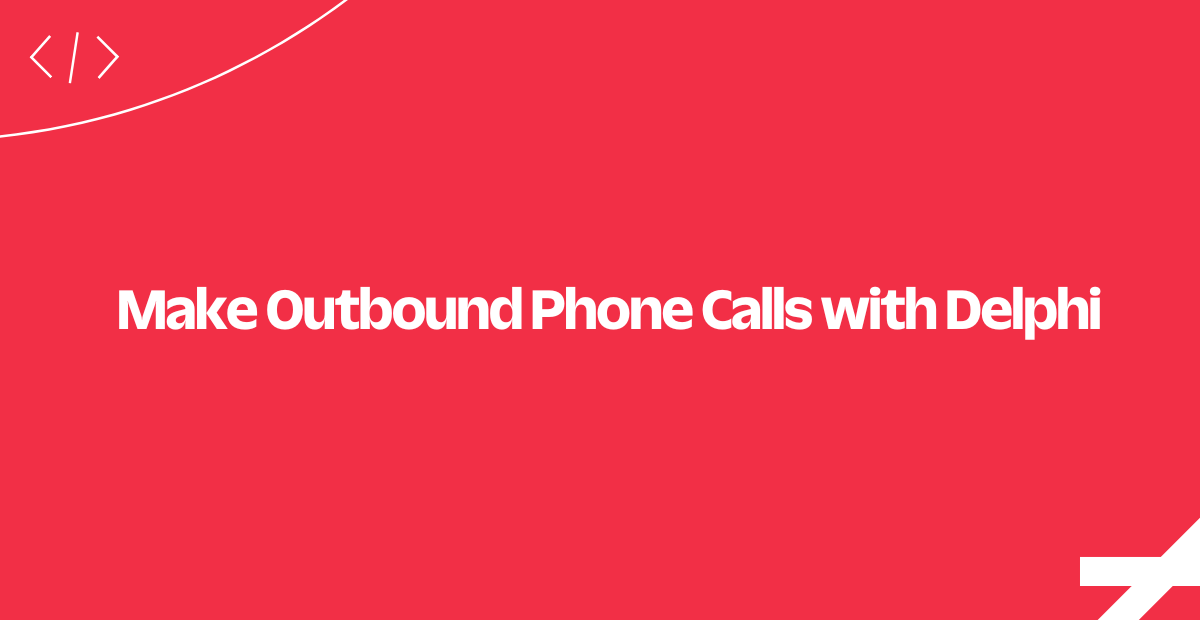
In this guide, we'll show you how to use Programmable Voice to make outbound phone calls from your Delphi applications. All you'll need is a voice-capable Twilio phone number, your account credentials, and five minutes to have a boatload of fun at your keyboard.
The code snippets in this guide were written and tested using Delphi 10.2.3. You can view the complete sample project on GitHub.
Let's get started!
In the Twilio console, search for and purchase an available phone number capable of making outbound calls. You'll use this phone number as the "From" phone number when you initiate an outbound call.
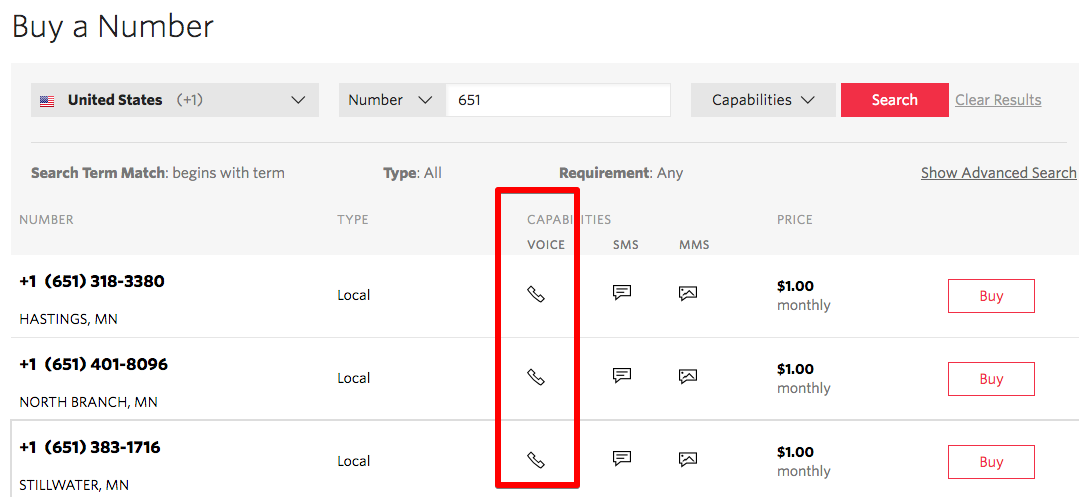
Retrieve your Twilio account credentials
First, you'll need to get your Twilio account credentials. They consist of your AccountSid and your Auth Token. They can be found on the home page of the console.
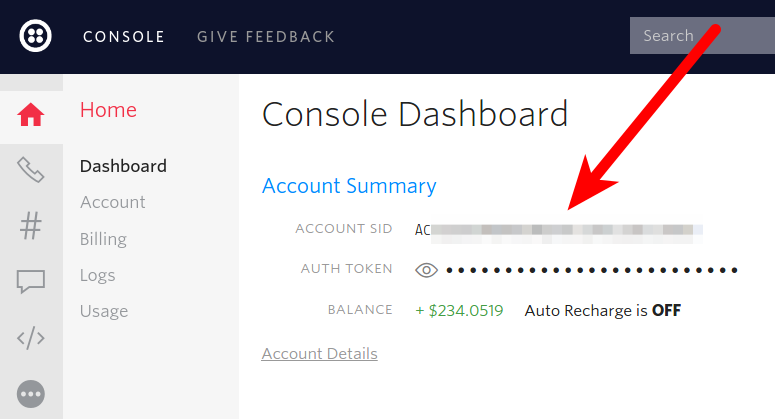
Make an outbound phone call
Now we're ready to make an outbound phone call using the provided TTwilioClient
.
There are a few key parameters to drill into when making the outbound call.
- "From" - the voice-enabled Twilio phone number you added to your account earlier
- "To" - the person you'd like to call
- "Twiml" - Instructions in the form TwiML that explains what should happen when the other party picks up the phone
- "Url" - Optionally, instead of passing the
Twiml
parameter, you can provide a Url that returns TwiML Voice instructions.
What is TwiML?
TwiML is the Twilio Markup Language, which is just to say that it's an XML document with special tags defined by Twilio to help you build your SMS and voice applications. TwiML is easier shown than explained. Here's some TwiML you might use to respond to an incoming phone call:
And here's some TwiML you might use to respond to an incoming SMS message:
Every TwiML document will have the root <Response> element and within that can contain one or more verbs. Verbs are actions you'd like Twilio to take, such as <Say> a greeting to a caller, or send an SMS <Message> in reply to an incoming message. For a full reference on everything you can do with TwiML, refer to our TwiML API Reference.
Of course, the TwiML you use to make the outbound call doesn't need to be a static file like in this example. Server-side code that you control can dynamically render TwiML to use for the outbound call. Check out our inbound call guide to see an example of an ASP.NET server which generates TwiML.
Happy hacking!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.