Generate an AI Competition Report with Exa, Anthropic, and Twilio SendGrid
Time to read: 3 minutes
Generate an AI Competition Report with Exa, Anthropic, and Twilio SendGrid
At an AI hackathon recently, my team worked on this project where a user inputs their startup idea and email to a Streamlit web app and then they receive an AI-generated competition report. Read on to learn how Sarah Chieng , Abel Regalado , and I built it using Exa , Anthropic , and Twilio SendGrid in Python!
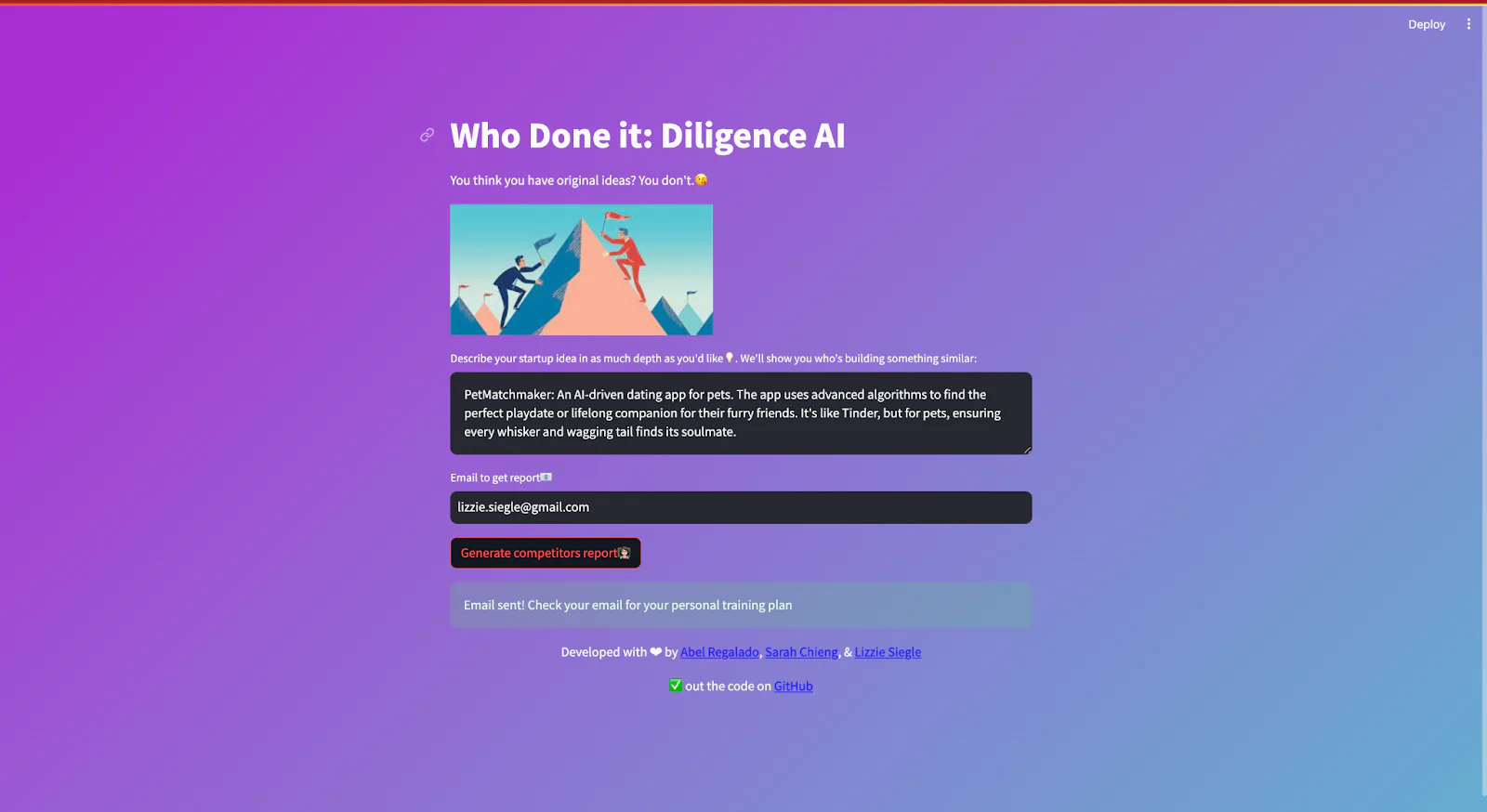
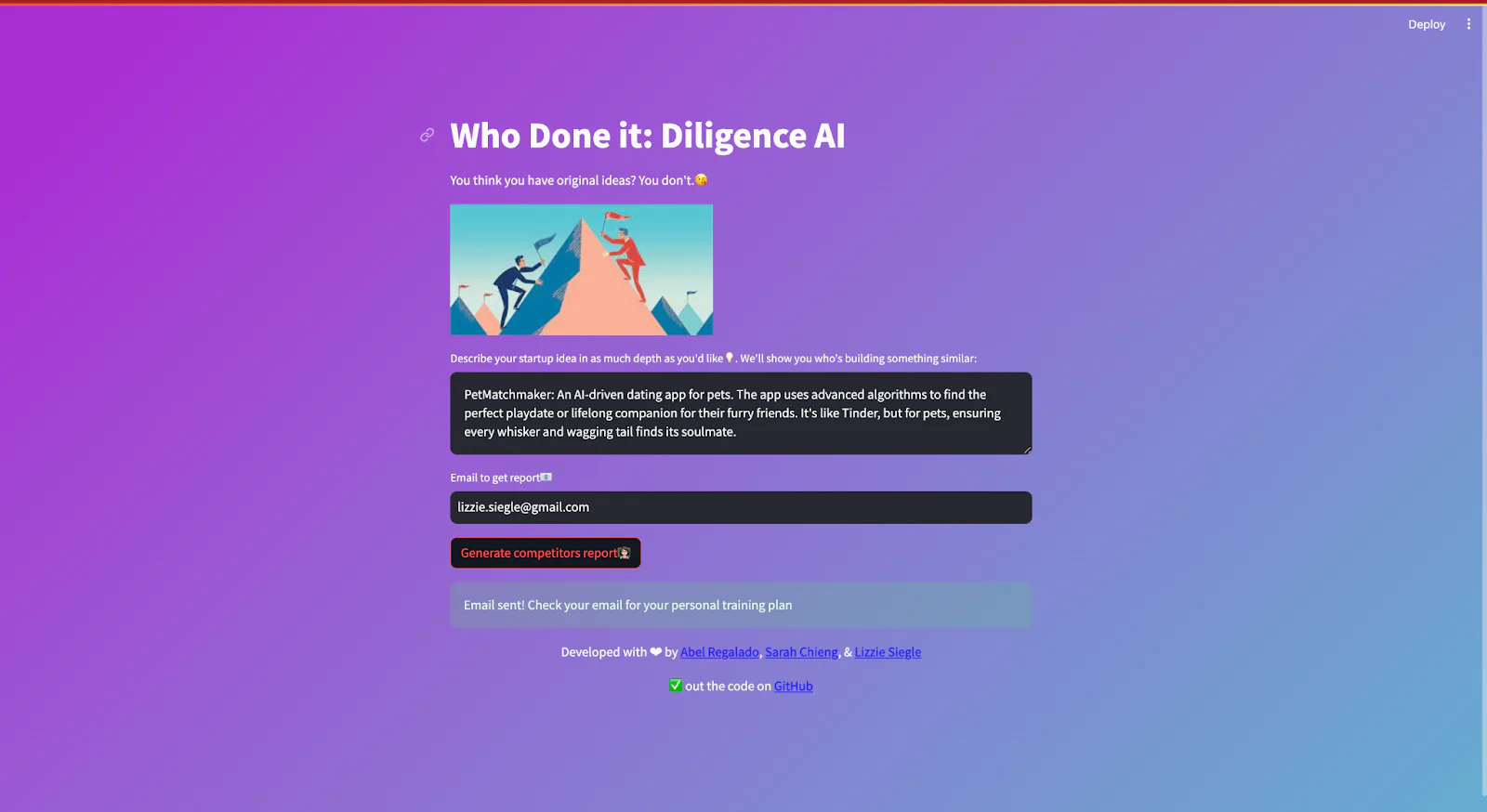
Prerequisites
- Twilio SendGrid account to send an email - make an account here and make an API key here
- An email address to test out this project
- Anthropic account to use their Completions API - get on the waitlist to use their APIs here so you can make your API key her e
- Python installed - download Python here
- Exa account to crawl the web via an API - make an account here and grab an API key here
Configuration
Since you will be installing some Python packages for this project, you need to make a new project directory and a virtual environment .
If you're using a Unix or macOS system, open a terminal and enter the following commands:
If you're following this tutorial on Windows, enter the following commands in a command prompt window:
Now it's time to dig into some code!
In that directory, make a file called app.py. Include the following import statements:
On the command line, make a new folder called css. In that folder, make a file called style.css containing the following code:
In app.py, add the following lines beneath import streamlit as st to use that CSS code:
Make a .env file and include the following, replacing the values with your API keys:
Access those API keys with the following Python code that creates an Exa object, sets the example_idea variable, and adds a title, subtext, and an image (which you can get from GitHub here ) to the Streamlit app:
Create a Streamlit text area and text input so the user can input their startup idea and email:
Generate Competitors with Exa Helper Function
Next, add this helper function to perform a snippet search for competitors relating to an input string (a user's startup idea).
If a user clicks the Generate competitors report button, a spinner is displayed and the find_competitors function is called on the user input idea. We loop through the output generated by Exa, parsing it for company title, URL, and a snippet about the related company from the web. This is added to an array competitors.
Pass Data to Anthropic to Generate Report
Craft a prompt to pass to Anthropic's Claude 2.1 model.
“HUMAN_PROMPT” and “AI_PROMPT” are strings to craft the correct prompt structure for the generation pipeline. They surround the "PROMPT" which includes the data generated by Exa–that's RAG by Exa!
We call Anthropic's completions API, passing it all the prompts.
Combine the introduction, report, and conclusion, and then send it as an email with Twilio SendGrid in Python!
If the email is sent, a message is shown in the Streamliti web app telling the user to check their email for the report. Otherwise, an error message is displayed.
Lastly, add a footer to the webapp that also links to the GitHub repo.
The complete code can be found here on GitHub !
What's Next for AI-generated Content with Twilio SendGrid?
Email is the perfect medium for long-form AI-generated content. You can do so much more with Anthropic, Exa, and Twilio SendGrid, like searching the web and summarizing relevant web pages, news articles, research papers, and more.
Developers can use Exa to perform Retrieval Augmented Generation (RAG) and then question-answering (QA) on podcasts, lectures, interviews, and so many other web pages and articles. I can't wait to see what you build–let me know online what you're working on with AI!
Let me know online what you're building!
- Twitter: @lizziepika
- GitHub: elizabethsiegle
- Email: lsiegle@twilio.com
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.